17 导航栏AppBar 、抽屉菜单Drawer、圆形图标ClipOval 的使用
1.源代码
import 'package:flutter/material.dart';
void main () => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("导航栏名称"),
leading: Builder(builder: (context){
return IconButton(
icon: Icon(Icons.dehaze,color: Colors.white,),
onPressed: (){
Scaffold.of(context).openDrawer();
},
);
}),
actions: <Widget>[
IconButton(
icon:Icon(Icons.share),
color: Colors.white,
onPressed: (){},
),
],
),
drawer: MyDrawer(),
)
);
}
}
class MyDrawer extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Drawer(
child: MediaQuery.removePadding(
context: context,
removeTop: true,
child: Column(
children: <Widget>[
Container(
padding: EdgeInsets.all(20.0),
color: Colors.blue,
child:
Row(
children: <Widget>[
ClipOval(
child: Image.network("https://ss0.bdstatic.com/70cFuHSh_Q1YnxGkpoWK1HF6hhy/it/u=3024387196,1621670548&fm=27&gp=0.jpg",width: 80.0,),
),
Container(
margin: EdgeInsets.only(left: 50.0),
child: Text("无名氏",style: TextStyle(
fontSize: 20.0,
color: Colors.white
),),
),
],
),
),
Expanded(
child: ListView(
children: <Widget>[
ListTile(
leading: Icon(Icons.person,color: Colors.orange,),
title: Text("个人信息"),
onTap: (){},
),
ListTile(
leading: Icon(Icons.wallpaper,color: Colors.orange,),
title: Text("我的相册"),
onTap: (){},
),
ListTile(
leading: Icon(Icons.wallpaper,color: Colors.orange,),
title: Text("我的相册"),
onTap: (){},
),
ListTile(
leading: Icon(Icons.settings,color: Colors.orange,),
title: Text("设置"),
onTap: (){},
),
],
),
)
],
)
),
);
}
}
2.解释源代码
import 'package:flutter/material.dart';
void main () => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("导航栏名称"),
leading: Builder(builder: (context){
return IconButton(
icon: Icon(Icons.dehaze,color: Colors.white,),
onPressed: (){
Scaffold.of(context).openDrawer();
},
);
}),
actions: <Widget>[
IconButton(
icon:Icon(Icons.share),
color: Colors.white,
onPressed: (){},
),
],
),
drawer: MyDrawer(),
)
);
}
}
class MyDrawer extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Drawer(
child: MediaQuery.removePadding(
context: context,
removeTop: true,
child: Column(
children: <Widget>[
Container(
padding: EdgeInsets.all(20.0),
color: Colors.blue,
child:
Row(
children: <Widget>[
ClipOval(
child: Image.network("https://ss0.bdstatic.com/70cFuHSh_Q1YnxGkpoWK1HF6hhy/it/u=3024387196,1621670548&fm=27&gp=0.jpg",width: 80.0,),
),
Container(
margin: EdgeInsets.only(left: 50.0),
child: Text("无名氏",style: TextStyle(
fontSize: 20.0,
color: Colors.white
),),
),
],
),
),
Expanded(
child: ListView(
children: <Widget>[
ListTile(
leading: Icon(Icons.person,color: Colors.orange,),
title: Text("个人信息"),
onTap: (){},
),
ListTile(
leading: Icon(Icons.wallpaper,color: Colors.orange,),
title: Text("我的相册"),
onTap: (){},
),
ListTile(
leading: Icon(Icons.wallpaper,color: Colors.orange,),
title: Text("我的相册"),
onTap: (){},
),
ListTile(
leading: Icon(Icons.settings,color: Colors.orange,),
title: Text("设置"),
onTap: (){},
),
],
),
)
],
)
),
);
}
}
3.效果图
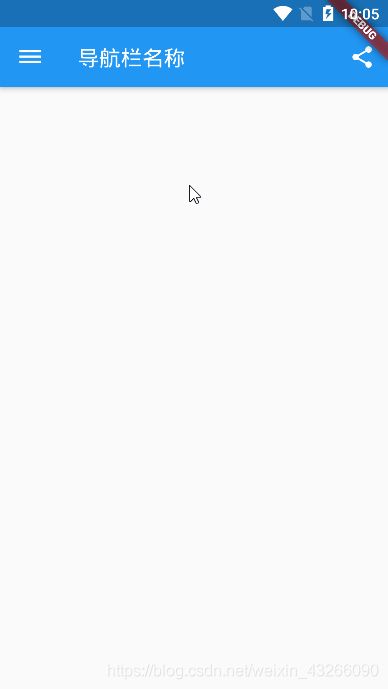
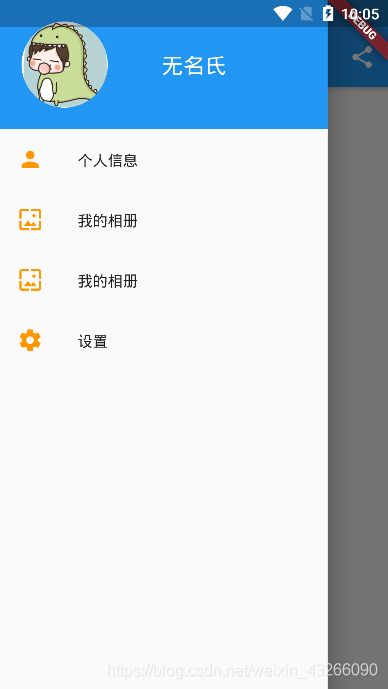