用于保存模型,以后再用就可以直接导入模型进行计算,方便。
例如:
- import tensorflow as tf;
- import numpy as np;
- import matplotlib.pyplot as plt;
-
- v1 = tf.Variable(tf.constant(1, shape=[1]), name='v1')
- v2 = tf.Variable(tf.constant(2, shape=[1]), name='v2')
-
- result = v1 + v2
-
- init = tf.initialize_all_variables()
-
- saver = tf.train.Saver()
-
- with tf.Session() as sess:
- sess.run(init)
- saver.save(sess, "/home/penglu/Desktop/lp/model.ckpt")
-
-
结果:
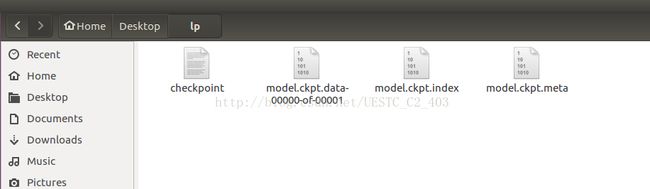
下次需要使用模型就可以用下面的代码:
- import tensorflow as tf;
- import numpy as np;
- import matplotlib.pyplot as plt;
-
- v1 = tf.Variable(tf.constant(1, shape=[1]), name='v1')
- v2 = tf.Variable(tf.constant(2, shape=[1]), name='v2')
-
- result = v1 + v2
-
- init = tf.initialize_all_variables()
-
- saver = tf.train.Saver()
-
- with tf.Session() as sess:
- saver.restore(sess, "/home/penglu/Desktop/lp/model.ckpt")
- print sess.run(result)
- import tensorflow as tf;
- import numpy as np;
- import matplotlib.pyplot as plt;
- saver = tf.train.import_meta_graph('/home/penglu/Desktop/lp/model.ckpt.meta')
- with tf.Session() as sess:
- "white-space:pre"> saver.restore(sess, "/home/penglu/Desktop/lp/model.ckpt")
- "white-space:pre"> print sess.run(tf.get_default_graph().get_tensor_by_name('add:0'))