class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
if(!head) return nullptr;
if(head->next==nullptr) return head;
ListNode helpNode(0);
helpNode.next=head;
ListNode* first=&helpNode;
ListNode* second=head;
ListNode* third=head->next;
ListNode* cur=&helpNode;
if(second->val!=third->val){
cur->next=second;
cur=cur->next;
}
first=first->next;
second=second->next;
third=third->next;
while(third){
if(second->val!=first->val&&second->val!=third->val){
cur->next=second;
cur=cur->next;
}
first=first->next;
second=second->next;
third=third->next;
}
if(second->val!=first->val){
cur->next=second;
cur=cur->next;
}
cur->next=nullptr;
return helpNode.next;
}
};
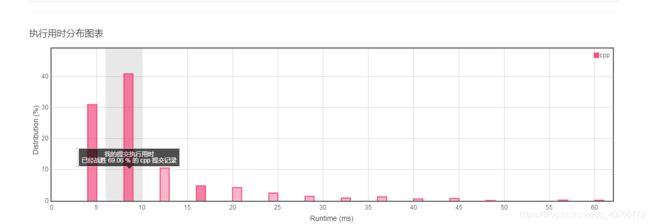
class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
if(head==nullptr) return nullptr;
ListNode helpNode(0);
helpNode.next=head;
ListNode* cur=head;
ListNode* pre=&helpNode;
while(cur){
while(cur->next&&cur->val==cur->next->val){
cur=cur->next;
}
if(pre->next==cur){
pre->next=cur;
pre=pre->next;
}else{
pre->next=cur->next;
}
cur=cur->next;
}
return helpNode.next;
}
};
没在意cur->next->val漏了cur->next判空
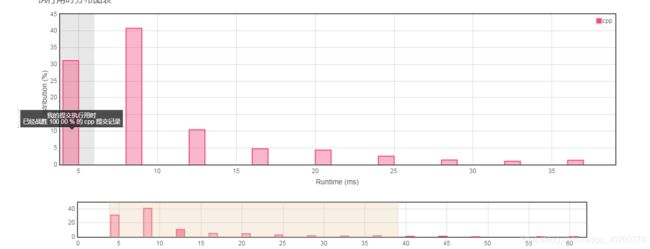