1 MyBatis简介
1 简介
MyBatis半自动化的ORM框架
Hibernate全自动的ORM框架
ORM:Object(JavaBean) Relation(关系:和数据库记录的关系)Mapping(数据库的记录和JavaBean对应)
1 HelloWorld(MyBatis操作数据库的基本环节)
1 创建数据表
2 JavaBean类
3 编写dao接口
4 MyBatis操作数据库★
1)导包
mybatis-3.2.8.jar
mysql-connector-java-5.1.37-bin.jar//数据库驱动
log4j.jar
注:log4j依赖配置文件。 这个配置文件可以是xml,也可以是properties文件。但是要求名字必须是log4j。必须放在类路径下(直接放在源码包下)
2)编写myBatis全局配置文件
这个配置文件的作用就是用来指导MyBatis如何正常工作。Xml配置文件中包含了对MyBatis系统的核心设置,包含获取数据库连接实例的数据源(DataSource)和决定事务范围和控制方式的事务管理器(TransactionManager)。从官方文档复制
注意:如果编写文件时没有提示,就需要导入约束文件。约束文件的URI地址:http://mybatis.org/dtd/mybatis-3-config.dtd。导入步骤:window-perference-xml-xml Catalog
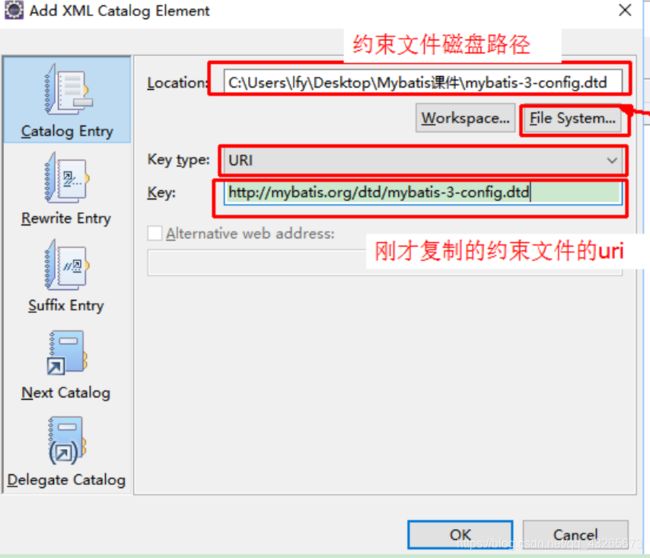
【myBatis全局配置文件】
- 编写SQL映射文件。
这个文件的作用就是PersonDao的实现文件,相当于PersonDaoImpl实现类。从官方文档复制
注:这个配置文件要有提示,也需要导入约束文件。约束文件的URI:http://mybatis.org/dtd/mybatis-3-mapper.dtd
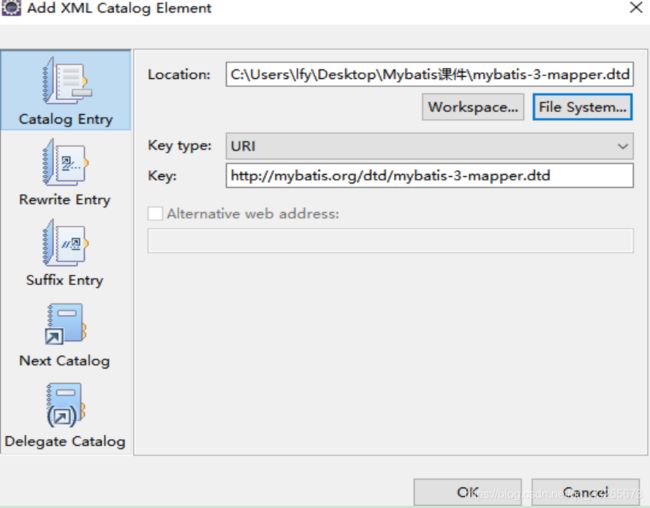
【编写SQL映射文件】
INSERT INTO tal_person(NAME,age,birth,registerTime,salary)
VALUES(#{name},#{age},#{birth},#{registerTime},#{salary})
UPDATE tal_person SET
NAME=#{name},age=#{age},birth=#{birth},
registerTime=#{registerTime},salary=#{salary}
WHERE id=#{id}
DELETE FROM tal_person WHERE id=#{id}
5 测试
public class MyBatisTest {
SqlSessionFactory sessionFactory = null;
//该方法在所有单元测试方法,前运行
@Before
public void getSession() throws IOException {
String resource = "mybatis-config.xml";
// 从类路径下获取这个文件的流
InputStream stream = Resources.getResourceAsStream(resource);
// 根据配置文件的流,使用SqlSessionFactory的建造器创建一个sqlsessionFactory对象
sessionFactory = new SqlSessionFactoryBuilder().build(stream);
}
// 根据id获取
@Test
public void testDelete() {
// 打开一次数据库连接的会话
SqlSession session = sessionFactory.openSession();
try {
// 执行更新操作
// 获取映射
PersonDao personDao = session.getMapper(PersonDao.class);
personDao.deletePerson(3);
session.commit();
} finally {
session.close();
}
}
// 获取所有
@Test
public void testGetAll() {
// 打开一次数据库连接的会话
SqlSession session = sessionFactory.openSession();
try {
// 执行更新操作
// 获取映射
PersonDao personDao = session.getMapper(PersonDao.class);
List all = personDao.getAll();
for (Person person : all) {
System.out.println(person);
}
session.commit();
} finally {
session.close();
}
}
// 根据id获取
@Test
public void testQueryById() {
// 打开一次数据库连接的会话
SqlSession session = sessionFactory.openSession();
try {
// 执行更新操作
// 获取映射
PersonDao personDao = session.getMapper(PersonDao.class);
Person person = personDao.getPersonById(2);
System.out.println(person);
session.commit();
} finally {
session.close();
}
}
// 更新操作
@Test
public void testUpdate() {
// 打开一次数据库连接的会话
SqlSession session = sessionFactory.openSession();
try {
// 执行更新操作
// 获取映射
PersonDao personDao = session.getMapper(PersonDao.class);
Person person = personDao.getPersonById(2);
person.setName("大圣归来");
personDao.updatePerson(person);
session.commit();
} finally {
session.close();
}
}
@Test
public void test() throws IOException {
// 3、SqlSessionFactory:一定是用来创建SqlSession的
// SqlSession:和数据库的一次会话。类似于我们的Connection;
// 4、使用SqlSessionFactory开启和数据库的一次会话
SqlSession session = sessionFactory.openSession();
// 5、SqlSession可以完成完整的增删改查
// 6、获取到接口是动态代理
try {
PersonDao dao = session.getMapper(PersonDao.class);
Person person = new Person();
person.setAge(20);
person.setName("张三丰");
person.setSalary(298.98);
person.setBirth(new Date());
// 7、保存
dao.savePerson(person);
// 8、提交会话,增删改才能生效
session.commit();
} catch (Exception e) {
e.printStackTrace();
} finally {
// 9、关闭会话。
session.close();
}
}
}
2 MyBatis全局配置文件详解
全局配置文件中的标签顺序是固定的。
3 SQL映射文件详解
INSERT INTO tbl_person(NAME,age,birth,registerTime,salary)
VALUES(#{name},#{age},#{birth},#{registerTime},#{salary})
parameterType="map">
SELECT * FROM tbl_person WHERE id=#{id}
DELETE FROM tbl_person WHERE id=${id} AND name="${name}"
DELETE FROM tbl_person WHERE id=#{id} AND name=#{name}
DELETE FROM tbl_person WHERE id=#{id}
UPDATE tbl_person set
name=#{name},age=#{age},birth=#{birth},
registerTime=#{registerTime},salary=#{salary}
WHERE id=#{id}
SELECT * FROM tbl_person WHERE id=#{id}
SELECT * FROM tbl_person
resultType="map">
SELECT * FROM tbl_person WHERE id=#{id}
SELECT * FROM tbl_person
4 用注解的方式代替SQL映射文件
可以在PersonDao中的方法上加注解的方式代替SQL映射文件。
【MyBatis配置文件写法】
【PersonDao示例】
public interface PersonDaoAnnotation {
@Insert("INSERT INTO tbl_person(NAME,age,birth,registerTime,salary) "
+ "VALUES(#{name},#{age},#{birth},#{registerTime},#{salary})")
public void savePerson(Person person);
@Delete("DELETE FROM tbl_person WHERE id=#{id}")
public void delPerson(Integer id);
@Update("UPDATE tbl_person set "
+ "name=#{name},age=#{age},birth=#{birth},"
+ "registerTime=#{registerTime},salary=#{salary} "
+ "WHERE id=#{id}")
public void updatePerson(Person person);
@Select("SELECT * FROM tbl_person WHERE id=#{id}")
public Person getPersonById(Integer id);
@Select("SELECT * FROM tbl_person")
public List getAll();
}
2 动态SQL
1 if/where/trim
1 接口方法
public List getStudentByConditionIf(Student student);
2 SQL映射文件
2 choose/when/otherwise
1接口方法
public List getStudentByConditionChoose(Student student);
2 SQL映射
3 foreach
相当于sql语句中的IN查询
1 接口方法
public List getStudentByForeach(@Param("ids")List ids);
2 SQL映射
4 set
用于更新
set动态设置set关键字
Set可以自动删除多余的英文逗号
1 接口方法
public void updateStudent(Student student);
2 SQL映射
UPDATE tbl_student
NAME=#{name},
score=#{score},
birth=#{birth},
age=#{age}
WHERE id=#{id}
3 关联查询
1 一对一
1 连接查询
接口方法:
public Lock getLockById(Integer id);
SQL映射:
javaType="Key">
2 分段查询
接口方法:
public Lock getLockByStep(Integer id);
public Key getKeyById(Integer id);
SQL映射:
select="dao.KeyDao.getKeyById">
3 延时加载
Lock中的key默认每次查询Lock时,key一定根据select指定的sql语句查询,并封装好。但是有时候我们不需要key对象,这样会浪费资源,所以希望在我们用到key时再去查。
延时加载步骤:
1 导入cglib
cglib-2.2.2.jar
asm-3.3.1.jar
2 在myBatis全局配置文件中开启延迟加载策略
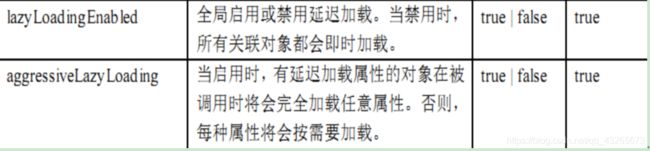
2 一对多
1 员工有一个部门属性
接口方法:
public Employee getEmpById(Integer id);
public Department getDeptById(Integer id);
SQL映射:
<association property="dept"
select="dao.DeptDao.getDeptById"
column="deptId"/>
2 部门都多个员工
接口方法:
public Department getDeptById(Integer id);
public Employee getEmpById(Integer id);
SQL映射:
<collection property="emps"
select="dao.EmpDao.getEmpByDeptId"
column="id">
4 缓存机制
1 一级默认缓存机制
1 默认
默认情况下,mybatis是启用一级缓存的,是SQLSession级别的,即同一个SQLSession接口对象调用相同的select语句。
默认情况下,select使用缓存,增删改不使用缓存。
当SQLSession flush(刷新)close(关闭)后,该session中的所以Cache将全部清空。
2 一级缓存失效的情况
在底层,缓存保存时,使用方法的签名+hashCode+查询sql的id+查询的参数
1 同一个SQLSession,查询条件不同时。因为在底层,所以还会去数据库查询。
2 SQLSession不同时。即使查询条件一样,也会再次发sql查询。
3 同一个SQLSession,查询条件一样。两次查询期间,执行任何一次增删改操作,也会重新查询。
4 同一个SQLSession,手动的清除缓存:session.clearCache()。
2 二级缓存机制
1 二级机制原理
第一次查出的数据会被放在一级缓存中。只有关闭了SQLSession后,一级缓存中的所有数据会同步到二级缓存中。
查询时,会先去二级缓存中看,如果二级缓存中没有,再去一级缓存中,一级缓存中没有,就查询数据库了。
2 开启二级缓存
1 开启全局二级缓存。在mybatis全局配置文件中配置。
2 SQL映射中配置缓存策略
3 bean必须实现序列化接口
public class User implements Serializable
3 整合第三方缓存ehcache
ehcache是一个强大的缓存框架,我们可以使用ehcache来替代mybatis的二级缓存。
1 导包
ehcache-core-2.6.8.jar //ehcache官方核心包
mybatis-ehcache-1.0.3.jar //ehcache与mybatis的整合包
slf4j-api-1.6.1.jar、slf4j-log4j12-1.6.2.jar // ehcache要用的日志包
2 编写配置文件
1 配置文件名字必须是ehcache.xml。放在类路径下
2 内容:
3 SQL映射中配置使用ehcache
4 bean不再需要实现序列化接口
4 和缓存有关的设置
1
作用:操作二级缓存,value必须是true。一级缓存本来就是默认开启的。
2 每一个sql标签都有一个flushCache 属性。增删改操作默认是true,即刷新缓存。一级二级缓存都会被清除。查询操作默认是false,如果设置为true,会禁用二级缓存,不会禁用一级。
3 select标签的useCache属性。true时,使用缓存。False是只能禁用二级缓存,一级依然开启。因此只有flushCache=”true”可以禁用一级缓存,其他设置不能改变一级缓存。
5 MyBatis&Spring&SpringMVC
1 导包
1 Spring环境
1 Spring核心容器+日志
commons-logging-1.1.3.jar
spring-beans-4.0.0.RELEASE.jar
spring-context-4.0.0.RELEASE.jar
spring-core-4.0.0.RELEASE.jar
spring-expression-4.0.0.RELEASE.jar
2 aop模块
com.springsource.net.sf.cglib-2.2.0.jar
com.springsource.org.aopalliance-1.0.0.jar
com.springsource.org.aspectj.weaver-1.6.8.RELEASE.jar
spring-aop-4.0.0.RELEASE.jar
spring-aspects-4.0.0.RELEASE.jar
3 数据库模块(只用到声明式事务功能,操作数据库用MyBatis)
spring-jdbc-4.0.0.RELEASE.jar
spring-orm-4.0.0.RELEASE.jar
spring-tx-4.0.0.RELEASE.jar
2 SpringMVC
1 基础模块
spring-web-4.0.0.RELEASE.jar
spring-webmvc-4.0.0.RELEASE.jar
2 文件上传
commons-fileupload-1.2.1.jar
commons-io-2.0.jar
3 jstl标签库
jstl.jar
Standard.jar
4 数据校验(JSR303)
核心校验:
hibernate-validator-5.0.0.CR2.jar
hibernate-validator-annotation-processor-5.0.0.CR2.jar
依赖包:
classmate-0.8.0.jar
jboss-logging-3.1.1.GA.jar
validation-api-1.1.0.CR1.jar
5 Ajax
jackson-annotations-2.1.5.jar
jackson-core-2.1.5.jar
jackson-databind-2.1.5.jar
6 验证码
kaptcha-2.3.2.jar
3 MyBatis
1 MyBatis核心jar包
mybatis-3.2.8.jar
日志:log4j.jar
2 延迟加载功能依赖cglib
asm-3.3.1.jar
cglib-2.2.2.jar
3 MyBatis使用ehcache做二级缓存
ehcache-core-2.6.8.jar
mybatis-ehcache-1.0.3.jar
slf4j-api-1.6.1.jar
slf4j-log4j12-1.6.2.jar
4 数据库驱动、连接池
mysql-connector-java-5.1.37-bin.jar
c3p0-0.9.1.2.jar
2 配置文件
目录结构:
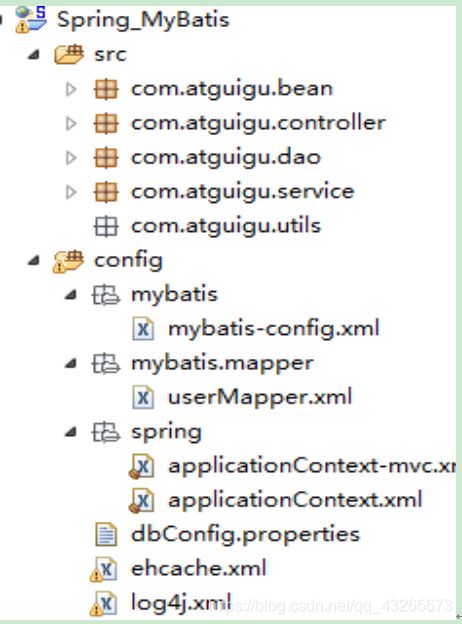
1 Spring配置文件
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
expression="org.springframework.stereotype.Controller" />
expression="org.springframework.web.bind.annotation.ControllerAdvice" />
class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
2 SpringMVC配置文件
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
use-default-filters="false">
expression="org.springframework.stereotype.Controller" />
expression="org.springframework.web.bind.annotation.ControllerAdvice" />
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
3 MyBatis配置文件
4 log4j配置文件
5 数据源dbconfig.properties配置文件
jdbc.username=root
jdbc.password=123456
jdbc.url=jdbc:mysql:///person
jdbc.driver=com.mysql.jdbc.Driver
6 ehcache配置文件
xsi:noNamespaceSchemaLocation="../config/ehcache.xsd">
maxElementsInMemory="1000"
maxElementsOnDisk="10000000"
eternal="false"
overflowToDisk="false"
timeToIdleSeconds="120"
timeToLiveSeconds="120"
diskExpiryThreadIntervalSeconds="120"
memoryStoreEvictionPolicy="LRU">
3 测试
1 bean层user.java
public class User{
private Integer id;
private String name;
private Integer age;
2 dao层UserDao.java
public interface UserDao {
public User getUserById(Integer id);
public void addUser(User user);
}
3 dao层的SQL映射文件userMapper.xml
INSERT INTO tbl_user(NAME,age) VALUES(#{name},#{age})
4 service层
@Service
public class UserService {
@Autowired
private UserDao userDao;
public User getUserById(Integer id){
return userDao.getUserById(id);
}
}
5 controller
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping("/getUser")
public String getUser(@RequestParam("id") Integer id,Map map){
User user = userService.getUserById(id);
map.put("user", user);
return "success";
}
}
6 jsp页面
index.jsp
查询一号用户
Success.jsp
${user }
6 MyBatis逆向工程
数据库建表,根据数据表,自动生成javaBean、接口、xml映射文件
MyBatis Generator(MBG):MyBatis代码生成器
使用步骤:
1 导包
mybatis-generator-core-1.3.2.jar
mysql-connector-java-5.1.37-bin.jar//数据库驱动
2 配置文件
userId="root"
password="123456">
3 测试
public class MBGTest {
public static void main(String[] args) throws Exception {
List warnings = new ArrayList();
boolean overwrite = true;
//获取配置文件,配置文件在当前工程下
File configFile = new File("mbg.xml");
ConfigurationParser cp = new ConfigurationParser(warnings);
Configuration config = cp.parseConfiguration(configFile);
DefaultShellCallback callback = new DefaultShellCallback(overwrite);
MyBatisGenerator myBatisGenerator = new MyBatisGenerator(config, callback, warnings);
myBatisGenerator.generate(null);
System.out.println("运行完成");
}
}
7 MyBatis插件
1 分页插件
1 导包
pagehelper-5.0.0.jar
jsqlparser-0.9.5.jar //sql解析工具
2 配置文件
在mybatis-config.xml中,配置插件。
3 方法调用
public static void main(String[] args) throws Exception {
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory builder = new SqlSessionFactoryBuilder().build(inputStream);
SqlSession openSession = builder.openSession();
BookMapper bookMapper = openSession.getMapper(BookMapper.class);
//配置分页
/*
* 1、导包
* 2、配置插件
* 3、调用
*/
Page