先来几张大神同事分享的ppt
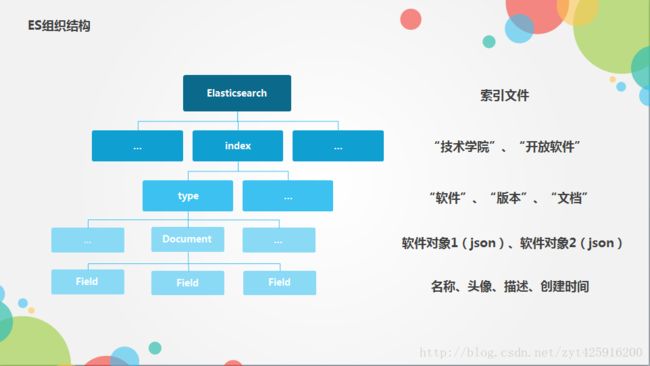
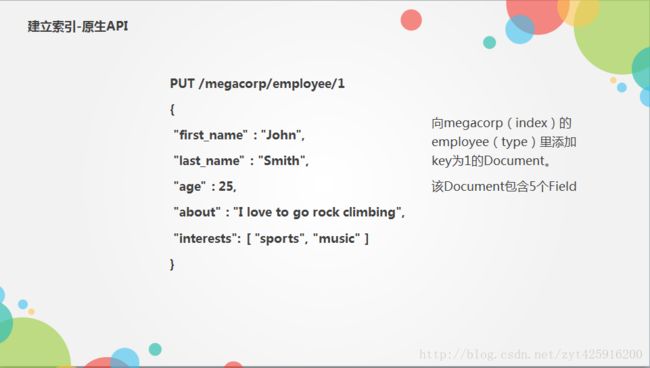
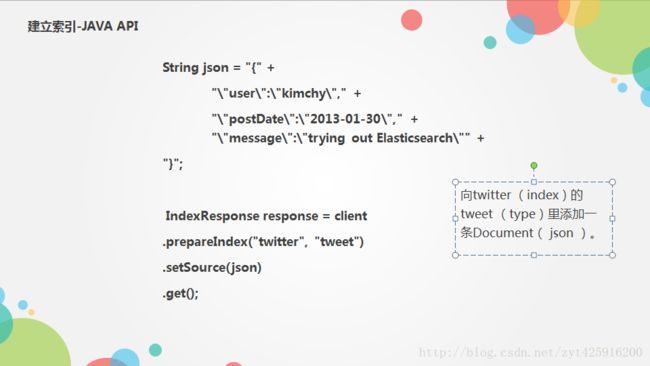
多的不说,快快上码
public PageInfo getAllRelatedPage(String keyword, String sortRule ,int pageSize,int pageNum) throws PcompException {
try {
String[] indexs = {Constants.PUBLIC_COMPONENT_SYSTEM, Constants.INNO_WORKS_SYSTEM};
String[] types = {PcompConstants.PCOMP_SOFTWARE, PcompConstants.PCOMP_SOFTWARE_ARTICLE, PcompConstants.PCOMP_VERSION, InnovationConstants.INNOVATION_WORKS};
SearchHits searchHits = getSearchHitsPage(keyword,indexs, types, sortRule, pageSize, pageNum);
if (searchHits == null) {
return null;
}
List searchResults = getSearchResults(searchHits.getHits());
return getResultPageInfo(searchResults, pageSize, pageNum, searchHits);
} catch (Exception e) {
throw new PcompException(e);
}
}
private SearchHits getSearchHitsPage(String keyword, String[] indexs ,String[] types, String sortRule, int pageSize , int pageNum) {
String[] stringMeta = esClient.getAllStringMeta(indexs, types);
if (stringMeta == null) {
return null;
}
MultiMatchQueryBuilder termQueryBuilder = QueryBuilders.multiMatchQuery(keyword, stringMeta);
QueryBuilder qb = new BoolQueryBuilder()
.must(QueryBuilders.termQuery("isDeleted", false))
.must(termQueryBuilder);
return esClient.searchDocumentPageSort(indexs, types, qb, sortRule, pageSize, pageNum);
}
/**
* 获取要搜索的目标域(例如:要搜索content、name等字段,就会返回["content","name"])
* @param indexs
* @param types
* @return
*/
public String[] getAllStringMeta(String indexs[], String types[]) {
HashSet<String> keySets = new HashSet<>();
String[] tempTypes = types;
HashMap<String,List<String>> hashMap = new HashMap<String,List<String>>();
List<String> list = new ArrayList<>();
list.add(PcompConstants.PCOMP_SOFTWARE);
list.add(PcompConstants.PCOMP_SOFTWARE_ARTICLE);
list.add(PcompConstants.PCOMP_VERSION);
hashMap.put(Constants.PUBLIC_COMPONENT_SYSTEM,list);
List<String> list1 = new ArrayList<>();
list1.add(InnovationConstants.INNOVATION_WORKS);
hashMap.put(Constants.INNO_WORKS_SYSTEM,list1);
try {
for(String index : indexs){
GetMappingsResponse getMappingsResponse = client.admin().indices().prepareGetMappings(index).get();
List<String> tempList = hashMap.get(index);
for(String type : tempList){
MappingMetaData mapMeta = getMappingsResponse.getMappings().get(index).get(type);
if(mapMeta == null){
break;
}
HashMap properties = (HashMap)mapMeta.getSourceAsMap().get("properties");
for (Object object : properties.keySet()) {
if (((HashMap) properties.get(object)).get("type").equals("string")) {
keySets.add((String) object);
}
}
}
}
return keySets.toArray(new String[]{});
} catch (Exception e) {
log.warn("Caught:", e);
return null;
}
}
//自带排序效果
public SearchHits searchDocumentPageSort(String[] indexes, String[] types, QueryBuilder queryBuilder , String sortRule, int pageSize , int pageNum) {
SortBuilder sortBuilder = new ScoreSortBuilder()
SearchRequestBuilder searchRequestBuilder = client.prepareSearch(indexes)
switch (sortRule) {
//相关度倒排
case SortRule.BY_CORRELATION_DESC:
sortBuilder.order(SortOrder.DESC)
searchRequestBuilder = searchRequestBuilder.addSort(sortBuilder)
break
//相关度正排
case SortRule.BY_CORRELATION:
sortBuilder.order(SortOrder.ASC)
searchRequestBuilder = searchRequestBuilder.addSort(sortBuilder)
break
//时间到排
case SortRule.BY_MODIFIED_TIME_DESC:
searchRequestBuilder = searchRequestBuilder.addSort("modifiedTime" , SortOrder.DESC)
break
//时间正排
case SortRule.BY_MODIFIED_TIME:
searchRequestBuilder = searchRequestBuilder.addSort("modifiedTime" , SortOrder.ASC)
break
}
SearchResponse searchResponse = searchRequestBuilder
.setTypes(types)
.addHighlightedField("*")
.setHighlighterRequireFieldMatch(false)
.setQuery(queryBuilder)
.setHighlighterPreTags("")
.setHighlighterPostTags("")
.setSize(pageSize)
.setFrom((pageNum - 1) * pageSize)
.execute()
.actionGet()
return searchResponse.getHits()
}
/**
* 将搜索结果转为List
* @param searchHit
* @return
* @throws PcompException
*/
private List getSearchResults(SearchHit[] searchHit)throws PcompException{
List searchResults = new LinkedList<>();
for (int i = 0; i < searchHit.length; i++) {
String json = searchHit[i].getSourceAsString();
SearchResult searchResult = new SearchResult();
switch (searchHit[i].type()){
case PcompConstants.PCOMP_SOFTWARE :
searchResult = getResult(JSON.parseObject(json, PcompSoftware.class),searchHit[i].getHighlightFields());break;
case PcompConstants.PCOMP_SOFTWARE_ARTICLE :
searchResult = getResult(JSON.parseObject(json, PcompSoftwareArticle.class),searchHit[i].getHighlightFields());break;
case PcompConstants.PCOMP_VERSION :
searchResult = getResult(JSON.parseObject(json, PcompVersion.class),searchHit[i].getHighlightFields());break;
case InnovationConstants.INNOVATION_WORKS :
searchResult = getResult(JSON.parseObject(json, Innovation.class),searchHit[i].getHighlightFields());break;
}
searchResults.add(searchResult);
}
return searchResults;
private SearchResult getResult(Object object, Map highlightFieldMap) throws PcompException {
SearchResult searchResult = new SearchResult()
SimpleDateFormat simpleDateFormat = simpleDateFormatThreadLocal.get()
if (object instanceof PcompSoftware) {
PcompSoftware pcompSoftware = (PcompSoftware) object
searchResult.setId(pcompSoftware.getId())
searchResult.setTitle(pcompSoftware.getName())
searchResult.setLink(StrUtils.makeString("opensoftware.html?pcompSoftwareId=", pcompSoftware.getId()))
// searchResult.setIntroduction(IntroductionUtils.getShortIntroduction(pcompSoftware.getIntroduction()))
searchResult.setIntroduction(highlightFieldMap.get("introduction")==null?pcompSoftware.getIntroduction():highlightFieldMap.get("introduction").toString())
searchResult.setAvatar(pcompSoftware.getAvatar())
searchResult.setModifiedTime(simpleDateFormat.format(pcompSoftware.getModifiedTime()))
searchResult.setPath(StrUtils.makeString("opensoftware.html?pcompSoftwareId=", pcompSoftware.getId()))
} else if (object instanceof PcompVersion) {
PcompVersion pcompVersion = (PcompVersion) object
searchResult.setId(pcompVersion.getId())
PcompSoftware pcompSoftware = pcompSoftwareManager.getPcompSoftwareByPcompSoftwareId(pcompVersion.getPcompSoftwareId())
searchResult.setTitle(StrUtils.makeString(
pcompSoftware.getName(),
Constants.NAME_SEPARATOR, pcompVersion.getVersionNumber()))
// searchResult.setIntroduction(IntroductionUtils.getShortIntroduction(pcompVersion.getIntroduction()))
searchResult.setIntroduction(highlightFieldMap.get("introduction")==null?pcompVersion.getIntroduction():highlightFieldMap.get("introduction").toString())
searchResult.setModifiedTime(simpleDateFormat.format(pcompVersion.getModifiedTime()))
searchResult.setAvatar(pcompSoftware.getAvatar())
searchResult.setLink(StrUtils.makeString("opensoftware.html?pcompSoftwareId=",pcompSoftware.getId(),"&category=history&versionId=",pcompVersion.getId()))
searchResult.setPath(StrUtils.makeString("opensoftware.html?pcompSoftwareId=",pcompSoftware.getId(),"&pcompVersionId=",pcompVersion.getId()))
}else if (object instanceof PcompSoftwareArticle) {
PcompSoftwareArticle pcompSoftwareArticle = (PcompSoftwareArticle) object
searchResult.setId(pcompSoftwareArticle.getId())
PcompSoftware pcompSoftware = pcompSoftwareManager.getPcompSoftwareByPcompSoftwareId(pcompSoftwareArticle.getPcompSoftwareId())
searchResult.setTitle(StrUtils.makeString(pcompSoftware.getName(),
Constants.NAME_SEPARATOR,
pcompSoftwareArticle.getTitle()))
// searchResult.setIntroduction(IntroductionUtils.getShortIntroduction(pcompSoftwareArticle.getContent()))
searchResult.setIntroduction(highlightFieldMap.get("content")==null?pcompSoftwareArticle.getContent():highlightFieldMap.get("content").toString())
searchResult.setModifiedTime(simpleDateFormat.format(pcompSoftwareArticle.getModifiedTime()))
searchResult.setAvatar(pcompSoftware.getAvatar())
searchResult.setLink(StrUtils.makeString("opensoftware.html?pcompSoftwareId=",pcompSoftware.getId(),"&category=article&articleId=",pcompSoftwareArticle.getId()))
searchResult.setPath(StrUtils.makeString("opensoftware.html?pcompSoftwareId=",pcompSoftware.getId(),"&pcompArticleId=",pcompSoftwareArticle.getId()))
}else if (object instanceof AcademyActivity) {
AcademyActivity academyActivity = (AcademyActivity) object
searchResult.setId(academyActivity.getId())
searchResult.setTitle(StrUtils.makeString(academyActivity.getTitle()))
searchResult.setIntroduction(IntroductionUtils.getShortIntroduction(academyActivity.getContent()))
searchResult.setModifiedTime(simpleDateFormat.format(academyActivity.getModifiedTime()))
searchResult.setAvatar(academyActivity.getImage())
searchResult.setLink(StrUtils.makeString("academyActivity.html?academyActivityId=",academyActivity.getId()))
searchResult.setPath(StrUtils.makeString("academyActivity.html?academyActivityId=", academyActivity.getId()))
}
else if (object instanceof AcademyDepartment) {
AcademyDepartment academyDepartment = (AcademyDepartment) object
searchResult.setId(academyDepartment.getId())
searchResult.setTitle(StrUtils.makeString(academyDepartment.getName()))
searchResult.setIntroduction(IntroductionUtils.getShortIntroduction(academyDepartment.getIntroduction()))
searchResult.setModifiedTime(simpleDateFormat.format(academyDepartment.getModifiedTime()))
searchResult.setAvatar(academyDepartment.getAvatar())
searchResult.setLink(StrUtils.makeString("academyDepartment.html?academyDepartmentId=", academyDepartment.getId()))
searchResult.setPath(
StrUtils.makeString("academyDepartment.html?academyDepartmentId=", academyDepartment.getId()))
}else if (object instanceof Innovation) {
Innovation innovation = (Innovation) object
searchResult.setId(innovation.getId().toString())
searchResult.setTitle(innovation.getWorksName())
searchResult.setIntroduction(highlightFieldMap.get("content")==null?innovation.getContent():highlightFieldMap.get("content").toString())
searchResult.setModifiedTime(simpleDateFormat.format(innovation.getModifiedTime()))
searchResult.setAvatar(innovation.getWorksImage())
searchResult.setPath(StrUtils.makeString("innodetail.html?workId=" , innovation.getId()))
searchResult.setLink(StrUtils.makeString("innodetail.html?workId=" , innovation.getId()))
} else {
searchResult = null
}
return searchResult
}
/**
* 将List searchResults转为PageInfo,
* 即为PageInfo赋上页面信息,页面展示的是PageInfo对象
* @param searchResults
* @param pageSize
* @param pageNum
* @param searchHit
* @return
*/
private PageInfo getResultPageInfo(List searchResults, int pageSize, int pageNum, SearchHits searchHit){
PageInfo searchPageInfo = new PageInfo<>();
searchPageInfo.setList(searchResults);
searchPageInfo.setPageSize(pageSize);
searchPageInfo.setFirstPage(1);
int lastPage = (int) (searchHit.getTotalHits() % pageSize == 0 ?
searchHit.getTotalHits() / pageSize :
searchHit.getTotalHits() / pageSize + 1);
searchPageInfo.setLastPage(lastPage);
searchPageInfo.setIsFirstPage(pageNum == 1);
searchPageInfo.setIsLastPage(pageNum == lastPage);
searchPageInfo.setHasPreviousPage(!searchPageInfo.isIsFirstPage());
searchPageInfo.setHasNextPage(!searchPageInfo.isIsLastPage());
searchPageInfo.setPageNum(pageNum);
int [] pageNums = new int[lastPage];
for(int i = 0; i < lastPage; i++){
pageNums[i] = i+1;
}
searchPageInfo.setNavigatepageNums(pageNums);
searchPageInfo.setTotal(searchHit.getTotalHits());
return searchPageInfo;
}