用到的技术
- nodejs搭建本地http服务器
- 应用node-http-proxy,做接口url的转发
搭建过程
1. 前提本地已安装node
2. npm初始化
npm init
3. 安装node-http-proxy模块
npm install http-proxy --save-dev
4. 项目结构示例
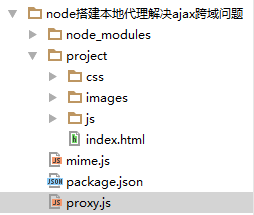
项目结构.png
- node_modules是安装的包
- project是自己在做的项目文件
- 项目文件中的入口index.html相对于project放在他的根目录下
- mime.js是自己做的后缀名关于文件类型 Content-Type的映射关系
- package.json是你npm init时初始化信息的文件
- proxy.js是需要node运行的代理服务文件
5. 关于mime文件的内容
exports.types = {
"css": "text/css",
"gif": "image/gif",
"html": "text/html",
"ico": "image/x-icon",
"jpeg": "image/jpeg",
"jpg": "image/jpeg",
"js": "text/javascript",
"json": "application/json",
"pdf": "application/pdf",
"png": "image/png",
"svg": "image/svg+xml",
"swf": "application/x-shockwave-flash",
"tiff": "image/tiff",
"txt": "text/plain",
"wav": "audio/x-wav",
"wma": "audio/x-ms-wma",
"wmv": "video/x-ms-wmv",
"xml": "text/xml",
"woff": "application/x-woff",
"woff2": "application/x-woff2",
"tff": "application/x-font-truetype",
"otf": "application/x-font-opentype",
"eot": "application/vnd.ms-fontobject"
};
- 也可以使用node的第三方模块 mime即可
npm install mime --save-dev
6. 关于proxy文件的内容
var PORT = 4000;
var http = require('http');
var url=require('url');
var fs=require('fs');
var mine=require('./mime').types;
var path=require('path');
var httpProxy = require('http-proxy');
//自己调整的变量target,rootfile
var target='http://microblog.people.com.cn' //接口协议域名端口
var rootfile ='./project/'; //index.html文件的相对路径
var proxy = httpProxy.createProxyServer({
target: target, //配置接口协议域名端口
// 下面用于设置https
// ssl: {
// key: fs.readFileSync('server_decrypt.key', 'utf8'),
// cert: fs.readFileSync('server.crt', 'utf8')
// },
// secure: false
})
proxy.on('error', function(err, req, res){
res.writeHead(500, {
'content-type': 'text/plain'
});
res.end('Something went wrong. And we are reporting a custom error message.');
});
var server = http.createServer(function (request, response) {
var pathname = url.parse(request.url).pathname;//得到请求的文件名
var realPath = path.join(rootfile, pathname);//得到请求的项目文件地址 注意这里要和文件地址对上
var ext = path.extname(realPath);//得到文件后缀
ext = ext ? ext.slice(1) : 'unknown';//文件后缀去掉‘.’ Content-Type
//判断如果是接口访问,则通过proxy转发
if(pathname.indexOf("action") > 0){//通过接口的关键字判断是不是接口,一般接口都已action结尾,如果是接口做以下处理
proxy.web(request, response);//代理请求
return;
}
//以下是请求本地文件
fs.exists(realPath, function (exists) {
if (!exists) {
response.writeHead(404, {
'Content-Type': 'text/plain'
});
response.write("This request URL " + pathname + " was not found on this server.");
response.end();
} else {
fs.readFile(realPath, "binary", function (err, file) {
if (err) {
response.writeHead(500, {
'Content-Type': 'text/plain'
});
response.end(err);
} else {
var contentType = mine[ext] || "text/plain";
response.writeHead(200, {
'Content-Type': contentType
});
response.write(file, "binary");
response.end();
}
});
}
});
});
server.listen(PORT);
7. index.html中ajax请求示例
- 用本地搭建的协议域名端口 http://localhost:4000 代替 真实接口的协议域名端口 http://microblog.people.com.cn。
//get验证
$.ajax({
url:'http://localhost:4000/queryComment.action',
type:'get',
success:function(data){
console.log(data);
}
})
//post验证
$('.sub').on('click',function(){
$.ajax({
url: 'http://localhost:4000/add.action',
type: 'post',
async: false,
data: {'content': 'hahah', 'sn': 12345},
success: function(data){
console.log(data);
},
error: function () {
alert('对不起,提交失败,请稍后重新提交');
}
})
})
8. 运行proxy.js创建服务,在浏览器中输入地址即可
当前目录下 命令行
node proxy.js
浏览器输入
http://localhost:4000/index.html
以上 即可解决接口跨域问题,提升自己的工作效率
9. 参考来源
- https://github.com/nodejitsu/node-http-proxy#using-https
- http://hao.jser.com/archive/10394/?utm_source=tuicool&utm_medium=referral