5 types:
js should be camelCase
number, string, boolean, null and undefined
eg: for string:
when in console typing:
"hi " + "goodbye" => "hi goodbye"
the length of string: String.length (0 based)
var name = "jaq";
name = "sl";
var number = 73;
var isRight = true;
javascript can change the value type of variable
var n = "jaq";
n = 15
undefined type:
var age; // this is undefined
Test in console
clear() // remove the history
alert("Hello there!");
console.log("Hello from the console!")
prompt("What is your name?") // this will pop up a dialogue and a
// place for you to type in sth
var userName = prompt("What is your name?")
connect js with html
Script Demo
more examples:
var firstName = prompt("what is your first name?");
var lastName = prompt("what is your last name?");
var age = prompt("what is your age?");
console.log("Your full name is " + firstName + " " + lastName)
Equality Operators
== performs type operation, === is not
var x = 99;
x == "99" // true
x === "99" // false
"" is empty string
false values:
fase 0 "" null undefined NaN
loop in js:
var count = 1;
while(cnt < 6) {
console.log("count is: " + count);
count++
}
the function of string type
var str = "hello";
str.indexOf("w");
str.charAt(0).toUpperCase() + str.slice(1);
function in js
function singSong(length, width) {
console.log(length * width);
return "how is you?"
}
js arrays:
var friends = ["Charlie", "Liz", "David", "Mattias"]
friends[4] = "Amelie";
Arrays Last few things
var friends = []; // no friends
var friends = new Array() // uncommon
// arrays can hold any type of data
var random_array = [49, true, "Herm", null];
// can also directly add new data
var friends = ["Charlie", "a", "c"];
friends[4] = "d";
Push and Pop of array
var colors = ["a", "b", "c"];
colors.push("d");
Use pop to remove the last item in an array
var colors = ["r", "o", "y"];
var col = colors.pop();
unshift: add to the front of an array
var colors = ["r", "o", "y"];
colors.unshift("in")
// ["in", "r", "o", "y"]
shift: remove the first item in an array
colors.shift(); // ["r", "o", "y"]
use indexOf() to find the index of an item in an array
use slice() to copy parts of an array
var fruits = [1, 2, 3];
var otherNums = nums.slice(a, b);
// a included, b not included
For loop
var colors = ["a", "b", "c"];
for(var i = 0; i< colors.length; i++) {
console.log(colors[i]);
}
when multiple parameters are introduced:
- The first one represents each element in the array (per loop iteration) that .forEach was called on.
- The second represents the index of said element.
- The third represents the array that .forEach was called on (it will be the same for every iteration of the loop).
array.splice(startIdx, how many, ele1, ele2, ...)
// the start index to add/remove elements from
// 2nd element: the # of elements to remove
// ele1, ele2, ... the elements to add to the array
Retrieve Data: bracket and dot notatioon
function in function
document.querySelector() // 使用方法
// get the first element in the document with class="example"
document.querySelector("p.example");
// change the text of an element with id="demo"
document.querySelector("#demo").innerHTML = "Hello World!";
// Get the first
element in the document where the parent is a
element.
document.querySelector("div > p");
p.classList.toggle(className)
// boolean type used to see if already have the className
p.classList.remove(className)
// remove className from the classList of p
use getAttribute() and setAttribute() to read and write attributes like src or href
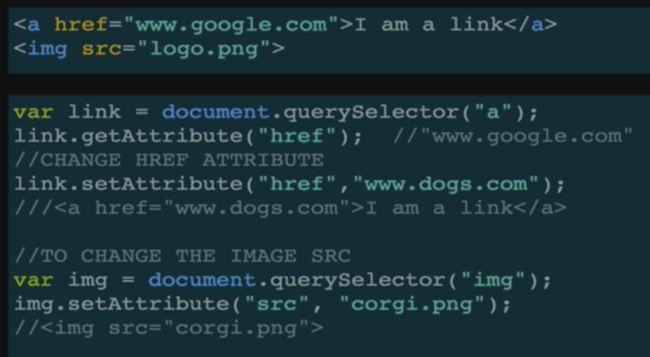

for(var i=0; i< links.length; i++) {
console.log(links[i].textContent);
}
for(var i=0; i< links.length; i++) {
links[i].style.background = "pink";
}
for(var i = 0; i < links.length; i++) {
links[i].style.border = "1px dashed purple";
links[i].style.color = "orange";
}
for(var i = 0; i< links.length; i++) {
console.log(links[i].getAttribute("href");
}
document.querySelector("ul").addEventListener("click", function(){
console.log("UL WAS CLICKED!");
});
var lis = document.querySelectorAll("li");
lis
for(var i = 0; i < lis.length; i++) {
lis[i].addEventListener("click", function(){
this.style.color = "pink";
});
}
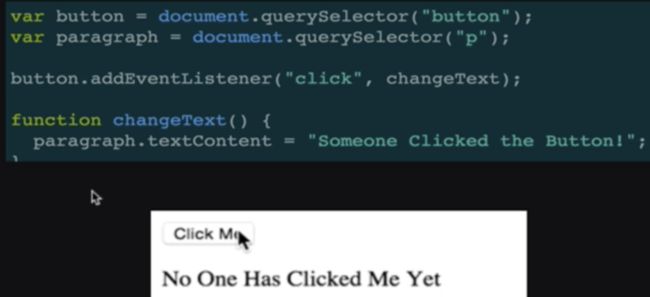
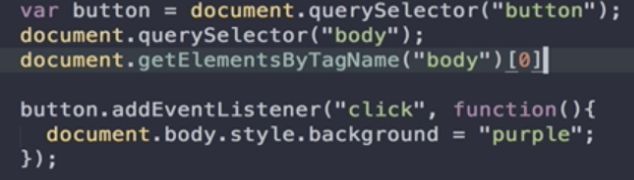
// change the background color
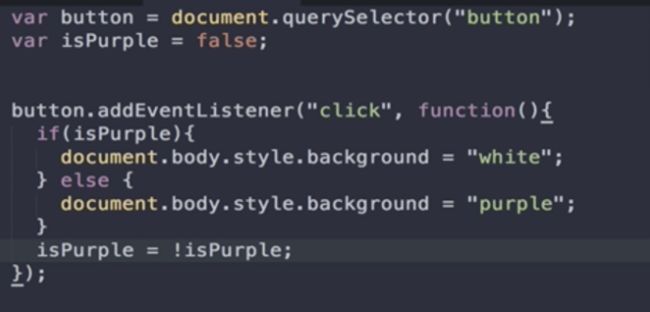
var button = document.querySelector("button");
var isPurple = false;
button.addEventListener("click", function(){
document.body.classList.toggle("purple");
});
"mouseOver" used when you want to change sth when the mouse is hanging over sth.
jQuery : make dom easier
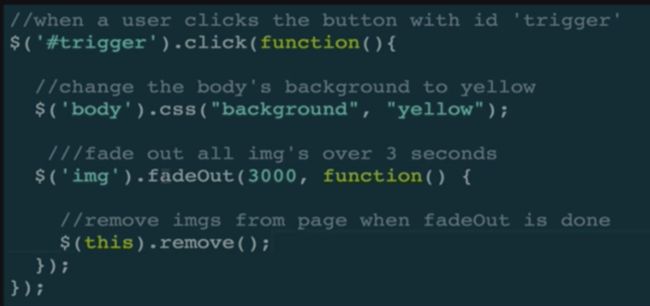
$('h1')[0];
// change the color to be yellow
$('h1').css('color', 'yellow')
// if not using jquery, then use:
document.querySelector("h1").style.color = "orange";
var lis = document.querySelectorAll("li");
for(var i = 0; i< lis.length; i++){
lis[i].style.color = "green";
}
$("li").css({
fontSize: "10px",
border: "3px dashed purple",
background: "rgba(89, 45, 20, 0.5)"
});
jQuery 用法

$("h1").text("New Text!!!"); // change the text to be "New Text!!!"

// can change the attribute type to be like "checkbox", "color"...
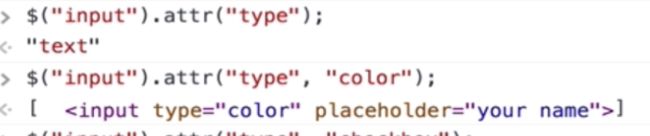
$("img").css("width", "200px");
$("img").last().attr("src", "https://www.baidu.com");
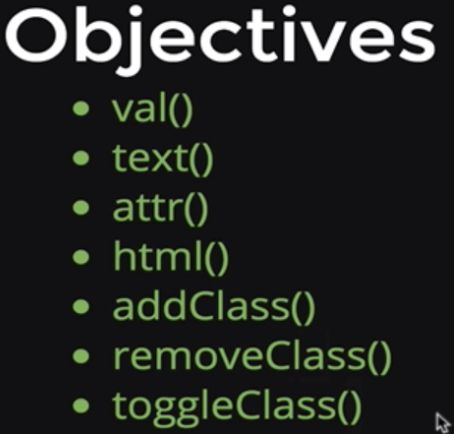
text-decoration: line-through;
click()
// prints when item with id 'submit' is clicked
$('#submit').click(function(){
console.log("Another click");
});
// alerts when ANY button is clicked
$('button').click(function(){
alert("Someone clicked a button");
$(this).css("background", "pink")
});
$("button").click(function(){
var text = $(this).text();
console.log(" You clicked " + text);
});
// on() it's not just for click events. on() supports all types of events
// double click event
$('button').on('dblclick', function(){
alert("Double Click!");
});

$("button").on("mouseenter", function(){ // also can use "mouseleave"
$(this).css("font-weight", "bold");
});
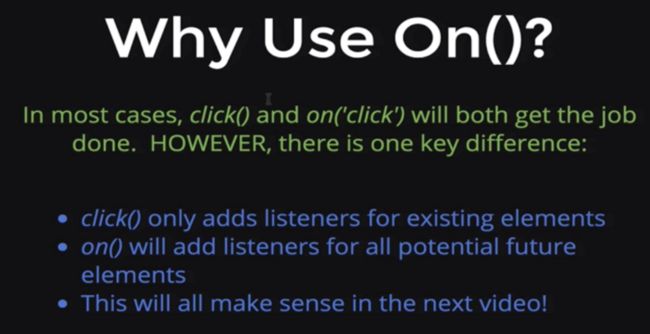
// fade in and fade out
// use fadeToggle to directly detect whether it is shown on screen,
// if no then fadein if yes then fadeout
// slidedown(), slideToggle(1000, function(){ });
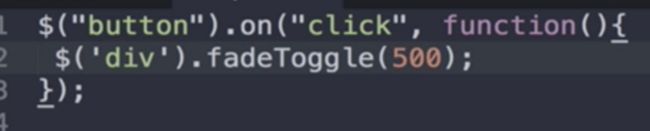