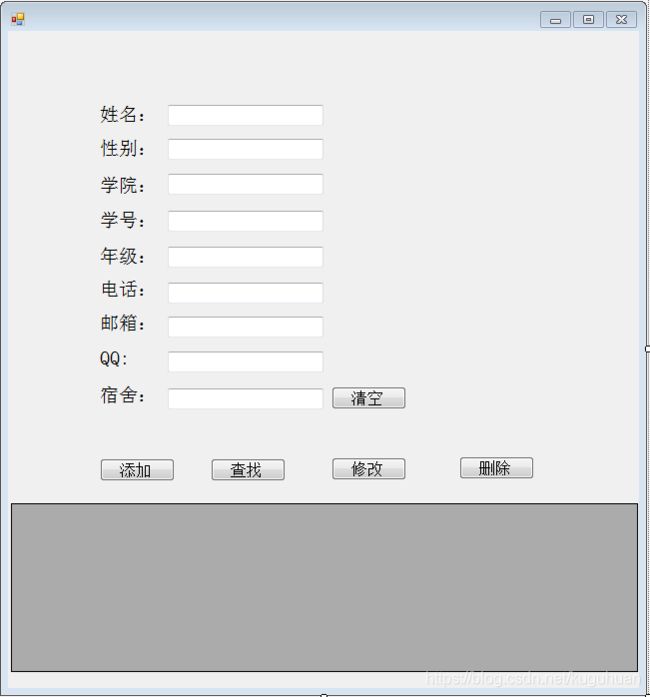
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace text3_CRUD
{
public partial class Form1 : Form
{
private string str = "data source=本地IP;initial catalog=数据库名;user ID=用户名;pwd=密码";
public Form1()
{
InitializeComponent();
}
private void TextBox5_TextChanged(object sender, EventArgs e)
{
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void Label10_Click(object sender, EventArgs e)
{
}
private void ButAdd_Click(object sender, EventArgs e)
{
string name = txtname.Text;
string sex = txtsex.Text;
string college = txtcollege.Text;
string id = txtid.Text;
string grade = txtgrade.Text;
string phone = txtphone.Text;
string email = txtemail.Text;
string qq = txtqq.Text;
string room = txtroom.Text;
using (var conn = new SqlConnection(this.str))
{
conn.Open();
Form1 f = new Form1();
if (f.Existence(id, conn))
{
SqlParameter[] para = new SqlParameter[]
{
new SqlParameter("@name",name),
new SqlParameter("@sex", sex),
new SqlParameter("@college", college),
new SqlParameter("@id", id),
new SqlParameter("@grade", grade),
new SqlParameter("@phone", phone),
new SqlParameter("@email", email),
new SqlParameter("@qq", qq),
new SqlParameter("@room", room),
};
string sql = "insert into Students values(@name, @sex, @college, @id, @grade, @phone, @email, @qq, @room);";
SqlCommand com = new SqlCommand(sql, conn);
com.Parameters.AddRange(para);
int result = (int)com.ExecuteNonQuery();
if (result > 0)
{
MessageBox.Show("添加成功!");
this.Form1_Load_1(null, null);
}
else
{
MessageBox.Show("添加失败!");
}
}
else
{
MessageBox.Show("数据已经存在!");
}
conn.Close();
}
}
public bool Existence(string id, SqlConnection conn)
{
string txtStr = string.Format( "select id from Students where id = '{0}' " ,id);
SqlDataAdapter sda = new SqlDataAdapter(txtStr, conn);
DataSet ds = new DataSet();
sda.Fill(ds);
DataTable dt = ds.Tables[0];
if(dt.Rows.Count > 0)
{
return false;
}
else
{
return true;
}
}
private void BtnSelect_Click(object sender, EventArgs e)
{
string name = txtname.Text;
string sex = txtsex.Text;
string college = txtcollege.Text;
string id = txtid.Text;
string grade = txtgrade.Text;
string phone = txtphone.Text;
string email = txtemail.Text;
string qq = txtqq.Text;
string room = txtroom.Text;
using(var conn = new SqlConnection(this.str))
{
conn.Open();
StringBuilder sb = new StringBuilder();
sb.Append("select name, sex, college, id, grade,phone, email, qq, room from Students where 1=1");
if (name != "")
{
sb.AppendFormat(" and name = '{0}'", name);
}
if (sex != "")
{
sb.AppendFormat(" and sex = '{0}'", sex);
}
if (college != "")
{
sb.AppendFormat(" and college = '{0}'", college);
}
if (id != "")
{
sb.AppendFormat(" and id = '{0}'", id);
}
if (grade != "")
{
sb.AppendFormat(" and grade = '{0}'", grade);
}
if (phone != "")
{
sb.AppendFormat(" and phone = '{0}'", phone);
}
if (email != "")
{
sb.AppendFormat(" and email = '{0}'", email);
}
if (qq != "")
{
sb.AppendFormat(" and qq = '{0}'", qq);
}
if (room != "")
{
sb.AppendFormat(" and room = '{0}'", room);
}
string sql = sb.ToString();
SqlDataAdapter adapter = new SqlDataAdapter(sql, conn);
DataSet ds = new DataSet();
adapter.Fill(ds);
dataGridView1.DataSource = ds.Tables[0];
conn.Close();
}
}
private void BtnUpdate_Click(object sender, EventArgs e)
{
string name = txtname.Text;
string sex = txtsex.Text;
string college = txtcollege.Text;
string id = txtid.Text;
string grade = txtgrade.Text;
string phone = txtphone.Text;
string email = txtemail.Text;
string qq = txtqq.Text;
string room = txtroom.Text;
SqlParameter[] para = new SqlParameter[]
{
new SqlParameter("@name",name),
new SqlParameter("@sex", sex),
new SqlParameter("@college", college),
new SqlParameter("@id", id),
new SqlParameter("@grade", grade),
new SqlParameter("@phone", phone),
new SqlParameter("@email", email),
new SqlParameter("@qq", qq),
new SqlParameter("@room", room)
};
using(var conn = new SqlConnection(this.str))
{
conn.Open();
string sql = "update Students set name = @name, sex = @sex, college = @college, id = @id, grade = @grade, phone = @phone, email = @email, qq = @qq, room = @room where id = @id";
SqlCommand com = new SqlCommand(sql, conn);
com.Parameters.AddRange(para);
int result = (int)com.ExecuteNonQuery();
if(result > 0)
{
MessageBox.Show("修改成功!");
this.Form1_Load_1(null, null);
conn.Close();
}
}
}
private void Form1_Load_1(object sender, EventArgs e)
{
using (var conn = new SqlConnection(this.str))
{
conn.Open();
string sql = "select * from Students";
SqlDataAdapter sda = new SqlDataAdapter(sql, conn);
DataSet ds = new DataSet();
sda.Fill(ds);
dataGridView1.DataSource = ds.Tables[0];
conn.Close();
}
}
private void DataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
}
private void DataGridView1_CellClick_1(object sender, DataGridViewCellEventArgs e)
{
txtname.Text = dataGridView1.SelectedRows[0].Cells["name"].Value.ToString();
txtsex.Text = dataGridView1.SelectedRows[0].Cells["sex"].Value.ToString();
txtcollege.Text = dataGridView1.SelectedRows[0].Cells["college"].Value.ToString();
txtid.Text = dataGridView1.SelectedRows[0].Cells["id"].Value.ToString();
txtgrade.Text = dataGridView1.SelectedRows[0].Cells["grade"].Value.ToString();
txtphone.Text = dataGridView1.SelectedRows[0].Cells["phone"].Value.ToString();
txtemail.Text = dataGridView1.SelectedRows[0].Cells["email"].Value.ToString();
txtqq.Text = dataGridView1.SelectedRows[0].Cells["qq"].Value.ToString();
txtroom.Text = dataGridView1.SelectedRows[0].Cells["room"].Value.ToString();
}
private void BtnDelete_Click(object sender, EventArgs e)
{
using(var conn = new SqlConnection(this.str))
{
conn.Open();
string sql = string.Format("delete from Students where id = '{0}'", txtid.Text);
SqlCommand com = new SqlCommand(sql, conn);
int result = (int)com.ExecuteNonQuery();
if(result > 0)
{
MessageBox.Show("删除成功!");
this.Form1_Load_1(null, null);
conn.Close();
}
}
}
private void BtnClear_Click(object sender, EventArgs e)
{
txtname.Text = null;
txtsex.Text = null;
txtcollege.Text = null;
txtid.Text = null;
txtgrade.Text = null;
txtphone.Text = null;
txtemail.Text = null;
txtqq.Text = null;
txtroom.Text = null;
}
}
}
Students表
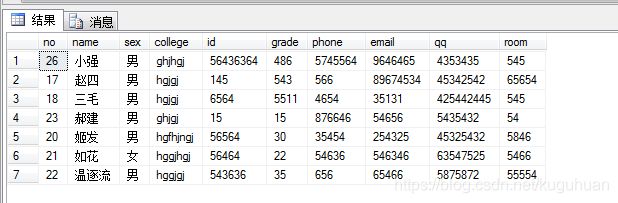