14.1 ConstrainedBox 和 SizeBox 的使用
1.源代码
import 'package:flutter/material.dart';
void main () => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
Widget blueBox = DecoratedBox(decoration: BoxDecoration(color: Colors.blue));
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.black,
body: Center(
child: Column(
children: <Widget>[
ConstrainedBox(
constraints: BoxConstraints.tight(Size(80.0, 80.0)),
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
),
ConstrainedBox(
constraints: BoxConstraints.tightFor(height: 80.0,width: 80.0),
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
),
ConstrainedBox(
constraints: BoxConstraints(minWidth: 80.0,minHeight: 80.0,maxWidth: 80.0,maxHeight: 80.0),
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
),
SizedBox(
width: 80.0,
height: 80.0,
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
)
],
)
)
)
);
}
}
2.解释源代码
import 'package:flutter/material.dart';
void main () => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
Widget blueBox = DecoratedBox(decoration: BoxDecoration(color: Colors.blue));
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.black,
body: Center(
child: Column(
children: <Widget>[
ConstrainedBox(
constraints: BoxConstraints.tight(Size(80.0, 80.0)),
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
),
ConstrainedBox(
constraints: BoxConstraints.tightFor(height: 80.0,width: 80.0),
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
),
ConstrainedBox(
constraints: BoxConstraints(minWidth: 80.0,minHeight: 80.0,maxWidth: 80.0,maxHeight: 80.0),
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
),
SizedBox(
width: 80.0,
height: 80.0,
child: Padding(padding: EdgeInsets.only(top: 10.0),child: blueBox,),
)
],
)
)
)
);
}
}
3.效果图
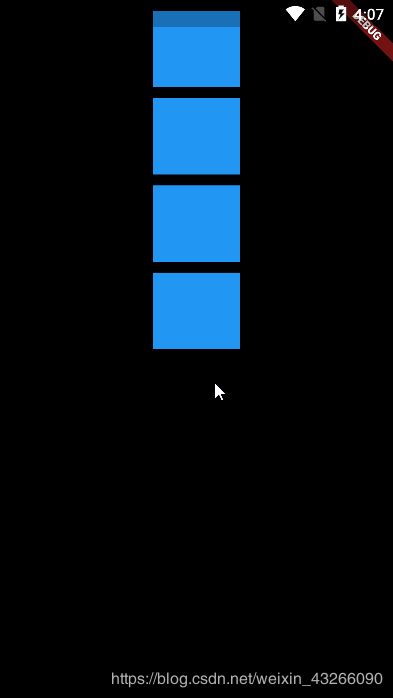