- centos7安装mongodb教程:centos7安装mongodb
- 官网demo:Insert Documents
insertOne
插入一个文档
insertMany
插入多个文档
insert
插入一或多个文档
- python操作mongodb插入文档
- 点击查看scrapy爬取鬼故事,数据存入mongodb实战
- 显示数据库和集合
show dbs;
use test;
show collections;
db.<collection>.find();
db.collection.insertOne()
db.collection.insertMany()
db.collection.insert()
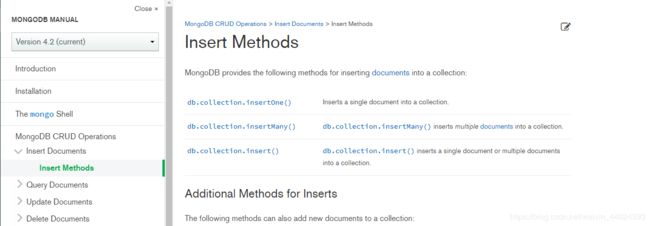
use test;
db.inventory.insertOne(
{ item: "canvas", qty: 100, tags: ["cotton"], size: { h: 28, w: 35.5, uom: "cm" } }
)
show colletions;
db.inventory.find()
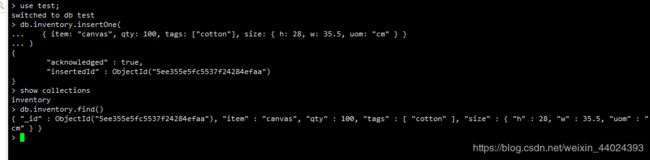
- 插入多个文档
insertMany()
接受一个列表,列表里面是多个字典
db.inventory.insertMany([
{ item: "journal", qty: 25, tags: ["blank", "red"], size: { h: 14, w: 21, uom: "cm" } },
{ item: "mat", qty: 85, tags: ["gray"], size: { h: 27.9, w: 35.5, uom: "cm" } },
{ item: "mousepad", qty: 25, tags: ["gel", "blue"], size: { h: 19, w: 22.85, uom: "cm" } }
])
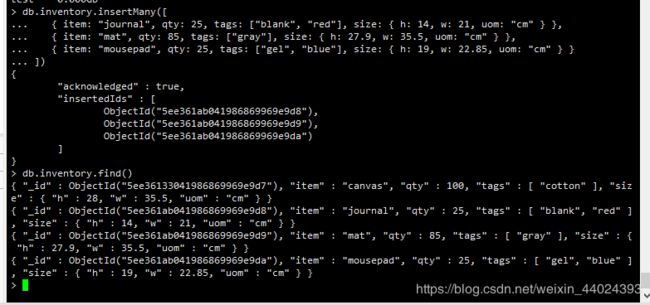
insert()
,即可接受一个字典,也可接受一个列表,列表放多个字典
db.collection.insert(
<document or array of documents>,
{
writeConcern: <document>,
ordered: <boolean>
}
)
db.products.insert( { item: "card", qty: 15 } )

db.products.insert(
[
{ _id: 11, item: "pencil", qty: 50, type: "no.2" },
{ item: "pen", qty: 20 },
{ item: "eraser", qty: 25 }
]
)
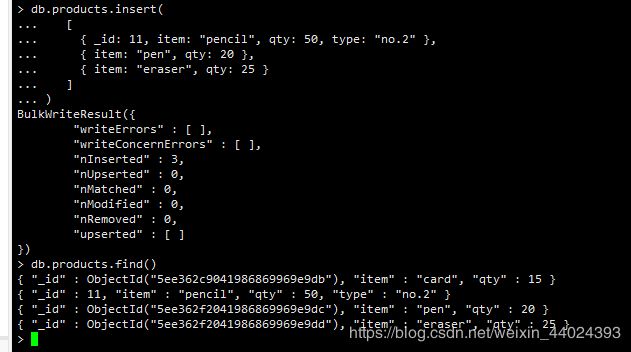
python操作mongodb插入文档
1. 安装pymongo模块
pip install -i https://pypi.douban.com/simple pymongo
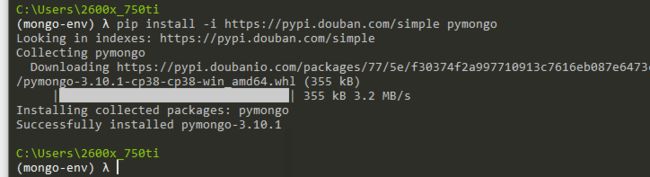
import pymongo
class MyMongo:
def __init__(self):
self.client = pymongo.MongoClient(host='192.168.19.128', port=27017)
self.db = self.client.test
def insert(self):
resp = self.db.inventory.insert_one({'hehe': 'hehe'})
print(resp)
def close(self):
self.client.close()
print('mongodb已关闭')
if __name__ == '__main__':
mon = MyMongo()
mon.insert()
mon.close()
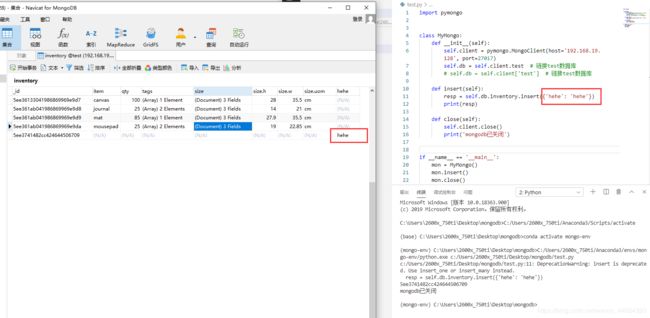
resp = self.db.inventory.insert_many([{
'haha': 'haha'
}, {
'aaa': 'aaa'
}, {
'bbb': 'bbb'
}])
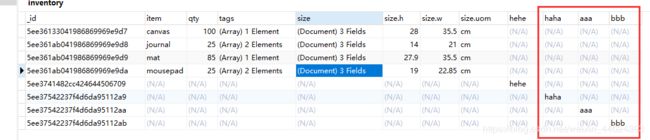
class MyMongo:
def __init__(self):
self.uri = 'mongodb://192.168.19.128:27017'
self.client = pymongo.MongoClient(self.uri)
self.db = self.client.test
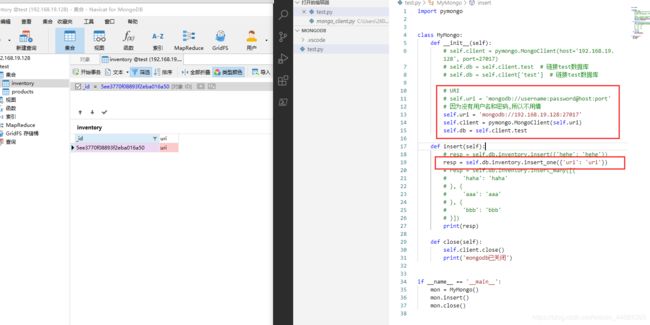