import numpy as np
import numpy.matlib
import matplotlib.pyplot as plt
N = 4
M = 1
distMeasurementErrRatio = 0.1
networkSize = 5
anchorLoc = np.matlib.array(([0, 0],
[networkSize, 0],
[0, networkSize],
[networkSize, networkSize]
))
mobileLoc = networkSize * np.matlib.rand(M, 2)
distance = np.matlib.zeros((N, M))
for m in range(0, M):
for n in range(0, N):
distance[n, m] = np.sqrt((anchorLoc[n, 0] - mobileLoc[m, 0]) ** 2 + (anchorLoc[n, 1] - mobileLoc[m, 1]) ** 2)
distanceNoisy = distance + np.multiply(distance, distMeasurementErrRatio * (np.matlib.rand(N, M) - 1 / 2))
numOfIteration = 15
mobileLocEst = networkSize * np.matlib.rand(M, 2)
for m in range(0, M):
for i in range(0, numOfIteration):
a = anchorLoc - np.matlib.repmat(mobileLocEst[m, :], N, 1)
distanceEst = np.matlib.sqrt(np.matlib.sum(np.multiply(a, a), 1))
distanceDrv = np.concatenate([np.true_divide((mobileLocEst[m, 0] - anchorLoc[:, 0].reshape(4, 1)), distanceEst),
np.true_divide((mobileLocEst[m, 1] - anchorLoc[:, 1].reshape(4, 1)),
distanceEst)], 1)
e = - (distanceDrv.T * distanceDrv).I * distanceDrv.T
delta = e * (distanceEst - distanceNoisy[:, m])
mobileLocEst[m, :] = mobileLocEst[m, :] + delta.T
Err = np.mean(np.sqrt(np.sum(np.multiply((mobileLocEst - mobileLoc), (mobileLocEst - mobileLoc)))))
plt.plot(mobileLocEst[:, 0], mobileLocEst[:, 1], 'o', label="MobileLocEst")
plt.plot(anchorLoc[:, 0], anchorLoc[:, 1], 'o', label="AnchorLocation")
plt.plot(mobileLoc[:, 0], mobileLoc[:, 1], 'o', label="MobileLocation")
plt.xlabel("x")
plt.ylabel("y")
plt.legend(loc='best')
plt.title("Err: "+str(Err)+" m")
plt.show()
if __name__ == '__main__':
pass
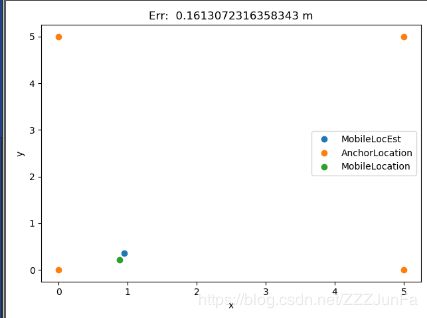