1、引入maven依赖
com.netflix.hystrix
hystrix-core
1.5.12
2、编写一个动态出现超时异常的继承HystrixCommand的类
package com.xueyou.hystrixdemo.v3;
import com.netflix.hystrix.HystrixCommand;
import java.util.Arrays;
import java.util.List;
public class CommandHelloworldWithFallBack extends HystrixCommand {
public static List times = Arrays.asList(100, 200, 300, 400, 500, 600,
700, 800, 900, 1000, 1100, 1200, 1300, 1400, 1500, 1600, 1700, 1800, 1900, 2000, 2100);
private String name;
private int timeIndex;
public CommandHelloworldWithFallBack(Setter setter, String name, int timeIndex) {
super(setter);
this.name = name;
this.timeIndex = timeIndex;
}
@Override
protected String getFallback() {
return "fall back timeMillSeconds is :" + times.get(timeIndex);
}
@Override
protected String run() {
try {
Thread.currentThread().sleep(times.get(this.getTimeIndex()));
} catch (InterruptedException e) {
}
return "ok timeMillSeconds is :" + times.get(timeIndex);
}
public int getTimeIndex() {
return timeIndex;
}
public void setTimeIndex(int timeIndex) {
this.timeIndex = timeIndex;
}
}
3、编写一些测试类进行测试
package com.xueyou.hystrixdemo.v3;
import com.netflix.hystrix.*;
public class HelloWorldFallBack {
public static void main(String[] args) {
int count = 0;
while (true) {
String s = new CommandHelloworldWithFallBack(HystrixCommand.Setter.withGroupKey(HystrixCommandGroupKey.Factory.asKey("ExampleGroup"))
.andThreadPoolKey(HystrixThreadPoolKey.Factory.asKey("threadpoolwithfallback"))
.andThreadPoolPropertiesDefaults(HystrixThreadPoolProperties.Setter()
.withCoreSize(10)
.withMaximumSize(10))
.andCommandPropertiesDefaults(HystrixCommandProperties.Setter()
.withExecutionTimeoutInMilliseconds(1000)
.withCircuitBreakerSleepWindowInMilliseconds(5000)
.withCircuitBreakerErrorThresholdPercentage(50)
.withCircuitBreakerRequestVolumeThreshold(1))
, "ccc", count % 20).execute();
System.out.println(s);
count++;
try {
Thread.currentThread().sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
}
}
}
}
4、运行结果:
ok timeMillSeconds is :100
ok timeMillSeconds is :200
ok timeMillSeconds is :300
ok timeMillSeconds is :400
ok timeMillSeconds is :500
ok timeMillSeconds is :600
ok timeMillSeconds is :700
ok timeMillSeconds is :800
ok timeMillSeconds is :900
ok timeMillSeconds is :1000
fall back timeMillSeconds is :1100
fall back timeMillSeconds is :1200
fall back timeMillSeconds is :1300
fall back timeMillSeconds is :1400
fall back timeMillSeconds is :1500
fall back timeMillSeconds is :1600
fall back timeMillSeconds is :1700
fall back timeMillSeconds is :1800
fall back timeMillSeconds is :1900
fall back timeMillSeconds is :2000
fall back timeMillSeconds is :100
fall back timeMillSeconds is :200
fall back timeMillSeconds is :300
fall back timeMillSeconds is :400
fall back timeMillSeconds is :500
fall back timeMillSeconds is :600
fall back timeMillSeconds is :700
fall back timeMillSeconds is :800
fall back timeMillSeconds is :900
fall back timeMillSeconds is :1000
fall back timeMillSeconds is :1100
fall back timeMillSeconds is :1200
fall back timeMillSeconds is :1300
fall back timeMillSeconds is :1400
fall back timeMillSeconds is :1500
fall back timeMillSeconds is :1600
fall back timeMillSeconds is :1700
fall back timeMillSeconds is :1800
fall back timeMillSeconds is :1900
fall back timeMillSeconds is :2000
fall back timeMillSeconds is :100
fall back timeMillSeconds is :200
fall back timeMillSeconds is :300
fall back timeMillSeconds is :400
fall back timeMillSeconds is :500
fall back timeMillSeconds is :600
fall back timeMillSeconds is :700
fall back timeMillSeconds is :800
fall back timeMillSeconds is :900
fall back timeMillSeconds is :1000
fall back timeMillSeconds is :1100
fall back timeMillSeconds is :1200
fall back timeMillSeconds is :1300
fall back timeMillSeconds is :1400
fall back timeMillSeconds is :1500
fall back timeMillSeconds is :1600
fall back timeMillSeconds is :1700
fall back timeMillSeconds is :1800
fall back timeMillSeconds is :1900
fall back timeMillSeconds is :2000
fall back timeMillSeconds is :100
fall back timeMillSeconds is :200
fall back timeMillSeconds is :300
fall back timeMillSeconds is :400
fall back timeMillSeconds is :500
fall back timeMillSeconds is :600
fall back timeMillSeconds is :700
fall back timeMillSeconds is :800
fall back timeMillSeconds is :900
fall back timeMillSeconds is :1000
fall back timeMillSeconds is :1100
fall back timeMillSeconds is :1200
fall back timeMillSeconds is :1300
fall back timeMillSeconds is :1400
fall back timeMillSeconds is :1500
fall back timeMillSeconds is :1600
fall back timeMillSeconds is :1700
fall back timeMillSeconds is :1800
fall back timeMillSeconds is :1900
fall back timeMillSeconds is :2000
fall back timeMillSeconds is :100
fall back timeMillSeconds is :200
fall back timeMillSeconds is :300
fall back timeMillSeconds is :400
fall back timeMillSeconds is :500
fall back timeMillSeconds is :600
fall back timeMillSeconds is :700
fall back timeMillSeconds is :800
fall back timeMillSeconds is :900
fall back timeMillSeconds is :1000
fall back timeMillSeconds is :1100
fall back timeMillSeconds is :1200
fall back timeMillSeconds is :1300
fall back timeMillSeconds is :1400
fall back timeMillSeconds is :1500
fall back timeMillSeconds is :1600
fall back timeMillSeconds is :1700
fall back timeMillSeconds is :1800
fall back timeMillSeconds is :1900
fall back timeMillSeconds is :2000
fall back timeMillSeconds is :100
fall back timeMillSeconds is :200
fall back timeMillSeconds is :300
fall back timeMillSeconds is :400
fall back timeMillSeconds is :500
fall back timeMillSeconds is :600
fall back timeMillSeconds is :700
ok timeMillSeconds is :800
ok timeMillSeconds is :900
ok timeMillSeconds is :1000
fall back timeMillSeconds is :1100
fall back timeMillSeconds is :1200
fall back timeMillSeconds is :1300
fall back timeMillSeconds is :1400
结果分析:
1、首先在run()方法没有超时的时候,是正常返回的,没有走getFallback()。
然后,时间超时了,这是时候会走getFallback()。
2、那么什么时候发生熔断呢,或者说熔断的触发条件是什么呢?(这里先不讨论线程是满了的情况,也就是说假设线程池足够用)
- withExecutionTimeoutInMilliseconds(1000)==>run()方法的执行时间超过最大阈值毫秒数。如果超过了,那么hystrix会当做本次为一次错误。
官网解释:
execution.isolation.thread.timeoutInMilliseconds
This property sets the time in milliseconds after which the caller will observe a timeout and walk away from the command execution. Hystrix marks the
HystrixCommand
as a TIMEOUT, and performs fallback logic. Note that there is configuration for turning off timeouts per-command, if that is desired (see command.timeout.enabled).
- withCircuitBreakerRequestVolumeThreshold(1)==>代表在一个rolling window内发生的所有请求的个数。如果这个个数很少,那么不会触发熔断,只有大于这阈值,才有可能触发熔断。
官网的解释是这样的:
circuitBreaker.requestVolumeThreshold
This property sets the minimum number of requests in a rolling window that will trip the circuit.
For example, if the value is 20, then if only 19 requests are received in the rolling window (say a window of 10 seconds) the circuit will not trip open even if all 19 failed.
- withCircuitBreakerErrorThresholdPercentage(50) ==>发生问题的请求占所有请求的百分比阈值(在一个rolling window内的所有请求里)。
这个是官网的解释:
circuitBreaker.errorThresholdPercentage
This property sets the error percentage at or above which the circuit should trip open and start short-circuiting requests to fallback logic.
从上面的解释我们能够看到熔断的触发条件首先在指定rolling window内请求的个数大于设定的阈值;同时,出现错误的请求个数占总请求数的百分比大于设置的百分比。
补充一下,rolling window这么关键,那么如何设置rolling window的大小呢?
metrics.rollingStats.timeInMilliseconds
3、什么时候熔断会自动恢复呢(也就是说什么条件才会触发熔断自动恢复呢)?
- withCircuitBreakerSleepWindowInMilliseconds(5000)==>当已经熔断的时候经过设定的毫秒数后,会重新走一次run()方法(熔断开始后,所有的请求会直接走getFallback(),不会走run()方法的)。也就是说,如果熔断了,没隔5秒会执行一下run()方法,看一下是否能够正常执行,如果能够正常执行,那么熔断自动恢复,否则继续处于熔断状态。
下面是官网的解释:
circuitBreaker.sleepWindowInMilliseconds
This property sets the amount of time, after tripping the circuit, to reject requests before allowing attempts again to determine if the circuit should again be closed.
最后附上异常hystrix的流程图
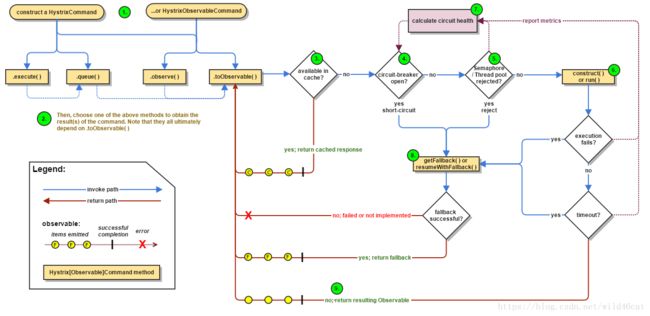