package org.nd4j.examples;
import org.nd4j.linalg.api.ndarray.INDArray;
import org.nd4j.linalg.factory.Nd4j;
import java.util.Arrays;
/**
* --- Nd4j 示例 2: 创建 INDArrays ---
*
*
* 在这个例子中,我们将看到许多不同的方法来创建INDArrays
*
* @author Alex Black
*/
public class Nd4jEx2_CreatingINDArrays {
public static void main(String[] args){
//在这里,我们将看到如何创建具有不同标量值初始化的INDArrays。
int nRows = 3;
int nColumns = 5;
//3行5列,0填充
INDArray allZeros = Nd4j.zeros(nRows, nColumns);
System.out.println("Nd4j.zeros(nRows, nColumns)");
System.out.println(allZeros);
//3行5列,1填充
INDArray allOnes = Nd4j.ones(nRows, nColumns);
System.out.println("\nNd4j.ones(nRows, nColumns)");
System.out.println(allOnes);
//3行5列,10填充
INDArray allTens = Nd4j.valueArrayOf(nRows, nColumns, 10.0);
System.out.println("\nNd4j.valueArrayOf(nRows, nColumns, 10.0)");
System.out.println(allTens);
//我们也可以从double[] 和 double[][]创建INDArrays(或,float/int等java数组)
double[] vectorDouble = new double[]{1,2,3};
INDArray rowVector = Nd4j.create(vectorDouble);
System.out.println("rowVector: " + rowVector);
//1 行, 3 列
System.out.println("rowVector.shape(): " + Arrays.toString(rowVector.shape()));
//手动指定: 3 行, 1 列
INDArray columnVector = Nd4j.create(vectorDouble, new int[]{3,1});
//注意打印: 行/列 向量被打印成一行
System.out.println("columnVector: " + columnVector);
//3 行, 1 列
System.out.println("columnVector.shape(): " + Arrays.toString(columnVector.shape()));
double[][] matrixDouble = new double[][]{
{1.0, 2.0, 3.0},
{4.0, 5.0, 6.0}};
INDArray matrix = Nd4j.create(matrixDouble);
System.out.println("\n 从 double[][]定义的INDArray:");
System.out.println(matrix);
//也可以创建随机INDArrays
//但是请注意,默认情况下,使用INDArray.toString()以截断精度打印随机值
int[] shape = new int[]{nRows, nColumns};
INDArray uniformRandom = Nd4j.rand(shape);
System.out.println("\n\n\n 统一随机数组:");
System.out.println(uniformRandom);
System.out.println("位置(0,0)处全精度随机值: " + uniformRandom.getDouble(0,0));
INDArray gaussianMeanZeroUnitVariance = Nd4j.randn(shape);
System.out.println("\n N(0,1)随机数组:");
System.out.println(gaussianMeanZeroUnitVariance);
//我们可以使用随机种子让它重复:
long rngSeed = 12345;
INDArray uniformRandom2 = Nd4j.rand(shape, rngSeed);
INDArray uniformRandom3 = Nd4j.rand(shape, rngSeed);
System.out.println("\n 带有一些固定种子的统一随机数组:");
System.out.println(uniformRandom2);
System.out.println();
System.out.println(uniformRandom3);
//当危急们不限于二维,三维或更高维也很简单
//3x4x5 INDArray
INDArray threeDimArray = Nd4j.ones(3,4,5);
//3x4x5x6 INDArray
INDArray fourDimArray = Nd4j.ones(3,4,5,6);
//3x4x5x6x7 INDArray
INDArray fiveDimArray = Nd4j.ones(3,4,5,6,7);
System.out.println("\n\n\n 创建更多维数的INDArrays:");
System.out.println("三维数组形状: " + Arrays.toString(threeDimArray.shape()));
System.out.println("四维数组形状: " + Arrays.toString(fourDimArray.shape()));
System.out.println("五维数组形状: " + Arrays.toString(fiveDimArray.shape()));
//我们也可以结合其它INDArrays创建INDArrays
INDArray rowVector1 = Nd4j.create(new double[]{1,2,3});
INDArray rowVector2 = Nd4j.create(new double[]{4,5,6});
//垂直堆叠:
INDArray vStack = Nd4j.vstack(rowVector1, rowVector2);
//水平堆叠
INDArray hStack = Nd4j.hstack(rowVector1, rowVector2);
System.out.println("\n\n\n 从其它INDArrays创建INDArrays , 使用 hstack 或 vstack:");
System.out.println("vStack:\n" + vStack);
System.out.println("hStack:\n" + hStack);
//还有一些其他的方法:
INDArray identityMatrix = Nd4j.eye(3);
System.out.println("\n\n\nNd4j.eye(3):\n" + identityMatrix);
//值为 1 到 10,分为10步
INDArray linspace = Nd4j.linspace(1,10,10);
System.out.println("Nd4j.linspace(1,10,10):\n" + linspace);
//创建正方形矩阵,把rowvector2作为对角线
INDArray diagMatrix = Nd4j.diag(rowVector2);
System.out.println("Nd4j.diag(rowVector2):\n" + diagMatrix);
}
}
运行结果
Nd4j.zeros(nRows, nColumns)
[[ 0, 0, 0, 0, 0],
[ 0, 0, 0, 0, 0],
[ 0, 0, 0, 0, 0]]
Nd4j.ones(nRows, nColumns)
[[ 1.0000, 1.0000, 1.0000, 1.0000, 1.0000],
[ 1.0000, 1.0000, 1.0000, 1.0000, 1.0000],
[ 1.0000, 1.0000, 1.0000, 1.0000, 1.0000]]
Nd4j.valueArrayOf(nRows, nColumns, 10.0)
[[ 10.0000, 10.0000, 10.0000, 10.0000, 10.0000],
[ 10.0000, 10.0000, 10.0000, 10.0000, 10.0000],
[ 10.0000, 10.0000, 10.0000, 10.0000, 10.0000]]
rowVector: [[ 1.0000, 2.0000, 3.0000]]
rowVector.shape(): [1, 3]
columnVector: [1.0000,
2.0000,
3.0000]
columnVector.shape(): [3, 1]
从 double[][]定义的INDArray:
[[ 1.0000, 2.0000, 3.0000],
[ 4.0000, 5.0000, 6.0000]]
统一随机数组:
[[ 0.2612, 0.0646, 0.4342, 0.0805, 0.0987],
[ 0.4138, 0.0900, 0.0167, 0.2613, 0.9905],
[ 0.6573, 0.5739, 0.7580, 0.2218, 0.4128]]
位置(0,0)处全精度随机值: 0.26116058230400085
N(0,1)随机数组:
[[ 0.9103, -0.0437, -0.7131, 0.2542, -0.4007],
[ -0.6663, -0.2015, -1.0871, 1.7668, -0.4649],
[ -0.8949, -0.3173, 0.2512, -0.0506, 0.1931]]
带有一些固定种子的统一随机数组:
[[ 0.2762, 0.2180, 0.0621, 0.0007, 0.2415],
[ 0.4948, 0.9348, 0.5204, 0.3029, 0.3290],
[ 0.7978, 0.0318, 0.1456, 0.9035, 0.9406]]
[[ 0.2762, 0.2180, 0.0621, 0.0007, 0.2415],
[ 0.4948, 0.9348, 0.5204, 0.3029, 0.3290],
[ 0.7978, 0.0318, 0.1456, 0.9035, 0.9406]]
创建更多维数的INDArrays:
三维数组形状: [3, 4, 5]
四维数组形状: [3, 4, 5, 6]
五维数组形状: [3, 4, 5, 6, 7]
从其它INDArrays创建INDArrays , 使用 hstack 或 vstack:
vStack:
[[ 1.0000, 2.0000, 3.0000],
[ 4.0000, 5.0000, 6.0000]]
hStack:
[[ 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000]]
Nd4j.eye(3):
[[ 1.0000, 0, 0],
[ 0, 1.0000, 0],
[ 0, 0, 1.0000]]
Nd4j.linspace(1,10,10):
[[ 1.0000, 2.0000, 3.0000, 4.0000, 5.0000, 6.0000, 7.0000, 8.0000, 9.0000, 10.0000]]
Nd4j.diag(rowVector2):
[[ 4.0000, 0, 0],
[ 0, 5.0000, 0],
[ 0, 0, 6.0000]]
翻译:风一样的男子
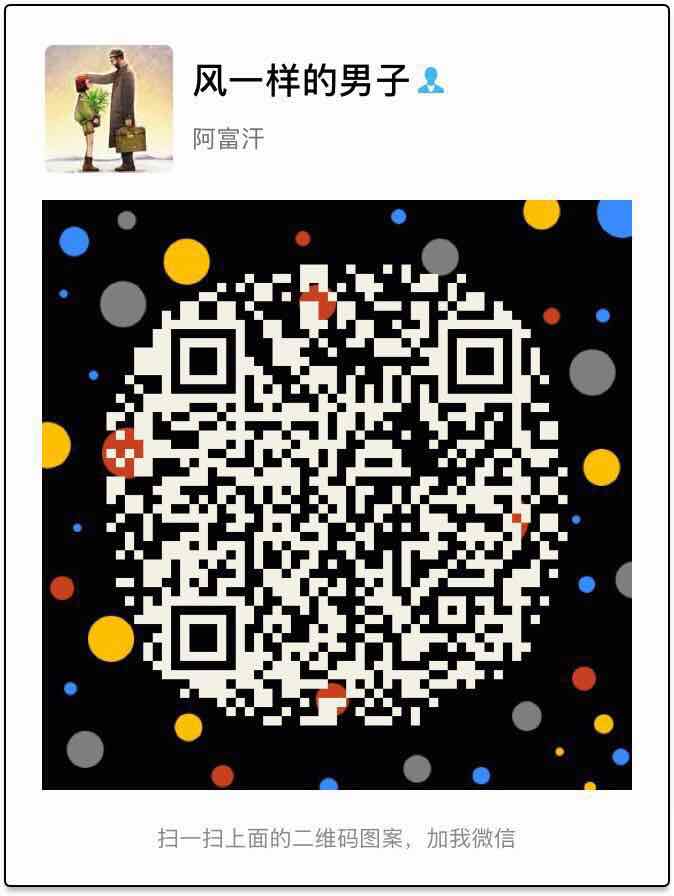
image