文章目录
- 局部效果展示
- 整体架构
- conf包
- sql语句
- 一、view视图层
- 二、service业务层
- 三、dao数据访问层
- 四、entity实体类
- 五、util工具包
- JdbcTemplate模板包
- JdbcUtil3工具类
- 六、rowmapper封装结果集
- RowMapper抽象类
- AccountRowMapper封装结果集类
局部效果展示
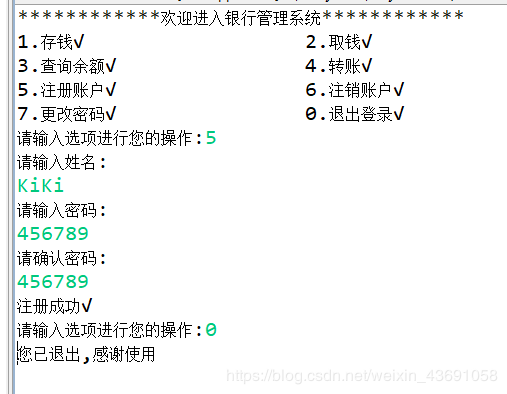
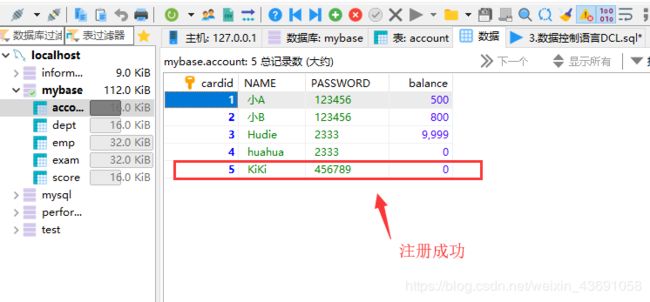
整体架构
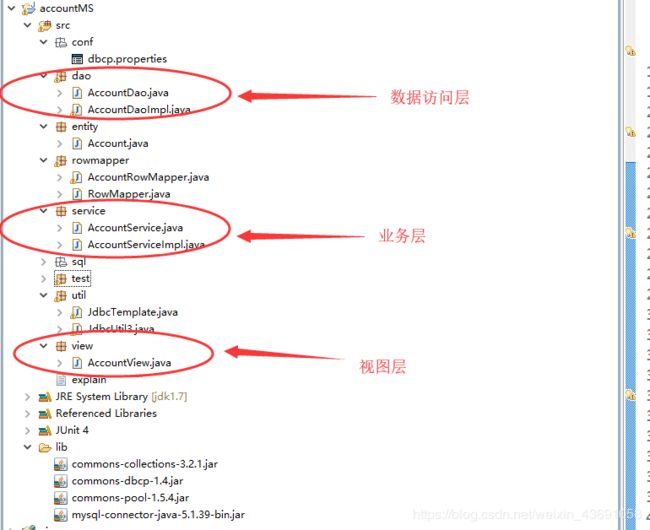
conf包
driverClassName=com.mysql.jdbc.Driver
url=jdbc\:mysql\:
username=root
password=Hudie
initialSize=10
maxActive=50
maxIdle=20
minIdle=5
maxWait=60000
connectionProperties=useUnicode\=true;characterEncoding\=utf-8
defaultAutoCommit=true
defaultTransactionIsolation=READ_COMMITTED
sql语句
CREATE TABLE ACCOUNT(
cardid INT(7) PRIMARY KEY AUTO_INCREMENT,
NAME VARCHAR(15) NOT NULL,
PASSWORD VARCHAR(6) NOT NULL,
balance double
);
INSERT INTO ACCOUNT(NAME,PASSWORD,balance) VALUES('小A','123456',500);
INSERT INTO ACCOUNT(NAME,PASSWORD,balance) VALUES('小B','123456',800);
SELECT * FROM ACCOUNT;
一、view视图层
package view;
import java.util.Scanner;
import service.AccountService;
import service.AccountServiceImpl;
import entity.Account;
public class AccountView {
private static Scanner in = new Scanner(System.in);
static AccountService accountService = new AccountServiceImpl();
public static void main(String[] args) {
System.out.println("************欢迎进入银行管理系统************");
System.out.println("1.存钱√ 2.取钱√");
System.out.println("3.查询余额√ 4.转账√");
System.out.println("5.注册账户√ 6.注销账户√");
System.out.println("7.更改密码√ 0.退出登录√");
while (true) {
System.out.print("请输入选项进行您的操作:");
int n = in.nextInt();
switch (n) {
case 1:
save();
break;
case 2:
draw();
break;
case 3:
queryBalance();
break;
case 4:
transfer();
break;
case 5:
regist();
break;
case 6:
logout();
break;
case 7:
changepassword();
break;
case 0:
System.out.println("您已退出,感谢使用");
System.exit(0);
break;
default:
throw new RuntimeException("您的输入有误,请重新输入!");
}
}
}
private static void logout() {
int cardid = -1;
String password = null;
System.out.println("请输入账号:");
cardid = in.nextInt();
System.out.println("请输入密码:");
password = in.next();
try{
accountService.logout(cardid,password);
System.out.println("账户注销完成!");
}catch(Exception e){
System.out.println(e.getMessage());
}
}
private static void changepassword() {
int cardid = -1;
String password = null;
String chpassword = null;
System.out.println("请输入账号:");
cardid = in.nextInt();
System.out.println("请输入密码:");
password = in.next();
System.out.println("请输入修改后的密码");
chpassword = in.next();
try{
accountService.changepassword(cardid,password,chpassword);
System.out.println("密码修改完成");
}catch(Exception e){
System.out.println(e.getMessage());
}
}
private static void draw() {
int cardid = -1;
String password = null;
double money= 0.0;
System.out.println("请输入账号:");
cardid = in.nextInt();
System.out.println("请输入密码:");
password = in.next();
System.out.println("请输入取钱金额:");
money = in.nextDouble();
try{
accountService.draw(cardid,password,money);
System.out.println("取钱完成");
}catch(Exception e){
System.out.println(e.getMessage());
}
}
private static void save() {
int cardid = -1;
String password = null;
double money= 0.0;
System.out.println("请输入账号:");
cardid = in.nextInt();
System.out.println("请输入密码:");
password = in.next();
System.out.println("请输入存钱金额:");
money = in.nextDouble();
try{
accountService.save(cardid,password,money);
System.out.println("存钱完成");
}catch(Exception e){
System.out.println(e.getMessage());
}
}
private static void transfer() {
int from_cardid = -1;
int to_cardid = -1;
String password = null;
double money= 0.0;
System.out.println("请输入账号:");
from_cardid = in.nextInt();
System.out.println("请输入密码:");
password = in.next();
System.out.println("请输入对方账号:");
to_cardid = in.nextInt();
System.out.println("请输入转账金额:");
money = in.nextDouble();
try {
accountService.transfer(from_cardid,password,to_cardid,money);
System.out.println("转账完成!");
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
private static void queryBalance() {
int cardid = -1;
String password = null;
System.out.println("请输入账户");
cardid = in.nextInt();
System.out.println("请输入密码");
password = in.next();
try{
Double balance = accountService.queryBalance(cardid,password);
System.out.println("您当前的余额为:"+balance);
}catch(Exception e){
System.out.println(e.getMessage());
}
}
private static void regist() {
String name = null;
String password = null;
String repassword = null;
System.out.println("请输入姓名:");
name = in.next();
System.out.println("请输入密码:");
password = in.next();
System.out.println("请确认密码:");
repassword = in.next();
Account account = new Account(null,name,password,0.0);
try{
accountService.regist(account,repassword);
System.out.println("注册成功√");
}catch(Exception e){
System.out.println(e.getMessage());
}
}
}
二、service业务层
service层抽象接口
package service;
import entity.Account;
public interface AccountService {
public void regist(Account account,String repassword);
public Double queryBalance(int cardid,String password);
public void transfer(int from_cardid, String password, int to_cardid,double money);
public void save(int cardid, String password, double money);
public void draw(int cardid, String password, double money);
public void changepassword(int cardid, String password,String chpassword);
public void logout(int cardid, String password);
}
service实体类
package service;
import java.sql.Connection;
import java.sql.SQLException;
import util.JdbcUtil3;
import dao.AccountDao;
import dao.AccountDaoImpl;
import entity.Account;
public class AccountServiceImpl implements AccountService {
AccountDao accountDao = new AccountDaoImpl();
Connection conn = null;
@Override
public void regist(Account account, String repassword) {
String name = account.getName();
if (name == null) {
throw new RuntimeException("用户名不能为空");
}
String password = account.getPassword();
if (!password.equals(repassword)) {
throw new RuntimeException("两次密码不一致");
}
try {
conn = JdbcUtil3.getConnection();
conn.setAutoCommit(false);
accountDao.insertAccount(account);
conn.commit();
} catch (Exception e) {
try {
conn.rollback();
} catch (SQLException e1) {
throw new RuntimeException("事务回滚异常");
}
} finally {
try {
conn.close();
} catch (SQLException e) {
throw new RuntimeException("关闭连接异常");
}
}
}
@Override
public Double queryBalance(int cardid, String password) {
Double balance = 0.0;
Account account = accountDao.queryAccountByCardid(cardid);
if (account == null) {
throw new RuntimeException("账号不存在");
}
if (!account.getPassword().equals(password)) {
throw new RuntimeException("密码输入错误");
}
try {
conn = JdbcUtil3.getConnection();
balance = account.getBalance();
} catch (Exception e) {
} finally {
try {
JdbcUtil3.release(null, null, conn);
} catch (Exception e) {
throw new RuntimeException("关闭连接异常");
}
}
return balance;
}
@Override
public void transfer(int from_cardid, String password, int to_cardid,double money) {
Account from_account = accountDao.queryAccountByCardid(from_cardid);
Account to_account = accountDao.queryAccountByCardid(to_cardid);
if(from_account == null){
throw new RuntimeException("您的账号不存在!");
}
if(to_account == null){
throw new RuntimeException("对方账号不存在!");
}
if(!from_account.getPassword().equals(password)){
throw new RuntimeException("密码输入错误!");
}
if(from_account.getBalance() < money){
throw new RuntimeException();
}
from_account.setBalance(from_account.getBalance()-money);
to_account.setBalance(to_account.getBalance()+money);
try{
conn = JdbcUtil3.getConnection();
conn.setAutoCommit(false);
accountDao.updateAccount(from_account);
accountDao.updateAccount(to_account);
conn.commit();
}catch(Exception e){
try {
conn.rollback();
} catch (Exception e1) {
System.out.println("事务回滚失败!");
}
}finally{
try {
conn.close();
} catch (Exception e2) {
System.out.println("数据库关闭失败");
}
}
}
@Override
public void save(int cardid, String password, double money) {
Account account = accountDao.queryAccountByCardid(cardid);
if (account == null) {
throw new RuntimeException("账号不存在");
}
if (!account.getPassword().equals(password)) {
throw new RuntimeException("密码输入错误");
}
account.setBalance(account.getBalance()+money);
try {
conn = JdbcUtil3.getConnection();
accountDao.updateAccount(account);
} catch (Exception e) {
} finally {
try {
JdbcUtil3.release(null, null, conn);
} catch (Exception e) {
throw new RuntimeException("关闭连接异常");
}
}
}
@Override
public void draw(int cardid, String password, double money) {
Account account = accountDao.queryAccountByCardid(cardid);
if (account == null) {
throw new RuntimeException("账号不存在");
}
if (!account.getPassword().equals(password)) {
throw new RuntimeException("密码输入错误");
}
account.setBalance(account.getBalance()-money);
try {
conn = JdbcUtil3.getConnection();
accountDao.updateAccount(account);
} catch (Exception e) {
} finally {
try {
JdbcUtil3.release(null, null, conn);
} catch (Exception e) {
throw new RuntimeException("关闭连接异常");
}
}
}
@Override
public void changepassword(int cardid, String password,String chpassword) {
Account account = accountDao.queryAccountByCardid(cardid);
if (account == null) {
throw new RuntimeException("账号不存在");
}
if (!account.getPassword().equals(password)) {
throw new RuntimeException("密码输入错误");
}
account.setPassword(chpassword);
try {
conn = JdbcUtil3.getConnection();
accountDao.changepassword(account);
} catch (Exception e) {
} finally {
try {
JdbcUtil3.release(null, null, conn);
} catch (Exception e) {
throw new RuntimeException("关闭连接异常");
}
}
}
@Override
public void logout(int cardid, String password) {
Account account = accountDao.queryAccountByCardid(cardid);
if (account == null) {
throw new RuntimeException("账号不存在");
}
if (!account.getPassword().equals(password)) {
throw new RuntimeException("密码输入错误");
}
try {
conn = JdbcUtil3.getConnection();
accountDao.logout(account);
} catch (Exception e) {
} finally {
try {
JdbcUtil3.release(null, null, conn);
} catch (Exception e) {
throw new RuntimeException("关闭连接异常");
}
}
}
}
三、dao数据访问层
dao层抽象接口
package dao;
import entity.Account;
public interface AccountDao {
public void insertAccount(Account account);
public Account queryAccountByCardid(int cardid);
public void updateAccount(Account account);
public void changepassword(Account account);
public void logout(Account account);
}
dao层类
package dao;
import rowmapper.AccountRowMapper;
import util.JdbcTemplate;
import entity.Account;
public class AccountDaoImpl implements AccountDao {
JdbcTemplate template = new JdbcTemplate();
@Override
public void insertAccount(Account account) {
template.update("insert into account(name,password,balance) values(?,?,?)", account.getName(),account.getPassword(),account.getBalance());
}
@Override
public Account queryAccountByCardid(int cardid) {
Account account = (Account)template.queryForObject("select * from account where cardid=?",new AccountRowMapper(),cardid);
return account;
}
@Override
public void updateAccount(Account account) {
template.update("update account set balance = ? where cardid = ?", account.getBalance(),account.getCardid());
}
@Override
public void changepassword(Account account) {
template.update("update account set PASSWORD = ? where cardid = ?",account.getPassword(),account.getCardid());
}
@Override
public void logout(Account account) {
template.update("DELETE FROM `mybase`.`account` WHERE `cardid`=?",account.getCardid());
}
}
四、entity实体类
package entity;
public class Account {
private Integer cardid;
private String name;
private String password;
private Double balance;
public Account() {
super();
}
public Account(Integer cardid, String name, String password, Double balance) {
super();
this.cardid = cardid;
this.name = name;
this.password = password;
this.balance = balance;
}
public Integer getCardid() {
return cardid;
}
public void setCardid(Integer cardid) {
this.cardid = cardid;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Double getBalance() {
return balance;
}
public void setBalance(Double balance) {
this.balance = balance;
}
@Override
public String toString() {
return "Account [cardid=" + cardid + ", name=" + name + ", password="
+ password + ", balance=" + balance + "]";
}
}
五、util工具包
JdbcTemplate模板包
package util;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import rowmapper.RowMapper;
public class JdbcTemplate<T> {
static Connection conn = null;
static PreparedStatement pstm = null;
static ResultSet rs = null;
public T queryForObject(String sql, RowMapper<T> rm, Object... args) {
T t = null;
try {
conn = JdbcUtil3.getConnection();
pstm = conn.prepareStatement(sql);
if (args.length != 0) {
for (int i = 0; i < args.length; i++) {
pstm.setObject(i + 1, args[i]);
}
}
rs = pstm.executeQuery();
if (rs.next()) {
t = rm.mappreRow(rs);
}
} catch (Exception e) {
System.out.println("数据库连接异常");
} finally {
try {
JdbcUtil3.release(rs, pstm, null);
} catch (Exception e) {
System.out.println("释放资源出现问题");
}
}
return t;
}
public List<T> queryForList(String sql,RowMapper<T> rm,Object...args){
List<T> list = null;
try {
conn = JdbcUtil3.getConnection();
pstm = conn.prepareStatement(sql);
if (args.length != 0) {
for (int i = 0; i < args.length; i++) {
pstm.setObject(i + 1, args[i]);
}
}
rs = pstm.executeQuery();
list = new ArrayList();
while(rs.next()) {
T t = rm.mappreRow(rs);
list.add(t);
}
} catch (Exception e) {
System.out.println("数据库连接异常");
} finally {
try {
JdbcUtil3.release(rs, pstm,null);
} catch (Exception e) {
System.out.println("释放资源出现问题");
}
}
return list;
}
public void update(String sql, Object... args) {
try {
conn = JdbcUtil3.getConnection();
pstm = conn.prepareStatement(sql);
if (args.length != 0) {
for (int i = 0; i < args.length; i++) {
pstm.setObject(i + 1, args[i]);
}
}
pstm.executeUpdate();
} catch (Exception e) {
System.out.println("数据库异常!");
} finally {
try {
JdbcUtil3.release(null, pstm, null);
} catch (Exception e) {
System.out.println("关闭连接异常!");
}
}
}
}
JdbcUtil3工具类
package util;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Properties;
import javax.sql.DataSource;
import org.apache.commons.dbcp.BasicDataSourceFactory;
public class JdbcUtil3 {
static DataSource pool = null;
static Properties pro = new Properties();
private static ThreadLocal<Connection> tl = new ThreadLocal<Connection>();
static{
InputStream is = null;
try {
is = JdbcUtil3.class.getResourceAsStream("/conf/dbcp.properties");
pro.load(is);
pool = BasicDataSourceFactory.createDataSource(pro);
} catch (Exception e) {
e.printStackTrace();
}finally{
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
public static Connection getConnection() throws Exception{
Connection conn = tl.get();
if(conn == null){
String url = pro.getProperty("url");
String user = pro.getProperty("username");
String password = pro.getProperty("password");
conn = pool.getConnection();
tl.set(conn);
}
return conn;
}
public static void release(ResultSet rs,PreparedStatement pstm,Connection conn) throws Exception{
if(rs!=null){
rs.close();
}
if(pstm!=null){
pstm.close();
}
if(conn!=null){
conn.close();
tl.remove();
}
}
}
六、rowmapper封装结果集
RowMapper抽象类
package rowmapper;
import java.sql.ResultSet;
public interface RowMapper<T> {
public T mappreRow(ResultSet rs);
}
AccountRowMapper封装结果集类
package rowmapper;
import java.sql.ResultSet;
import java.sql.SQLException;
import entity.Account;
import rowmapper.RowMapper;
public class AccountRowMapper implements RowMapper {
@Override
public Account mappreRow(ResultSet rs) {
Account account = new Account();
try {
account.setCardid(rs.getInt(1));
account.setName(rs.getString("name"));
account.setPassword(rs.getString(3));
account.setBalance(rs.getDouble(4));
} catch (SQLException e) {
e.printStackTrace();
}
return account;
}
}