树状结构工具
创建类TreeUtil
类属性
private List rootList; //根节点对象存放到这里
private List bodyList; //其他节点存放到这里,可以包含根节点
private Function treeId; //获取子级id接口
private Function treeRootId;//获取上级id接口
private BiConsumer treeSetList;//用于setList的接口
两个构造方法
/**
* 传入根节点与非根节点,实现三个函数接口
* @param rootList 根节点
* @param bodyList 非根节点
* @param treeId 函数->获取id
* @param treeRootId ->获取根id
* @param treeSetList ->保存集合方法
*/
public TreeUtil(List rootList, List bodyList, Function treeId, Function treeRootId, BiConsumer treeSetList) {
this.rootList = rootList;
this.bodyList = bodyList;
this.treeId = treeId;
this.treeRootId = treeRootId;
this.treeSetList = treeSetList;
}
/**
*传入集合,实现三个函数接口
* @param salesAreaTrees 集合
* @param treeId 函数->获取id
* @param treeRootId ->获取根id
* @param treeSetList ->保存集合方法
*/
public TreeUtil(List salesAreaTrees, Function treeId, Function treeRootId, BiConsumer treeSetList) {
this.treeId = treeId;
this.treeRootId = treeRootId;
this.treeSetList = treeSetList;
//调用内部方法分离出根节点与非根节点
this.getRootList(salesAreaTrees);
}
分离方法
/**
* 得到RootList和BodyList
*
* @param list
*/
private void getRootList(List list) {
rootList = new ArrayList<>();
bodyList = new ArrayList<>();
List
递归
public List getTree() { //调用的方法入口
if (bodyList != null && !bodyList.isEmpty()) {
//声明一个map,用来过滤已操作过的数据
Map map = Maps.newHashMapWithExpectedSize(bodyList.size());
rootList.forEach(beanTree -> getChild(beanTree, map));
return rootList;
}
return rootList;
}
private void getChild(T t, Map map) {
List childList = new ArrayList<>();
bodyList.stream()
.filter(c -> !map.containsKey(treeId.apply(c)))
.filter(c -> Objects.equals(this.treeRootId.apply(c),this.treeId.apply(t)))
.forEach(c -> {
map.put(this.treeId.apply(c), this.treeRootId.apply(c));
getChild(c, map);
childList.add(c);
});
this.treeSetList.accept(t, childList);
}
到此此工具类构建完毕.
使用示例:
构建函数
List list=service.getList;
Function function=Division::getId;
Function function2=Division::getParentId;
BiConsumer> treeSetList=Division::setChildList;
构建工具类
TreeUtil treeUtil= new TreeUtil<>(list,function,function2,treeSetList);
调用方法获得树状结构
treeUtil.getTree();
工具类完整代码
import com.google.common.collect.Maps;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.stream.Collectors;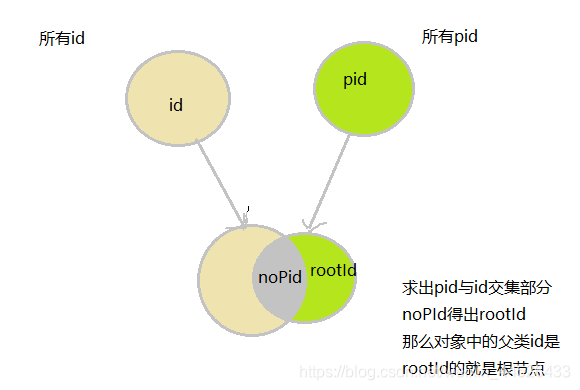
/**
* @author 72060500
* @version 1.0.1
* 树状工具类
*/
public class TreeUtil {
private List rootList; //根节点对象存放到这里
private List bodyList; //其他节点存放到这里,可以包含根节点
private Function treeId; //获取子级id接口
private Function treeRootId;//获取上级id接口
private BiConsumer treeSetList;//用于setList的接口
/**
* 传入根节点与非根节点,实现三个函数接口
* @param rootList 根节点
* @param bodyList 非根节点
* @param treeId 函数->获取id
* @param treeRootId ->获取根id
* @param treeSetList ->保存集合方法
*/
public TreeUtil(List rootList, List bodyList, Function treeId, Function treeRootId, BiConsumer treeSetList) {
this.rootList = rootList;
this.bodyList = bodyList;
this.treeId = treeId;
this.treeRootId = treeRootId;
this.treeSetList = treeSetList;
}
/**
*传入集合,实现三个函数接口
* @param salesAreaTrees 集合
* @param treeId 函数->获取id
* @param treeRootId ->获取根id
* @param treeSetList ->保存集合方法
*/
public TreeUtil(List salesAreaTrees, Function treeId, Function treeRootId, BiConsumer treeSetList) {
this.treeId = treeId;
this.treeRootId = treeRootId;
this.treeSetList = treeSetList;
//调用内部方法分离出根节点与非根节点
this.getRootList(salesAreaTrees);
}
public List getTree() { //调用的方法入口
if (bodyList != null && !bodyList.isEmpty()) {
//声明一个map,用来过滤已操作过的数据
Map map = Maps.newHashMapWithExpectedSize(bodyList.size());
rootList.forEach(beanTree -> getChild(beanTree, map));
return rootList;
}
return rootList;
}
private void getChild(T t, Map map) {
List childList = new ArrayList<>();
bodyList.stream()
.filter(c -> !map.containsKey(treeId.apply(c)))
.filter(c -> Objects.equals(this.treeRootId.apply(c),this.treeId.apply(t)))
.forEach(c -> {
map.put(this.treeId.apply(c), this.treeRootId.apply(c));
getChild(c, map);
childList.add(c);
});
this.treeSetList.accept(t, childList);
}
/**
* 得到RootList和BodyList
*
* @param list
*/
private void getRootList(List list) {
rootList = new ArrayList<>();
bodyList = new ArrayList<>();
List ids = list.stream()
.parallel()
.map(p -> this.treeId.apply(p))
.collect(Collectors.toList());
List pids = list.stream()
.parallel()
.map(p -> this.treeRootId.apply(p))
.collect(Collectors.toList());
//(求id和pid的交集->用于推导非根节点 )
List notRootPids = ids.stream()
.parallel()
.filter(id -> pids.contains(id))
.collect(Collectors.toList());
//非根节点
rootList=list.stream()
.parallel()
.filter(item -> !notRootPids.contains(this.treeRootId.apply(item)))
.collect(Collectors.toList());
//通过非根节点->根节点
bodyList = list.stream()
.parallel()
.filter(item -> !rootList.contains(item))
.collect(Collectors.toList());
}
}