上篇回顾
上一篇printBanner()打印Banner中非了springboot如何打印Banner
目录
1. 创建应用上下文
2. DefaultResourceLoader
3. AbstractApplicationContext
4. GenericApplicationContext
4.1 SimpleAliasRegistry
4.2 DefaultSingletonBeanRegistry
4.3 FactoryBeanRegistrySupport
4.4 AbstractBeanFactory
4.5 AbstractAutowireCapableBeanFactory
4.6 DefaultListableBeanFactory
4.1 SimpleAliasRegistry
5. GenericWebApplicationContext
6. ServletWebServerApplicationContext
7.AnnotationConfigServletWebServerApplicationContext
7.1 AnnotatedBeanDefinitionReader
7.2 ClassPathBeanDefinitionScanner
8. 总结
1. 创建应用上下文
在初始化SpringApplication实例中, 已经分析了当前模块web类型为SERVLET, 所以当前实例化了一个AnnotationConfigServletWebServerApplicationContext对象
public class SpringApplication {
public ConfigurableApplicationContext run(String... args) {
StopWatch stopWatch = new StopWatch();
stopWatch.start();
//应用上下文
ConfigurableApplicationContext context = null;
Collection exceptionReporters = new ArrayList<>();
configureHeadlessProperty();
SpringApplicationRunListeners listeners = getRunListeners(args);
listeners.starting();
try {
ApplicationArguments applicationArguments = new DefaultApplicationArguments(
args);
ConfigurableEnvironment environment = prepareEnvironment(listeners,
applicationArguments);
configureIgnoreBeanInfo(environment);
Banner printedBanner = printBanner(environment);
//本文的重点
//创建应用上下文
//AnnotationConfigServletWebServerApplicationContext
context = createApplicationContext();
//...
}
public static final String DEFAULT_SERVLET_WEB_CONTEXT_CLASS = "org.springframework.boot."
+ "web.servlet.context.AnnotationConfigServletWebServerApplicationContext";
//创建应用上下文
protected ConfigurableApplicationContext createApplicationContext() {
Class contextClass = this.applicationContextClass;
if (contextClass == null) {
try {
switch (this.webApplicationType) {
case SERVLET:
//我们使用的是servlet web环境
//实例化AnnotationConfigServletWebServerApplicationContext对象
contextClass = Class.forName(DEFAULT_SERVLET_WEB_CONTEXT_CLASS);
break;
case REACTIVE:
contextClass = Class.forName(DEFAULT_REACTIVE_WEB_CONTEXT_CLASS);
break;
default:
contextClass = Class.forName(DEFAULT_CONTEXT_CLASS);
}
}
catch (ClassNotFoundException ex) {
throw new IllegalStateException(
"Unable create a default ApplicationContext, "
+ "please specify an ApplicationContextClass",
ex);
}
}
//返回的是一个AnnotationConfigServletWebServerApplicationContext对象
return (ConfigurableApplicationContext) BeanUtils.instantiateClass(contextClass);
}
}
由上面类图,我们可以看出, 类继承关系如下:
- AnnotationConfigServletWebServerApplicationContext类继承了ServletWebServerApplicationContext
- ServletWebServerApplicationContext继承了GenericWebApplicationContext
- GenericWebApplicationContext继承了GenericApplicationContext
- GenericApplicationContext继承了AbstractApplicationContext
- AbstractApplicationContext继承了DefaultResourceLoader
2. DefaultResourceLoader
用来加载Resource, 初始化的过程中, 实例化了ClassLoader,
//默认的资源加载器
public class DefaultResourceLoader implements ResourceLoader {
@Nullable
private ClassLoader classLoader;
//自定义ProtocolResolver, 用于获取资源
private final Set protocolResolvers = new LinkedHashSet<>(4);
//
private final Map, Map> resourceCaches = new ConcurrentHashMap<>(4);
//实例化ClassLoader
public DefaultResourceLoader() {
this.classLoader = ClassUtils.getDefaultClassLoader();
}
//加载资源
@Override
public Resource getResource(String location) {
Assert.notNull(location, "Location must not be null");
//自定义资源加载方式
for (ProtocolResolver protocolResolver : this.protocolResolvers) {
//调用ProtocolResolver的resolve方法
Resource resource = protocolResolver.resolve(location, this);
if (resource != null) {
//如果获取到资源,立即返回
return resource;
}
}
if (location.startsWith("/")) {
//先判断是否是根目录
return getResourceByPath(location);
}
else if (location.startsWith(CLASSPATH_URL_PREFIX)) {
//再判断是否是classpath下的资源
return new ClassPathResource(location.substring(CLASSPATH_URL_PREFIX.length()), getClassLoader());
}
else {
try {
//先当做一个URL处理
URL url = new URL(location);
//先判断是否是一个file
//不是file的话,再从URL中获取
return (ResourceUtils.isFileURL(url) ? new FileUrlResource(url) : new UrlResource(url));
}
catch (MalformedURLException ex) {
//获取不到资源的话
//当做resource处理
return getResourceByPath(location);
}
}
}
}
3. AbstractApplicationContext
抽象ApplicationContext, 定义了ApplicationContext一些模板方法, 在实例化的过程中, 调用了getResourcePatternResolver()方法, 构造了一个PathMatchingResourcePatternResolver, 规定了如何查找资源, 例如从classpath, 根路径, 从war包等查找资源
public abstract class AbstractApplicationContext extends DefaultResourceLoader
implements ConfigurableApplicationContext {
private ResourcePatternResolver resourcePatternResolver;
//初始化一个resourcePatternResolver
public AbstractApplicationContext() {
this.resourcePatternResolver = getResourcePatternResolver();
}
//该方法被GenericWebApplicationContext类重写
//实际调用的是GenericWebApplicationContext的getResourcePatternResolver()方法
protected ResourcePatternResolver getResourcePatternResolver() {
//this实现了DefaultResourceLoader
//可以作为PathMatchingResourcePatternResolver构造函数的参数
return new PathMatchingResourcePatternResolver(this);
}
}
4. GenericApplicationContext
初始化了一个DefaultListableBeanFactory
public class GenericApplicationContext extends AbstractApplicationContext implements BeanDefinitionRegistry {
private final DefaultListableBeanFactory beanFactory;
//初始化beanFactory
public GenericApplicationContext() {
this.beanFactory = new DefaultListableBeanFactory();
}
}
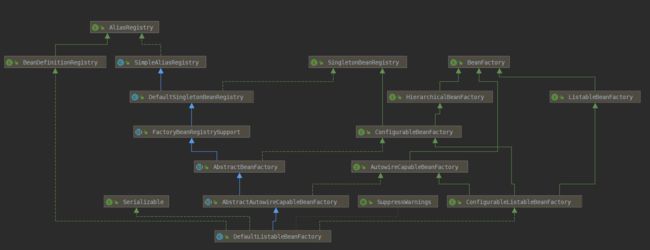
由上面类图, 我们可以看出DefaultListableBeanFactory的继承关系:
- DefaultListableBeanFactory继承了AbstractAutowireCapableBeanFactory
- AbstractAutowireCapableBeanFactory继承了AbstractBeanFactory
- AbstractBeanFactory继承了FactoryBeanRegistrySupport
- FactoryBeanRegistrySupport继承了DefaultSingletonBeanRegistry
- DefaultSingletonBeanRegistry继承了SimpleAliasRegistry
4.1 SimpleAliasRegistry
提供了bean别名的增删改查功能
public class SimpleAliasRegistry implements AliasRegistry {
//key是bean别名
//value是原始bean名称
private final Map aliasMap = new ConcurrentHashMap<>(16);
}
4.2 DefaultSingletonBeanRegistry
默认的单例Bean注册器, 提供了单例bean增删改查等功能
public class DefaultSingletonBeanRegistry extends SimpleAliasRegistry implements SingletonBeanRegistry {
//缓存单例bean, key为bean名称,value为bean实例
private final Map singletonObjects = new ConcurrentHashMap<>(256);
//缓存beanFactory, key为bean名称,value为beanFactory
private final Map> singletonFactories = new HashMap<>(16);
//早期单例缓存, key为bean名称,value为bean实例
private final Map earlySingletonObjects = new HashMap<>(16);
//单例bean名称set
private final Set registeredSingletons = new LinkedHashSet<>(256);
//正在创建的单例bean名称set
private final Set singletonsCurrentlyInCreation =
Collections.newSetFromMap(new ConcurrentHashMap<>(16));
//当前在创建检查中排除的bean名称set
private final Set inCreationCheckExclusions =
Collections.newSetFromMap(new ConcurrentHashMap<>(16));
//异常set
@Nullable
private Set suppressedExceptions;
//正在销毁的bean名称set
private boolean singletonsCurrentlyInDestruction = false;
//一次性的bean实例
private final Map disposableBeans = new LinkedHashMap<>();
//bean包含关系map, key为bean名称, value为被包含的bean名称
private final Map> containedBeanMap = new ConcurrentHashMap<>(16);
//bean依赖关系缓存, key为bean名称,value为依赖该bean的bean名称
private final Map> dependentBeanMap = new ConcurrentHashMap<>(64);
//bean依赖关系缓存,key为bean名称,value为该bean依赖的bean名称
private final Map> dependenciesForBeanMap = new ConcurrentHashMap<>(64);
}
4.3 FactoryBeanRegistrySupport
提供了FactoryBean的增删改查方法
public abstract class FactoryBeanRegistrySupport extends DefaultSingletonBeanRegistry {
//缓存FactoryBean单例的Map
private final Map factoryBeanObjectCache = new ConcurrentHashMap<>(16);
}
4.4 AbstractBeanFactory
抽象BeanFactory, 定义了通用的beanFactory的模板方法, 添加了对beanFactory对Scope的支持, scope主要有五种, singleton, prototype, request, session和application,
- ConfigurableBeanFactory定义两个SCOPE
- SCOPE_SINGLETON = "singleton"
- SCOPE_PROTOTYPE = "prototype"
- WebApplicationContext接口中定义了三个SCOPE
- SCOPE_REQUEST = "request"
- SCOPE_SESSION = "session"
- SCOPE_APPLICATION = "application"
public abstract class AbstractBeanFactory extends FactoryBeanRegistrySupport implements ConfigurableBeanFactory {
//自定义PropertyEditorRegistrar属性编辑器注册器
//用于编辑Factory下的所有bean
private final Set propertyEditorRegistrars = new LinkedHashSet<>(4);
//自定义PropertyEditor属性编辑器
private final Map, Class> customEditors = new HashMap<>(4);
//String值解析器
private final List embeddedValueResolvers = new CopyOnWriteArrayList<>();
//Bean创建处理器
private final List beanPostProcessors = new CopyOnWriteArrayList<>();
//Bean Scope范围支持
//父接口ConfigurableBeanFactory中定义了两个SCOPE
//SCOPE_SINGLETON = "singleton";
//SCOPE_PROTOTYPE = "prototype";
//WebApplicationContext接口中定义了三个SCOPE
//SCOPE_REQUEST = "request";
//SCOPE_SESSION = "session";
//SCOPE_APPLICATION = "application"
private final Map scopes = new LinkedHashMap<>(8);
}
4.5 AbstractAutowireCapableBeanFactory
抽象自动配置BeanFactory, 实现了创建Bean, 实例化Bean, 字段配置Bean, 自动装配依赖Bean的方法
public abstract class AbstractAutowireCapableBeanFactory extends AbstractBeanFactory
implements AutowireCapableBeanFactory {
//创建Bean策略,默认为cglib
private InstantiationStrategy instantiationStrategy = new CglibSubclassingInstantiationStrategy();
public AbstractAutowireCapableBeanFactory() {
//显示调用父类方法
super();
//自动装配忽略BeanNameAware接口
ignoreDependencyInterface(BeanNameAware.class);
//自动装配忽略BeanFactoryAware接口
ignoreDependencyInterface(BeanFactoryAware.class);
//自动装配忽略BeanClassLoaderAware接口
ignoreDependencyInterface(BeanClassLoaderAware.class);
}
}
4.6 DefaultListableBeanFactory
BeanFactory默认实现, spring IOC默认容器类
public class DefaultListableBeanFactory extends AbstractAutowireCapableBeanFactory
implements ConfigurableListableBeanFactory, BeanDefinitionRegistry, Serializable {
//key为依赖类型, value为自动配置的值
private final Map, Object> resolvableDependencies = new ConcurrentHashMap<>(16);
//key为bean名称, value为bean定义
private final Map beanDefinitionMap = new ConcurrentHashMap<>(256);
//key为依赖的类型, value为所有bean名称列表
private final Map, String[]> allBeanNamesByType = new ConcurrentHashMap<>(64);
//key为依赖类型, value为单例bean的名称数组
private final Map, String[]> singletonBeanNamesByType = new ConcurrentHashMap<>(64);
//按注册的顺序, 记录的bean名称列表
private volatile List beanDefinitionNames = new ArrayList<>(256);
//手动添加的bean名称列表
private volatile Set manualSingletonNames = new LinkedHashSet<>(16);
public DefaultListableBeanFactory() {
//显示调用父类构造函数
super();
}
}
5. GenericWebApplicationContext
重写了AbstractApplicationContext的getResourcePatternResolver()方法, 返回一个ServletContextResourcePatternResolver对象, 构造函数中显示调用父类GenericApplicationContext的构造函数
public class GenericWebApplicationContext extends GenericApplicationContext
implements ConfigurableWebApplicationContext, ThemeSource {
//显式调用父GenericApplicationContext类构造方法
//什么都不做
public GenericWebApplicationContext() {
super();
}
//ServletContextResourcePatternResolver
//重写了父类获取资源的逻辑
//从ServletContext中获取资源
@Override
protected ResourcePatternResolver getResourcePatternResolver() {
return new ServletContextResourcePatternResolver(this);
}
}
6. ServletWebServerApplicationContext
隐式调用父类GenericWebApplicationContext构造函数, 什么都没有做
public class ServletWebServerApplicationContext extends GenericWebApplicationContext
implements ConfigurableWebServerApplicationContext {
//什么都不做
//隐式调用父类GenericWebApplicationContext构造方法
public ServletWebServerApplicationContext() {
}
}
7.AnnotationConfigServletWebServerApplicationContext
首先, 初始化一个AnnotatedBeanDefinitionReader, 然后再实例化一个ClassPathBeanDefinitionScanner对象
//注解配置ServletWeb服务应用上下文
//当前SpringApplication上下文
public class AnnotationConfigServletWebServerApplicationContext
extends ServletWebServerApplicationContext implements AnnotationConfigRegistry {
//注解Bean读取器,用来去取bean
private final AnnotatedBeanDefinitionReader reader;
//ClassPath中的Bean扫描器,用来扫描bean
private final ClassPathBeanDefinitionScanner scanner;
//被注册到容器中的Class对象列表
private final Set> annotatedClasses = new LinkedHashSet<>();
//需要扫描的包
private String[] basePackages;
//构造函数
public AnnotationConfigServletWebServerApplicationContext() {
//注解Bean读取器 传入this
this.reader = new AnnotatedBeanDefinitionReader(this);
//Classpath中的Bean扫描器
this.scanner = new ClassPathBeanDefinitionScanner(this);
}
}
7.1 AnnotatedBeanDefinitionReader();
用于读取和解析bean定义
//注解Bean读取器
//用来读取和解析bean
public class AnnotatedBeanDefinitionReader {
private final BeanDefinitionRegistry registry;
//bean名称生成器
private BeanNameGenerator beanNameGenerator = new AnnotationBeanNameGenerator();
//Scope解析器
private ScopeMetadataResolver scopeMetadataResolver = new AnnotationScopeMetadataResolver();
private ConditionEvaluator conditionEvaluator;
//registry传入的AnnotationConfigServletWebServerApplicationContext对象
public AnnotatedBeanDefinitionReader(BeanDefinitionRegistry registry) {
this(registry, getOrCreateEnvironment(registry));
}
//构造函数
//传入的registry就是AnnotationConfigServletWebServerApplicationContext实例
public AnnotatedBeanDefinitionReader(BeanDefinitionRegistry registry, Environment environment) {
Assert.notNull(registry, "BeanDefinitionRegistry must not be null");
Assert.notNull(environment, "Environment must not be null");
this.registry = registry;
//条件评估器的初始化
this.conditionEvaluator = new ConditionEvaluator(registry, environment, null);
//注解配置注册器
AnnotationConfigUtils.registerAnnotationConfigProcessors(this.registry);
}
//获取或创建环境
private static Environment getOrCreateEnvironment(BeanDefinitionRegistry registry) {
Assert.notNull(registry, "BeanDefinitionRegistry must not be null");
if (registry instanceof EnvironmentCapable) {
//实现了EnvironmentCapable
//调用了AbstractApplicationContext中的getEnvironment方法
//获取到了StandardEnvironment实例
return ((EnvironmentCapable) registry).getEnvironment();
}
return new StandardEnvironment();
}
}
AnnotationBeanNameGenerator
注解bean名称生成器, 用于生成Bean名称
//bean名称生成器
//主要为Component注解生成名称
//包括Component子注解: Component,Respository,Service,Controller
//还包括java6的ManagedBean
public class AnnotationBeanNameGenerator implements BeanNameGenerator {
//Spring Component注解
private static final String COMPONENT_ANNOTATION_CLASSNAME = "org.springframework.stereotype.Component";
@Override
public String generateBeanName(BeanDefinition definition, BeanDefinitionRegistry registry) {
//如果是注解的bean定义
if (definition instanceof AnnotatedBeanDefinition) {
//先从注解获取bean名称
String beanName = determineBeanNameFromAnnotation((AnnotatedBeanDefinition) definition);
//不为空直接返回
if (StringUtils.hasText(beanName)) {
return beanName;
}
}
//注解没有定义bean名称,那么构造一个bean名称
return buildDefaultBeanName(definition, registry);
}
/**
* 构造bean名称
*/
protected String buildDefaultBeanName(BeanDefinition definition) {
String beanClassName = definition.getBeanClassName();
Assert.state(beanClassName != null, "No bean class name set");
//获取Class名称
String shortClassName = ClassUtils.getShortName(beanClassName);
//将类名作为bean名称
return Introspector.decapitalize(shortClassName);
}
}
public class Introspector {
public static String decapitalize(String name) {
if (name == null || name.length() == 0) {
return name;
}
if (name.length() > 1 && Character.isUpperCase(name.charAt(1)) &&
Character.isUpperCase(name.charAt(0))){
//如果第一个和第二个字符都是大写,直接返回名称
return name;
}
//将第一个字符转换为小写,返回类名
char chars[] = name.toCharArray();
chars[0] = Character.toLowerCase(chars[0]);
return new String(chars);
}
}
AnnotationScopeMetadataResolver
Scope注解的解析器, 解析出Scope的模式ScopedProxyMode, 以及Scope的名称
//@Scope注解的解析器
public class AnnotationScopeMetadataResolver implements ScopeMetadataResolver {
//Scope注解
protected Class scopeAnnotationType = Scope.class;
//代理模式
private final ScopedProxyMode defaultProxyMode;
//默认不使用代理
public AnnotationScopeMetadataResolver() {
this.defaultProxyMode = ScopedProxyMode.NO;
}
//解析@Scope注解
public ScopeMetadata resolveScopeMetadata(BeanDefinition definition) {
ScopeMetadata metadata = new ScopeMetadata();
if (definition instanceof AnnotatedBeanDefinition) {
//注解bean
AnnotatedBeanDefinition annDef = (AnnotatedBeanDefinition)definition;
//获取到metadata放入名为attributes的map中
AnnotationAttributes attributes = AnnotationConfigUtils.attributesFor(annDef.getMetadata(), this.scopeAnnotationType);
if (attributes != null) {
//将@Scope注解的value和proxyMode放入到metadata中
metadata.setScopeName(attributes.getString("value"));
ScopedProxyMode proxyMode = (ScopedProxyMode)attributes.getEnum("proxyMode");
if (proxyMode == ScopedProxyMode.DEFAULT) {
proxyMode = this.defaultProxyMode;
}
metadata.setScopedProxyMode(proxyMode);
}
}
return metadata;
}
}
//代理模式枚举
public enum ScopedProxyMode {
//默认代理模式,默认使NO,
//如果在component-scan中配置了默认值,将会使用这个默认值
DEFAULT,
//不使用代理
NO,
//接口,使用jdk动态代理
INTERFACES,
//类,使用cglib动态代理
TARGET_CLASS;
}
ConditionEvaluator
@Conditional注解的条件评估器, 评估是否满足条件
//@Conditional注解的解析器
//也可用于@ConditionalOnBean,@ConditionalOnClass,@ConditionalOnExpression,@ConditionalOnMissingBean等子注解
class ConditionEvaluator {
//内部类
private final ConditionContextImpl context;
//构造函数
public ConditionEvaluator(@Nullable BeanDefinitionRegistry registry,
@Nullable Environment environment, @Nullable ResourceLoader resourceLoader) {
this.context = new ConditionContextImpl(registry, environment, resourceLoader);
}
//判断是否应该跳过
public boolean shouldSkip(@Nullable AnnotatedTypeMetadata metadata, @Nullable ConfigurationPhase phase) {
if (metadata == null || !metadata.isAnnotated(Conditional.class.getName())) {
//metadata为空或者没有使用@Conditional注解,不跳过
return false;
}
if (phase == null) {
//ConfigurationPhase对象为空,也就是没有设置生效条件
if (metadata instanceof AnnotationMetadata &&
ConfigurationClassUtils.isConfigurationCandidate((AnnotationMetadata) metadata)) {
//如果是注解的话,使用PARSE_CONFIGURATION配置验证是否跳过
return shouldSkip(metadata, ConfigurationPhase.PARSE_CONFIGURATION);
}
//不是注解的话,使用REGISTER_BEAN配置验证是否跳过
return shouldSkip(metadata, ConfigurationPhase.REGISTER_BEAN);
}
List conditions = new ArrayList<>();
//遍历配置类的条件注解,得到条件数据,放到conditions集合中
for (String[] conditionClasses : getConditionClasses(metadata)) {
for (String conditionClass : conditionClasses) {
Condition condition = getCondition(conditionClass, this.context.getClassLoader());
conditions.add(condition);
}
}
//按Order注解排序
AnnotationAwareOrderComparator.sort(conditions);
//条件排序
for (Condition condition : conditions) {
ConfigurationPhase requiredPhase = null;
if (condition instanceof ConfigurationCondition) {
requiredPhase = ((ConfigurationCondition) condition).getConfigurationPhase();
}
//添加验证满足,那就跳过
if ((requiredPhase == null || requiredPhase == phase) && !condition.matches(this.context, metadata)) {
return true;
}
}
return false;
}
//私有静态内部类
private static class ConditionContextImpl implements ConditionContext {
public ConditionContextImpl(@Nullable BeanDefinitionRegistry registry,
@Nullable Environment environment, @Nullable ResourceLoader resourceLoader) {
//传入的是AnnotationConfigServletWebServerApplicationContext对象
this.registry = registry;
this.beanFactory = deduceBeanFactory(registry);
this.environment = (environment != null ? environment : deduceEnvironment(registry));
this.resourceLoader = (resourceLoader != null ? resourceLoader : deduceResourceLoader(registry));
this.classLoader = deduceClassLoader(resourceLoader, this.beanFactory);
}
//推断获取beanFactory
@Nullable
private ConfigurableListableBeanFactory deduceBeanFactory(@Nullable BeanDefinitionRegistry source) {
if (source instanceof ConfigurableListableBeanFactory) {
return (ConfigurableListableBeanFactory) source;
}
if (source instanceof ConfigurableApplicationContext) {
//AnnotationConfigServletWebServerApplicationContext实现了ConfigurableApplicationContext
//调用了GenericApplicationContext中的getBeanFactory方法
//获取到一个DefaultListableBeanFactory对象
return (((ConfigurableApplicationContext) source).getBeanFactory());
}
return null;
}
//获取环境
private Environment deduceEnvironment(@Nullable BeanDefinitionRegistry source) {
if (source instanceof EnvironmentCapable) {
//AnnotationConfigServletWebServerApplicationContex实现了EnvironmentCapable
//调用了AbstractApplicationContext中的getEnvironment方法
//获取到了StandardEnvironment实例
return ((EnvironmentCapable) source).getEnvironment();
}
return new StandardEnvironment();
}
//获取ResourceLoader
private ResourceLoader deduceResourceLoader(@Nullable BeanDefinitionRegistry source) {
if (source instanceof ResourceLoader) {
//AnnotationConfigServletWebServerApplicationContex实现了ResourceLoader
//所以直接强转
return (ResourceLoader) source;
}
return new DefaultResourceLoader();
}
//获取ClassLoader
@Nullable
private ClassLoader deduceClassLoader(@Nullable ResourceLoader resourceLoader,
@Nullable ConfigurableListableBeanFactory beanFactory) {
//传入ResourceLoader为null
if (resourceLoader != null) {
ClassLoader classLoader = resourceLoader.getClassLoader();
if (classLoader != null) {
return classLoader;
}
}
if (beanFactory != null) {
//获取到beanFactory不为null
//使用beanFactory中的classloader
return beanFactory.getBeanClassLoader();
}
return ClassUtils.getDefaultClassLoader();
}
}
AnnotationConfigUtils.registerAnnotationConfigProcessors(this.registry)
注册bean处理器, bean名称需要加上org.springframework.context.annotation前缀:
- beanFactory设置AnnotationAwareOrderComparator
- 处理Ordered类、@Order注解和@Priority
- 处理完成之后排序
- beanFactory设置ContextAnnotationAutowireCandidateResolver
- 处理@Lazy注解
- 配置延迟加载
- 注册ConfigurationClassPostProcessor类型的bean
- 处理@Configuration注解
- 非常重要的一个类
- bean名称为internalConfigurationAnnotationProcessor
- 注册AutowiredAnnotationBeanPostProcessor类型的bean
- 处理@Autowired, @Value注解
- bean名称为internalAutowiredAnnotationProcessor
- 注册CommonAnnotationBeanPostProcessor类型的bean
- 处理@PostConstruct,@PreDestroy注解
- bean名称为internalCommonAnnotationProcessor
- 注册EventListenerMethodProcessor类型的bean
- 处理@EventListener注解
- bean名称为internalEventListenerProcessor
- 注册DefaultEventListenerFactory类型的bean
- 默认的监听器工厂
- 处理@EventListener注解
- bean名称为internalEventListenerFactory
public static Set registerAnnotationConfigProcessors(
BeanDefinitionRegistry registry, @Nullable Object source) {
//获取到父类GenericApplicationContext的beanFactory
DefaultListableBeanFactory beanFactory = unwrapDefaultListableBeanFactory(registry);
if (beanFactory != null) {
//添加注解排序器
if (!(beanFactory.getDependencyComparator() instanceof AnnotationAwareOrderComparator)) {
//AnnotationAwareOrderComparator处理Ordered类、@Order注解和@Priority
beanFactory.setDependencyComparator(AnnotationAwareOrderComparator.INSTANCE);
}
//处理@Lazy注解
if (!(beanFactory.getAutowireCandidateResolver() instanceof ContextAnnotationAutowireCandidateResolver)) {
beanFactory.setAutowireCandidateResolver(new ContextAnnotationAutowireCandidateResolver());
}
}
Set beanDefs = new LinkedHashSet<>(8);
if (!registry.containsBeanDefinition(CONFIGURATION_ANNOTATION_PROCESSOR_BEAN_NAME)) {
//注册ConfigurationClassPostProcessor类型的bean
//处理@Configuration注解
RootBeanDefinition def = new RootBeanDefinition(ConfigurationClassPostProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, CONFIGURATION_ANNOTATION_PROCESSOR_BEAN_NAME));
}
if (!registry.containsBeanDefinition(AUTOWIRED_ANNOTATION_PROCESSOR_BEAN_NAME)) {
//注册类型AutowiredAnnotationBeanPostProcessor的bean
//处理@Autowired,@Value注解
RootBeanDefinition def = new RootBeanDefinition(AutowiredAnnotationBeanPostProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, AUTOWIRED_ANNOTATION_PROCESSOR_BEAN_NAME));
}
if (jsr250Present && !registry.containsBeanDefinition(COMMON_ANNOTATION_PROCESSOR_BEAN_NAME)) {
//注册类型CommonAnnotationBeanPostProcessor的bean
//处理@PostConstruct和@PreDestroy注解
RootBeanDefinition def = new RootBeanDefinition(CommonAnnotationBeanPostProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, COMMON_ANNOTATION_PROCESSOR_BEAN_NAME));
}
if (jpaPresent && !registry.containsBeanDefinition(PERSISTENCE_ANNOTATION_PROCESSOR_BEAN_NAME)) {
//注册一个名称为PersistenceAnnotationBeanPostProcessor,类型为PersistenceAnnotationBeanPostProcessor的bean
//处理@PersistenceUnit,@PersistenceContext注解
RootBeanDefinition def = new RootBeanDefinition();
try {
//我们没有用到PersistenceAnnotationBeanPostProcessor
//所以不会添加
def.setBeanClass(ClassUtils.forName(PERSISTENCE_ANNOTATION_PROCESSOR_CLASS_NAME,
AnnotationConfigUtils.class.getClassLoader()));
}
catch (ClassNotFoundException ex) {
throw new IllegalStateException(
"Cannot load optional framework class: " + PERSISTENCE_ANNOTATION_PROCESSOR_CLASS_NAME, ex);
}
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, PERSISTENCE_ANNOTATION_PROCESSOR_BEAN_NAME));
}
if (!registry.containsBeanDefinition(EVENT_LISTENER_PROCESSOR_BEAN_NAME)) {
//注册类型为EventListenerMethodProcessor的bean
//处理@EventListener注解
RootBeanDefinition def = new RootBeanDefinition(EventListenerMethodProcessor.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, EVENT_LISTENER_PROCESSOR_BEAN_NAME));
}
if (!registry.containsBeanDefinition(EVENT_LISTENER_FACTORY_BEAN_NAME)) {
//注册类型为DefaultEventListenerFactory的bean
//EventListenerFactory的默认实现,支持EventListener注解
RootBeanDefinition def = new RootBeanDefinition(DefaultEventListenerFactory.class);
def.setSource(source);
beanDefs.add(registerPostProcessor(registry, def, EVENT_LISTENER_FACTORY_BEAN_NAME));
}
return beanDefs;
}
7.2 ClassPathBeanDefinitionScanner
如果basePackages不为空的话, 扫描basePackages中定义的bean, 当前应用中没有配置basePackages, 所以ClassPathBeanDefinitionScanner不会去扫描bean
//ClassPath中Bean扫描器
public class ClassPathBeanDefinitionScanner extends ClassPathScanningCandidateComponentProvider {
private final BeanDefinitionRegistry registry;
private BeanDefinitionDefaults beanDefinitionDefaults = new BeanDefinitionDefaults();
@Nullable
private String[] autowireCandidatePatterns;
private BeanNameGenerator beanNameGenerator = new AnnotationBeanNameGenerator();
private ScopeMetadataResolver scopeMetadataResolver = new AnnotationScopeMetadataResolver();
private boolean includeAnnotationConfig = true;
// registry是AnnotationConfigServletWebServerApplicationContext的实例
//useDefaultFilters默认为true
public ClassPathBeanDefinitionScanner(BeanDefinitionRegistry registry, boolean useDefaultFilters,
Environment environment, @Nullable ResourceLoader resourceLoader) {
Assert.notNull(registry, "BeanDefinitionRegistry must not be null");
this.registry = registry;
if (useDefaultFilters) {
//添加@Component,@ManagedBean,@Named注解扫描
registerDefaultFilters();
}
//getOrCreateEnvironment(registry)
//得到一个StandardEnvironment
setEnvironment(environment);
// (registry instanceof ResourceLoader ? (ResourceLoader) registry : null
//registry实现了ResourceLoader,registry强转为ResourceLoader
setResourceLoader(resourceLoader);
}
@SuppressWarnings("unchecked")
protected void registerDefaultFilters() {
//添加一个Component注解扫描
//Compent注解包括子注解:Repository,Service,Controller
this.includeFilters.add(new AnnotationTypeFilter(Component.class));
ClassLoader cl = ClassPathScanningCandidateComponentProvider.class.getClassLoader();
try {
//添加ManagedBean注解
this.includeFilters.add(new AnnotationTypeFilter(
((Class) ClassUtils.forName("javax.annotation.ManagedBean", cl)), false));
logger.trace("JSR-250 'javax.annotation.ManagedBean' found and supported for component scanning");
}
catch (ClassNotFoundException ex) {
}
try {
//添加Named注解
this.includeFilters.add(new AnnotationTypeFilter(
((Class) ClassUtils.forName("javax.inject.Named", cl)), false));
logger.trace("JSR-330 'javax.inject.Named' annotation found and supported for component scanning");
}
catch (ClassNotFoundException ex) {
}
}
//扫描basePackages下使用注解的类
public int scan(String... basePackages) {
//DefaultListableBeanFactory#getBeanDefinitionCount()
//获取beanDefinitionMap元素的数量
//beanDefinitionMap = new ConcurrentHashMap<>(256);
int beanCountAtScanStart = this.registry.getBeanDefinitionCount();
//扫描方法
doScan(basePackages);
//默认为true
if (this.includeAnnotationConfig) {
//该方法实例化reader的时候已经执行过一次了
AnnotationConfigUtils.registerAnnotationConfigProcessors(this.registry);
}
//返回实际扫描的数量
return (this.registry.getBeanDefinitionCount() - beanCountAtScanStart);
}
//扫描方法
protected Set doScan(String... basePackages) {
Assert.notEmpty(basePackages, "At least one base package must be specified");
Set beanDefinitions = new LinkedHashSet<>();
for (String basePackage : basePackages) {
//扫描到Compent注解的bean列表
Set candidates = findCandidateComponents(basePackage);
for (BeanDefinition candidate : candidates) {
//获取Scope注解的值
ScopeMetadata scopeMetadata = this.scopeMetadataResolver.resolveScopeMetadata(candidate);
candidate.setScope(scopeMetadata.getScopeName());
//生成bean名称
String beanName = this.beanNameGenerator.generateBeanName(candidate, this.registry);
if (candidate instanceof AbstractBeanDefinition) {
//bean默认配置
postProcessBeanDefinition((AbstractBeanDefinition) candidate, beanName);
}
if (candidate instanceof AnnotatedBeanDefinition) {
//配置bean
AnnotationConfigUtils.processCommonDefinitionAnnotations((AnnotatedBeanDefinition) candidate);
}
if (checkCandidate(beanName, candidate)) {
BeanDefinitionHolder definitionHolder = new BeanDefinitionHolder(candidate, beanName);
definitionHolder =
AnnotationConfigUtils.applyScopedProxyMode(scopeMetadata, definitionHolder, this.registry);
beanDefinitions.add(definitionHolder);
//注册bean
registerBeanDefinition(definitionHolder, this.registry);
}
}
}
return beanDefinitions;
}
}
8. 总结
实例化AnnotationConfigServletWebServerApplicationContext过程中, 会先调用父类GenericApplicationContext构造函数, 实例化了一个DefaultListableBeanFactory, 作为Spring IOC容器, AnnotationConfigServletWebServerApplicationContext的构造函数实例化了AnnotatedBeanDefinitionReader对象, 用于读取Spring的bean定义, 在实例化AnnotatedBeanDefinitionReader的过程中, 注册了几个bean, 用来处理相应的注解
下一篇
我们将会在下一篇SpringBootExceptionReporter异常上报, 继续阅读springboot源码