一、Spring与hibernate整合的关键点
1.hibernate的SessionFacetory对象交给spring创建
2.hibernate的事务(编程式事务控制)交给spring去控制
二、整合步骤
1)引入jar包
Hibernate相关jar
Spring core(5个)
Spring aop 包(4个)
Spring orm(5个,含数据驱动包、连接池包)
2)配置
hibernate.cfg.xml
bean.xml
3)搭建环境、单独测试
2.1 导包
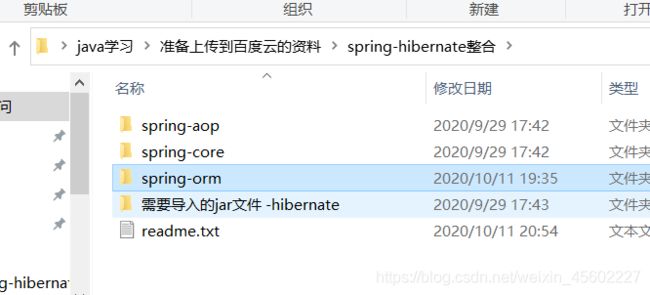
2.2 整合方式一 – 简单整合:直接加载hibernate.cfg.xml文件的方式整合
>>>>>> hibernate.cfg.xml
<hibernate-configuration>
<session-factory >
<property name="hibernate.connection.driver_class">com.mysql.jdbc.Driverproperty>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/testproperty>
<property name="hibernate.connection.username">rootproperty>
<property name="hibernate.connection.password">rootproperty>
<property name="hibernate.dialect">org.hibernate.dialect.MySQL5Dialectproperty>
<property name="hibernate.show_sql">trueproperty>
<property name="hibernate.format_sql">falseproperty>
<property name="hibernate.hbm2ddl.auto">createproperty>
<mapping resource="org/jsoft2/entity/LogEntity.hbm.xml"/>
session-factory>
hibernate-configuration>
>>>>>> bean.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="configLocation" value="classpath:org/jsoft2/hibernate.cfg.xml">property>
bean>
<bean id="txManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="get*" read-only="true"/>
<tx:method name="find*" read-only="true"/>
<tx:method name="*" read-only="false"/>
tx:attributes>
tx:advice>
<aop:config>
<aop:pointcut expression="execution(* org.jsoft2.service.*.*(..))" id="pt"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pt"/>
aop:config>
<bean id="logDao" class="org.jsoft2.dao.LogDao">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<bean id="logService" class="org.jsoft2.service.LogService">
<property name="logDao" ref="logDao">property>
bean>
beans>
>>>>>> LogDao.java
public class LogDao {
private SessionFactory sessionFactory;
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public void add(LogEntity log) {
sessionFactory.getCurrentSession().save(log);
}
}
>>>>>> LogService .java
public class LogService {
private LogDao logDao;
public void setLogDao(LogDao logDao) {
this.logDao = logDao;
}
public void addLog() {
LogEntity log=new LogEntity();
log.setContent("新增。。。");
logDao.add(log);
}
}
>>>>>> testApp .java
/**
* spring与hibernate整合
* 方式一: 直接加载hibernate.cfg.xml进行整合
*
* @author BGS
*
*/
public class testApp {
public static void main(String[] args) {
ApplicationContext ac=new ClassPathXmlApplicationContext("org/jsoft2/bean.xml");
LogService logService = ac.getBean("logService",LogService.class);
logService.addLog();
}
}
2.3 整合方式二 – 部分整合:连接池交给spring管理 【一部分配置写到hibernate中,一份分在spring中完成】
>>>>>> hibernate.cfg.xml
<hibernate-configuration>
<session-factory >
<property name="hibernate.dialect">org.hibernate.dialect.MySQL5Dialectproperty>
<property name="hibernate.show_sql">trueproperty>
<property name="hibernate.format_sql">falseproperty>
<property name="hibernate.hbm2ddl.auto">createproperty>
<mapping resource="org/jsoft3/entity/LogEntity.hbm.xml"/>
session-factory>
hibernate-configuration>
>>>>>> bean.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/test">property>
<property name="driverClass" value="com.mysql.jdbc.Driver">property>
<property name="user" value="root">property>
<property name="password" value="root">property>
<property name="initialPoolSize" value="3">property>
<property name="maxPoolSize" value="6">property>
<property name="maxIdleTime" value="1000">property>
bean>
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="configLocation" value="classpath:org/jsoft2/hibernate.cfg.xml">property>
<property name="dataSource" ref="dataSource">property>
bean>
<bean id="txManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="get*" read-only="true"/>
<tx:method name="find*" read-only="true"/>
<tx:method name="*" read-only="false"/>
tx:attributes>
tx:advice>
<aop:config>
<aop:pointcut expression="execution(* org.jsoft2.service.*.*(..))" id="pt"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pt"/>
aop:config>
<bean id="logDao" class="org.jsoft2.dao.LogDao">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<bean id="logService" class="org.jsoft2.service.LogService">
<property name="logDao" ref="logDao">property>
bean>
beans>
>>>>>> LogDao.java
public class LogDao {
private SessionFactory sessionFactory;
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public void add(LogEntity log) {
sessionFactory.getCurrentSession().save(log);
}
}
>>>>>> LogService .java
public class LogService {
private LogDao logDao;
public void setLogDao(LogDao logDao) {
this.logDao = logDao;
}
public void addLog() {
LogEntity log=new LogEntity();
log.setContent("新增。。。");
logDao.add(log);
}
}
>>>>>> testApp .java
/**
* spring与hibernate整合
* 方式一: 直接加载hibernate.cfg.xml进行整合
*
* @author BGS
*
*/
public class testApp {
public static void main(String[] args) {
ApplicationContext ac=new ClassPathXmlApplicationContext("org/jsoft2/bean.xml");
LogService logService = ac.getBean("logService",LogService.class);
logService.addLog();
}
}
2.3 整合方式三 – 完全整合:所有的配置全部都在Spring配置文件中完成
>>>>>> bean.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/test">property>
<property name="driverClass" value="com.mysql.jdbc.Driver">property>
<property name="user" value="root">property>
<property name="password" value="root">property>
<property name="initialPoolSize" value="3">property>
<property name="maxPoolSize" value="6">property>
<property name="maxIdleTime" value="1000">property>
bean>
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource">property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialectprop>
<prop key="hibernate.show_sql">trueprop>
<prop key="hibernate.hbm2ddl.auto">updateprop>
props>
property>
<property name="mappingDirectoryLocations">
<list>
<value>classpath:org/jsoft/entityvalue>
list>
property>
bean>
<bean id="txManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="get*" read-only="true"/>
<tx:method name="find*" read-only="true"/>
<tx:method name="*" read-only="false"/>
tx:attributes>
tx:advice>
<aop:config>
<aop:pointcut expression="execution(* org.jsoft.service.*.*(..))" id="pt"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pt"/>
aop:config>
<bean id="deptDao" class="org.jsoft.dao.DeptDao">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<bean id="deptService" class="org.jsoft.service.DeptService">
<property name="deptDao" ref="deptDao">property>
bean>
<bean id="deptAction" class="org.jsoft.action.DeptAction">
<property name="deptService" ref="deptService">property>
bean>
beans>
>>>>>> LogDao.java
public class LogDao {
private SessionFactory sessionFactory;
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public void add(LogEntity log) {
sessionFactory.getCurrentSession().save(log);
}
}
>>>>>> LogService .java
public class LogService {
private LogDao logDao;
public void setLogDao(LogDao logDao) {
this.logDao = logDao;
}
public void addLog() {
LogEntity log=new LogEntity();
log.setContent("新增。。。");
logDao.add(log);
}
}
>>>>>> testApp .java
public class testApp {
public static void main(String[] args) {
ApplicationContext ac=new ClassPathXmlApplicationContext("org/jsoft2/bean.xml");
LogService logService = ac.getBean("logService",LogService.class);
logService.addLog();
}
}
三、实例
3.1 实例代码(使用安全整合方式整合hibernate和spring)
>>>>>> bean-base.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:dwr="http://directwebremoting.org/schema/spring-dwr/spring-dwr-2.4.xsd"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="jdbcUrl" value="jdbc:mysql://localhost:3306/test">property>
<property name="driverClass" value="com.mysql.jdbc.Driver">property>
<property name="user" value="root">property>
<property name="password" value="root">property>
<property name="initialPoolSize" value="3">property>
<property name="maxPoolSize" value="6">property>
<property name="maxIdleTime" value="1000">property>
bean>
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource">property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialectprop>
<prop key="hibernate.show_sql">trueprop>
<prop key="hibernate.hbm2ddl.auto">updateprop>
props>
property>
<property name="mappingDirectoryLocations">
<list>
<value>classpath:org/hlp/entityvalue>
list>
property>
bean>
<bean id="txManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="get*" read-only="true"/>
<tx:method name="find*" read-only="true"/>
<tx:method name="*" read-only="false"/>
tx:attributes>
tx:advice>
<aop:config >
<aop:pointcut expression="execution(* org.hlp.dao.*.*(..))" id="pt"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pt"/>
aop:config>
<bean id="adminDao" class="org.hlp.dao.AdminDao">
<property name="sf" ref="sessionFactory">property>
bean>
beans>
>>>>>> AdminEntity.java
public class AdminEntity {
private String id;
private String account;
private String pwd;
private String name;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
>>>>>> AdminEntity.hbm.xml
<hibernate-mapping package="org.hlp.entity" >
<class name="AdminEntity" table="t_admin">
<id name="id" column="id" >id>
<property name="account" column="account">property>
<property name="pwd" column="pwd">property>
class>
hibernate-mapping>
>>>>>> AdminDao.java
public class AdminDao {
private SessionFactory sf;
public SessionFactory getSf() {
return sf;
}
public void setSf(SessionFactory sf) {
this.sf = sf;
}
public void save() {
AdminEntity admin=new AdminEntity();
admin.setId(UUID.randomUUID().toString());
admin.setAccount(UUID.randomUUID().toString());
admin.setName(UUID.randomUUID().toString());
admin.setPwd("admin");
Session session = sf.getCurrentSession();
session.save(admin);
}
public static void main(String[] args) {
ApplicationContext ac=new ClassPathXmlApplicationContext("bean-base.xml");
AdminDao adminDao=(AdminDao) ac.getBean("adminDao");
adminDao.save();
}
}
3.2 注意事项
在spring与hibernate整合时,由于事务由spring管理,所以在写增删改查时,
无需开启事务和提交事务。示例如以下代码。
【前提:配置事务拦截时,必须配置到写增删改查的类】
public void save() {
AdminEntity admin=new AdminEntity();
admin.setId(UUID.randomUUID().toString());
admin.setAccount(UUID.randomUUID().toString());
admin.setName(UUID.randomUUID().toString());
admin.setPwd("admin");
Session session = sf.getCurrentSession();
session.save(admin);
}
四、整合细节
4.1 spring与hibernate整合总结
spring整合hibernate的关键点在于
1.hibernate的SessionFactory对象交给spring创建
2.hibernate的事务管理交给spring
spring整合hibernate有三种方式:
1.直接加载hibernate.cfg.xml整合
2.连接池交给spring【数据源配置下在spring中,其他配置写在hibernate中】
3.所有的配置全部在spring中完成
>>>>>> DeptDao.java
public class DeptDao {
private SessionFactory sessionFactory;
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public void save(Dept dept) {
sessionFactory.getCurrentSession().save(dept);
}
}
>>>>>> DeptService.java
public class DeptService {
private DeptDao deptDao;
public void setDeptDao(DeptDao deptDao) {
this.deptDao = deptDao;
}
public void save(Dept dept){
deptDao.save(dept);
}
}
>>>>>> App .java
public class App {
private ApplicationContext ac = new ClassPathXmlApplicationContext("bean.xml");
@Test
public void testApp() throws Exception {
DeptService deptServie = (DeptService) ac.getBean("deptService");
System.out.println(deptServie.getClass());
deptServie.save(new Dept());
}
}
>>>>>> bean.xml【Spring管理SessionFactory的3中方式】
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="deptDao" class="cn.itcast.dao.DeptDao">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<bean id="deptService" class="cn.itcast.service.DeptService">
<property name="deptDao" ref="deptDao">property>
bean>
<bean id="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="com.mysql.jdbc.Driver">property>
<property name="jdbcUrl" value="jdbc:mysql:///hib_demo">property>
<property name="user" value="root">property>
<property name="password" value="root">property>
<property name="initialPoolSize" value="3">property>
<property name="maxPoolSize" value="10">property>
<property name="maxStatements" value="100">property>
<property name="acquireIncrement" value="2">property>
bean>
<bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="dataSource" ref="dataSource">property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialectprop>
<prop key="hibernate.show_sql">trueprop>
<prop key="hibernate.hbm2ddl.auto">updateprop>
props>
property>
<property name="mappingDirectoryLocations">
<list>
<value>classpath:cn/itcast/entity/value>
list>
property>
bean>
<bean id="txManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory">property>
bean>
<tx:advice id="txAdvice" transaction-manager="txManager">
<tx:attributes>
<tx:method name="*" read-only="false"/>
tx:attributes>
tx:advice>
<aop:config>
<aop:pointcut expression="execution(* cn.itcast.service.*.*(..))" id="pt"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="pt"/>
aop:config>
beans>
4.2 spring与hibernate整合,session默认以线程的方式创建
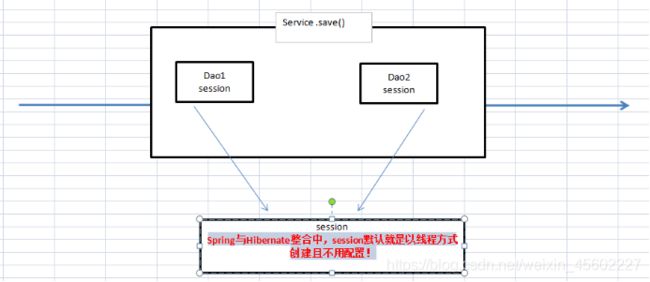
3.3 spring加载多个bean-*.xml配置文件,配置文件中的依赖注入可以跨文件
spring可以加载多个配置文件。
多个配置文件的依赖注入可以跨文件。
即bean1.xml声明的对象,可以在bean2.xml中作为属性被其他对象注入。
>>>>>> bean-controller.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="deptAction" class="org.jsoft.action.DeptAction">
<property name="deptService" ref="deptService">property>
bean>
beans>
>>>>>> bean-service.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<bean id="deptService" class="org.jsoft.service.DeptService">
<property name="deptDao" ref="deptDao">property>
bean>
beans>
3.3 spring与hibernate整合,dao层获取session对象通过sessionFactory.getCurrentSession()方法
public class DeptDao {
private SessionFactory sessionFactory;
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
public List<DeptEntity> query() {
Session session = sessionFactory.getCurrentSession();
Query query = session.createQuery("from DeptEntity");
List<DeptEntity> list = query.list();
return list;
}
}