方法一:重写TextView的onDraw方法,也挺直观就是不太好控制显示完 图片后再显示字体所占空间的位置关系。
效果图:

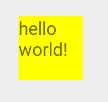
自定义View代码如下:
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.graphics.drawable.Drawable;
import android.support.annotation.NonNull;
import android.text.TextPaint;
import android.util.AttributeSet;
import android.widget.TextView;
/**
* @author Demon
*/
public class TextViewImage_1 extends TextView{
private Drawable exampleDrawable;
private TextPaint textPaint;
private float textHeight;
public TextViewImage_1(Context context) {
super(context);
init(null, 0);
}
public TextViewImage_1(Context context, AttributeSet attrs) {
super(context, attrs);
init(attrs, 0);
}
public TextViewImage_1(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
init(attrs, defStyle);
}
private void init(AttributeSet attrs, int defStyle) {
final TypedArray a = getContext().obtainStyledAttributes(
attrs, R.styleable.TextViewImage_1, defStyle, 0);
if (a.hasValue(R.styleable.TextViewImage_1_exampleDrawable)) {
exampleDrawable = a.getDrawable(
R.styleable.TextViewImage_1_exampleDrawable);
assert exampleDrawable != null;
exampleDrawable.setCallback(this);
}
a.recycle();
textPaint = new TextPaint();
textPaint.setFlags(Paint.ANTI_ALIAS_FLAG);
textPaint.setTextAlign(Paint.Align.LEFT);
invalidateTextPaintAndMeasurements();
}
private void invalidateTextPaintAndMeasurements() {
Paint.FontMetrics fontMetrics = textPaint.getFontMetrics();
textHeight = fontMetrics.bottom;
}
@Override
protected void onDraw(@NonNull Canvas canvas) {
super.onDraw(canvas);
int paddingLeft = getPaddingLeft();
int paddingTop = getPaddingTop();
int paddingRight = getPaddingRight();
int paddingBottom = getPaddingBottom();
if (exampleDrawable != null) {
int contentWidth = getWidth()- paddingLeft - paddingRight;
int contentHeight = getHeight() - paddingTop - paddingBottom;
exampleDrawable.setBounds(paddingLeft, paddingTop,
paddingLeft + contentWidth, paddingTop + contentHeight);
exampleDrawable.draw(canvas);
}
}
/**
* Gets the example drawable attribute value.
*
* @return The example drawable attribute value.
*/
public Drawable getExampleDrawable() {
return exampleDrawable;
}
/**
* Sets the view's example drawable attribute value. In the example view, this drawable is
* drawn above the text.
*
* @param exampleDrawable The example drawable attribute value to use.
*/
public void setExampleDrawable(Drawable exampleDrawable) {
exampleDrawable = exampleDrawable;
}
xml文件引用:
<bingyan.net.textimage.TextViewImage_1
android:background="#ffff00" android:layout_width="300dp"
android:layout_height="300dp"
app:exampleDrawable="@mipmap/ic_launcher"
android:id="@+id/textView_image"/>
方法二:利用TextView支持部分Html的特性,直接用api赋图片。
效果图如下
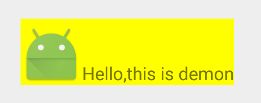
代码段如下:
String html = "
";
Html.ImageGetter imageGetter = new Html.ImageGetter() {
@Override
public Drawable getDrawable(String source) {
int id = Integer.parseInt(source);
Drawable src = getResources().getDrawable(id);
src.setBounds(0, 0, src.getIntrinsicWidth(), src.getIntrinsicHeight());
return src;
}
};
CharSequence charSequence = Html.fromHtml(html, imageGetter, null);
textView.setText(charSequence);
textView.append("Hello,this is demon");
方法三: 用ImageSpan和SpannableString
效果图:
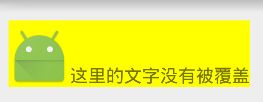
代码块:
Bitmap b = BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher);
ImageSpan imgSpan = new ImageSpan(this, b);
SpannableString spanString = new SpannableString("icon"+"这里的文字没有被覆盖");
spanString.setSpan(imgSpan, 0, 4, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
其实SpannableString很强大,下面再来看下它的几个常见用法
文本高亮:
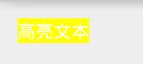
代码块:
SpannableStringBuilder spannable=new SpannableStringBuilder("高亮文本");
ForegroundColorSpan span=new ForegroundColorSpan(Color.WHITE);
spannable.setSpan(span, 0, 4, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannable);
加下划线:
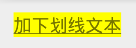
代码块:
SpannableStringBuilder spannable=new SpannableStringBuilder("加下划线文本");
CharacterStyle span=new UnderlineSpan();
spannable.setSpan(span, 0, 6, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannable);
组合使用:
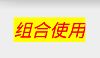
代码块:
SpannableStringBuilder spannable=new SpannableStringBuilder("组合使用");
CharacterStyle span_1=new StyleSpan(android.graphics.Typeface.ITALIC);
CharacterStyle span_2=new ForegroundColorSpan(Color.RED);
spannable.setSpan(span_1, 0, 4, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
spannable.setSpan(span_2, 0, 4, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannable);
有时需要将某关键字高亮:
最简单的想法是用循环实现:

代码块:
String tmp = "bingyantestbingyantestbingyanbingyantestbingyanbingyanbingyan";
SpannableStringBuilder spannable = new SpannableStringBuilder(tmp);
String target = "bingyan";
CharacterStyle span;
Pattern pattern = Pattern.compile(target);
Matcher matcher = pattern.matcher(tmp);
while (matcher.find()) {
span= new ForegroundColorSpan(Color.RED);
spannable.setSpan(span, matcher.start(), matcher.end(), Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
}
textView.setText(spannable);