本文介绍了requests的基本用法以及如何使用requests抓取云栖社区博客文章。
Python3 基础教程最全总结
Python3 进阶教程最全总结
一文掌握Python基础知识
一文掌握Python列表/元组/字典/集合
一文掌握Python函数用法
Python面向对象之类与对象详解
Python面向对象之装饰器与封装详解
Python面向对象之继承和多态详解
Python异常处理和模块详解
Python文件(I/O)操作详解
Python网络编程之Socket原理与基本用法
Python多线程threading模块基本用法
Python爬虫正则表达式详解 爬爬爬爬个虫子
Python爬虫实战Urllib抓取段子
Python爬虫实战抓包分析视频评论
Python爬虫实战Requests抓取博客文章
Python爬虫实战Scrapy抓取商品信息并写入数据库
本文代码运行环境:
1.安装requests:
pip install requests
2.导入requests:
import requests
方法 | 实例 |
---|---|
requests.get() | r = requests.get('https://api.github.com/events') |
requests.post() | r = requests.post('http://httpbin.org/post', data = {'key':'value'}) |
requests.put() | r = requests.put('http://httpbin.org/put', data = {'key':'value'}) |
requests.delete() | r = requests.delete('http://httpbin.org/delete') |
requests.head() | r = requests.head('http://httpbin.org/get') |
requests.options() | r = requests.options('http://httpbin.org/get') |
常用参数说明:
params
:get请求的参数,以字典格式存储;headers
:添加头信息,伪装浏览器,以字典格式存储;proxies
:设置代理,IP等等,同样以字典格式存储;cookies
:传递cookie参数,以字典格式存储;data
:通常用于post请求中,存储的是post请求发过去的数据,以字典格式存储;常用属性说明:
text
:读取已经解码的服务器响应的内容;content
:读取二进制类型的数据(bytes);encoding
:读取当前网页的编码;cookies
:读取当前网页的cookies;url
:读取当前网页的url;status_code
:状态码,检查请求是否成功;或者使用:r.raise_for_status()
方法检查有无异常,无输出说明正常。import requests, re, random
user_agent_pool = [
'Mozilla/5.0 (Windows; U; Windows NT 6.1; en-US) AppleWebKit/532.5 (KHTML, like Gecko) Chrome/4.0.249.0 Safari/532.5',
]
headers_pool = {'User-Agent':random.choice(user_agent_pool)}
# 如果get中设置了该参数,需要打开Fiddler
proxies_pool = [
{
'http':'http://127.0.0.1:6666',
},
]
obj_url = 'https://www.jd.com/'
regular_ex = '(.*?) '
request_pull = requests.get(obj_url,
headers=headers_pool,
proxies=random.choice(proxies_pool))
response_title = re.compile(regular_ex,re.S).findall(request_pull.text)
response_title
输出:
['京东(JD.COM)-正品低价、品质保障、配送及时、轻松购物!']
如果是手工构建 URL,那么数据会以键/值对的形式置于 URL 中,跟在一个问号的后面。例如,https://yc.aliwx.com.cn/login?modal=1
。 Requests 提供了 params 关键字参数,以一个字符串字典来提供这些参数。看一个实例:
'''
阿里文学登录页面:https://yc.aliwx.com.cn/login?modal=1
'''
params_pull = {'modal':'1',}
response_con = requests.get('https://yc.aliwx.com.cn/login',params=params_pull)
抓取完成后,我们来查看一下属性信息:
type(response_con)
'''
requests.models.Response
'''
response_con.encoding
'''
'utf-8'
'''
response_con.cookies
'''
'''
response_con.url
'''
'https://yc.aliwx.com.cn/login?modal=1'
'''
response_con.status_code
'''
200
'''
requests.utils.dict_from_cookiejar(response_con.cookies)
'''
{'ctoken': 'Yvs1foGyVtn92lOMyFoz6dYj'}
'''
使用在线测试工具来测试post,百度搜的在线测试工具http://coolaf.com/zh/tool/gp
,如果自己有可以用自己的。
我们打开这个网站,输入要测试的地址,比如我用的阿里文学https://yc.aliwx.com.cn/
,参数栏随便写,我输入的6666,然后点击测试,F12,打开开发者模式,如下图,找到相应的头信息,用来编写post()
方法的参数。
代码如下:
post_test_url = 'http://coolaf.com/zh/tool/gp'
post_test_data = {
'url':'https://yc.aliwx.com.cn/',
'stylepe':'post',
'parms':6666,
}
response_post = requests.post(post_test_url, data=post_test_data)
查看属性:
response_post
'''
'''
response_con.text
'''
'\r\n\n\n\n \n \n \n \n \n \n \n 阿里文学创作平台 \n \n\n\n\n \n\n\n\n\n'
'''
首先登陆云栖社区网站主页:https://yq.aliyun.com/,搜索你想要搜索文章的关键字:
然后搜索完成后,右击空白处–>“查看网页源码”,输入搜索结果条目数索引到包含结果条目的字段,记住该字段,后续代码会用到。
接着,我们分析网页页码变化规律,首先从地址栏复制网页的url,粘贴到 word 文档中(word自动格式化换行了,方便对比),然后翻页,重复此操作三四次即可,我复制的url如下:
可以看出,该网页的网页变化规律中只有两个关键字参数,q
和p
,想要验证也很简单,我们拿上图中最后一个url来分析,分析流程如下:
q
和p
,证毕。网页页码变化规律搞清楚了,接下来我们分析如何获取每篇文章的url。
我们在网页源码模式下,随便搜索在结果页中包含的文章名关键字,比如我是在第5页搜索的“python爬虫从入门到放弃前奏学习方法”文章名中的“从入门到放弃”字段。如下图:
记住包含 href="/articles/422216"
(在源码模式下,点击该字段是可以直接跳转到该篇文章的,说明这就是包含在网页url的字段)的字段,用于构建正则表达式来获取每篇文章的url,这是第二个需要记住的字段。
有了单篇文章的url,接下来我们分析每篇文章的标题和内容如何提取。
进入到单篇文章的界面,比如我是点击href="/articles/422216"
直接跳转的,你也可以在搜索页中,随便打开一篇文章,然后右击空白处,打开源码模式,然后搜索文章标题的关键字,比如我搜的是“从入门到放弃”:
记住这个字段,后边我们需要依此来构建正则表达式。这是需要记住的第三个字段。
有了文章标题,接下来我们获取文章内容。
记住这个字段,后续我们需要这个字段构建正则表达式。这是我们需要记住的第四个字段。
至此我们已经有了四个字段,分别是:网页页码变化规律字段、构建文章url字段、文章标题字段、文章内容字段,接下来我们进入实战。
依据四个字段构建正则表达式:
pat_total_page = '找到(.*?)条关于'
- 文章url:
pat_url = '.*?'
- 文章标题:
pat_title = '(.*?)
'
- 文章内容:
pat_content = '(.*?)'
如果对正则表达式有疑问,可以看python爬虫系列的第一篇文章:点击此处
4.2 完整代码:
import requests,re,time
search_key = 'python爬虫'
obj_url = 'https://yq.aliyun.com/search/articles/'
page_regular = {'q':search_key}
response_data = requests.get(obj_url, params=page_regular).text
pat_total_page = '找到(.*?)条关于'
total_line = re.compile(pat_total_page,re.S).findall(response_data)[0]
total_page = int(total_line)//15 + 1 # 计算总页数,每页15条搜索结果
# for i in range(0, int(total_page)):
for i in range(0, 5): # 手下留情,爬下来也不看,爬取5页就行了
print('------正在爬取第'+str(i+1)+'页------')
index = str(i+1)
getdata = {
'q':search_key,
'p':index,
}
data = requests.get(obj_url, params=getdata).text
pat_url = '.*?'
try:
articles = re.compile(pat_url, re.S).findall(data)
articles_list = []
for j in articles:
single_url = 'https://yq.aliyun.com'+j
articles_list.append(single_url)
single_data = requests.get(single_url).text
pat_title = '(.*?)
'
pat_content = '(.*?)'
title = re.compile(pat_title,re.S).findall(single_data)[0]
content = re.compile(pat_content,re.S).findall(single_data)[0]
file_save = open('D:/Project/05 Python/yq_articles/'+str(i)+'_'+str(time.time())+'.html','w',encoding='utf-8')
file_save.write(title+'
'+content)
file_save.close()
except Exception as error:
pass
print(articles_list)
print('\nAll done!')
输出:
------正在爬取第1页------
['https://yq.aliyun.com/articles/751147', 'https://yq.aliyun.com/articles/748260', 'https://yq.aliyun.com/articles/751285', 'https://yq.aliyun.com/articles/747369', 'https://yq.aliyun.com/articles/748141', 'https://yq.aliyun.com/articles/748052', 'https://yq.aliyun.com/articles/745952', 'https://yq.aliyun.com/articles/748891', 'https://yq.aliyun.com/articles/726724', 'https://yq.aliyun.com/articles/743343', 'https://yq.aliyun.com/articles/747293', 'https://yq.aliyun.com/articles/746469', 'https://yq.aliyun.com/articles/704974', 'https://yq.aliyun.com/articles/744998', 'https://yq.aliyun.com/articles/75546']
------正在爬取第2页------
['https://yq.aliyun.com/articles/705610', 'https://yq.aliyun.com/articles/705028', 'https://yq.aliyun.com/articles/706346', 'https://yq.aliyun.com/articles/743377', 'https://yq.aliyun.com/articles/705838', 'https://yq.aliyun.com/articles/705748', 'https://yq.aliyun.com/articles/705957', 'https://yq.aliyun.com/articles/743728', 'https://yq.aliyun.com/articles/743341', 'https://yq.aliyun.com/articles/44704', 'https://yq.aliyun.com/articles/44703', 'https://yq.aliyun.com/articles/742407', 'https://yq.aliyun.com/articles/705133', 'https://yq.aliyun.com/articles/710095', 'https://yq.aliyun.com/articles/689397']
------正在爬取第3页------
['https://yq.aliyun.com/articles/743008', 'https://yq.aliyun.com/articles/490366', 'https://yq.aliyun.com/articles/73765', 'https://yq.aliyun.com/articles/603852', 'https://yq.aliyun.com/articles/490301', 'https://yq.aliyun.com/articles/652326', 'https://yq.aliyun.com/articles/452035', 'https://yq.aliyun.com/articles/649223', 'https://yq.aliyun.com/articles/655048', 'https://yq.aliyun.com/articles/232384', 'https://yq.aliyun.com/articles/652279', 'https://yq.aliyun.com/articles/91179', 'https://yq.aliyun.com/articles/650733', 'https://yq.aliyun.com/articles/364417', 'https://yq.aliyun.com/articles/108850']
------正在爬取第4页------
['https://yq.aliyun.com/articles/659161', 'https://yq.aliyun.com/articles/705245', 'https://yq.aliyun.com/articles/108917', 'https://yq.aliyun.com/articles/625529', 'https://yq.aliyun.com/articles/740184', 'https://yq.aliyun.com/articles/702342', 'https://yq.aliyun.com/articles/633029', 'https://yq.aliyun.com/articles/670844', 'https://yq.aliyun.com/articles/740183', 'https://yq.aliyun.com/articles/700858', 'https://yq.aliyun.com/articles/740266', 'https://yq.aliyun.com/articles/75550', 'https://yq.aliyun.com/articles/619950', 'https://yq.aliyun.com/articles/149768', 'https://yq.aliyun.com/articles/75518']
------正在爬取第5页------
['https://yq.aliyun.com/articles/78787', 'https://yq.aliyun.com/articles/702897', 'https://yq.aliyun.com/articles/108964', 'https://yq.aliyun.com/articles/704378', 'https://yq.aliyun.com/articles/696961', 'https://yq.aliyun.com/articles/584718', 'https://yq.aliyun.com/articles/422216', 'https://yq.aliyun.com/articles/659164', 'https://yq.aliyun.com/articles/694891', 'https://yq.aliyun.com/articles/703830', 'https://yq.aliyun.com/articles/232385', 'https://yq.aliyun.com/articles/703650', 'https://yq.aliyun.com/articles/232392', 'https://yq.aliyun.com/articles/333179', 'https://yq.aliyun.com/articles/703361']
All done!
爬取结果:
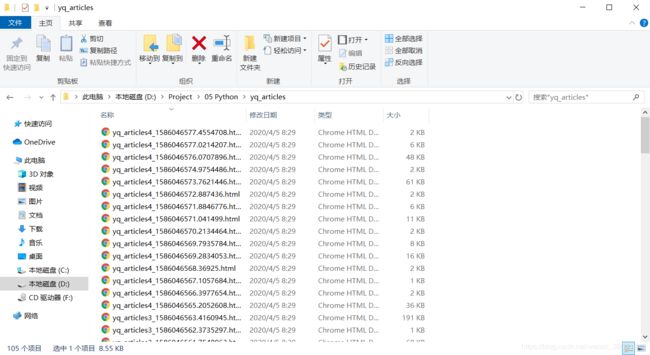
我们随便打开一篇,可以看到,内容已经抓取下来了:
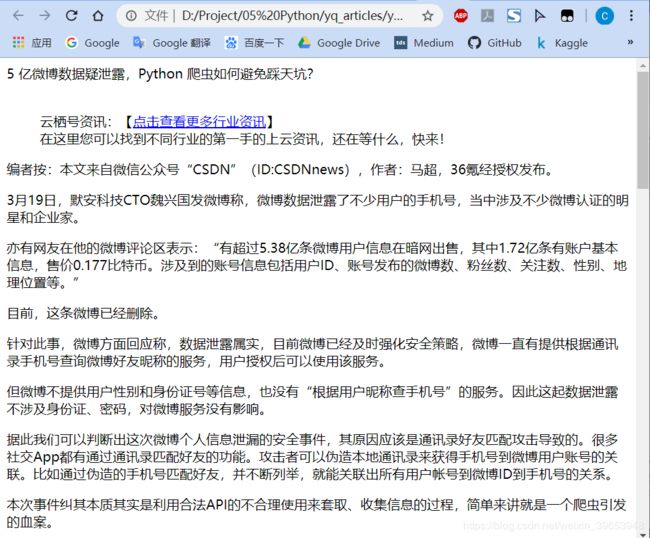
码字不易,有用点赞,Good luck!
参考:
https://edu.aliyun.com/course/1994
http://2.python-requests.org/zh_CN/latest/user/quickstart.html
https://www.liaoxuefeng.com/wiki/1016959663602400/1183249464292448