文章目录
- 【4.8.1】
- 【4.8.2】
- 【4.8.3】
- 【4.8.4】
- 【4.8.5】
- 【4.8.6】
- 【4.8.7】
- 【4.8.8】
【4.8.1】
#include
int main()
{
char last_name[20];
char first_name[20];
printf("\n请输入您的姓氏:\n");
scanf("%s",first_name);
printf("\n请输入您的名字:\n");
scanf("%s",last_name);
printf("\n您的名字为:%s,%s",last_name ,first_name);
return 0;
}
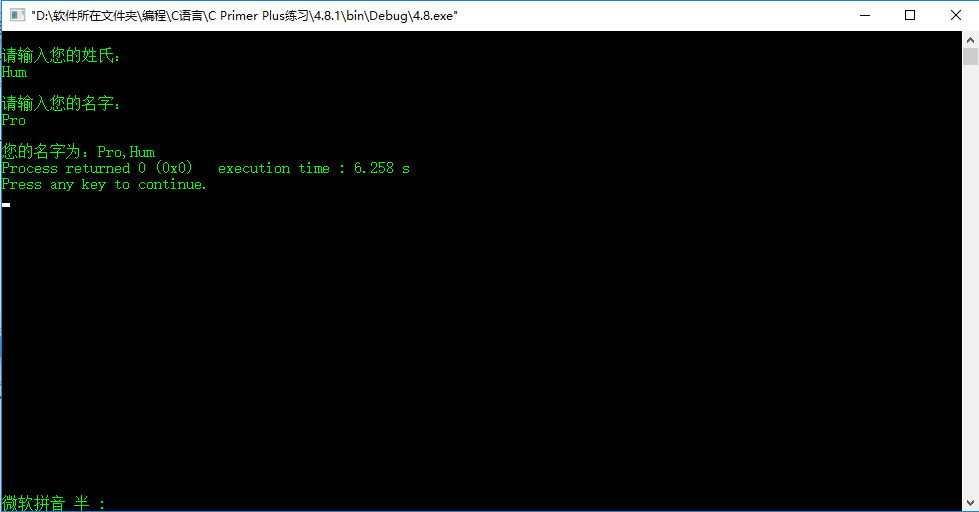
【4.8.2】
#include
#include
int main ()
{
char first_name[20];
char name[20];
int width;
printf("\n\t请输入您的姓氏:");
scanf("%s",first_name);
printf("\n\t请输入您的名字:");
scanf("%s",name);
printf("\n\t\"%s,%s\"\n\n",name,first_name);
printf("\n\t\"%10s%10s\"\n\n",name,first_name);
printf("\n\t\"%-10s%-10s\"\n\n",name,first_name);
width = strlen(first_name) + 3;
printf("\n\t%*s%s\n\n",width,first_name,name);
return 0;
}
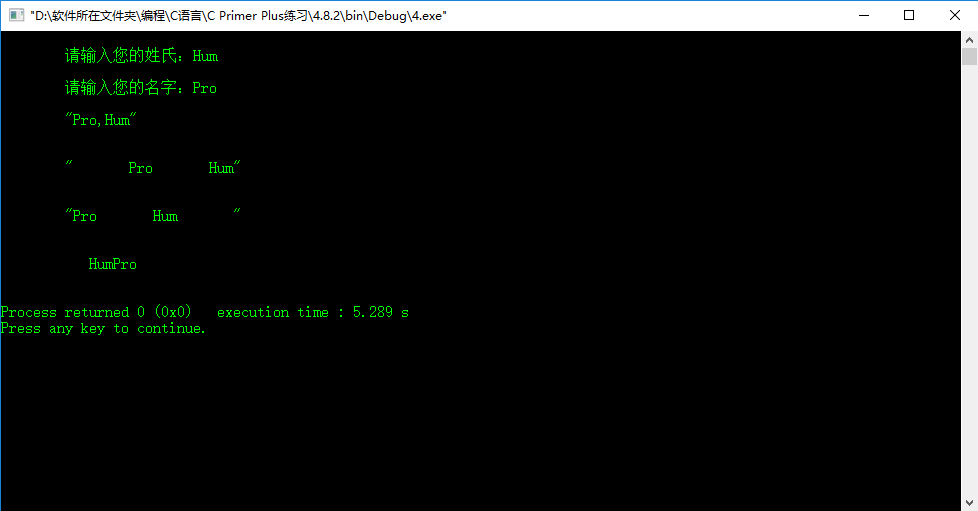
【4.8.3】
#include
int main()
{
float i;
printf("请输入一个浮点数:21.29\n");
scanf("%f",&i);
printf("\nThis input is %.1f or %.1e .\t",i,i);
printf("编译器对浮点数进行四舍五入。\n\n");
printf("This input is %+2.3f or %.3E .\t",i,i);
printf("编译器没有对浮点数进行四舍五入。\n\n");
return 0;
}
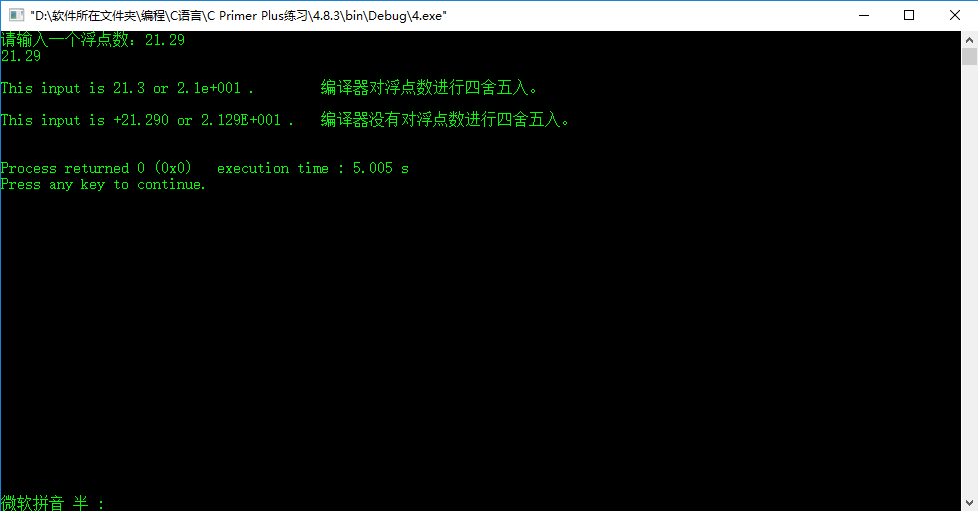
【4.8.4】
#include
int main()
{
system("color 0A");
float tall;
char name[20];
printf("\n\tPlease tell me your name:");
scanf("%s",name);
printf("\n\tPlease tell me your height(cm):");
scanf("%f",&tall);
printf("\n\t%s,you are %1.3f meter tall.\n\n",name ,tall/100);
return 0;
}
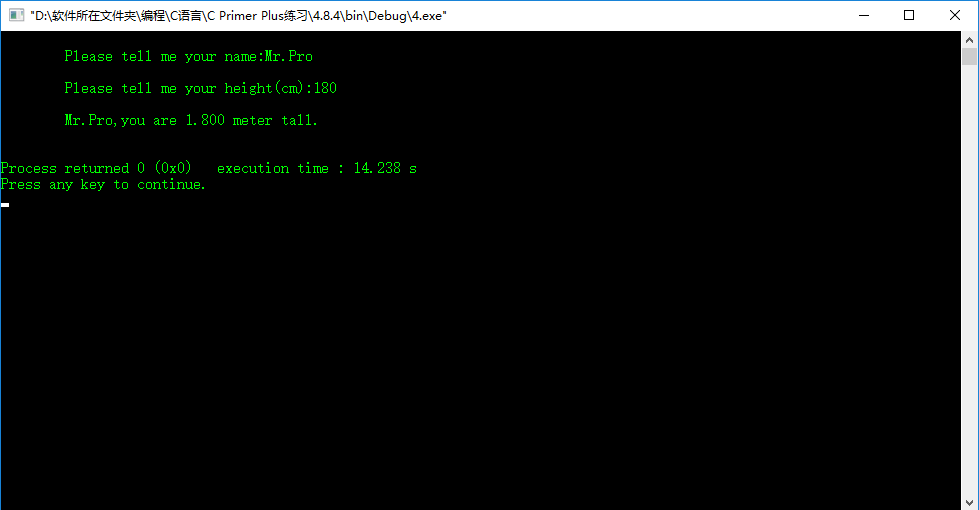
【4.8.5】
#include
int main()
{
system("color 0A");
float tall;
char name[20];
printf("\n\tPlease tell me your name:");
scanf("%s",name);
printf("\n\tPlease tell me your height(cm):");
scanf("%f",&tall);
printf("\n\t%s,you are %1.3f meter tall.\n\n",name ,tall/100);
return 0;
}
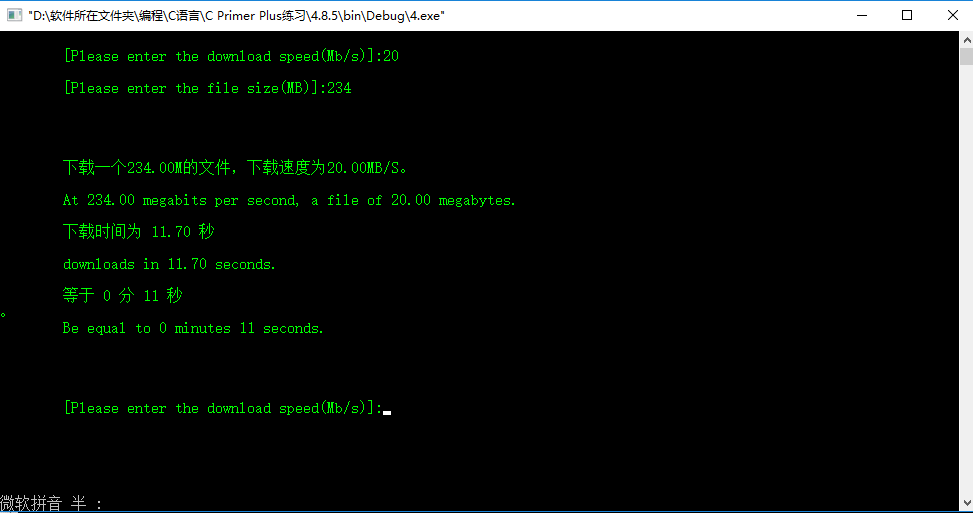
【4.8.6】
#include
#include
int main()
{
char fname[10];
char lname[10];
printf("\n\tPlease enter your first name:");
scanf("%s",fname);
printf("\n\tPlease enter your last name:");
scanf("%s",lname);
printf("\n\t%s %s\n",fname ,lname);
printf("\t%*d %*d\n",strlen(fname),strlen(fname) ,strlen(lname),strlen(lname));
printf("\t%s %s\n",fname ,lname);
printf("\t%*d %*d\n",-strlen(fname),strlen(fname) ,-strlen(lname),strlen(lname));
return 0;
}
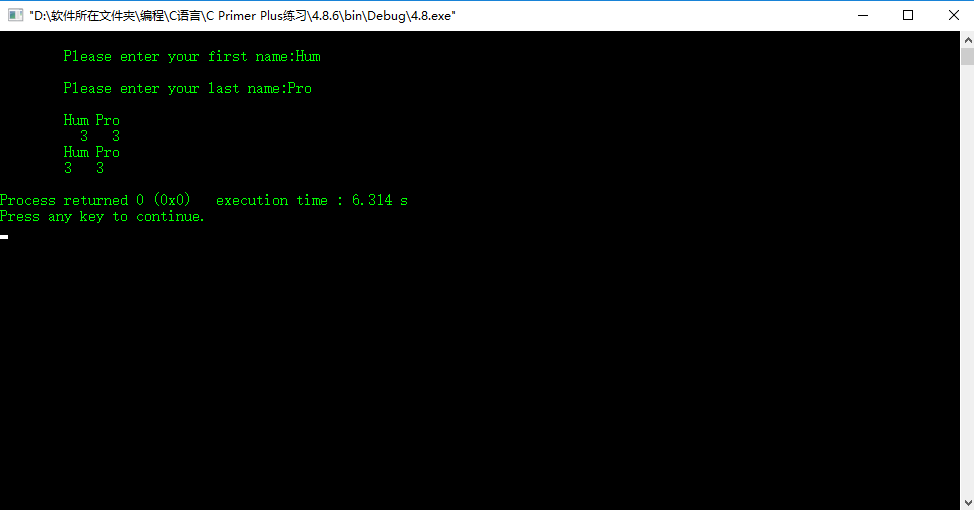
【4.8.7】
#include
#include
int main()
{
system("color 0A");
double a = 1.0/3.0;
float b = 1.0/3.0;
printf("\n\ta = %.6f \t\t\tb = %.6f\n",a ,b);
printf("\n\ta = %.12f \t\tb = %.12f\n",a ,b);
printf("\n\ta = %.16f \t\tb = %.16f\n",a ,b);
printf("\n\tdouble类型最少有效数字位数:%d\tfloat类型最少有效数字位数:%d\n\n",DBL_DIG ,FLT_DIG);
return 0;
}
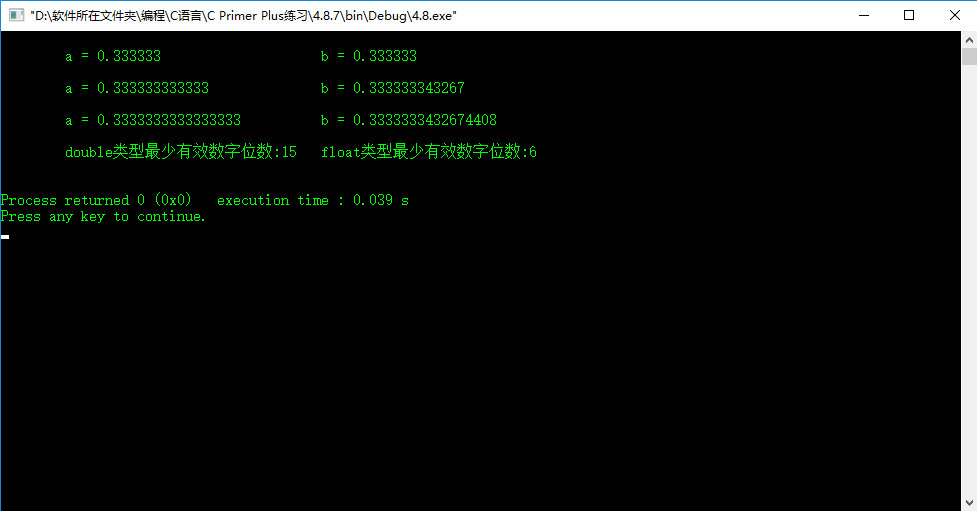
【4.8.8】
#include
#include
#define GALLON 3.785
#define MILE 1.609
int main()
{
system("color 0A");
float mile;
float gallon;
printf("\n\tPlease enter the mileage of the trip:\n");
printf("\t请输入旅行的里程km:");
scanf("\t%f",&mile);
printf("\n\tPlease enter the amount of petrol you need to consume:\n");
printf("\t请输入消耗的汽油量L:");
scanf("\t%f",&gallon);
printf("\n\tThe oil consumption is %.1f mile /gallon\n",mile / gallon);
printf("\t油耗为%.1f英里/加仑\n",mile / gallon);
printf("\n\tLitre per 100km:%.1fL\n",gallon*GALLON/(mile*MILE)*100);
printf("\t百公里消耗为:%.1f升\n",gallon*GALLON/(mile*MILE)*100);
return 0;
}
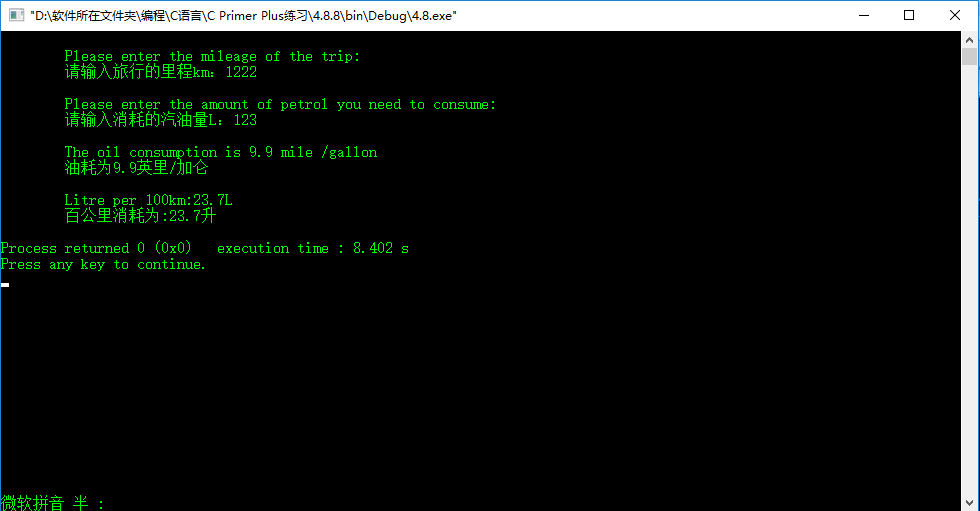