pom.xml引入坐标
<!-- google验证码 -->
<dependency>
<groupId>com.github.penggle</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
验证码配置文件 KaptchaConfig
@Configuration
public class KaptchaConfig {
@Bean(name = "captchaProducer")
public DefaultKaptcha getKaptchaBean()
{
DefaultKaptcha defaultKaptcha = new DefaultKaptcha();
Properties properties = new Properties();
properties.setProperty("kaptcha.border", "yes");
properties.setProperty("kaptcha.border.color", "105,179,90");
properties.setProperty("kaptcha.textproducer.font.color", "blue");
properties.setProperty("kaptcha.image.width", "160");
properties.setProperty("kaptcha.image.height", "60");
properties.setProperty("kaptcha.textproducer.font.size", "28");
properties.setProperty("kaptcha.session.key", "kaptchaCode");
properties.setProperty("kaptcha.textproducer.char.spac", "35");
properties.setProperty("kaptcha.textproducer.char.length", "5");
properties.setProperty("kaptcha.textproducer.font.names", "Arial,Courier");
properties.setProperty("kaptcha.noise.color", "white");
Config config = new Config(properties);
defaultKaptcha.setConfig(config);
return defaultKaptcha;
}
@Bean(name = "captchaProducerMath")
public DefaultKaptcha getKaptchaBeanMath()
{
DefaultKaptcha defaultKaptcha = new DefaultKaptcha();
Properties properties = new Properties();
properties.setProperty("kaptcha.border", "yes");
properties.setProperty("kaptcha.border.color", "105,179,90");
properties.setProperty("kaptcha.textproducer.font.color", "blue");
properties.setProperty("kaptcha.image.width", "100");
properties.setProperty("kaptcha.image.height", "30");
properties.setProperty("kaptcha.textproducer.font.size", "20");
properties.setProperty("kaptcha.session.key", "kaptchaCodeMath");
properties.setProperty("kaptcha.textproducer.impl", "com.mycourse.support.KaptchaTextCreator");
properties.setProperty("kaptcha.textproducer.char.spac", "5");
properties.setProperty("kaptcha.textproducer.char.length", "6");
properties.setProperty("kaptcha.textproducer.font.names", "Arial,Courier");
properties.setProperty("kaptcha.noise.color", "black");
properties.setProperty("kaptcha.noise.impl", "com.google.code.kaptcha.impl.NoNoise");
properties.setProperty("kaptcha.obscurificator.impl", "com.google.code.kaptcha.impl.ShadowGimpy");
Config config = new Config(properties);
defaultKaptcha.setConfig(config);
return defaultKaptcha;
}
}
验证码文本生成器 KaptchaTextCreator
public class KaptchaTextCreator extends DefaultTextCreator {
private static final String[] CNUMBERS = "0,1,2,3,4,5,6,7,8,9,10".split(",");
@Override
public String getText()
{
Integer result = 0;
Random random = new Random();
int x = random.nextInt(10);
int y = random.nextInt(10);
StringBuilder suChinese = new StringBuilder();
int randomoperands = (int) Math.round(Math.random() * 2);
if (randomoperands == 0)
{
result = x * y;
suChinese.append(CNUMBERS[x]);
suChinese.append("*");
suChinese.append(CNUMBERS[y]);
}
else if (randomoperands == 1)
{
if (!(x == 0) && y % x == 0)
{
result = y / x;
suChinese.append(CNUMBERS[y]);
suChinese.append("/");
suChinese.append(CNUMBERS[x]);
}
else
{
result = x + y;
suChinese.append(CNUMBERS[x]);
suChinese.append("+");
suChinese.append(CNUMBERS[y]);
}
}
else if (randomoperands == 2)
{
if (x >= y)
{
result = x - y;
suChinese.append(CNUMBERS[x]);
suChinese.append("-");
suChinese.append(CNUMBERS[y]);
}
else
{
result = y - x;
suChinese.append(CNUMBERS[y]);
suChinese.append("-");
suChinese.append(CNUMBERS[x]);
}
}
else
{
result = x + y;
suChinese.append(CNUMBERS[x]);
suChinese.append("+");
suChinese.append(CNUMBERS[y]);
}
suChinese.append("=@" + result);
return suChinese.toString();
}
}
验证码生成控制器 CaptchaController
@Controller
@RequestMapping("/captcha")
public class CaptchaController {
@Autowired
private Producer captchaProducer;
@Autowired
private Producer captchaProducerMath;
@GetMapping(value = "/captchaImage")
public ModelAndView getKaptchaImage(HttpServletRequest request, HttpServletResponse response)
{
ServletOutputStream out = null;
try
{
HttpSession session = request.getSession();
response.setDateHeader("Expires", 0);
response.setHeader("Cache-Control", "no-store, no-cache, must-revalidate");
response.addHeader("Cache-Control", "post-check=0, pre-check=0");
response.setHeader("Pragma", "no-cache");
response.setContentType("image/jpeg");
String type = request.getParameter("type");
String capStr = null;
String code = null;
BufferedImage bi = null;
if ("math".equals(type))
{
String capText = captchaProducerMath.createText();
capStr = capText.substring(0, capText.lastIndexOf("@"));
code = capText.substring(capText.lastIndexOf("@") + 1);
bi = captchaProducerMath.createImage(capStr);
}
else if ("char".equals(type))
{
capStr = code = captchaProducer.createText();
bi = captchaProducer.createImage(capStr);
}
session.setAttribute(Constants.KAPTCHA_SESSION_KEY, code);
out = response.getOutputStream();
ImageIO.write(bi, "jpg", out);
System.out.println("code = "+code);
System.out.println("bi = " + bi);
System.out.println("out =" + out);
out.flush();
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
try
{
if (out != null)
{
out.close();
}
}
catch (IOException e)
{
e.printStackTrace();
}
}
return null;
}
}
验证码校验Controller
@RestController
public class TestController {
@PostMapping("test")
public String kaptcha(Map<Object, String> map, HttpServletRequest request) {
HttpSession session = request.getSession();
String code = (String) session.getAttribute(Constants.KAPTCHA_SESSION_KEY);
String inputCode = request.getParameter("kaptcha");
if(!inputCode.equals(code)){
System.out.println("验证码输入错误");
map.put("msgKaptcha", "验证码错误");
return "fail";
}
System.out.println("验证码输入正确");
return "success";
}
Html (使用thymeleaf显示错误提示)
<form th:action="@{/test}" method="post">
<div class="kaptcha">
<input name="kaptcha" type="text" placeholder="验证码">
<img style="height: 45px;width: 110px;" id="codeImg" alt="点击更换" title="点击更换" src="captcha/captchaImage?type=math"/>
<p class="msgInput" th:if="${not #strings.isEmpty(msgKaptcha)}"
th:text="${msgKaptcha}">p>
div>
form>
点击更换验证码
$('#codeImg').click(function () {
var url = "captcha/captchaImage?type=math&s=" + Math.random();
$("#codeImg").attr("src", url);
});
实图效果
验证码具体属性可参考上面的配置类
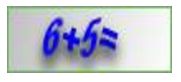