- RPC框架Dubbo深入分析
radcb55226
程序员rpcdubbo网络协议
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门,即可获取!依赖于Zookeeper的稳定性Redis支持基于客户端双写的集群方式,性能高要求服务器时间同步,用于检查心跳过期脏数据Multicast去中心化,不需要安装注册中心依赖于网络拓普和路由,跨机房有风险SimpleDogfooding,注册中心本身也是一个标准的RPC服务没有集群支持,可能单点故障cl
- Vue学习教程-04数据代理
番茄番茄君
vue.js学习javascript
文章目录一、什么是数据代理二、数据代理过程三、vue数据代理应用1.数据代理代码2.代理底层逻辑总结一、什么是数据代理数据代理是一种技术,通过代理、拦截对象属性及方法的访问请求,实现与该对象的交互。在Vue中,数据代理是指在Vue实例化一个组件时,Vue会将组件中的data属性中的数据转化为getter/setter,并将这些getter/setter注册到Vue的响应式系统中,在Vue实例中访问
- DeepSeek 助力 Vue 开发:打造丝滑的日期选择器(Date Picker),未使用第三方插件
宝码香车
#DeepSeek#Vuevue.js前端javascriptecmascriptDeepSeek
前言:哈喽,大家好,今天给大家分享一篇文章!并提供具体代码帮助大家深入理解,彻底掌握!创作不易,如果能帮助到大家或者给大家一些灵感和启发,欢迎收藏+关注哦目录DeepSeek助力Vue开发:打造丝滑的日期选择器(DatePicker),未使用第三方插件前言进入安装好的DeepSeek页面效果指令输入**属性(Props)****事件(Events)**组件代码,src\components\Dat
- Docker 与持续集成 / 持续部署(CI/CD)的集成(二)
计算机毕设定制辅导-无忧学长
#Dockerdockerci/cd容器
五、代码示例与解释(一)Dockerfile示例以下是一个简单的基于PythonFlask应用的Dockerfile示例:#使用Python3.10-slim作为基础镜像FROMpython:3.10-slim#设置工作目录WORKDIR/app#复制项目文件到容器内的工作目录COPY./app#安装项目依赖RUNpipinstall-rrequirements.txt#暴露应用运行的端口EXPO
- 【ISO 14229-1:2023 UDS诊断(ECU复位0x11服务)测试用例CAPL代码全解析①】
车端域控测试工程师
测试用例汽车学习经验分享CANoeCapl
ISO14229-1:2023UDS诊断【ECU复位0x11服务】_TestCase01作者:车端域控测试工程师更新日期:2025年02月16日关键词:UDS诊断协议、ECU复位服务、0x11服务、ISO14229-1:2023TC11-001测试用例用例ID测试场景验证要点参考条款预期结果TC11-001硬复位功能验证发送0x110x01请求硬复位§8.2.1收到0x510x01响应,ECU重启
- 设计模式之单例模式
Forget the Dream
设计模式设计模式单例模式c++
概念单例模式是一种创建型设计模式1,它保证一个类在整个系统运行期间只有一个实例,并且提供一个全局访问点来访问这个唯一实例。无论在系统的任何地方、任何时间,对该类进行实例化操作,获取到的都是同一个对象实例。这就像在一个公司中,通常会有一个唯一的总经理,无论从哪个部门去获取总经理这个角色的实例,得到的都是同一个人。实现原理实现方式一般通过将类的构造函数设置为私有,防止外部代码通过常规的new操作符来创
- 微信小程序中的canvas(2D)
mini..
微信小程序小程序
微信小程序中的组件提供了一个用于绘制图形的画布,支持2D绘图。以下是对微信小程序中2D组件的详细介绍,包括属性、方法、事件和示例代码。一、组件属性基本属性canvas-id:在自定义组件中使用时,指定组件的唯一标识符,用于创建绘图上下文。disable-scroll:是否禁用滚动,通过设置为true可以禁用画布的滚动事件。二、绘图上下文对象(CanvasContext)通过wx.createCan
- ORB-SLAM2源码学习:System.cc:System::System SLAM系统的构造函数
PaLu-LvL
计算机视觉#ORB-SLAM2c++学习计算机视觉算法opencv
前言ORB-SLAM2源码学习:rgbd_tum.cc源文件-CSDN博客之前我们在具体的实例的代码中初始化了一个SLAM的系统,现在让我们来看看这个SLAM的构造函数具体进行了什么操作。总的来说:该函数主要干了以下事情:1.初始化一些参数(列表初始化)2.加载并检查配置文件和词汇表3.创建一些对象如关键帧数据库、地图、绘制器等。4.启动并初始化多个线程:跟踪线程、本地建图线程、回环检测线程、可视
- DeepSeek 助力 Vue 开发:打造丝滑的瀑布流布局(Masonry Layout)
宝码香车
#DeepSeek#Vuevue.js前端javascriptDeepSeekecmascript
前言:哈喽,大家好,今天给大家分享一篇文章!并提供具体代码帮助大家深入理解,彻底掌握!创作不易,如果能帮助到大家或者给大家一些灵感和启发,欢迎收藏+关注哦目录DeepSeek助力Vue开发:打造丝滑的瀑布流布局(MasonryLayout)前言进入安装好的DeepSeek页面效果指令输入**组件属性(Props)****组件事件(Events)**think组件代码1.组件实现代码2.调用示例3.
- Windows配置永久路由同时访问内外网
ღ张明宇࿐
bash
1.桌面新建一个txt文本文档,然后输入一下代码:routedelete0.0.0.0routeadd0.0.0.0mask0.0.0.0192.168.43.1routeadd10.37.0.0mask255.255.0.010.20.6.1解释如下:删除默认设置routedelete0.0.0.0外网路由,全走无线routeadd0.0.0.0mask0.0.0.0192.168.0.1公司内
- DataWorks Copilot × DeepSeek-R1 来了!给你的智能数据开发加满 buff
DataWorksCopilot×DeepSeek-R1来了!DataWorksCopilot,作为一站式智能数据开发治理平台DataWorks的智能助手,借助AI推理和自然语言处理能力,通过提供代码辅助和智能应用开发功能,为开发者和企业用户带来便捷高效的数据开发体验。现在,DataWorksCopilot与DeepSeek-R1模型深度对接,支持DeepSeek-R1-671B模型与DeepSe
- 深入了解与全面使用DeepSeek:从基础到高级应用
一位卑微的码农
人工智能大数据java-eespringboot
引言随着AI技术的发展,DeepSeek作为一款先进的智能助手,为用户提供了强大的文本生成、代码分析、数学公式处理等能力。本文将详细介绍DeepSeek的基础知识、安装配置、API调用方法以及高级应用技巧,帮助你充分挖掘这一工具的潜力。一、认识DeepSeek1.1DeepSeek简介DeepSeek是由深度求索公司开发的人工智能平台,它支持三种主要模式:基础模型(V3)、深度思考(R1)和联网搜
- 【数值模型后处理系列】通风系数计算及垂直层插值
北潇
数值模型Python实用基础技能pythonWRF
一、通风系数1.1通风系数简介通风系数(VentilationCoefficient,VC)可以用来表征扩散条件,其计算公式如下(参考USIyerandPErnestRaj的文章):其中mixingdepth选用WRF输出的边界层高度(PBLH),meanwindspeed近似用边界层顶的风速与地面风速做平均(当然也可多选几层)。1.2Python代码实现VC的计算计算VC的示例代码:fromne
- 批量检测微信小程序封禁状态的 PHP 脚本示例
php
代码解析:设置AppID列表:修改$appIds数组,将'appid1','appid2','appid3'替换为您的小程序AppID。状态检查流程:使用file_get_contents函数请求API,获取小程序的状态信息。解析API返回结果:通过json_decode解析JSON格式的响应,根据code字段判断小程序的封禁状态,并输出相应提示。错误处理:如果接口调用失败或返回格式错误,脚本将输
- 批量检测多个微信小程序的封禁状态源码、接口
php微信小程序
PHP脚本示例,用于批量检测多个微信小程序的封禁状态。您可以将脚本中的appid1,appid2,appid3替换为实际的小程序应用ID,从而获取每个小程序的状态信息。代码说明:设置需要检查的AppID列表:修改$appIds数组中的'appid1','appid2','appid3'为您实际的小程序应用ID。检查每个AppID的状态:脚本通过file_get_contents函数调用API接口,
- 2024年4月批量检测微信小程序是否封禁接口源码
php小程序
上述是代码,$appids=array('appid1','appid2','appid3');//使用实际的appid,在这一行,输入你需要检测appid即可,就可以得到检测结果
- JavaScript设计模式 -- 状态模式
鎈卟誃筅甡
javascript设计模式状态模式
在软件开发中,很多对象的行为会随着其内部状态的变化而改变。如果将所有状态逻辑写在一个类中,代码不仅臃肿而且难以维护。**状态模式(StatePattern)**正是为了解决这个问题而设计的。通过将对象的状态封装成独立的状态类,并将状态相关的行为转移到这些状态类中,状态模式让对象在内部状态发生变化时自动切换行为,达到了将状态转换与行为实现分离的目的。本文将详细介绍状态模式的核心思想、基本结构与优缺点
- 从Paxos到Zookeeper笔记1——第一章:分布式架构
半臻(火白)
分布式大数据zookeeper
第1章:分布式架构将多台机器组成分布式的处理方式越来越收到业界的青睐。1.1从集中式到分布式由于大型主机拥有卓越的性能和良好的稳定性,在单机处理方面优势非常明显。但是随着计算机系统向网络化和微型化的方向发展,传统的集中式处理越来越不适应人们的需求。大型主机的缺点:(1)操作难度大。(2)价格昂贵(3)虽然大型主机稳定,但是一旦出现故障后果严重(4)扩容非常困难阿里提出的“去IOE”运动,让计算和存
- 如何在不依赖函数调用功能的情况下结合工具与大型语言模型
Jason9510
语言模型人工智能
当大型语言模型(LLM)原生不支持函数调用功能时,如何实现智能工具调度?本文通过自然语言解析+结构化输出控制的方法来实现。GitHub代码地址核心实现步骤定义工具函数使用@tool装饰器声明可调用工具:fromlangchain_core.toolsimporttool@tooldefmultiply_by_max(a:int,b:list[int])->int:"""将a乘以b列表中的最大值""
- 2025最新版二级域名分发最新开心版 支持易支付接口和聚合登录接口
专业软件系统开发
源码下载付费域名分发域名分发系统源码
内容目录一、详细介绍宝塔面板环境PHP版本8.0至8.3PHP扩展SG15Mysql5.6或5.71Panel环境二、效果展示1.部分代码2.效果图展示请添加图片描述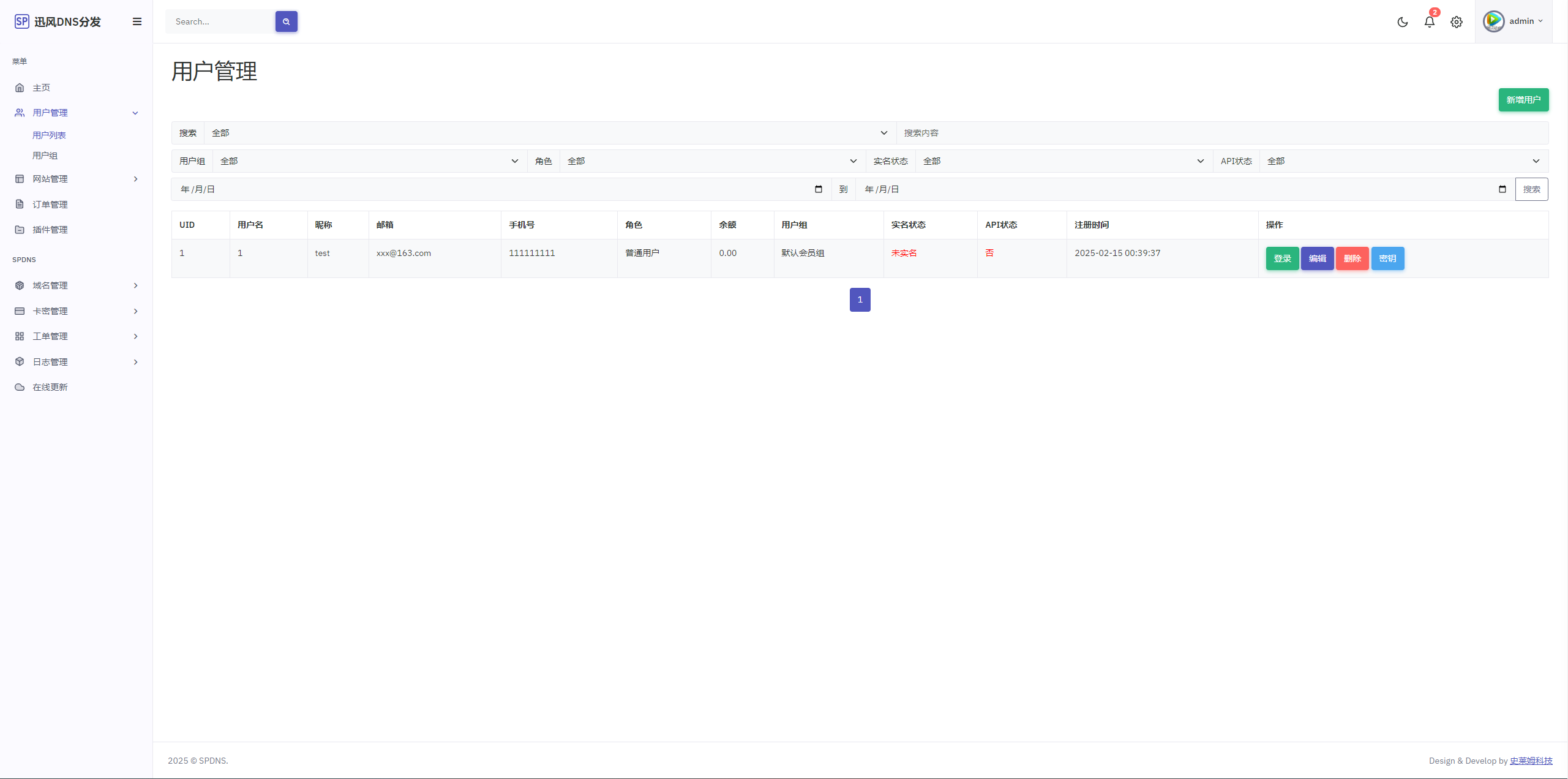三、学习资料下载一、详细介绍一站式对域名进行二级分发,自助添加,自助修改
- 使用bitnamiredis-sentinel部署Redis 哨兵模式
m0_67265654
面试学习路线阿里巴巴sentinelredis数据库
使用bitnami/redis-sentinel部署Redis哨兵模式为什么使用Bitnami镜像?Bitnami密切跟踪上游源代码更改,并使用我们的自动化系统及时发布此镜像的新版本。借助Bitnami镜像,可以尽快提供最新的错误修复和功能。Bitnami容器、虚拟机和云镜像使用相同的组件和配置方法-可以根据你的项目需求轻松切换格式。我们所有的镜像都基于minideb,这是一个基于Debian的极
- vue3 - 【完整源码】实现网页整屏大量图片、div 容器的自适应瀑布流布局,宽高度不固定的列表式瀑布流展示效果(高效无 BUG 网站瀑布流效果,超详细代码注释,可根据需求快速进行改造!)
街尾杂货店&
前端组件与功能(开箱即用)vue3瀑布流布局详细教程vue3图片列表瀑布流组件宽高不固定的div实现瀑布vue3最好用的瀑布流组件插件vue3瀑布流布局完整示例源码
效果图在vue3.js网站项目中,实现图片、普通div容器的瀑布流效果完整示例,支持动态加载数据、自定义一行放多少个、各列之间的间距等等!你可以直接复制组件源码,按照配置文档稍微改改就能用到你的项目中去了,比绝大部分文章提供的示例都要流畅、快速。核心组件源码组件的存放位置无所谓,最后使用的时候能正确引入就行了。创建瀑布流组件Waterfall.vue,复制代码。
- Knox原理与代码实例讲解
AI天才研究院
计算DeepSeekR1&大数据AI人工智能大模型计算科学神经计算深度学习神经网络大数据人工智能大型语言模型AIAGILLMJavaPython架构设计AgentRPA
Knox原理与代码实例讲解1.背景介绍在现代分布式系统中,安全性和隔离性是非常重要的需求。ApacheKnox是一个反向代理服务器,旨在为ApacheHadoop集群提供单一入口点,增强安全性和集中化管理。它位于Hadoop集群与客户端应用程序之间,充当网关和负载均衡器的角色。Knox的主要目标是:提供集中式身份验证和授权,减轻客户端应用程序的负担。实现多租户支持,允许不同的组织或部门安全地共享同
- 常见数据结构的简介(基本概念 & 操作 & 时间复杂度)
子诚之
编程
文章目录0.概览1.线性表、栈和队列2.数组2.1基本操作1)时间复杂度2)案例3.字符串3.1存储结构3.2基本操作1)时间复杂度2)案例:最大公共字符串4.二叉树4.1储存结构4.2基本操作1)时间复杂度2)案例:使用字典树判断字符串是否存在5.哈希/散列表5.1哈希函数5.2基本操作1)时间复杂度2)案例:构建哈希表《重学数据结构与算法》学习笔记0.概览数据结构增删查特点线性表变长栈队列数组
- app,waf笔记
qq_45981247
笔记
API攻防知识点:1、HTTP接口类-测评2、RPC类接口-测评3、WebService类-测评内容点:SOAP(SimpleObjectAccessProtocol)简单对象访问协议是交换数据的一种协议规范,是一种轻量级的、简单的、基于XML(标准通用标记语言下的一个子集)的协议,它被设计成在WEB上交换结构化的和固化的信息。SOAP部署WebService的专有协议。SOAP使用HTTP来发送
- Python随机森林算法详解与案例实现
闲人编程
python算法python随机森林数据分析人工智能
目录Python随机森林算法详解与案例实现1、随机森林算法概述2、随机森林的原理3、实现步骤4、分类案例:使用随机森林预测鸢尾花品种4.1数据集介绍4.2代码实现4.3代码解释4.4运行结果5、回归案例:使用随机森林预测波士顿房价5.1数据集介绍5.2代码实现5.3代码解释5.4运行结果6、随机森林的优缺点7、改进方向8、应用场景9、总结Python随机森林算法详解与案例实现1、随机森林算法概述随
- DeepSeek 助力 Vue 开发:打造丝滑的开关切换(Switch)
宝码香车
#DeepSeekvue.js前端javascriptDeepSeekecmascript
前言:哈喽,大家好,今天给大家分享一篇文章!并提供具体代码帮助大家深入理解,彻底掌握!创作不易,如果能帮助到大家或者给大家一些灵感和启发,欢迎收藏+关注哦目录DeepSeek助力Vue开发:打造丝滑的开关切换(Switch)前言进入安装好的DeepSeek页面效果指令输入think组件代码组件特点说明:额外建议:代码测试页面效果自己部署DeepSeek安装地址相关文章️✍️️️️⚠️⬇️·正文开始
- Android技术笔记
桃花镇童长老ᥫ᭡
Androidandroid笔记
1、TextView设置宽度(行内可包含的字符数)android:ems="12"//宽度为12个字符的宽度。android:maxEms="18"//最大宽度为18个字符的宽度。android:minEms="10"//最小宽度为10个字符的宽度。android:letterSpacing="0.1"//控制文字水平间距注意事项:1、只有在android:layout_width=“wrap_c
- 基于深度学习YOLOv10的PCB板缺陷检测系统(附完整资源+PySide6界面+训练代码)
人工智能_SYBH
深度学习YOLO人工智能目标检测python
引言:在现代制造业中,电子元件和PCB(印刷电路板)是非常重要的基础设施。PCB缺陷检测是生产过程中至关重要的一步。传统的缺陷检测方法主要依靠人工检查,这不仅效率低,而且容易受到人眼疲劳的影响。随着深度学习技术的不断发展,基于深度学习的自动化缺陷检测已成为研究的热点,尤其是在计算机视觉领域。YOLO(YouOnlyLookOnce)系列算法凭借其高速和高精度的优势,成为了目标检测领域的佼佼者。本文
- 【蓝桥杯C/C++】彻底理解双指针算法
不会喷火的小火龙
#蓝桥杯算法与数据结构算法数据结构c++
目录学习目标什么是双指针?双指针的分类核心思想模板写法经典例题移除元素双指针法分析题意具体代码最长连续不重复子序列输入格式输出格式数据范围输入样例:输出样例:核心思路数组元素的目标和输入格式输出格式数据范围输入样例:输出样例:核心思路总结一下学习目标了解双指针算法是什么以及分类理解双指针算法的原理会用代码编写双指针算法在实际题目中灵活运用双指针在数组的开章中我们提到了这个算法,如果没有看的话可以学
- java封装继承多态等
麦田的设计者
javaeclipsejvmcencapsulatopn
最近一段时间看了很多的视频却忘记总结了,现在只能想到什么写什么了,希望能起到一个回忆巩固的作用。
1、final关键字
译为:最终的
&
- F5与集群的区别
bijian1013
weblogic集群F5
http请求配置不是通过集群,而是F5;集群是weblogic容器的,如果是ejb接口是通过集群。
F5同集群的差别,主要还是会话复制的问题,F5一把是分发http请求用的,因为http都是无状态的服务,无需关注会话问题,类似
- LeetCode[Math] - #7 Reverse Integer
Cwind
java题解MathLeetCodeAlgorithm
原题链接:#7 Reverse Integer
要求:
按位反转输入的数字
例1: 输入 x = 123, 返回 321
例2: 输入 x = -123, 返回 -321
难度:简单
分析:
对于一般情况,首先保存输入数字的符号,然后每次取输入的末位(x%10)作为输出的高位(result = result*10 + x%10)即可。但
- BufferedOutputStream
周凡杨
首先说一下这个大批量,是指有上千万的数据量。
例子:
有一张短信历史表,其数据有上千万条数据,要进行数据备份到文本文件,就是执行如下SQL然后将结果集写入到文件中!
select t.msisd
- linux下模拟按键输入和鼠标
被触发
linux
查看/dev/input/eventX是什么类型的事件, cat /proc/bus/input/devices
设备有着自己特殊的按键键码,我需要将一些标准的按键,比如0-9,X-Z等模拟成标准按键,比如KEY_0,KEY-Z等,所以需要用到按键 模拟,具体方法就是操作/dev/input/event1文件,向它写入个input_event结构体就可以模拟按键的输入了。
linux/in
- ContentProvider初体验
肆无忌惮_
ContentProvider
ContentProvider在安卓开发中非常重要。与Activity,Service,BroadcastReceiver并称安卓组件四大天王。
在android中的作用是用来对外共享数据。因为安卓程序的数据库文件存放在data/data/packagename里面,这里面的文件默认都是私有的,别的程序无法访问。
如果QQ游戏想访问手机QQ的帐号信息一键登录,那么就需要使用内容提供者COnte
- 关于Spring MVC项目(maven)中通过fileupload上传文件
843977358
mybatisspring mvc修改头像上传文件upload
Spring MVC 中通过fileupload上传文件,其中项目使用maven管理。
1.上传文件首先需要的是导入相关支持jar包:commons-fileupload.jar,commons-io.jar
因为我是用的maven管理项目,所以要在pom文件中配置(每个人的jar包位置根据实际情况定)
<!-- 文件上传 start by zhangyd-c --&g
- 使用svnkit api,纯java操作svn,实现svn提交,更新等操作
aigo
svnkit
原文:http://blog.csdn.net/hardwin/article/details/7963318
import java.io.File;
import org.apache.log4j.Logger;
import org.tmatesoft.svn.core.SVNCommitInfo;
import org.tmateso
- 对比浏览器,casperjs,httpclient的Header信息
alleni123
爬虫crawlerheader
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException
{
String type=req.getParameter("type");
Enumeration es=re
- java.io操作 DataInputStream和DataOutputStream基本数据流
百合不是茶
java流
1,java中如果不保存整个对象,只保存类中的属性,那么我们可以使用本篇文章中的方法,如果要保存整个对象 先将类实例化 后面的文章将详细写到
2,DataInputStream 是java.io包中一个数据输入流允许应用程序以与机器无关方式从底层输入流中读取基本 Java 数据类型。应用程序可以使用数据输出流写入稍后由数据输入流读取的数据。
- 车辆保险理赔案例
bijian1013
车险
理赔案例:
一货运车,运输公司为车辆购买了机动车商业险和交强险,也买了安全生产责任险,运输一车烟花爆竹,在行驶途中发生爆炸,出现车毁、货损、司机亡、炸死一路人、炸毁一间民宅等惨剧,针对这几种情况,该如何赔付。
赔付建议和方案:
客户所买交强险在这里不起作用,因为交强险的赔付前提是:“机动车发生道路交通意外事故”;
如果是交通意外事故引发的爆炸,则优先适用交强险条款进行赔付,不足的部分由商业
- 学习Spring必学的Java基础知识(5)—注解
bijian1013
javaspring
文章来源:http://www.iteye.com/topic/1123823,整理在我的博客有两个目的:一个是原文确实很不错,通俗易懂,督促自已将博主的这一系列关于Spring文章都学完;另一个原因是为免原文被博主删除,在此记录,方便以后查找阅读。
有必要对
- 【Struts2一】Struts2 Hello World
bit1129
Hello world
Struts2 Hello World应用的基本步骤
创建Struts2的Hello World应用,包括如下几步:
1.配置web.xml
2.创建Action
3.创建struts.xml,配置Action
4.启动web server,通过浏览器访问
配置web.xml
<?xml version="1.0" encoding="
- 【Avro二】Avro RPC框架
bit1129
rpc
1. Avro RPC简介 1.1. RPC
RPC逻辑上分为二层,一是传输层,负责网络通信;二是协议层,将数据按照一定协议格式打包和解包
从序列化方式来看,Apache Thrift 和Google的Protocol Buffers和Avro应该是属于同一个级别的框架,都能跨语言,性能优秀,数据精简,但是Avro的动态模式(不用生成代码,而且性能很好)这个特点让人非常喜欢,比较适合R
- lua set get cookie
ronin47
lua cookie
lua:
local access_token = ngx.var.cookie_SGAccessToken
if access_token then
ngx.header["Set-Cookie"] = "SGAccessToken="..access_token.."; path=/;Max-Age=3000"
end
- java-打印不大于N的质数
bylijinnan
java
public class PrimeNumber {
/**
* 寻找不大于N的质数
*/
public static void main(String[] args) {
int n=100;
PrimeNumber pn=new PrimeNumber();
pn.printPrimeNumber(n);
System.out.print
- Spring源码学习-PropertyPlaceholderHelper
bylijinnan
javaspring
今天在看Spring 3.0.0.RELEASE的源码,发现PropertyPlaceholderHelper的一个bug
当时觉得奇怪,上网一搜,果然是个bug,不过早就有人发现了,且已经修复:
详见:
http://forum.spring.io/forum/spring-projects/container/88107-propertyplaceholderhelper-bug
- [逻辑与拓扑]布尔逻辑与拓扑结构的结合会产生什么?
comsci
拓扑
如果我们已经在一个工作流的节点中嵌入了可以进行逻辑推理的代码,那么成百上千个这样的节点如果组成一个拓扑网络,而这个网络是可以自动遍历的,非线性的拓扑计算模型和节点内部的布尔逻辑处理的结合,会产生什么样的结果呢?
是否可以形成一种新的模糊语言识别和处理模型呢? 大家有兴趣可以试试,用软件搞这些有个好处,就是花钱比较少,就算不成
- ITEYE 都换百度推广了
cuisuqiang
GoogleAdSense百度推广广告外快
以前ITEYE的广告都是谷歌的Google AdSense,现在都换成百度推广了。
为什么个人博客设置里面还是Google AdSense呢?
都知道Google AdSense不好申请,这在ITEYE上也不是讨论了一两天了,强烈建议ITEYE换掉Google AdSense。至少,用一个好申请的吧。
什么时候能从ITEYE上来点外快,哪怕少点
- 新浪微博技术架构分析
dalan_123
新浪微博架构
新浪微博在短短一年时间内从零发展到五千万用户,我们的基层架构也发展了几个版本。第一版就是是非常快的,我们可以非常快的实现我们的模块。我们看一下技术特点,微博这个产品从架构上来分析,它需要解决的是发表和订阅的问题。我们第一版采用的是推的消息模式,假如说我们一个明星用户他有10万个粉丝,那就是说用户发表一条微博的时候,我们把这个微博消息攒成10万份,这样就是很简单了,第一版的架构实际上就是这两行字。第
- 玩转ARP攻击
dcj3sjt126com
r
我写这片文章只是想让你明白深刻理解某一协议的好处。高手免看。如果有人利用这片文章所做的一切事情,盖不负责。 网上关于ARP的资料已经很多了,就不用我都说了。 用某一位高手的话来说,“我们能做的事情很多,唯一受限制的是我们的创造力和想象力”。 ARP也是如此。 以下讨论的机子有 一个要攻击的机子:10.5.4.178 硬件地址:52:54:4C:98
- PHP编码规范
dcj3sjt126com
编码规范
一、文件格式
1. 对于只含有 php 代码的文件,我们将在文件结尾处忽略掉 "?>" 。这是为了防止多余的空格或者其它字符影响到代码。例如:<?php$foo = 'foo';2. 缩进应该能够反映出代码的逻辑结果,尽量使用四个空格,禁止使用制表符TAB,因为这样能够保证有跨客户端编程器软件的灵活性。例
- linux 脱机管理(nohup)
eksliang
linux nohupnohup
脱机管理 nohup
转载请出自出处:http://eksliang.iteye.com/blog/2166699
nohup可以让你在脱机或者注销系统后,还能够让工作继续进行。他的语法如下
nohup [命令与参数] --在终端机前台工作
nohup [命令与参数] & --在终端机后台工作
但是这个命令需要注意的是,nohup并不支持bash的内置命令,所
- BusinessObjects Enterprise Java SDK
greemranqq
javaBOSAPCrystal Reports
最近项目用到oracle_ADF 从SAP/BO 上调用 水晶报表,资料比较少,我做一个简单的分享,给和我一样的新手 提供更多的便利。
首先,我是尝试用JAVA JSP 去访问的。
官方API:http://devlibrary.businessobjects.com/BusinessObjectsxi/en/en/BOE_SDK/boesdk_ja
- 系统负载剧变下的管控策略
iamzhongyong
高并发
假如目前的系统有100台机器,能够支撑每天1亿的点击量(这个就简单比喻一下),然后系统流量剧变了要,我如何应对,系统有那些策略可以处理,这里总结了一下之前的一些做法。
1、水平扩展
这个最容易理解,加机器,这样的话对于系统刚刚开始的伸缩性设计要求比较高,能够非常灵活的添加机器,来应对流量的变化。
2、系统分组
假如系统服务的业务不同,有优先级高的,有优先级低的,那就让不同的业务调用提前分组
- BitTorrent DHT 协议中文翻译
justjavac
bit
前言
做了一个磁力链接和BT种子的搜索引擎 {Magnet & Torrent},因此把 DHT 协议重新看了一遍。
BEP: 5Title: DHT ProtocolVersion: 3dec52cb3ae103ce22358e3894b31cad47a6f22bLast-Modified: Tue Apr 2 16:51:45 2013 -070
- Ubuntu下Java环境的搭建
macroli
java工作ubuntu
配置命令:
$sudo apt-get install ubuntu-restricted-extras
再运行如下命令:
$sudo apt-get install sun-java6-jdk
待安装完毕后选择默认Java.
$sudo update- alternatives --config java
安装过程提示选择,输入“2”即可,然后按回车键确定。
- js字符串转日期(兼容IE所有版本)
qiaolevip
TODateStringIE
/**
* 字符串转时间(yyyy-MM-dd HH:mm:ss)
* result (分钟)
*/
stringToDate : function(fDate){
var fullDate = fDate.split(" ")[0].split("-");
var fullTime = fDate.split("
- 【数据挖掘学习】关联规则算法Apriori的学习与SQL简单实现购物篮分析
superlxw1234
sql数据挖掘关联规则
关联规则挖掘用于寻找给定数据集中项之间的有趣的关联或相关关系。
关联规则揭示了数据项间的未知的依赖关系,根据所挖掘的关联关系,可以从一个数据对象的信息来推断另一个数据对象的信息。
例如购物篮分析。牛奶 ⇒ 面包 [支持度:3%,置信度:40%] 支持度3%:意味3%顾客同时购买牛奶和面包。 置信度40%:意味购买牛奶的顾客40%也购买面包。 规则的支持度和置信度是两个规则兴
- Spring 5.0 的系统需求,期待你的反馈
wiselyman
spring
Spring 5.0将在2016年发布。Spring5.0将支持JDK 9。
Spring 5.0的特性计划还在工作中,请保持关注,所以作者希望从使用者得到关于Spring 5.0系统需求方面的反馈。