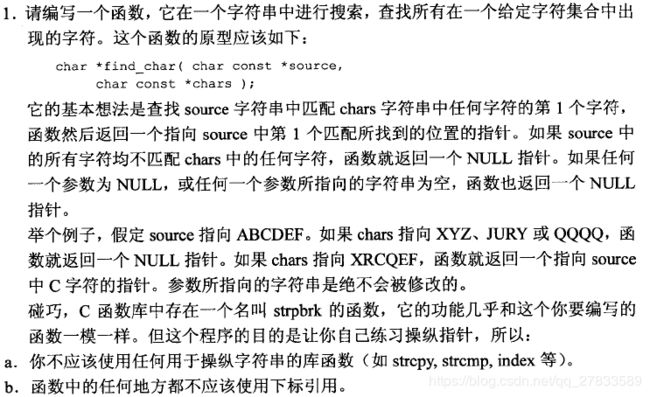
#include
#include
char *find_char(char const *source,char const *chars)
{
if(source == NULL || chars == NULL)
return NULL;
else
{
char *temp=chars;
while(*source != '\0')
{
while(*chars != '\0')
{
if(*source == *chars)
return source;
else
chars++;
}
source++;
chars=temp;
}
return NULL;
}
}
int main()
{
char *source="ABCDEF",*chars="cxAF";
char *res=find_char(source,chars);
if(res != NULL)
printf("%c",*res);
else
printf("NULL");
return 0;
}
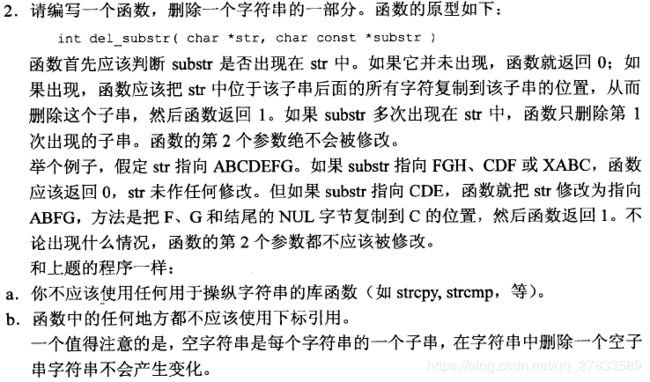
#include
#include
int del_substr(char *str, char const *substr)
{
char *substr_head=substr,*temp;
while(*str != '\0')
{
if(*str == *substr)
{
temp=str;
while(*substr != '\0' && *substr == *str)
{
substr++;
str++;
}
if(*substr == '\0')
{
while(*str != '\0')
{
*temp++ = *str++;
}
*temp='\0';
return 1;
}
else
substr=substr_head;
}
str++;
}
return 0;
}
int main()
{
char str[]="ABCDEFG",*substr="CD";
int res=del_substr(str,substr);
printf("%d\n%s\n",res,str);
return 0;
}
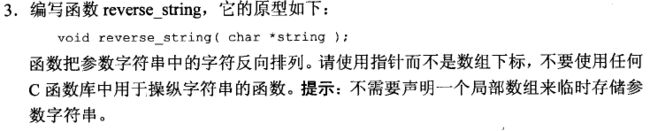
#include
#include
void reverse_string(char *string)
{
char *head=string,*tail=string,temp;
while(*tail != '\0')
tail++;
tail--;
while(head<tail)
{
temp=*head;
*head=*tail;
*tail=temp;
head++;
tail--;
}
}
int main()
{
char str[]="ABCDEfFGjhj";
printf("%s\n",str);
reverse_string(str);
printf("%s\n",str);
return 0;
}
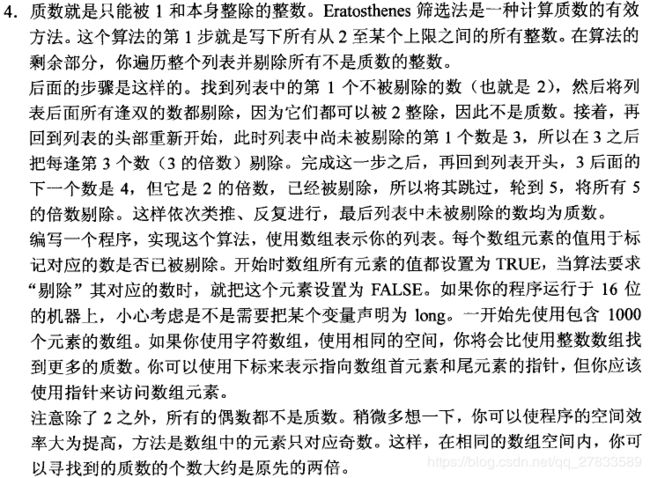
没有使用指针来访问数组
#include
#include
#include
#define SIZE 1000
#define true 1
#define false 0
int main()
{
char array[SIZE];
memset(array,true,SIZE);
array[0]=array[1]=false;
for(int i=2;i<SIZE;i++)
{
if(array[i]==true)
{
for(int j=i*i;j<SIZE;j+=i)
array[j]=false;
}
}
for(int i=0;i<SIZE;i++)
if(array[i]==true)
printf("%d ",i);
return 0;
}

#include
#include
#include
#include
#define SIZE 1000
#define true 1
#define false 0
void set_bit(char bit_array[],unsigned bit_number);
void clear_bit(char bit_array[],unsigned bit_number);
void assign_bit(char bit_array[],unsigned bit_number,int value);
int test_bit(char bit_array[],unsigned bit_number);
int character_offset(unsigned bit_number);
int bit_offset(unsigned bit_number);
int main()
{
char array[SIZE];
memset(array,0xff,SIZE);
clear_bit(array,0);
clear_bit(array,1);
for(int i=2;i<SIZE*CHAR_BIT;i++)
{
if(test_bit(array,i))
{
for(int j=i*i;j<SIZE*CHAR_BIT;j+=i)
clear_bit(array,j);
}
}
for(int i=0;i<SIZE*CHAR_BIT;i++)
if(test_bit(array,i))
printf("%d ",i);
return 0;
}
int character_offset(unsigned bit_number)
{
return bit_number / CHAR_BIT;
}
int bit_offset(unsigned bit_number)
{
return bit_number % CHAR_BIT;
}
void set_bit(char bit_array[],unsigned bit_number)
{
bit_array[character_offset(bit_number)] |= 1<<bit_offset(bit_number);
}
void clear_bit(char bit_array[],unsigned bit_number)
{
bit_array[character_offset(bit_number)] &= ~(1<<bit_offset(bit_number));
}
void assign_bit(char bit_array[],unsigned bit_number,int value)
{
if(value)
set_bit(bit_array,bit_number);
else
clear_bit(bit_array,bit_number);
}
int test_bit(char bit_array[],unsigned bit_number)
{
char ch = bit_array[character_offset(bit_number)]>>bit_offset(bit_number);
if(ch & 1)
return 1;
else
return 0;
}
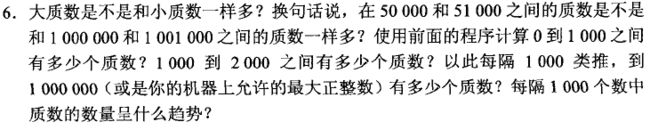
#include
#include
#include
#define SIZE 10000
#define true 1
#define false 0
#define interval 1000
int main()
{
char array[SIZE];
memset(array,true,SIZE);
array[0]=array[1]=false;
for(int i=2;i<SIZE;i++)
{
if(array[i]==true)
{
for(int j=i*i;j<SIZE;j+=i)
array[j]=false;
}
}