最快的学习区块链的方法就是学习怎么构建区块链
你在这里的原因可能和我一样,对 加密数字货币(Cryptocurrencies) 非常感兴趣。并且你想要知道 区块链(Blockchains) 是怎么工作的—它们背后的技术
但是理解区块链不是那么简单—-或者不适合我。我在浩瀚的视频里找寻,在满目疮痍的指南里追随,还被几个破例子所挫败
我喜欢通过做来学习。这能让我集中精力去处理代码层面上的主要问题。如果你跟随我这样做,在本文的最后你将得到一个可以正常工作的区块链
请记住区块链是不可更改的,顺序的记录链我们称为块(Blocks) 这些块可以包含了交易(transactions),文件或者其他你喜欢的数据。但是最重要的是通过哈希(hash) 把这些块链在一起,就像海上有一群毫不相关的孤岛(块),有人通过桥(哈希)把这些岛连接在一起。
如果你不知道哈希是什么,这里有解释
本文的受众群体 你应该可以轻松阅读和编写基本的python代码,并且理解HTTP的request是怎么工作的,因为我们通过HTTP与我们的区块链交互。
你需要准备什么? 确保python3.6+已经安装,并且你需要安装Flask和Rquests 两个库
pip install Flask==0.12.2 requests==2.18.4
对了,你还需要一个HTTP客户端,比如 Postman 或者curl 。任何可以完成相同工作的工具都可以。
哪里能找到最终代码 源代码在github上,点击这里
打开一个你最爱的文本编辑器或者IDE,个人喜好PyCharm.创建一个新文件blockchain.py
我们只会用一个文件,如果你有点迷糊了,可以参考源代码
我们创建一个 Blockchain
类,它的构造函数的功能是创建一个空的list(来存储我们的区块链)和一个存储交易信息的list。我们类的蓝图:
class Blockchain(object):
def __init__(self):
self.chain = []
self.current_transactions = []
def new_block(self):
# Creates a new Block and adds it to the chain
pass
def new_transaction(self):
# Adds a new transaction to the list of transactions
pass
@staticmethod
def hash(block):
# Hashes a Block
pass
@property
def last_block(self):
# Returns the last Block in the chain
pass
我们的 Blockchain
类的职责就是管理这些链。这些链能存储交易并且有一些可以添加区块到链的辅助方法。让我们丰满这些方法吧
每一个区块都有一个索引(index),一个时间戳(timestamp)(Unix时间),一个交易集合(transaction),一个证明(proof)(后面会解释),还有最重要的前一个区块的哈希(hash of previous Block)
下面有一个简单的区块样子:
# Example of a Block in our Blockchain
block = {
'index': 1,
'timestamp': 1506057125.900785,
'transactions': [
{
'sender': "8527147fe1f5426f9dd545de4b27ee00",
'recipient': "a77f5cdfa2934df3954a5c7c7da5df1f",
'amount': 5,
}
],
'proof': 324984774000,
'previous_hash': "2cf24dba5fb0a30e26e83b2ac5b9e29e1b161e5c1fa7425e73043362938b9824"
}
此时,区块链的思路就是这样:每一个新的区块都包含它自身和前一个区块的哈希。这是最重要的特性,保证了区块链的不可更改的特性:如果有攻击者破坏了早先的区块,会导致该区块的后续子链都会包含错误的哈希。
可以理解吗?如果不理解,花点时间理解这个概念,因为这是区块链的核心概念。
我们需要一个添加交易到区块的方式。我们的 new_transaction()
方法将会帮助我们处理这个问题:
class Blockchain(object):
...
def new_transaction(self, sender, recipient, amount):
"""
Creates a new transaction to go into the next mined Block
:param sender: Address of the Sender
:param recipient: Address of the Recipient
:param amount: Amount
:return: The index of the Block that will hold this transaction
"""
self.current_transactions.append({
'sender': sender,
'recipient': recipient,
'amount': amount,
})
return self.last_block['index'] + 1
在new_transaction()
添加一个交易到list(current_transactions),它返回交易被添加的那个区块的索引,也就是下一个要被挖矿的区块的索引。这将会在后面非常有用,用户通过这种方式提交交易。
当我们的Blockchain
被实例化的时候,我们需要给它发送一个创世区块(genesis block)–一个没有生产者的区块。我们也需要添加一个证明(proof)到我们的创世区块中,说明这是挖矿的结果(可能这不是很好理解,就是初始化一个block,手动的,但告诉计算机这是我们挖矿的结果是有效的)。我们会在后面详细说明这个。
此外我们需要在我们的构造器里创建 创世区块,我们丰富了new_block()
,new_transaction()
,hash()
这些方法:
import hashlib
import json
from time import time
class Blockchain(object):
def __init__(self):
self.current_transactions = []
self.chain = []
# Create the genesis block
self.new_block(previous_hash=1, proof=100)
def new_block(self, proof, previous_hash=None):
"""
Create a new Block in the Blockchain
:param proof: The proof given by the Proof of Work algorithm
:param previous_hash: (Optional) Hash of previous Block
:return: New Block
"""
block = {
'index': len(self.chain) + 1,
'timestamp': time(),
'transactions': self.current_transactions,
'proof': proof,
'previous_hash': previous_hash or self.hash(self.chain[-1]),
}
# Reset the current list of transactions
self.current_transactions = []
self.chain.append(block)
return block
def new_transaction(self, sender, recipient, amount):
"""
Creates a new transaction to go into the next mined Block
:param sender: Address of the Sender
:param recipient: Address of the Recipient
:param amount: Amount
:return: The index of the Block that will hold this transaction
"""
self.current_transactions.append({
'sender': sender,
'recipient': recipient,
'amount': amount,
})
return self.last_block['index'] + 1
@property
def last_block(self):
return self.chain[-1]
@staticmethod
def hash(block):
"""
Creates a SHA-256 hash of a Block
:param block: Block
:return:
"""
# We must make sure that the Dictionary is Ordered, or we'll have inconsistent hashes
block_string = json.dumps(block, sort_keys=True).encode()
return hashlib.sha256(block_string).hexdigest()
上面的代码是非常直观的,我添加了一些注释让它可以更清晰。我们已经完成了区块链的整体架构。但在这里,我们必须思考区块链是怎么创建,锻造或者挖矿的
工作证明算法(Proof of Work algorithm PoW)描述了怎么在区块链上创建或者挖掘新的区块。PoW的目标就是发现一个可以解决问题的数字。从计算机角度来讲,这个数字对于整个网络上的每个人,必须很难被发现但很容易验证。这就是PoW背后的核心思想。
我们看一个简单例子来帮助理解!
我们决定一个整数x乘上一个整数y的结果的哈希值必须0结尾。所以,hash(x*y)=ac23dc...0
。为了简化说明,我们假定x=5
,python实现:
from hashlib import sha256
x = 5
y = 0 # 我们不知道y是多少
while sha256(f'{x*y}'.encode()).hexdigest()[-1]!="0":
y+=1
print(f'The solution is y = {y}')
this solution is y=21
,因为此时的计算出的hash才是0结尾
hash(5 * 21) = 1253e9373e...5e3600155e860
在比特币中,工作证明算法被称为Hashcash。大体上,与我们上面这个例子差不多。这个算法就是矿工们为了创建一个新的区块,一起竞争解决这个问题(就是算一个数字)。通常来说,计算的困难度取决于字符串中搜索到字符个数。然后,矿工们通过交易得到一个币作为解决方案(solution)的奖励。
网络必须能够轻松验证这个解决方案。
让我们在我们的区块链上实现一个相似的算法。我们的规则将和上面例子很相似:
找到一个数字p ,当p与前一个区块的solution(也就是上一个hash)进行hash时,计算出的hash结果已4个0开头
import json
from time import time
from uuid import uuid4
class Blockchain(object):
...
def proof_of_work(self, last_proof):
"""
Simple Proof of Work Algorithm:
- Find a number p' such that hash(pp') contains leading 4 zeroes, where p is the previous p'
- p is the previous proof, and p' is the new proof
:param last_proof:
:return:
"""
proof = 0
while self.valid_proof(last_proof, proof) is False:
proof += 1
return proof
@staticmethod
def valid_proof(last_proof, proof):
"""
Validates the Proof: Does hash(last_proof, proof) contain 4 leading zeroes?
:param last_proof: Previous Proof
:param proof: Current Proof
:return: True if correct, False if not.
"""
guess = f'{last_proof}{proof}'.encode()
guess_hash = hashlib.sha256(guess).hexdigest()
return guess_hash[:4] == "0000"
为了调节算法的难度,我们修改了开头数字的个数。4个开头数字是非常有效的。你会发现增加一个开头0会对找到解决方案花费的时间产生巨大的影响。
我们的类几乎快完成了,要开始准备通过HTTP请求进行交互了
我们将使用Python的Flask框架进行开发。这是一个微框架并且很轻易就完成endpoints到python函数的映射。这允许我们可以在网络上通过HTTP请求与我们的区块链进行交互。
我们将创建三个方法:
/transactions/new
来在链上创建一个新交易/mine
告诉我们的服务器挖到了新的区块/chain
返回全部的区块链我们的“服务器”将会将会在区块链网络上形成一个单节点。让我们创建一些模板代码:
import hashlib
import json
from textwrap import dedent
from time import time
from uuid import uuid4
from flask import Flask
class Blockchain(object):
...
# Instantiate our Node
app = Flask(__name__)#实例化节点
# Generate a globally unique address for this node
node_identifier = str(uuid4()).replace('-', '')#为节点创建一个随机的名字
# Instantiate the Blockchain
blockchain = Blockchain()#实例化区块链类
#定义/mine的endpoint,get请求
@app.route('/mine', methods=['GET'])
def mine():
return "We'll mine a new Block"
#定义了/transactions/new,post请求,原因是我们将会发送一些数据给他
@app.route('/transactions/new', methods=['POST'])
def new_transaction():
return "We'll add a new transaction"
# 定义了/chain ,返回整个区块链
@app.route('/chain', methods=['GET'])
def full_chain():
response = {
'chain': blockchain.chain,
'length': len(blockchain.chain),
}
return jsonify(response), 200
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
更多阅读Flask
这就是交易请求的样子。这是用户向服务器发送的具体内容。
{
"sender": "my address",
"recipient": "someone else's address",
"amount": 5
}
因为我们已经添加交易到区块上的类方法,剩下的工作就很简单了。让我们编写添加交易的函数吧
import hashlib
import json
from textwrap import dedent
from time import time
from uuid import uuid4
from flask import Flask, jsonify, request
...
@app.route('/transactions/new', methods=['POST'])
def new_transaction():
values = request.get_json()
# Check that the required fields are in the POST'ed data
required = ['sender', 'recipient', 'amount']
if not all(k in values for k in required):
return 'Missing values', 400
# Create a new Transaction
index = blockchain.new_transaction(values['sender'], values['recipient'], values['amount'])
response = {'message': f'Transaction will be added to Block {index}'}
return jsonify(response), 201
我们挖矿的endpoints就是魔法发生的地方,而且非常容易。只需要做三件事:
1. 计算工作证明(PoW)
2. 奖励矿工通过添加交易给予矿工1个币
3. 通过将新的区块添加到链中来伪造(创造)新块
import hashlib
import json
from time import time
from uuid import uuid4
from flask import Flask, jsonify, request
...
@app.route('/mine', methods=['GET'])
def mine():
# We run the proof of work algorithm to get the next proof...
last_block = blockchain.last_block
last_proof = last_block['proof']
proof = blockchain.proof_of_work(last_proof)
# We must receive a reward for finding the proof.
# The sender is "0" to signify that this node has mined a new coin.
blockchain.new_transaction(
sender="0",
recipient=node_identifier,
amount=1,
)
# Forge the new Block by adding it to the chain
previous_hash = blockchain.hash(last_block)
block = blockchain.new_block(proof, previous_hash)
response = {
'message': "New Block Forged",
'index': block['index'],
'transactions': block['transactions'],
'proof': block['proof'],
'previous_hash': block['previous_hash'],
}
return jsonify(response), 200
```
**注意** 挖掘区块的接收者(recipient)是我们节点的地址。
我们在这里完成的大部分工作只是与Blockchain类中的方法进行交互。到这里,我们已经完成了区块链,可以开始与之交互了。
# Step 3:区块链交互
你可以用简单的`cURL`或者`Postman` 在网上与我们的API进行交互。
开启我们的服务:
python blockchain.py
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
我们先尝试通过GET请求挖一个矿(block)出来:
`http://127.0.0.1:5000/mine`:
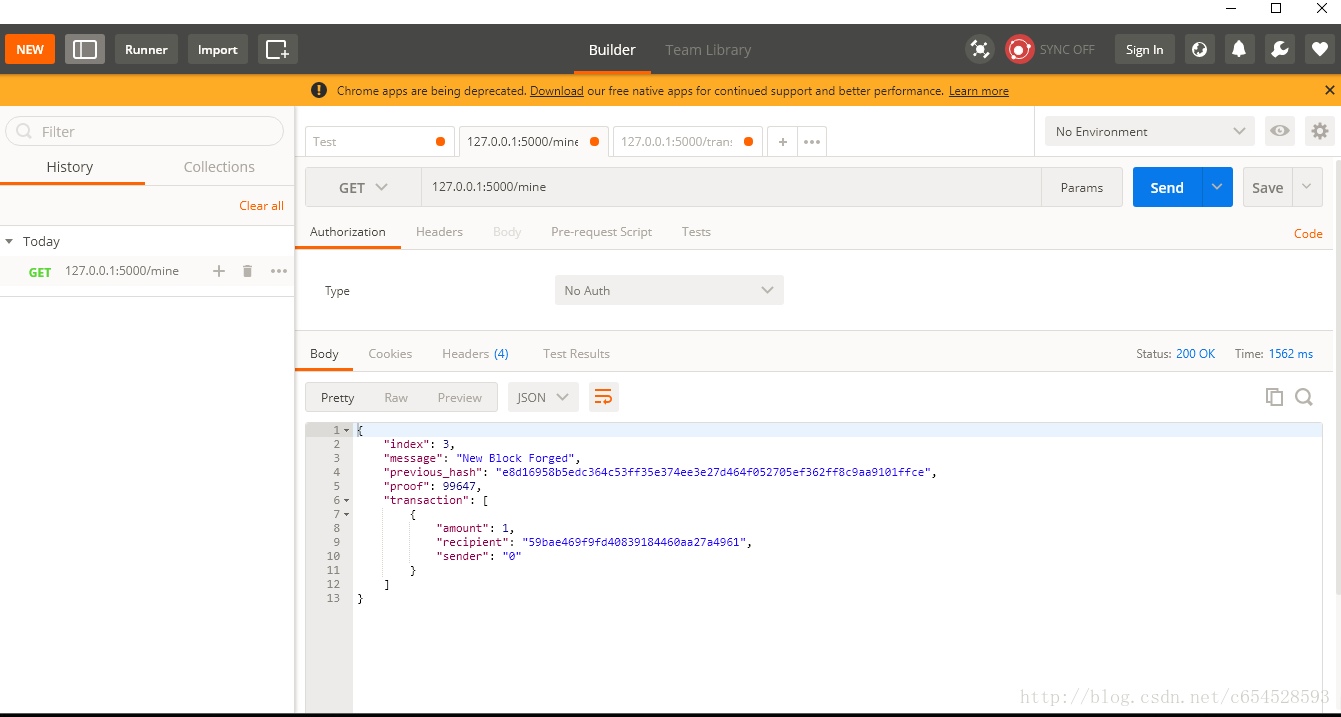
我们用POST请求到`http://localhost:5000/transactions/new`创建一个新交易,发送内容如下(注意勾选json格式):
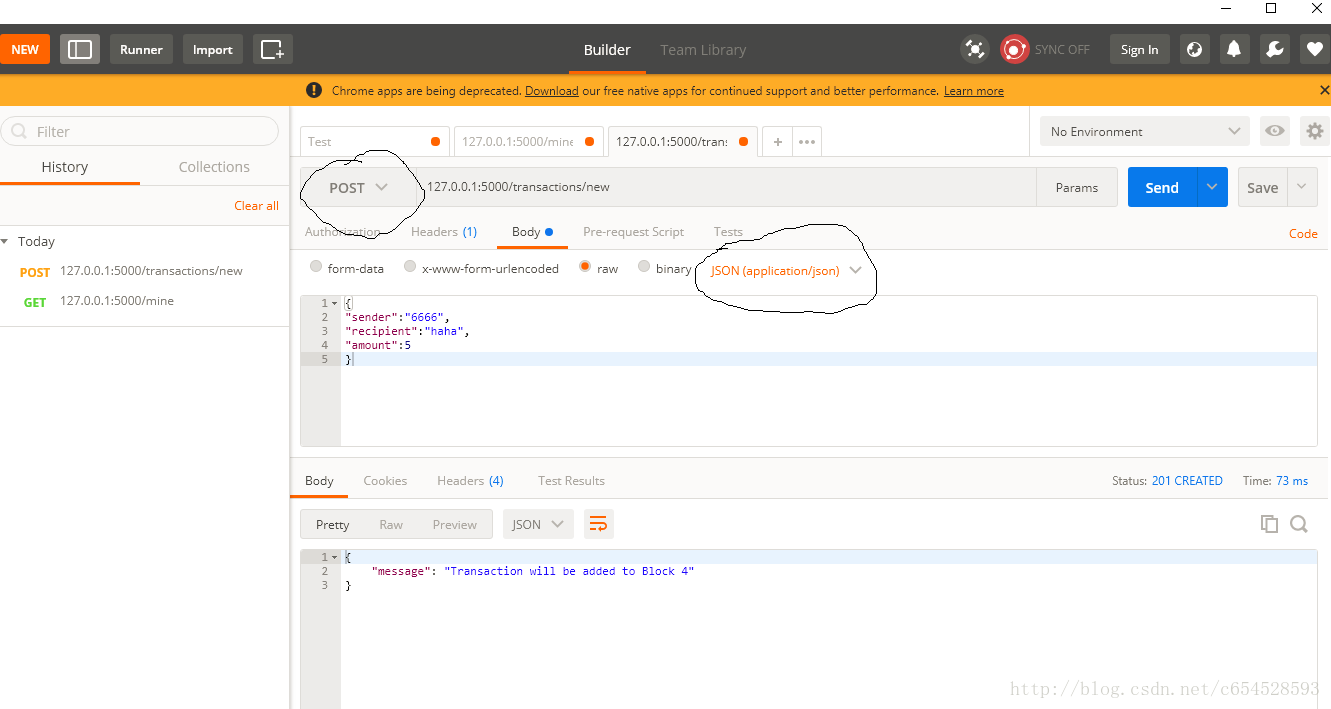
如果您不使用Postman,那么您可以使用cURL进行等效请求:
```linux
$ curl -X POST -H "Content-Type: application/json" -d '{
"sender": "d4ee26eee15148ee92c6cd394edd974e",
"recipient": "someone-other-address",
"amount": 5
}' "http://localhost:5000/transactions/new"
<div class="se-preview-section-delimiter">div>
我重启了服务,然后挖了两个block,总共3个(包括genesis block)。我们通过http://localhost:5000/chain
检查我们完整的区块链
{
"chain": [
{
"index": 1,
"previous_hash": 1,
"proof": 100,
"timestamp": 1519459719.95761,
"transaction": []
},
{
"index": 2,
"previous_hash": "f8c11eb5715c313053155a374e51ae1973e491d90739042c033927ce6722e26e",
"proof": 10682,
"timestamp": 1519459743.3767612,
"transaction": [
{
"amount": 1,
"recipient": "59bae469f9fd40839184460aa27a4961",
"sender": "0"
}
]
},
{
"index": 3,
"previous_hash": "e8d16958b5edc364c53ff35e374ee3e27d464f052705ef362ff8c9aa9101ffce",
"proof": 99647,
"timestamp": 1519459782.6483908,
"transaction": [
{
"amount": 1,
"recipient": "59bae469f9fd40839184460aa27a4961",
"sender": "0"
}
]
}
],
"length": 3
}
class="se-preview-section-delimiter">div>
Step 4:一致性
这个就非常酷了。我们有了一个可以接收交易并且允许挖矿的基本区块链了。但是整个区块链的观点是它们应该去中心化(decentralized) 。如果它们是去中心化的,我们怎么才能保证他们反映都是一条区块链呢?我们把这个问题称之为一致性问题,如果我们的网络有多个节点,我们就必须实现一致性算法(Consensus Algorithm)
注册新节点
在我们实现一致性算法之前,我们需要一种让节点知道网络上的相邻节点。我们在网络上的每一个节点都应该保留其他节点的注册表(Set)。因此,我们需要一些其他的endpoint:
/nodes/register
接收以URL形式的节点列表
/nodes/resolve
实现了我们的一致性算法,它可以解决任何冲突,确保节点具有正确的链。
我们需要修改Blockchain类的构造器,给注册节点提供一个方法:
...
from urllib.parse import urlparse
...
class Blockchain(object):
def __init__(self):
...
self.nodes = set()
...
def register_node(self, address):
"""
Add a new node to the list of nodes
:param address: Address of node. Eg. 'http://192.168.0.5:5000'
:return: None
"""
parsed_url = urlparse(address)
self.nodes.add(parsed_url.netloc)
class="se-preview-section-delimiter">div>
注意这里我们用 set()
来持有节点列表。这是一个比较廉价的方法来确保节点的添加是幂等的,这意味着无论我们添加特定节点多少次,它都只会出现一次。
实现一致性算法
综上所述,当一个节点与另一个节点有不同的链时,就会产生冲突。为了解决这个问题,我们指定最长的有效链为权威链。换句话来说,网络上最长的链是实际上唯一链。通过这个算法,我们可以实现网络上所以节点都维护同一条链。
...
import requests
class Blockchain(object)
...
def valid_chain(self, chain):
"""
Determine if a given blockchain is valid
:param chain: A blockchain
:return: True if valid, False if not
"""
last_block = chain[0]
current_index = 1
while current_index < len(chain):
block = chain[current_index]
print(f'{last_block}')
print(f'{block}')
print("\n-----------\n")
# Check that the hash of the block is correct
if block['previous_hash'] != self.hash(last_block):
return False
# Check that the Proof of Work is correct
if not self.valid_proof(last_block['proof'], block['proof']):
return False
last_block = block
current_index += 1
return True
def resolve_conflicts(self):
"""
This is our Consensus Algorithm, it resolves conflicts
by replacing our chain with the longest one in the network.
:return: True if our chain was replaced, False if not
"""
neighbours = self.nodes
new_chain = None
# We're only looking for chains longer than ours
max_length = len(self.chain)
# Grab and verify the chains from all the nodes in our network
for node in neighbours:
response = requests.get(f'http://{node}/chain')
if response.status_code == 200:
length = response.json()['length']
chain = response.json()['chain']
# Check if the length is longer and the chain is valid
if length > max_length and self.valid_chain(chain):
max_length = length
new_chain = chain
# Replace our chain if we discovered a new, valid chain longer than ours
if new_chain:
self.chain = new_chain
return True
return False
class="se-preview-section-delimiter">div>
第一个方法valid_chain()
负责通过循环遍历每个块并验证散列和证明来检查链是否有效。
resolve_conflicts()
是一种循环遍历所有邻居节点的方法,下载它们的链并使用上述方法验证它们。如果找到一个有效的链条,其长度大于我们的链条,我们将替换我们的链条。
让我们在我们的API中注册两个endpoint,一个负责添邻近节点,另一个负责解决冲突。
@app.route('/nodes/register', methods=['POST'])
def register_nodes():
values = request.get_json()
nodes = values.get('nodes')
if nodes is None:
return "Error: Please supply a valid list of nodes", 400
for node in nodes:
blockchain.register_node(node)
response = {
'message': 'New nodes have been added',
'total_nodes': list(blockchain.nodes),
}
return jsonify(response), 201
@app.route('/nodes/resolve', methods=['GET'])
def consensus():
replaced = blockchain.resolve_conflicts()
if replaced:
response = {
'message': 'Our chain was replaced',
'new_chain': blockchain.chain
}
else:
response = {
'message': 'Our chain is authoritative',
'chain': blockchain.chain
}
return jsonify(response), 200
到这里我们的程序总算是写完了。我们可以开始启动我们的程序了,嗯,多个节点。在电脑的不同端口上启动该程序。比如节点1 http://localhost:5000
和节点2 http://localhost:5001
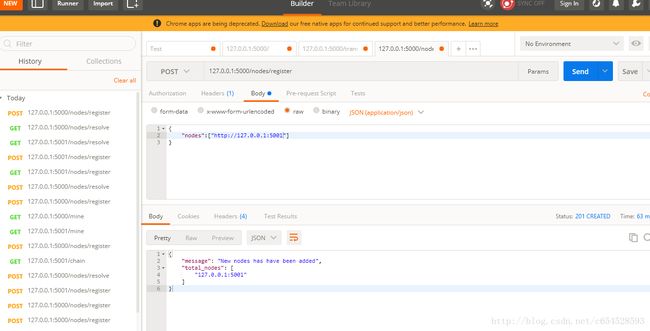
然后我在这节点2上挖了一些新的区块,确保链式最长的。然后我在节点1进行 GET /nodes/resolve
,节点1的链就会被一致性算法替换掉。
第一个方法`valid_chain()` 负责通过循环遍历每个块并验证散列和证明来检查链是否有效。
`resolve_conflicts()` 是一种循环遍历所有邻居节点的方法,下载它们的链并使用上述方法验证它们。**如果找到一个有效的链条,其长度大于我们的链条,我们将替换我们的链条。**
让我们在我们的API中注册两个endpoint,一个负责添邻近节点,另一个负责解决冲突。
```python
@app.route('/nodes/register', methods=['POST'])
def register_nodes():
values = request.get_json()
nodes = values.get('nodes')
if nodes is None:
return "Error: Please supply a valid list of nodes", 400
for node in nodes:
blockchain.register_node(node)
response = {
'message': 'New nodes have been added',
'total_nodes': list(blockchain.nodes),
}
return jsonify(response), 201
@app.route('/nodes/resolve', methods=['GET'])
def consensus():
replaced = blockchain.resolve_conflicts()
if replaced:
response = {
'message': 'Our chain was replaced',
'new_chain': blockchain.chain
}
else:
response = {
'message': 'Our chain is authoritative',
'chain': blockchain.chain
}
return jsonify(response), 200
到这里我们的程序总算是写完了。我们可以开始启动我们的程序了,嗯,多个节点。在电脑的不同端口上启动该程序。比如节点1 http://localhost:5000
和节点2 http://localhost:5001
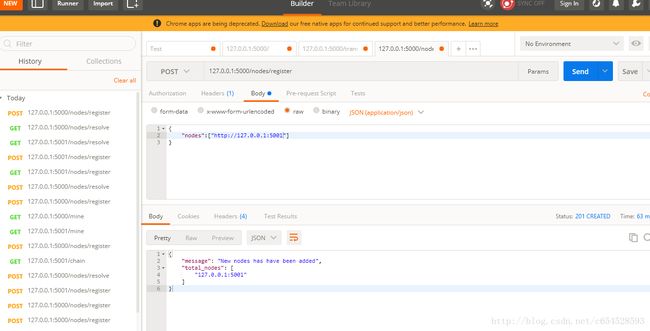
然后我在这节点2上挖了一些新的区块,确保链式最长的。然后我在节点1进行 GET /nodes/resolve
,节点1的链就会被一致性算法替换掉。
好像没翻完= =,观众老爷对不住了