- 【问题解决】org.springframework.web.util.NestedServletException Handler dispatch failed;
m0_74825260
面试学习路线阿里巴巴前端
详细异常信息:org.springframework.web.util.NestedServletException:Handlerdispatchfailed;nestedexceptionisjava.lang.NoClassDefFoundError:javax/xml/bind/DatatypeConverteratorg.springframework.web.servlet.Dispa
- 字节跳动后端日常实习一二+hr面面经(已OC)
桜翊
字节跳动面试字节跳动
北航计算机大三,这学期打算找个实习,看了一圈大厂发现字节实习生给的薪资最高,福利也挺不错的,就通过内推投了字节的后端实习,很快就收到了面试邀约,从一面到收到offer一共6天,以下是本次面试的面经(部分题目可能记不太清了)一面:上来先是自我介绍,大概介绍了一下学校年级所学课程和做过的项目,然后开始提问面试官先是让我从做过的项目里挑一个来讲,我就挑了之前小学期的一个Spring项目“用户登录状态如何
- 基于springboot的鲜花销售商城网站
程序猿麦小七
毕业设计Java后台JavaWebspringbootjava后端
项目描述临近学期结束,还是毕业设计,你还在做java程序网络编程,期末作业,老师的作业要求觉得大了吗?不知道毕业设计该怎么办?网页功能的数量是否太多?没有合适的类型或系统?等等。这里根据疫情当下,你想解决的问题,今天给大家介绍一篇基于springboot的鲜花销售商城网站。功能需求本文设计并实现的商城系统,通过互联网来实现电子商城这一新兴产业,电子商城主要依靠于计算机互联网技术。如果缺少了这个技术
- 【Spring Cloud Alibaba】基于Spring Boot 3.x 搭建教程
m0_74824534
面试学习路线阿里巴巴springboot后端java
目录前言一、开发环境二、简介1.主要功能2.组件三、搭建过程1-主体工程搭建2-服务注册与发现组件——Nacos的安装3-服务注册与发现——服务提供者4-服务注册与发现——服务消费者5-服务配置中心6-OpenFeign服务接口调用7-OpenFeign高级特性8-SpringCloudGateway网关9-OpenFeign集成Sentinel实现服务降级前言本教程主要介绍如何基于SpringB
- springboot527基于Java企业项目管理系统(论文+源码)_kaic
开心毕设
数据库rabbitmqkafka
摘要如今社会上各行各业,都喜欢用自己行业的专属软件工作,互联网发展到这个时候,人们已经发现离不开了互联网。新技术的产生,往往能解决一些老技术的弊端问题。因为传统企业项目管理系统信息管理难度大,容错率低,管理人员处理数据费工费时,所以专门为解决这个难题开发了一个企业项目管理系统,可以解决许多问题。企业项目管理系统按照操作主体分为管理员和用户。管理员的功能包括操作日志管理、字典管理、论坛管理、公告管理
- 关于跨域和端口问题
Mayer999
Javanginx
写在文章之前nginx相信大家并不陌生,但nginx到底有什么用,和tomcat有什么区别,笔者最近遇到了这些问题,在此总结下。还有关于跨域和前后端交互不清楚的,相信会有所收获。环境:开发工具:IntellijIDEA2019.3jdk:1.8.0_181springboot:2.1.11springcloud:Finchley.RC1一、端口问题1.1nginx解决端口问题域名问题解决了,但是现
- 解决IDEA创建SpringBoot项目时不能选择java8的问题
奔跑吧小吕
intellij-ideajavaide
问题原因:当我创建一个springboot项目时,发现选不了java8了,查看官方文档之后,springboot不在支持java8了。解决方式:改成阿里云的服务
- JSR-107与SpringBoot缓存
weixin_39515823
SpringBootspring
文章目录JSR-107与SpringBoot缓存JSR-107JSR-107核心接口JSR-107图示Spring的缓存抽象缓存抽象定义重要接口Spring缓存使用重要概念&缓存注解缓存初体验@Cacheable注解的属性SpEL表达式@Cacheable的运行流程@CachePut&@CacheEvict&@CacheConfig@CachePut@CacheEvict@CacheConfig缓
- 入门SpringBoot-mybatis
``Lotus。
SpringBootMyBatismybatisspringbootjava
通上次学的SpringBoot来整合一、使用注解版的Mybatis整合SpringBoot1、实体类(entity)packagecom.cxy.entity;importlombok.Data;@DatapublicclassUser{privateLongid;privateStringname;privateIntegerage;privateStringemail;}2、mapperpac
- 【Spring】配置文件的使用
m0_74823507
面试学习路线阿里巴巴springjava后端
在Spring框架中,application.properties(或application.yml)文件用于配置Spring应用程序的各种属性。我们可以通过多种方式来使用这些配置,包括使用@Value和@ConfigurationProperties注解来绑定配置到Java对象。下面是对不同配置类型的说明,以及如何在代码中使用它们的示例。1.配置变量(单个属性)可以在application.pr
- Spring 事务
zhujilisa
Springspring
Spring事务使用Spring事务Spring事务传播使用Spring事务引入依赖org.springframeworkspring-tx5.3.20开启Spring事务注解@EnableTransactionManagement@Transactional该注解实际是导入了两个bean:1.AutoProxyRegistrar.class:通过beanDefinition向Spring容器注册
- Spring容器扩展点
zhujilisa
Springspring
Spring容器扩展点BeanDefinitionRegistryPostProcessorBeanFactoryPostProcessorImportSelectorImportBeanDefinitionRegistorBeanPostProcessorInstantiationAwareBeanPostProcessor--postProcessBeforeInstantiationSmar
- ElasticSearch基础入门(六)使用Spring Data ElasticSearch添加、修改、删除数据
全端工程师
elasticsearchelasticsearch
ElasticSearch基础入门(六)使用SpringDataElasticSearch添加、修改、删除文档一、概述二、新增文档1.新增一条2.批量新增三、修改文档四、删除文档一、概述SpringData的强大之处,就在于你不用写任何DAO处理,自动根据方法名或类的信息进行CRUD操作。只要你定义一个接口,然后继承Repository提供的一些子接口,就能具备各种基本的CRUD功能。我们想要操作
- 【Spring MVC】基本原理和工作流程
cangloe
javaspringmvcjava
SpringMVC是SpringFramework提供的一个基于Model-View-Controller(MVC)模式的Web框架。它用于构建灵活且可扩展的Web应用程序。SpringMVC将应用程序的业务逻辑、用户界面和导航逻辑分开,从而简化开发过程,提高代码的可维护性和可测试性。以下是对SpringMVC的基本原理和工作流程的详细讲解。SpringMVC基本原理1.MVC架构Model(模型
- 深入解析Spring核心扩展点:BeanFactoryPostProcessor与BeanDefinitionRegistryPostProcessor
冬天vs不冷
springspringoraclejava
目录一、引言二、核心概念与区别1、BeanFactoryPostProcessor2、BeanDefinitionRegistryPostProcessor3、核心区别三、执行时机与流程四、典型应用场景1、BeanFactoryPostProcessor的使用场景2、BeanDefinitionRegistryPostProcessor的使用场景五、实现与注册方式1、实现自定义处理器2、注册到Sp
- SpringCloud微服务详解:java项目业绩怎么写
m0_56712078
程序员java后端面试
前言原来,一瞬间,一句话,真的可以改变一个人的命运。说一个前几年一个热门话题:“是否应该跳出舒适圈。”一时间,这个话题便引发众人议论:支持方:愿意挑战不擅长领域的人,勇气可嘉,值得学习。反对派:做自己擅长的事情不好吗?为何非要跳出舒适圈呢?其实,每个人的决定都取决于当下自己的状态以及那一瞬间的冲动,也可能你成了雷军,也可能你和下图一样,大家懂我意思吧一念之间的决定,你敢尝试吗?今天想说的是对于想转
- Spring MVC的工作流程
ItKevin爱java
javawebjavajavaSpringMVC
SpringMVC的工作流程是一个典型的MVC(Model-View-Controller)架构在Web开发中的应用。下面将详细描述SpringMVC的工作流程:一、用户发起请求用户请求:用户通过浏览器向服务器发送HTTP请求,这个请求首先被SpringMVC的前端控制器(DispatcherServlet)拦截。二、DispatcherServlet处理请求请求转发:DispatcherServ
- Spring MVC流程
zhujilisa
Springspring
SpringMVC启动流程启动流程父子容器请求处理MultipartFile解析参数传递返回值处理HandlerInterceptor启动流程启动Tomcat解析web.xml创建DispatcherServlet调用DIspatcherServlet的init方法4.1创建Spring容器4.2发布ContextRefresheEvent4.3在OnRefreshed方法中触发initStrat
- JAVA【微服务】Spring AI 使用详解
C_V_Better
javaAI人工智能人工智能java微服务后端数据结构开发语言
目录一、前言二、SpringAI概述2.1什么是SpringAI2.2SpringAI特点2.3SpringAI带来的便利2.4SpringAI应用领域2.4.1聊天模型2.4.2文本到图像模型2.4.3音频转文本2.4.4嵌入大模型使用2.4.5矢量数据库支持2.4.6数据工程ETL框架三、SpringAI对接ChatGPT3.1前置准备3.2添加必要的依赖3.3接入操作流程3.3.1配置文件3
- 关于idea中新建springboot项目Java版本不能选择11和8的解决办法
aniceperson999
intellij-ideajavaide
原因:spring2.X版本在2023年11月24日停止维护了,因此创建spring项目时不再有2.X版本的选项,只能从3.1.X版本开始选择而Spring3.X版本不支持JDK8,JDK11,最低支持JDK17,因此JDK11也无法选择了当然,停止维护只代表我们无法用idea主动创建spring2.X版本的项目了,不代表我们无法使用,该使用依然能使用,丝毫不受影响目前阿里云还是支持创建Sprin
- 使用Idea创建springboot项目
奔跑吧邓邓子
SpringBoot深入浅出常见问题解答(FAQ)高效运维javaidea
提示:“奔跑吧邓邓子”的高效运维专栏聚焦于各类运维场景中的实际操作与问题解决。内容涵盖服务器硬件(如IBMSystem3650M5)、云服务平台(如腾讯云、华为云)、服务器软件(如Nginx、Apache、GitLab、Redis、Elasticsearch、Kubernetes、Docker等)、开发工具(如Git、HBuilder)以及网络安全(如挖矿病毒排查、SSL证书配置)等多个方面。无论
- 用idea创建低版本springboot2.X的项目
詹皇wm
问题总结intellij-ideajava
文章目录我的环境:创建项目:进入创建项目页面:进入Springboot版本选择和添加依赖页面进入项目打开pom.xml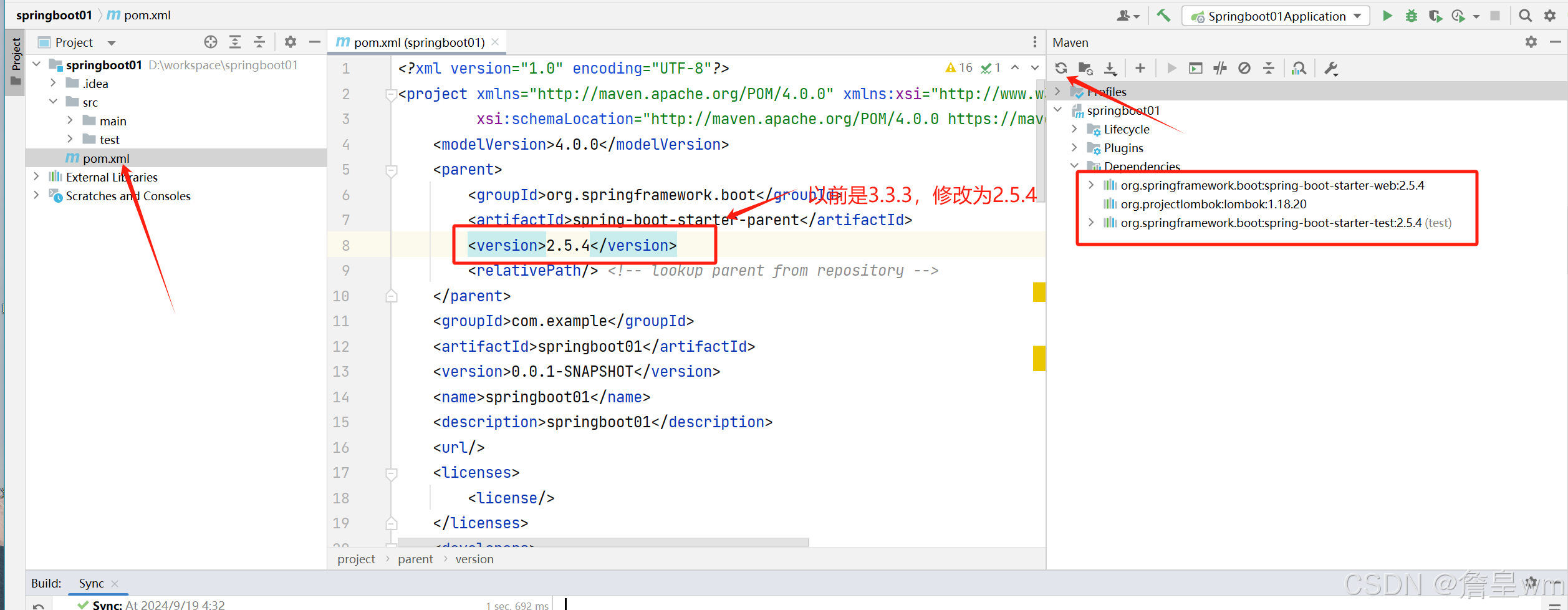*补充*_本文章主要是对于现在idea配置大部分都是自带SpringBoot3.X,怎么创建低版本springboo
- Springboot 整合 Java DL4J 实现企业门禁人脸识别系统
伏羲栈
人工智能深度学习JavaDL4J-深度学习实战springbootjavaDeeplearning4jdeeplearning人工智能深度学习spring
博主简介:历代文学网(PC端可以访问:https://literature.sinhy.com/#/literature?__c=1000,移动端可微信小程序搜索“历代文学”)总架构师,15年工作经验,精通Java编程,高并发设计,Springboot和微服务,熟悉Linux,ESXI虚拟化以及云原生Docker和K8s,热衷于探索科技的边界,并将理论知识转化为实际应用。保持对新技术的好奇心,乐于
- 计算机毕业设计 ——jspssm507Springboot 的论坛管理系统
奔强的程序
课程设计
博主小档案:花花,一名来自世界500强的资深程序猿,毕业于国内知名985高校。技术专长:花花在深度学习任务中展现出卓越的能力,包括但不限于java、python等技术。近年来,花花更是将触角延伸至AI领域,对于机器学习、自然语言处理、智能推荐等前沿技术都有独到的见解和实践经验。服务内容:1、提供科研入门辅导(主要是代码方面)2、代码部署3、定制化需求解决等4、期末考试复习计算机毕业设计——jsps
- 高性能PHP框架webman爬虫引擎插件,如何爬取数据
Ai 编码
php教程php爬虫开发语言
文章精选推荐1JetBrainsAiassistant编程工具让你的工作效率翻倍2ExtraIcons:JetBrainsIDE的图标增强神器3IDEA插件推荐-SequenceDiagram,自动生成时序图4BashSupportPro这个ides插件主要是用来干嘛的?5IDEA必装的插件:SpringBootHelper的使用与功能特点6Aiassistant,又是一个写代码神器7Cursor
- Spring中如何优雅的使用策略模式
齐 飞
Spring策略模式springjava
文章目录一、策略模式是什么?定义结构二、策略模式的优缺点与使用场景优缺点使用场景三、在Spring中使用策略模式1.抽象策略类2.具体策略类3.环境类4.枚举5.服务类使用策略模式前先回顾一下策略模式是什么,只想看在spring中如何使用,可直接跳转到第三章。一、策略模式是什么?定义策略模式定义了一系列算法,并将每个算法封装起来,使它们可以相互替换,且算法的变化不会影响使用算法的客户(满足开闭原则
- 策略模式在业务中的实际应用
落叶s178
游戏开发策略模式bash开发语言
策略模式1、策略模式结构图策略模式主要由以上三个身份组成,这里就不过多介绍策略模式的基础知识,默认大家已经对策略模式已经有了一个基础的认识。2、业务需求现有一个广告点击数据埋点上报的需求,上报的埋点数据根据点击的广告位置不同做区分进行上报,每个广告位置的数据进行分表存储。(eg:这里大家也不必深究分表存储为什么要这么做,只聊策略模式的实际应用)3、代码实现由于是实战案例,基于SpringBoot框
- Spring Boot整合FTP实现文件的上传和下载
anhao78
ftp实现文件的上传和下载
第一步:pom.xml文件中引入依赖包 commons-net commons-net 3.3第二步:编写ftp上传文件工具类packagecom.split.ftp;importlombok.Data;importlombok.extern.slf4j.Slf4j;importorg.apache.commons.net.ftp.*;importorg.springframework
- Invalid bound statement, No converter found for return value of type:
噢!不杰克
springboot
SpringBoot2.0学习的Bug(Invalidboundstatement,Noconverterfoundforreturnvalueoftype:)一、Invalidboundstatement:绑定语句无效,这个问题很复杂,每一个人的解决方式不同,我的是mapper-locations中映射路径问题,我看着没问题,但还是重新copy路径试了一下。还真是这个问题。mybatis:con
- 在 Spring Boot 中使用异步线程时的 HttpServletRequest 复用问题
老友@
后端springbootjava后端requestTomcat异步线程多线程
在SpringBoot中使用异步线程时的HttpServletRequest复用问题一、问题描述:异步线程操作导致请求复用时`Cookie`解析失败1.场景背景2.问题根源二、问题详细分析1.场景重现2.问题分析三、解决方案四、总结一、问题描述:异步线程操作导致请求复用时Cookie解析失败1.场景背景在一个Web应用中,通常每个请求都会有一个HttpServletRequest对象来保存该请求的
- JAVA中的Enum
周凡杨
javaenum枚举
Enum是计算机编程语言中的一种数据类型---枚举类型。 在实际问题中,有些变量的取值被限定在一个有限的范围内。 例如,一个星期内只有七天 我们通常这样实现上面的定义:
public String monday;
public String tuesday;
public String wensday;
public String thursday
- 赶集网mysql开发36条军规
Bill_chen
mysql业务架构设计mysql调优mysql性能优化
(一)核心军规 (1)不在数据库做运算 cpu计算务必移至业务层; (2)控制单表数据量 int型不超过1000w,含char则不超过500w; 合理分表; 限制单库表数量在300以内; (3)控制列数量 字段少而精,字段数建议在20以内
- Shell test命令
daizj
shell字符串test数字文件比较
Shell test命令
Shell中的 test 命令用于检查某个条件是否成立,它可以进行数值、字符和文件三个方面的测试。 数值测试 参数 说明 -eq 等于则为真 -ne 不等于则为真 -gt 大于则为真 -ge 大于等于则为真 -lt 小于则为真 -le 小于等于则为真
实例演示:
num1=100
num2=100if test $[num1]
- XFire框架实现WebService(二)
周凡杨
javawebservice
有了XFire框架实现WebService(一),就可以继续开发WebService的简单应用。
Webservice的服务端(WEB工程):
两个java bean类:
Course.java
package cn.com.bean;
public class Course {
private
- 重绘之画图板
朱辉辉33
画图板
上次博客讲的五子棋重绘比较简单,因为只要在重写系统重绘方法paint()时加入棋盘和棋子的绘制。这次我想说说画图板的重绘。
画图板重绘难在需要重绘的类型很多,比如说里面有矩形,园,直线之类的,所以我们要想办法将里面的图形加入一个队列中,这样在重绘时就
- Java的IO流
西蜀石兰
java
刚学Java的IO流时,被各种inputStream流弄的很迷糊,看老罗视频时说想象成插在文件上的一根管道,当初听时觉得自己很明白,可到自己用时,有不知道怎么代码了。。。
每当遇到这种问题时,我习惯性的从头开始理逻辑,会问自己一些很简单的问题,把这些简单的问题想明白了,再看代码时才不会迷糊。
IO流作用是什么?
答:实现对文件的读写,这里的文件是广义的;
Java如何实现程序到文件
- No matching PlatformTransactionManager bean found for qualifier 'add' - neither
林鹤霄
java.lang.IllegalStateException: No matching PlatformTransactionManager bean found for qualifier 'add' - neither qualifier match nor bean name match!
网上找了好多的资料没能解决,后来发现:项目中使用的是xml配置的方式配置事务,但是
- Row size too large (> 8126). Changing some columns to TEXT or BLOB
aigo
column
原文:http://stackoverflow.com/questions/15585602/change-limit-for-mysql-row-size-too-large
异常信息:
Row size too large (> 8126). Changing some columns to TEXT or BLOB or using ROW_FORMAT=DYNAM
- JS 格式化时间
alxw4616
JavaScript
/**
* 格式化时间 2013/6/13 by 半仙
[email protected]
* 需要 pad 函数
* 接收可用的时间值.
* 返回替换时间占位符后的字符串
*
* 时间占位符:年 Y 月 M 日 D 小时 h 分 m 秒 s 重复次数表示占位数
* 如 YYYY 4占4位 YY 占2位<p></p>
* MM DD hh mm
- 队列中数据的移除问题
百合不是茶
队列移除
队列的移除一般都是使用的remov();都可以移除的,但是在昨天做线程移除的时候出现了点问题,没有将遍历出来的全部移除, 代码如下;
//
package com.Thread0715.com;
import java.util.ArrayList;
public class Threa
- Runnable接口使用实例
bijian1013
javathreadRunnablejava多线程
Runnable接口
a. 该接口只有一个方法:public void run();
b. 实现该接口的类必须覆盖该run方法
c. 实现了Runnable接口的类并不具有任何天
- oracle里的extend详解
bijian1013
oracle数据库extend
扩展已知的数组空间,例:
DECLARE
TYPE CourseList IS TABLE OF VARCHAR2(10);
courses CourseList;
BEGIN
-- 初始化数组元素,大小为3
courses := CourseList('Biol 4412 ', 'Psyc 3112 ', 'Anth 3001 ');
--
- 【httpclient】httpclient发送表单POST请求
bit1129
httpclient
浏览器Form Post请求
浏览器可以通过提交表单的方式向服务器发起POST请求,这种形式的POST请求不同于一般的POST请求
1. 一般的POST请求,将请求数据放置于请求体中,服务器端以二进制流的方式读取数据,HttpServletRequest.getInputStream()。这种方式的请求可以处理任意数据形式的POST请求,比如请求数据是字符串或者是二进制数据
2. Form
- 【Hive十三】Hive读写Avro格式的数据
bit1129
hive
1. 原始数据
hive> select * from word;
OK
1 MSN
10 QQ
100 Gtalk
1000 Skype
2. 创建avro格式的数据表
hive> CREATE TABLE avro_table(age INT, name STRING)STORE
- nginx+lua+redis自动识别封解禁频繁访问IP
ronin47
在站点遇到攻击且无明显攻击特征,造成站点访问慢,nginx不断返回502等错误时,可利用nginx+lua+redis实现在指定的时间段 内,若单IP的请求量达到指定的数量后对该IP进行封禁,nginx返回403禁止访问。利用redis的expire命令设置封禁IP的过期时间达到在 指定的封禁时间后实行自动解封的目的。
一、安装环境:
CentOS x64 release 6.4(Fin
- java-二叉树的遍历-先序、中序、后序(递归和非递归)、层次遍历
bylijinnan
java
import java.util.LinkedList;
import java.util.List;
import java.util.Stack;
public class BinTreeTraverse {
//private int[] array={ 1, 2, 3, 4, 5, 6, 7, 8, 9 };
private int[] array={ 10,6,
- Spring源码学习-XML 配置方式的IoC容器启动过程分析
bylijinnan
javaspringIOC
以FileSystemXmlApplicationContext为例,把Spring IoC容器的初始化流程走一遍:
ApplicationContext context = new FileSystemXmlApplicationContext
("C:/Users/ZARA/workspace/HelloSpring/src/Beans.xml&q
- [科研与项目]民营企业请慎重参与军事科技工程
comsci
企业
军事科研工程和项目 并非要用最先进,最时髦的技术,而是要做到“万无一失”
而民营科技企业在搞科技创新工程的时候,往往考虑的是技术的先进性,而对先进技术带来的风险考虑得不够,在今天提倡军民融合发展的大环境下,这种“万无一失”和“时髦性”的矛盾会日益凸显。。。。。。所以请大家在参与任何重大的军事和政府项目之前,对
- spring 定时器-两种方式
cuityang
springquartz定时器
方式一:
间隔一定时间 运行
<bean id="updateSessionIdTask" class="com.yang.iprms.common.UpdateSessionTask" autowire="byName" />
<bean id="updateSessionIdSchedule
- 简述一下关于BroadView站点的相关设计
damoqiongqiu
view
终于弄上线了,累趴,戳这里http://www.broadview.com.cn
简述一下相关的技术点
前端:jQuery+BootStrap3.2+HandleBars,全站Ajax(貌似对SEO的影响很大啊!怎么破?),用Grunt对全部JS做了压缩处理,对部分JS和CSS做了合并(模块间存在很多依赖,全部合并比较繁琐,待完善)。
后端:U
- 运维 PHP问题汇总
dcj3sjt126com
windows2003
1、Dede(织梦)发表文章时,内容自动添加关键字显示空白页
解决方法:
后台>系统>系统基本参数>核心设置>关键字替换(是/否),这里选择“是”。
后台>系统>系统基本参数>其他选项>自动提取关键字,这里选择“是”。
2、解决PHP168超级管理员上传图片提示你的空间不足
网站是用PHP168做的,反映使用管理员在后台无法
- mac 下 安装php扩展 - mcrypt
dcj3sjt126com
PHP
MCrypt是一个功能强大的加密算法扩展库,它包括有22种算法,phpMyAdmin依赖这个PHP扩展,具体如下:
下载并解压libmcrypt-2.5.8.tar.gz。
在终端执行如下命令: tar zxvf libmcrypt-2.5.8.tar.gz cd libmcrypt-2.5.8/ ./configure --disable-posix-threads --
- MongoDB更新文档 [四]
eksliang
mongodbMongodb更新文档
MongoDB更新文档
转载请出自出处:http://eksliang.iteye.com/blog/2174104
MongoDB对文档的CURD,前面的博客简单介绍了,但是对文档更新篇幅比较大,所以这里单独拿出来。
语法结构如下:
db.collection.update( criteria, objNew, upsert, multi)
参数含义 参数  
- Linux下的解压,移除,复制,查看tomcat命令
y806839048
tomcat
重复myeclipse生成webservice有问题删除以前的,干净
1、先切换到:cd usr/local/tomcat5/logs
2、tail -f catalina.out
3、这样运行时就可以实时查看运行日志了
Ctrl+c 是退出tail命令。
有问题不明的先注掉
cp /opt/tomcat-6.0.44/webapps/g
- Spring之使用事务缘由(3-XML实现)
ihuning
spring
用事务通知声明式地管理事务
事务管理是一种横切关注点。为了在 Spring 2.x 中启用声明式事务管理,可以通过 tx Schema 中定义的 <tx:advice> 元素声明事务通知,为此必须事先将这个 Schema 定义添加到 <beans> 根元素中去。声明了事务通知后,就需要将它与切入点关联起来。由于事务通知是在 <aop:
- GCD使用经验与技巧浅谈
啸笑天
GC
前言
GCD(Grand Central Dispatch)可以说是Mac、iOS开发中的一大“利器”,本文就总结一些有关使用GCD的经验与技巧。
dispatch_once_t必须是全局或static变量
这一条算是“老生常谈”了,但我认为还是有必要强调一次,毕竟非全局或非static的dispatch_once_t变量在使用时会导致非常不好排查的bug,正确的如下: 1
- linux(Ubuntu)下常用命令备忘录1
macroli
linux工作ubuntu
在使用下面的命令是可以通过--help来获取更多的信息1,查询当前目录文件列表:ls
ls命令默认状态下将按首字母升序列出你当前文件夹下面的所有内容,但这样直接运行所得到的信息也是比较少的,通常它可以结合以下这些参数运行以查询更多的信息:
ls / 显示/.下的所有文件和目录
ls -l 给出文件或者文件夹的详细信息
ls -a 显示所有文件,包括隐藏文
- nodejs同步操作mysql
qiaolevip
学习永无止境每天进步一点点mysqlnodejs
// db-util.js
var mysql = require('mysql');
var pool = mysql.createPool({
connectionLimit : 10,
host: 'localhost',
user: 'root',
password: '',
database: 'test',
port: 3306
});
- 一起学Hive系列文章
superlxw1234
hiveHive入门
[一起学Hive]系列文章 目录贴,入门Hive,持续更新中。
[一起学Hive]之一—Hive概述,Hive是什么
[一起学Hive]之二—Hive函数大全-完整版
[一起学Hive]之三—Hive中的数据库(Database)和表(Table)
[一起学Hive]之四-Hive的安装配置
[一起学Hive]之五-Hive的视图和分区
[一起学Hive
- Spring开发利器:Spring Tool Suite 3.7.0 发布
wiselyman
spring
Spring Tool Suite(简称STS)是基于Eclipse,专门针对Spring开发者提供大量的便捷功能的优秀开发工具。
在3.7.0版本主要做了如下的更新:
将eclipse版本更新至Eclipse Mars 4.5 GA
Spring Boot(JavaEE开发的颠覆者集大成者,推荐大家学习)的配置语言YAML编辑器的支持(包含自动提示,