- python异步--asyncio
HWQlet
pythonpython异步编程
在python2.x和python3.x早期版本的时候,协程的主流实现方法是gevent,这个我之前讲过asyncio在python3.4后内置在python中了,在后面还有async/await,更后面有aiohttp,flask实现就有参照aiohttpasync和await分别又来替换早期协程的asyncio.coroutine和yieldfrom。从此以后,协程就是python中一个新的语
- python+flask计算机毕业设计基于Android平台的景区移动端旅游软件系统(程序+开题+论文)
Node.js彤彤 程序
pythonflask课程设计
本系统(程序+源码+数据库+调试部署+开发环境)带论文文档1万字以上,文末可获取,系统界面在最后面。系统程序文件列表开题报告内容研究背景随着移动互联网技术的飞速发展,智能手机已成为人们日常生活中不可或缺的一部分,特别是在旅游领域,移动端应用以其便捷性、实时性和个性化服务的特点,极大地改变了人们的旅游体验方式。当前,旅游市场日益繁荣,游客对于旅游信息获取、行程规划、景点导航、票务预订及个性化服务的需
- python中Flask模块的使用
weixin_30315905
pythonjson
1.简介在服务器上运行Flask接口,就能使用requests模块获取该接口的值。先运行接口文件,再运行requests文件,即可获取值。2.示例2.1一个简单的flask接口1importjson2fromflaskimportFlask,request34#python类型5data={6'name':'John',7'age':18,8'location':'nanjing'910}1112
- python web开发flask库安装与使用
范哥来了
python前端flask
要在Python中使用Flask进行Web开发,首先需要安装Flask库。Flask是一个轻量级的Web框架,它使开发者能够快速构建网站或web服务。下面是安装Flask和创建一个简单的Flask应用程序的基本步骤。安装Flask确保您的环境中已经安装了Python(推荐版本3.7或更高)。接着,您可以通过pip来安装Flask。打开命令行工具(如终端或命令提示符),然后执行以下命令:pipins
- python-flask复习(一)
胖虎是只mao
python-webpython函数pythonpythonflask
一、Python现阶段三大主流Web框架Django、Tornado、Flask对比Django主要特点是大而全,集成了很多组件(例如Models、Admin、Form等等),不管你用得到用不到,反正它全都有,属于全能型框架,通常用于大型Web应用,由于内置组件足够强大所以使用Django开发可以一气呵成,优点是大而全,缺点也就暴露出来了,这么多的资源一次性全部加载,肯定会造成一部分的资源浪费;T
- 基于 Docker 和 Flask 构建高并发微服务架构
TechStack 创行者
#服务器容器Linux架构dockerflask容器微服务
基于Docker和Flask构建高并发微服务架构一、微服务架构概述(一)微服务架构的优点微服务架构是一种将应用程序拆分为多个小型、自治服务的架构风格,在当今的软件开发领域具有显著的优势。高度可扩展性:每个微服务可以独立进行扩展。例如,在电商系统中,订单服务在促销活动期间可能会面临高并发的订单处理需求,此时可以仅对订单服务进行横向扩展,增加服务实例数量,而无需对整个系统进行大规模的扩容,从而提高资源
- python-56-基于Vue和Flask进行前后端分离的项目开发示例实战
皮皮冰燃
python3pythonvue.jsflask
文章目录1创建Vue前端项目1.1运行demo1.2实现需求2flask部署上述dist(前后端未分离)2.1代码app.py2.2运行访问3nginx部署(前后端分离)3.1nginx前端服务3.3.1windows安装nginx3.3.2修改nginx.conf配置文件3.3.3启动nginx3.3.3停止nginx3.2启动后端服务3.2.1app.py(去除前端渲染)3.2.2启动flas
- Python,C++开发餐饮后厨环境远程管理APP
Geeker-2025
pythonc++
开发一款用于**餐饮后厨环境远程管理**的App,结合Python和C++的优势,可以实现高效的后端数据处理、实时的环境监控以及用户友好的前端界面。以下是一个详细的开发方案,涵盖技术选型、功能模块、开发步骤等内容。##技术选型###后端(Python)-**编程语言**:Python-**Web框架**:Django或Flask-**数据库**:PostgreSQL或MySQL-**实时通信**:
- 基于Python的大学生思想政治教育平台mysql(Django Flask Vue Pycharm )
QQ_188083800
pythonmysqldjango
文章目录具体实现截图项目技术介绍研究方案源码获取详细视频演示:文章底部获取博主联系方式!!!!系统设计核心代码部分展示django项目示例源码/演示视频获取方式具体实现截图项目技术介绍我国主流校园使用的是传统开发基于Java语言通过SpringBoot框架开发管理系统,开发周期长,开发人员学习成本高。使用如Django或Flask开发框架可以大量的减少开发者需要写的代码量,使开发人员可以最少的代码
- 如何使用 Python 实现简单的 Web 服务器?
程序员黄同学
Python面试题Pythonpython
为了实现一个简单的Web服务器,Python提供了多种方法。对于快速原型设计和学习目的来说,最简单的方法之一是使用内置的http.server模块。然而,在实际开发中,更常见的做法是使用像Flask或Django这样的框架来构建更为复杂的应用程序。下面我将介绍如何用Python创建一个基本的Web服务器,并提供一些实用建议和注意事项。我们将从最基础的开始,然后逐步深入到更复杂的场景。使用http.
- 【python】Python中常见的KeyError报错分析
景天科技苑
python开发语言python报错KeyError
✨✨欢迎大家来到景天科技苑✨✨养成好习惯,先赞后看哦~作者简介:景天科技苑《头衔》:大厂架构师,华为云开发者社区专家博主,阿里云开发者社区专家博主,CSDN全栈领域优质创作者,掘金优秀博主,51CTO博客专家等。《博客》:Python全栈,前后端开发,小程序开发,人工智能,js逆向,App逆向,网络系统安全,数据分析,Django,fastapi,flask等框架,linux,shell脚本等实操
- Python与C ++开发匿名捐赠1对1管理APP
Geeker-2025
pythonc++
开发一款用于**匿名捐赠1对1管理**的App,结合Python和C++的优势,可以实现高效的后端数据处理、实时的捐赠监控以及用户友好的前端界面。以下是一个详细的开发方案,涵盖技术选型、功能模块、开发步骤等内容。##技术选型###后端(Python)-**编程语言**:Python-**Web框架**:Django或Flask-**数据库**:PostgreSQL或MySQL-**实时通信**:W
- 【Python】Flask与Django对比详解:教你如何选择最适合你的Web框架
小芬熊
面试学习路线阿里巴巴pythonflaskdjango
文章目录引言:为何选择PythonWeb框架?Flask简介:轻量级的灵活之选??Flask的核心特点Django简介:全能型的强大框架??Django的核心特点Flask与Django的详细对比架构设计功能与扩展性性能与效率模板系统ORM(对象关系映射)详细对比表格适用场景总结案例分享:如何选择适合的框架小李的博文项目:选择Flask??小张的电商平台:选择Django??了解更多AI内容结论:
- python的后端开发框架django,flask,flaskapi
myjzwsz
pythondjangoflask
Django、Flask和Flask-API是Python中流行的后端开发框架,它们在功能、应用场景以及架构上有不同的特点和使用场景。下面我给你详细介绍每个框架的应用示例、区别和应用场景:1.DjangoDjango是一个功能全面的Web开发框架,强调“快速开发”和“无需重新发明轮子”。它自带了很多功能,如认证、ORM(数据库映射)、表单处理、管理后台等。应用示例:社交媒体平台:像Instagra
- python基于django/flask体育馆管理系统Django-SpringBoot-php-Node.js-flask
QQ_511008285
pythondjangoflaskspringbootphpnode.js
目录技术栈介绍具体实现截图系统设计研究方法:设计步骤设计流程核心代码部分展示研究方法详细视频演示试验方案论文大纲源码获取/详细视频演示技术栈介绍Django-SpringBoot-php-Node.js-flask本课题的研究方法和研究步骤基本合理,难度适中,本选题是学生所学专业知识的延续,符合学生专业发展方向,对于提高学生的基本知识和技能以及钻研能力有益。该学生能够在预定时间内完成该课题的设计。
- Flask应用调试模式下外网访问的技巧
带娃的IT创业者
flaskpython后端
Flask应用调试模式下外网访问的技巧在调试模式下让外网访问你的Flask应用需要注意安全性问题,因为调试模式会暴露更多信息。以下是几种方法让你在开发过程中从外网访问你的应用:方法一:修改Flask运行参数最简单的方法是修改Flask的运行参数,让它监听所有网络接口:flaskrun--host=0.0.0.0--port=5000或者在Python脚本中:python-c"fromappimpo
- PyQt6内嵌http.server Web 和Flask Web服务器方法详解
mosquito_lover1
pythonpyqtflaskhttp
PyQt6可以内嵌一个简单的Web服务器。虽然PyQt6本身不提供直接的Web服务器功能,但可以结合Python的标准库(如http.server)或其他Web框架(如Flask、FastAPI等)来实现。示例:使用http.server内嵌Web服务器以下是一个简单的例子,展示如何在PyQt6应用中内嵌一个基本的Web服务器:importsysfromPyQt6.QtWidgetsimportQ
- python+MySQL+HTML实现自习室座位管理系统
IT小本本
pythonpythonmysqlhtml
自习室座位管理系统项目介绍自习室座位管理系统是一个基于PythonFlask框架开发的Web应用,旨在提供高效、便捷的自习室座位预约和管理功能。该系统适用于学校图书馆、自习室等场所,帮助管理员有效管理座位资源,同时为学生提供便捷的座位预约服务。功能特点1、用户认证模块用户注册:学生可以注册账号,填写个人信息用户登录:支持学生和管理员登录找回密码:通过邮箱验证重置密码2、座位管理模块座位预约:学生可
- python基于django/flask网上书城系统Django-SpringBoot-php-Node.js-flask
QQ_1963288475
pythondjangoflaskspringbootphplaravelnode.js
目录技术栈介绍具体实现截图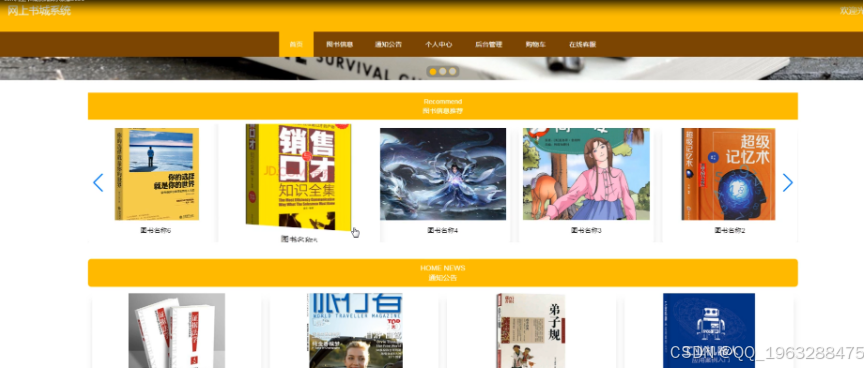系统设计研究方法:设计步骤设计流程核心代码部分展示研究方法详细视频演示试验方案论文大纲源码获取/详细视频演示技术栈介绍Django-SpringBoot-php-Node.js-flask本课题的研究方法和研
- 【DeepSeek应用】本地部署deepseek模型后,如何在vscode中调用该模型进行代码撰写,检视和优化?
AndrewHZ
深度学习新浪潮AI算法工程师面试指北vscode人工智能深度学习DeepSeek算法语言模型编辑器
若已成功在本地部署了DeepSeek模型(例如通过vscode-llm、ollama或私有API服务),在VSCode中调用本地模型进行代码撰写、检视和优化的完整流程如下:1.准备工作:确认本地模型服务状态模型服务类型:若使用HTTPAPI服务(如FastAPI/Flask封装),假设服务地址为http://localhost:8000。若使用ollama部署,模型名称为deepseek,调用命令
- 使用Python Flask构建Web应用程序
代码快速拳
pythonflask前端Python
Flask是一个轻量级的PythonWeb框架,它提供了构建Web应用程序所需的基本功能。它简单易用,非常适合小型项目和原型开发。本文将介绍如何使用Flask构建一个简单的Web应用程序,并提供相应的源代码。首先,我们需要安装Flask。可以使用以下命令使用pip安装Flask:pipinstallflask一旦安装完成,我们就可以开始构建我们的Web应用程序了。首先,创建一个Python文件,命
- 2024年一文1800字从0到1使用Python Flask实战构建Web应用(1)
2401_84564025
程序员pythonflask前端
现在我也找了很多测试的朋友,做了一个分享技术的交流群,共享了很多我们收集的技术文档和视频教程。如果你不想再体验自学时找不到资源,没人解答问题,坚持几天便放弃的感受可以加入我们一起交流。而且还有很多在自动化,性能,安全,测试开发等等方面有一定建树的技术大牛分享他们的经验,还会分享很多直播讲座和技术沙龙可以免费学习!划重点!开源的!!!qq群号:110685036第三部分:运行Flask应用在app.
- 【python web】一文掌握 Flask 的基础用法
数据知道
python前端flask
文章目录一、Flask介绍1.1安装Flask二、Flask的基本使用2.1创建第一个Flask应用2.2路由与视图函数2.3请求与响应2.4响应对象2.5模板渲染2.6模板继承2.7静态文件管理2.8Blueprint蓝图2.9错误处理三、Flask扩展与插件四、部署Flask应用五、总结Flask是一个轻量级的PythonWeb框架,因其简单易用、灵活性高而受到广泛欢迎。本文将全面介绍Flas
- open-webui使用searXNG插件连接自定义的联网搜索服务程序
chinayeren
教程pythonaillamachatgpt
项目背景因为国内无法访问内置的一些免费搜索插件,安装完searXNG本地服务端后根据教程中连接始终无法连接,docker方案国内也无法使用的情况下,本地使用python写一个Flask服务程序使用爬虫技术提供联网搜索数据。下面是实现代码V1#!/usr/bin/python3#_*_coding:utf-8_*_##Copyright(C)2025-2025#@Title:这是一个模拟searXN
- python+django+vue医院门诊挂号预约管理系统57wsx
QQ_402205496
djangopycharmpython
开发语言:Python框架:django/flaskPython版本:python3.7.7数据库:mysql数据库工具:Navicat开发软件:PyCharm主要功能有:管理员功能:系统首页、个人中心、用户管理、医生管理、疫情公告管理、行动轨迹管理、异样报告管理、科室信息管理、异常报告管理、系统管理、医院信息管理、预约信息管理、在线聊天管理、聊天回复管理、我的收藏管理。用户功能模块:系统首页、个
- 【flask扩展】Flask-SQLAlchemy的安装与配置
爱音斯坦牛
flask框架从入门到实战flaskpython后端
个人简介作者简介:大家好,我是阿牛,全栈领域新星创作者。博主的个人网站:阿牛的博客小屋支持我:点赞+收藏⭐️+留言系列专栏:flask框架快速入门格言:要成为光,因为有怕黑的人!目录个人简介前言Flask-SQLAlchemy的介绍与数据库驱动的选择Flask-SQLAlchemy与flask-mysqldb的安装Flask-SQLAlchemy的配置其他常用的SQLAlchemy字段类型常用的S
- Flask 学习-95.Flask-SQLAlchemy 查询今天当天的数据
上海-悠悠
flaskflask学习python
前言查询今天的数据,或者查询某一天的数据SQLDATE()function使我们能够从特定的历史或当前时间戳值访问日期值。DATE()函数Date()函数返回从传递的datetime表达式中提取的日期。DATE(datetimeexpression)SQL语句按create_time获取某一天的数据select*frommytablewhereDATE(create_time)=='2022-11
- 【手把手教你-Python】如何让 Flask 根据现有表结构生成 SQLAlchemy 模型文件?
EricLing2022
pythonflask开发语言sqldatabase
首先你要具备一定Python基础,了解Flask框架,以及数据库工具SQLAchemy。为什么会有这个需求?在使用Python编写项目代码时,假设数据库中的表已经存在,我们需要根据现有的表结构来编写SQLAlchemy模型文件。为了避免逐行编写代码,我们可以使用一个工具来“一键生成”模型文件,那就是sqlacodegen。准备工作Python版本:3.11.5(只要是3.xx版本应该都可以)安装好
- Flask 框架app = Flask(__name__) 解析
Nolannk
flask框架
fromflaskimportFlaskapp=Flask(name)@app.route(‘/’)defhello_world():return‘HelloWorld!’ifname==‘main‘:app.run(host=’0.0.0.0’,port=9000)第4行,引入Flask类,Flask类实现了一个WSGI应用第5行,app是Flask的实例,它接收包或者模块的名字作为参数,但一般
- python记录6from flask import Flaskapp = Flask(__name__) @app.route(‘/‘)def hello_world(): retu
我讨厌python
python开发语言后端
利用pycharm实现视频分镜1.下载pip3installpycharm或者官网下载2.网页显示文字步骤想要在网页上显示:Hello,World!第一步先在PyCharm上新建项目然后在main.py中输入以下代码fromflaskimportFlaskapp=Flask(__name__)@app.route('/')defhello_world():return'Hello,World!'i
- 项目中 枚举与注解的结合使用
飞翔的马甲
javaenumannotation
前言:版本兼容,一直是迭代开发头疼的事,最近新版本加上了支持新题型,如果新创建一份问卷包含了新题型,那旧版本客户端就不支持,如果新创建的问卷不包含新题型,那么新旧客户端都支持。这里面我们通过给问卷类型枚举增加自定义注解的方式完成。顺便巩固下枚举与注解。
一、枚举
1.在创建枚举类的时候,该类已继承java.lang.Enum类,所以自定义枚举类无法继承别的类,但可以实现接口。
- 【Scala十七】Scala核心十一:下划线_的用法
bit1129
scala
下划线_在Scala中广泛应用,_的基本含义是作为占位符使用。_在使用时是出问题非常多的地方,本文将不断完善_的使用场景以及所表达的含义
1. 在高阶函数中使用
scala> val list = List(-3,8,7,9)
list: List[Int] = List(-3, 8, 7, 9)
scala> list.filter(_ > 7)
r
- web缓存基础:术语、http报头和缓存策略
dalan_123
Web
对于很多人来说,去访问某一个站点,若是该站点能够提供智能化的内容缓存来提高用户体验,那么最终该站点的访问者将络绎不绝。缓存或者对之前的请求临时存储,是http协议实现中最核心的内容分发策略之一。分发路径中的组件均可以缓存内容来加速后续的请求,这是受控于对该内容所声明的缓存策略。接下来将讨web内容缓存策略的基本概念,具体包括如如何选择缓存策略以保证互联网范围内的缓存能够正确处理的您的内容,并谈论下
- crontab 问题
周凡杨
linuxcrontabunix
一: 0481-079 Reached a symbol that is not expected.
背景:
*/5 * * * * /usr/IBMIHS/rsync.sh
- 让tomcat支持2级域名共享session
g21121
session
tomcat默认情况下是不支持2级域名共享session的,所有有些情况下登陆后从主域名跳转到子域名会发生链接session不相同的情况,但是只需修改几处配置就可以了。
打开tomcat下conf下context.xml文件
找到Context标签,修改为如下内容
如果你的域名是www.test.com
<Context sessionCookiePath="/path&q
- web报表工具FineReport常用函数的用法总结(数学和三角函数)
老A不折腾
Webfinereport总结
ABS
ABS(number):返回指定数字的绝对值。绝对值是指没有正负符号的数值。
Number:需要求出绝对值的任意实数。
示例:
ABS(-1.5)等于1.5。
ABS(0)等于0。
ABS(2.5)等于2.5。
ACOS
ACOS(number):返回指定数值的反余弦值。反余弦值为一个角度,返回角度以弧度形式表示。
Number:需要返回角
- linux 启动java进程 sh文件
墙头上一根草
linuxshelljar
#!/bin/bash
#初始化服务器的进程PId变量
user_pid=0;
robot_pid=0;
loadlort_pid=0;
gateway_pid=0;
#########
#检查相关服务器是否启动成功
#说明:
#使用JDK自带的JPS命令及grep命令组合,准确查找pid
#jps 加 l 参数,表示显示java的完整包路径
#使用awk,分割出pid
- 我的spring学习笔记5-如何使用ApplicationContext替换BeanFactory
aijuans
Spring 3 系列
如何使用ApplicationContext替换BeanFactory?
package onlyfun.caterpillar.device;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.xml.XmlBeanFactory;
import
- Linux 内存使用方法详细解析
annan211
linux内存Linux内存解析
来源 http://blog.jobbole.com/45748/
我是一名程序员,那么我在这里以一个程序员的角度来讲解Linux内存的使用。
一提到内存管理,我们头脑中闪出的两个概念,就是虚拟内存,与物理内存。这两个概念主要来自于linux内核的支持。
Linux在内存管理上份为两级,一级是线性区,类似于00c73000-00c88000,对应于虚拟内存,它实际上不占用
- 数据库的单表查询常用命令及使用方法(-)
百合不是茶
oracle函数单表查询
创建数据库;
--建表
create table bloguser(username varchar2(20),userage number(10),usersex char(2));
创建bloguser表,里面有三个字段
&nbs
- 多线程基础知识
bijian1013
java多线程threadjava多线程
一.进程和线程
进程就是一个在内存中独立运行的程序,有自己的地址空间。如正在运行的写字板程序就是一个进程。
“多任务”:指操作系统能同时运行多个进程(程序)。如WINDOWS系统可以同时运行写字板程序、画图程序、WORD、Eclipse等。
线程:是进程内部单一的一个顺序控制流。
线程和进程
a. 每个进程都有独立的
- fastjson简单使用实例
bijian1013
fastjson
一.简介
阿里巴巴fastjson是一个Java语言编写的高性能功能完善的JSON库。它采用一种“假定有序快速匹配”的算法,把JSON Parse的性能提升到极致,是目前Java语言中最快的JSON库;包括“序列化”和“反序列化”两部分,它具备如下特征:  
- 【RPC框架Burlap】Spring集成Burlap
bit1129
spring
Burlap和Hessian同属于codehaus的RPC调用框架,但是Burlap已经几年不更新,所以Spring在4.0里已经将Burlap的支持置为Deprecated,所以在选择RPC框架时,不应该考虑Burlap了。
这篇文章还是记录下Burlap的用法吧,主要是复制粘贴了Hessian与Spring集成一文,【RPC框架Hessian四】Hessian与Spring集成
 
- 【Mahout一】基于Mahout 命令参数含义
bit1129
Mahout
1. mahout seqdirectory
$ mahout seqdirectory
--input (-i) input Path to job input directory(原始文本文件).
--output (-o) output The directory pathna
- linux使用flock文件锁解决脚本重复执行问题
ronin47
linux lock 重复执行
linux的crontab命令,可以定时执行操作,最小周期是每分钟执行一次。关于crontab实现每秒执行可参考我之前的文章《linux crontab 实现每秒执行》现在有个问题,如果设定了任务每分钟执行一次,但有可能一分钟内任务并没有执行完成,这时系统会再执行任务。导致两个相同的任务在执行。
例如:
<?
//
test
.php
- java-74-数组中有一个数字出现的次数超过了数组长度的一半,找出这个数字
bylijinnan
java
public class OcuppyMoreThanHalf {
/**
* Q74 数组中有一个数字出现的次数超过了数组长度的一半,找出这个数字
* two solutions:
* 1.O(n)
* see <beauty of coding>--每次删除两个不同的数字,不改变数组的特性
* 2.O(nlogn)
* 排序。中间
- linux 系统相关命令
candiio
linux
系统参数
cat /proc/cpuinfo cpu相关参数
cat /proc/meminfo 内存相关参数
cat /proc/loadavg 负载情况
性能参数
1)top
M:按内存使用排序
P:按CPU占用排序
1:显示各CPU的使用情况
k:kill进程
o:更多排序规则
回车:刷新数据
2)ulimit
ulimit -a:显示本用户的系统限制参
- [经营与资产]保持独立性和稳定性对于软件开发的重要意义
comsci
软件开发
一个软件的架构从诞生到成熟,中间要经过很多次的修正和改造
如果在这个过程中,外界的其它行业的资本不断的介入这种软件架构的升级过程中
那么软件开发者原有的设计思想和开发路线
- 在CentOS5.5上编译OpenJDK6
Cwind
linuxOpenJDK
几番周折终于在自己的CentOS5.5上编译成功了OpenJDK6,将编译过程和遇到的问题作一简要记录,备查。
0. OpenJDK介绍
OpenJDK是Sun(现Oracle)公司发布的基于GPL许可的Java平台的实现。其优点:
1、它的核心代码与同时期Sun(-> Oracle)的产品版基本上是一样的,血统纯正,不用担心性能问题,也基本上没什么兼容性问题;(代码上最主要的差异是
- java乱码问题
dashuaifu
java乱码问题js中文乱码
swfupload上传文件参数值为中文传递到后台接收中文乱码 在js中用setPostParams({"tag" : encodeURI( document.getElementByIdx_x("filetag").value,"utf-8")});
然后在servlet中String t
- cygwin很多命令显示command not found的解决办法
dcj3sjt126com
cygwin
cygwin很多命令显示command not found的解决办法
修改cygwin.BAT文件如下
@echo off
D:
set CYGWIN=tty notitle glob
set PATH=%PATH%;d:\cygwin\bin;d:\cygwin\sbin;d:\cygwin\usr\bin;d:\cygwin\usr\sbin;d:\cygwin\us
- [介绍]从 Yii 1.1 升级
dcj3sjt126com
PHPyii2
2.0 版框架是完全重写的,在 1.1 和 2.0 两个版本之间存在相当多差异。因此从 1.1 版升级并不像小版本间的跨越那么简单,通过本指南你将会了解两个版本间主要的不同之处。
如果你之前没有用过 Yii 1.1,可以跳过本章,直接从"入门篇"开始读起。
请注意,Yii 2.0 引入了很多本章并没有涉及到的新功能。强烈建议你通读整部权威指南来了解所有新特性。这样有可能会发
- Linux SSH免登录配置总结
eksliang
ssh-keygenLinux SSH免登录认证Linux SSH互信
转载请出自出处:http://eksliang.iteye.com/blog/2187265 一、原理
我们使用ssh-keygen在ServerA上生成私钥跟公钥,将生成的公钥拷贝到远程机器ServerB上后,就可以使用ssh命令无需密码登录到另外一台机器ServerB上。
生成公钥与私钥有两种加密方式,第一种是
- 手势滑动销毁Activity
gundumw100
android
老是效仿ios,做android的真悲催!
有需求:需要手势滑动销毁一个Activity
怎么办尼?自己写?
不用~,网上先问一下百度。
结果:
http://blog.csdn.net/xiaanming/article/details/20934541
首先将你需要的Activity继承SwipeBackActivity,它会在你的布局根目录新增一层SwipeBackLay
- JavaScript变换表格边框颜色
ini
JavaScripthtmlWebhtml5css
效果查看:http://hovertree.com/texiao/js/2.htm代码如下,保存到HTML文件也可以查看效果:
<html>
<head>
<meta charset="utf-8">
<title>表格边框变换颜色代码-何问起</title>
</head>
<body&
- Kafka Rest : Confluent
kane_xie
kafkaRESTconfluent
最近拿到一个kafka rest的需求,但kafka暂时还没有提供rest api(应该是有在开发中,毕竟rest这么火),上网搜了一下,找到一个Confluent Platform,本文简单介绍一下安装。
这里插一句,给大家推荐一个九尾搜索,原名叫谷粉SOSO,不想fanqiang谷歌的可以用这个。以前在外企用谷歌用习惯了,出来之后用度娘搜技术问题,那匹配度简直感人。
环境声明:Ubu
- Calender不是单例
men4661273
单例Calender
在我们使用Calender的时候,使用过Calendar.getInstance()来获取一个日期类的对象,这种方式跟单例的获取方式一样,那么它到底是不是单例呢,如果是单例的话,一个对象修改内容之后,另外一个线程中的数据不久乱套了吗?从试验以及源码中可以得出,Calendar不是单例。
测试:
Calendar c1 =
- 线程内存和主内存之间联系
qifeifei
java thread
1, java多线程共享主内存中变量的时候,一共会经过几个阶段,
lock:将主内存中的变量锁定,为一个线程所独占。
unclock:将lock加的锁定解除,此时其它的线程可以有机会访问此变量。
read:将主内存中的变量值读到工作内存当中。
load:将read读取的值保存到工作内存中的变量副本中。
- schedule和scheduleAtFixedRate
tangqi609567707
javatimerschedule
原文地址:http://blog.csdn.net/weidan1121/article/details/527307
import java.util.Timer;import java.util.TimerTask;import java.util.Date;
/** * @author vincent */public class TimerTest {
 
- erlang 部署
wudixiaotie
erlang
1.如果在启动节点的时候报这个错 :
{"init terminating in do_boot",{'cannot load',elf_format,get_files}}
则需要在reltool.config中加入
{app, hipe, [{incl_cond, exclude}]},
2.当generate时,遇到:
ERROR