想要学习GC,首先需要理解为什么需要GC?
MibBridge *pBridge= new cmBaseGroupBridge();
//如果注册失败,使用Delete释放该对象所占内存区域
if(pBridge->Register(kDestroy)!=NO ERROR)
delete pBridge;
MibBridge *pBridge=new cmBaseGroupBridge();
pBridge->Register(kDestroy);
官网介绍:https://docs.oracle.com/javase/8/docs/technotes/guides/vm/gctuning/toc.html
自动内存管理的优点
关于自动内存管理的担忧
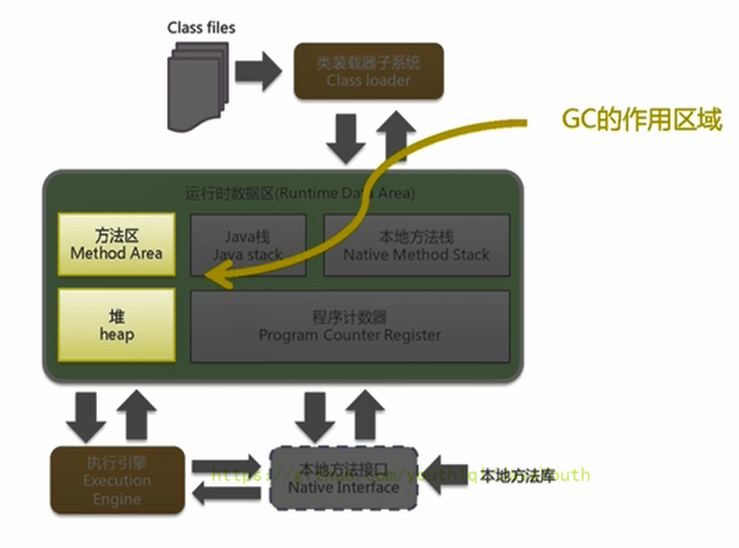
垃圾标记阶段:主要是为了判断对象是否存活
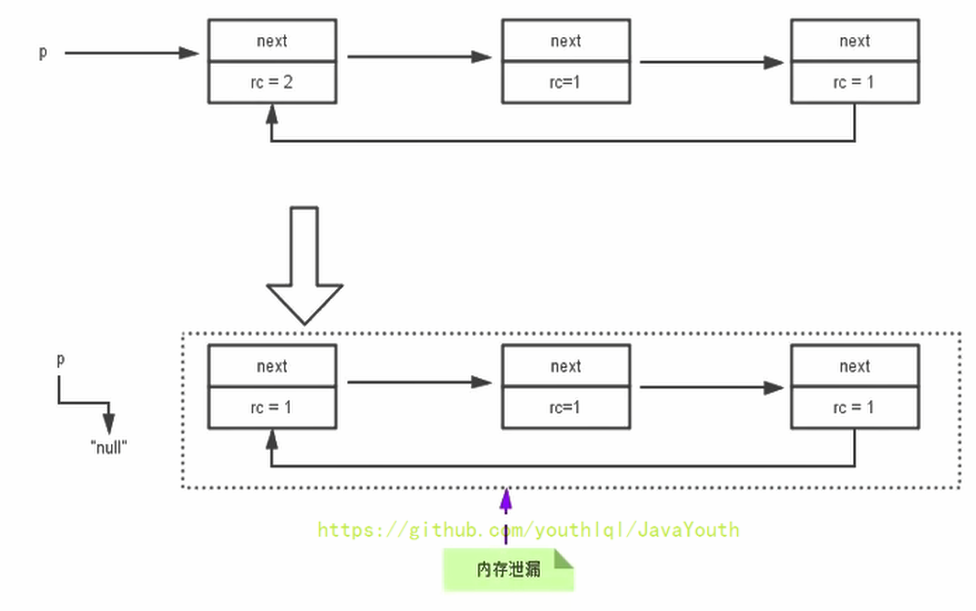
当p的指针断开的时候,内部的引用形成一个循环,计数器都还算1,无法被回收,这就是循环引用,从而造成内存泄漏
/**
* -XX:+PrintGCDetails
* 证明:java使用的不是引用计数算法
*/
public class RefCountGC {
//这个成员属性唯一的作用就是占用一点内存
private byte[] bigSize = new byte[5 * 1024 * 1024];//5MB
Object reference = null;
public static void main(String[] args) {
RefCountGC obj1 = new RefCountGC();
RefCountGC obj2 = new RefCountGC();
obj1.reference = obj2;
obj2.reference = obj1;
obj1 = null;
obj2 = null;
//显式的执行垃圾回收行为
//这里发生GC,obj1和obj2能否被回收?
System.gc();
}
}
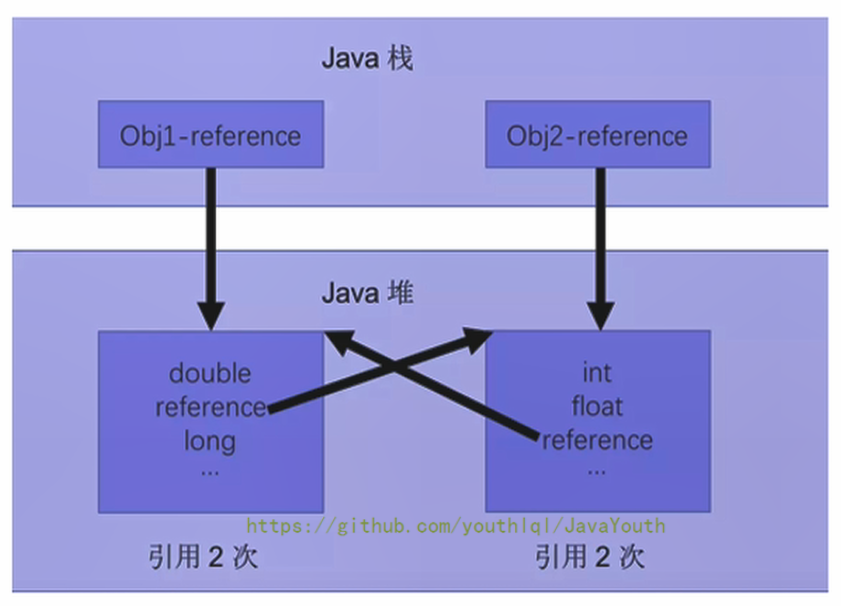
obj1.reference
和obj2.reference
置为null。则在Java堆中的两块内存依然保持着互相引用,无法被回收没有进行GC时
把下面的几行代码注释掉,让它来不及
System.gc();//把这行代码注释掉
Heap
PSYoungGen total 38400K, used 14234K [0x00000000d5f80000, 0x00000000d8a00000, 0x0000000100000000)
eden space 33280K, 42% used [0x00000000d5f80000,0x00000000d6d66be8,0x00000000d8000000)
from space 5120K, 0% used [0x00000000d8500000,0x00000000d8500000,0x00000000d8a00000)
to space 5120K, 0% used [0x00000000d8000000,0x00000000d8000000,0x00000000d8500000)
ParOldGen total 87552K, used 0K [0x0000000081e00000, 0x0000000087380000, 0x00000000d5f80000)
object space 87552K, 0% used [0x0000000081e00000,0x0000000081e00000,0x0000000087380000)
Metaspace used 3496K, capacity 4498K, committed 4864K, reserved 1056768K
class space used 387K, capacity 390K, committed 512K, reserved 1048576K
Process finished with exit code 0
进行GC
打开那行代码的注释
[GC (System.gc()) [PSYoungGen: 13569K->808K(38400K)] 13569K->816K(125952K), 0.0012717 secs] [Times: user=0.00 sys=0.00, real=0.00 secs]
[Full GC (System.gc()) [PSYoungGen: 808K->0K(38400K)] [ParOldGen: 8K->670K(87552K)] 816K->670K(125952K), [Metaspace: 3491K->3491K(1056768K)], 0.0051769 secs] [Times: user=0.00 sys=0.00, real=0.00 secs]
Heap
PSYoungGen total 38400K, used 333K [0x00000000d5f80000, 0x00000000d8a00000, 0x0000000100000000)
eden space 33280K, 1% used [0x00000000d5f80000,0x00000000d5fd34a8,0x00000000d8000000)
from space 5120K, 0% used [0x00000000d8000000,0x00000000d8000000,0x00000000d8500000)
to space 5120K, 0% used [0x00000000d8500000,0x00000000d8500000,0x00000000d8a00000)
ParOldGen total 87552K, used 670K [0x0000000081e00000, 0x0000000087380000, 0x00000000d5f80000)
object space 87552K, 0% used [0x0000000081e00000,0x0000000081ea7990,0x0000000087380000)
Metaspace used 3498K, capacity 4498K, committed 4864K, reserved 1056768K
class space used 387K, capacity 390K, committed 512K, reserved 1048576K
Process finished with exit code 0
1、从打印日志就可以明显看出来,已经进行了GC
2、如果使用引用计数算法,那么这两个对象将会无法回收。而现在两个对象被回收了,说明Java使用的不是引用计数算法来进行标记的。
可达性分析算法:也可以称为根搜索算法、追踪性垃圾收集
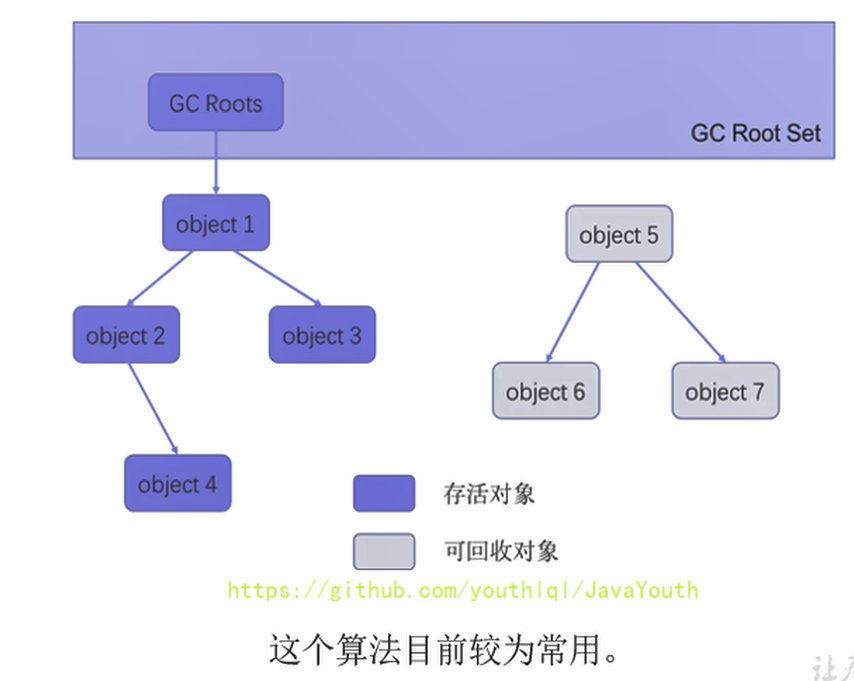
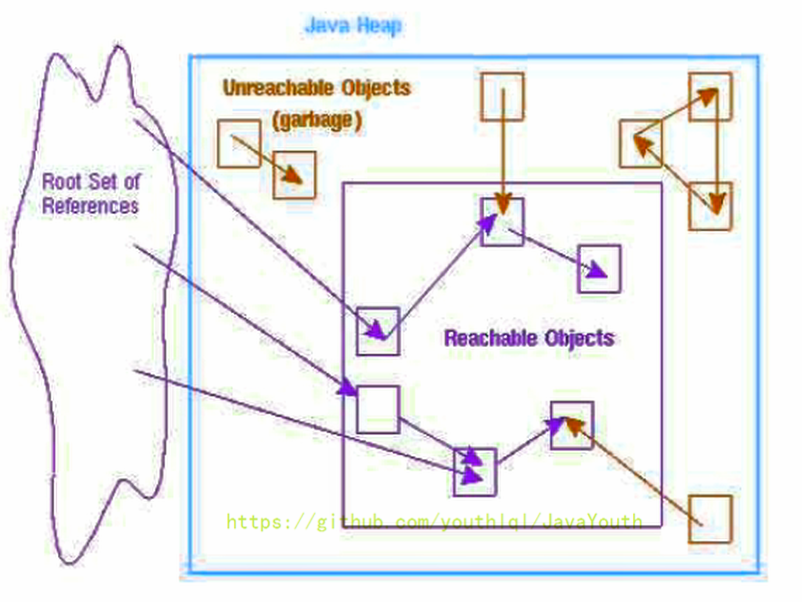
小技巧
由于Root采用栈方式存放变量和指针,所以如果一个指针,它保存了堆内存里面的对象,但是自己又不存放在堆内存里面,那它就是一个Root。
对象销毁前的回调函数:finalize()
Object 类中 finalize() 源码
// 等待被重写
protected void finalize() throws Throwable { }
由于finalize()方法的存在,虚拟机中的对象一般处于三种可能的状态。
判定一个对象objA是否可回收,至少要经历两次标记过程:
通过 JVisual VM 查看 Finalizer 线程
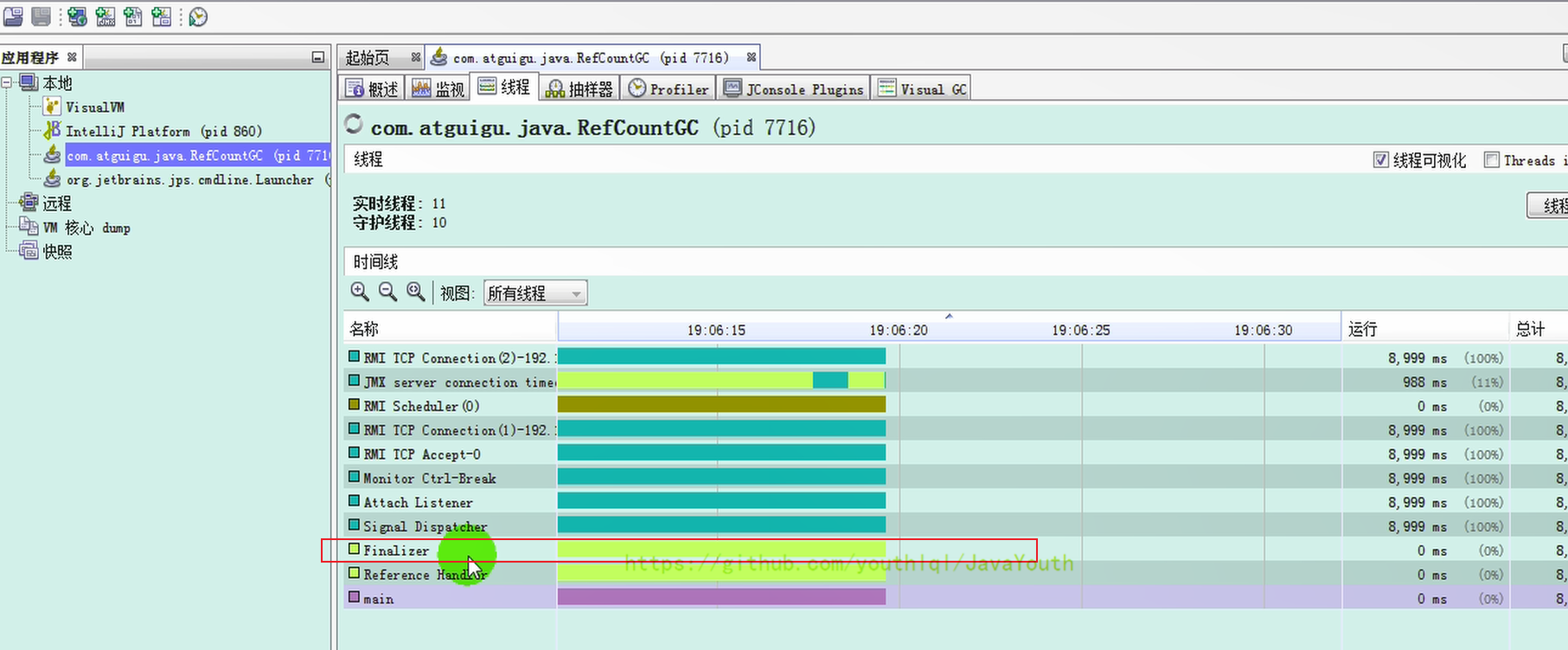
我们重写 CanReliveObj 类的 finalize()方法,在调用其 finalize()方法时,将 obj 指向当前类对象 this
/**
* 测试Object类中finalize()方法,即对象的finalization机制。
*
*/
public class CanReliveObj {
public static CanReliveObj obj;//类变量,属于 GC Root
//此方法只能被调用一次
@Override
protected void finalize() throws Throwable {
super.finalize();
System.out.println("调用当前类重写的finalize()方法");
obj = this;//当前待回收的对象在finalize()方法中与引用链上的一个对象obj建立了联系
}
public static void main(String[] args) {
try {
obj = new CanReliveObj();
// 对象第一次成功拯救自己
obj = null;
System.gc();//调用垃圾回收器
System.out.println("第1次 gc");
// 因为Finalizer线程优先级很低,暂停2秒,以等待它
Thread.sleep(2000);
if (obj == null) {
System.out.println("obj is dead");
} else {
System.out.println("obj is still alive");
}
System.out.println("第2次 gc");
// 下面这段代码与上面的完全相同,但是这次自救却失败了
obj = null;
System.gc();
// 因为Finalizer线程优先级很低,暂停2秒,以等待它
Thread.sleep(2000);
if (obj == null) {
System.out.println("obj is dead");
} else {
System.out.println("obj is still alive");
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
如果注释掉finalize()方法
//此方法只能被调用一次
@Override
protected void finalize() throws Throwable {
super.finalize();
System.out.println("调用当前类重写的finalize()方法");
obj = this;//当前待回收的对象在finalize()方法中与引用链上的一个对象obj建立了联系
}
输出结果:
第1次 gc
obj is dead
第2次 gc
obj is dead
放开finalize()方法
输出结果:
第1次 gc
调用当前类重写的finalize()方法
obj is still alive
第2次 gc
obj is dead
第一次自救成功,但由于 finalize() 方法只会执行一次,所以第二次自救失败
1、虽然Jvisualvm很强大,但是在内存分析方面,还是MAT更好用一些
2、此小节主要是为了实时分析GC Roots是哪些东西,中间需要用到一个dump的文件
方式一:命令行使用 jmap
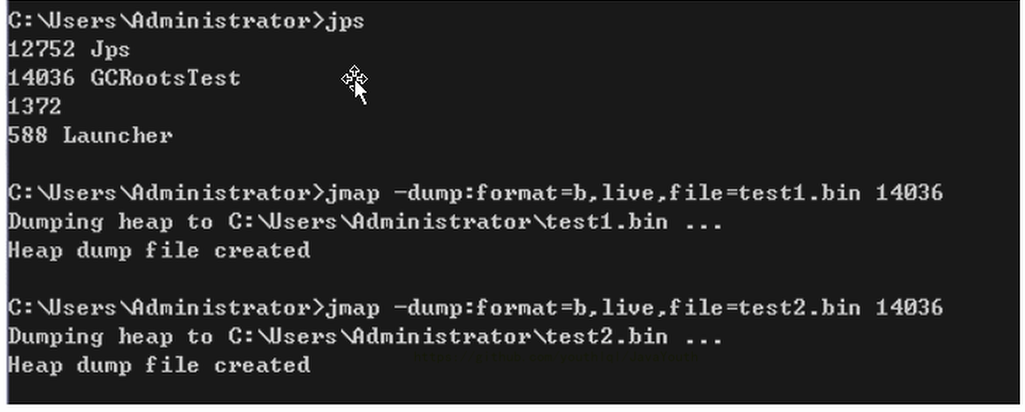
方式二:使用JVisualVM
代码:
public class GCRootsTest {
public static void main(String[] args) {
List<Object> numList = new ArrayList<>();
Date birth = new Date();
for (int i = 0; i < 100; i++) {
numList.add(String.valueOf(i));
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("数据添加完毕,请操作:");
new Scanner(System.in).next();
numList = null;
birth = null;
System.out.println("numList、birth已置空,请操作:");
new Scanner(System.in).next();
System.out.println("结束");
}
}
如何捕捉堆内存快照
1、先执行第一步,然后停下来,去生成此步骤dump文件
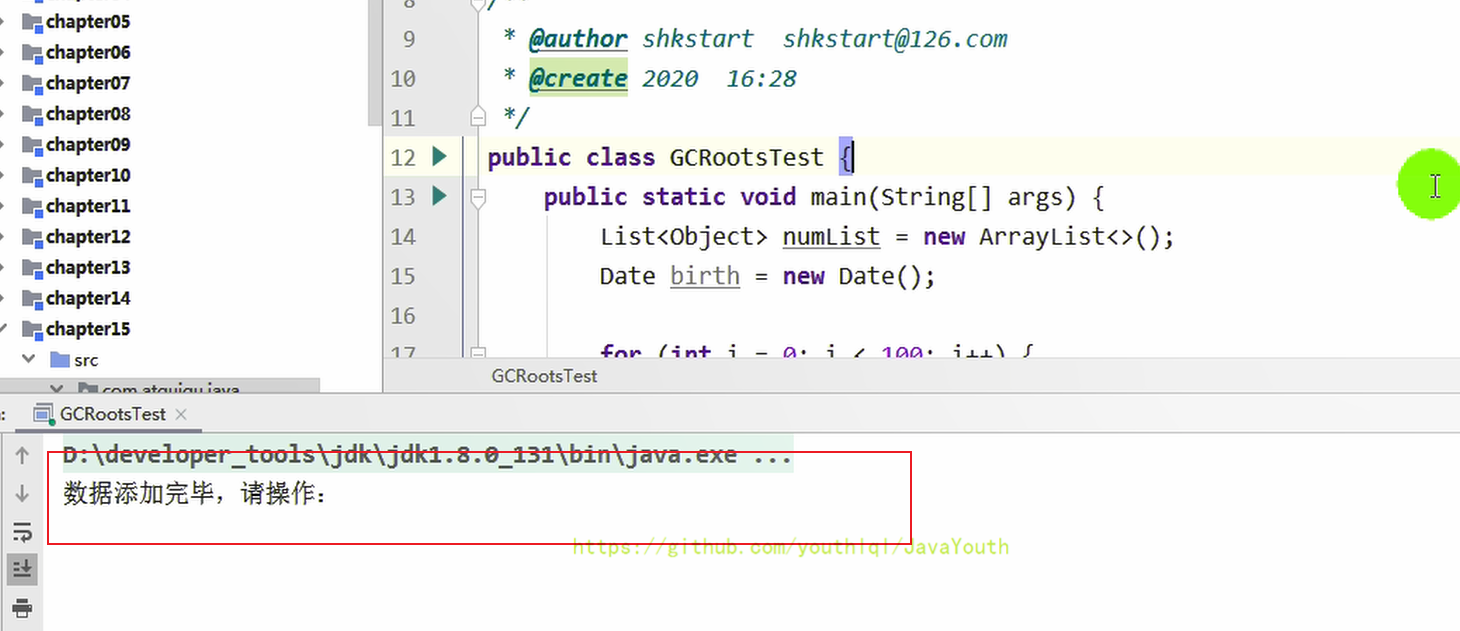
2、 点击【堆 Dump】
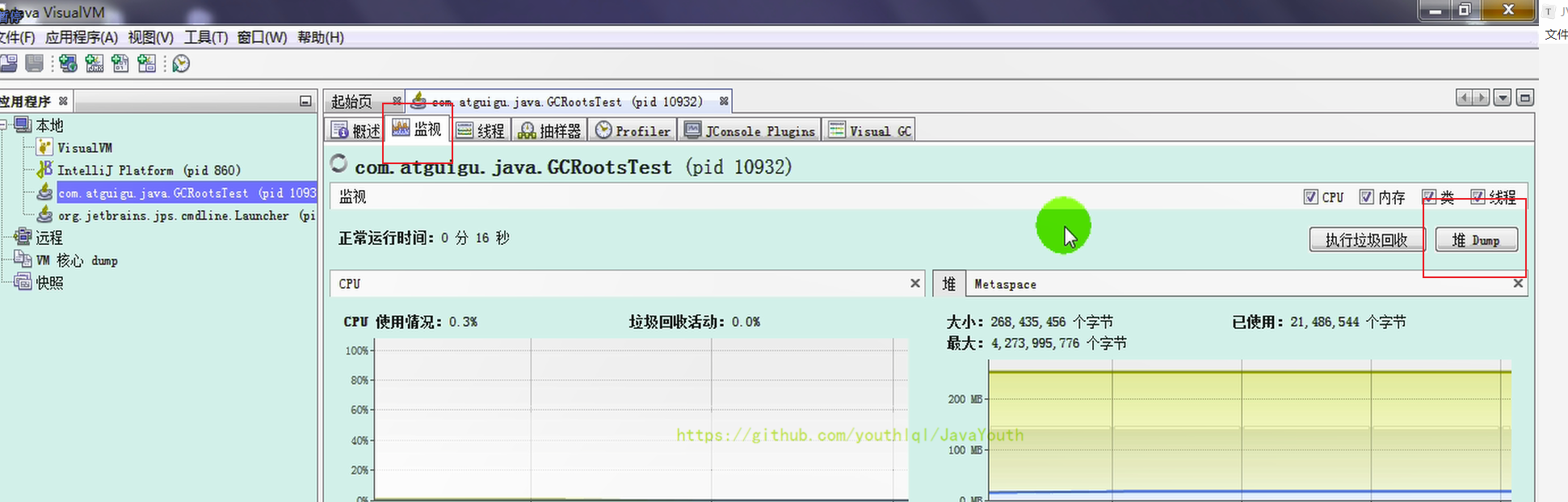
3、右键 --> 另存为即可
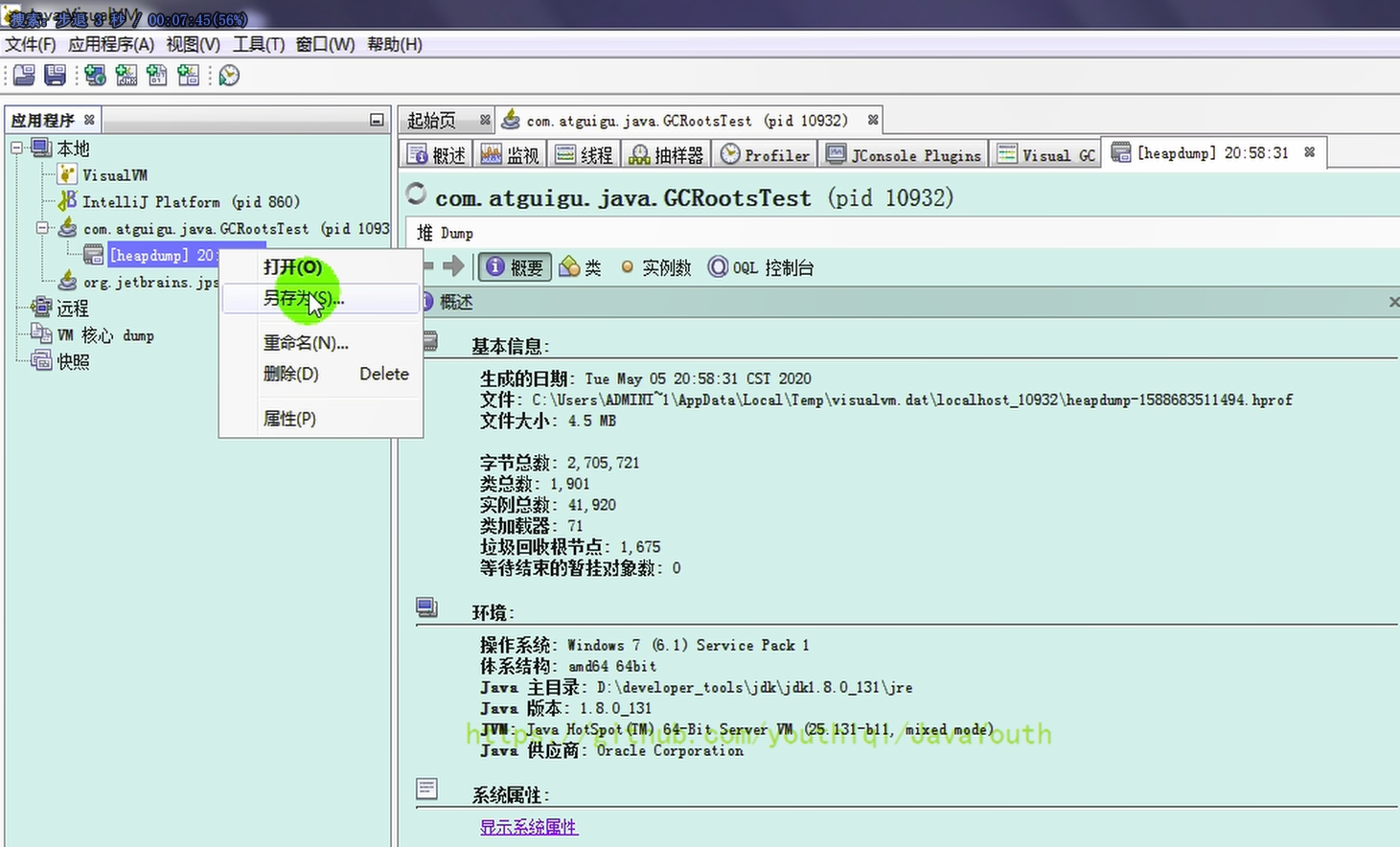
4、输入命令,继续执行程序
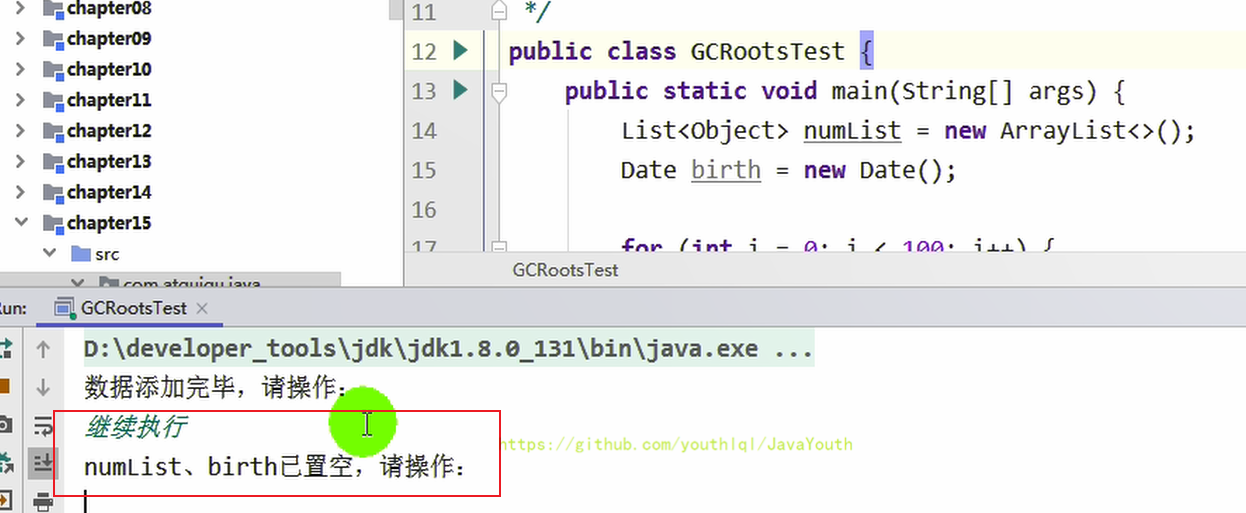
5、我们接着捕获第二张堆内存快照
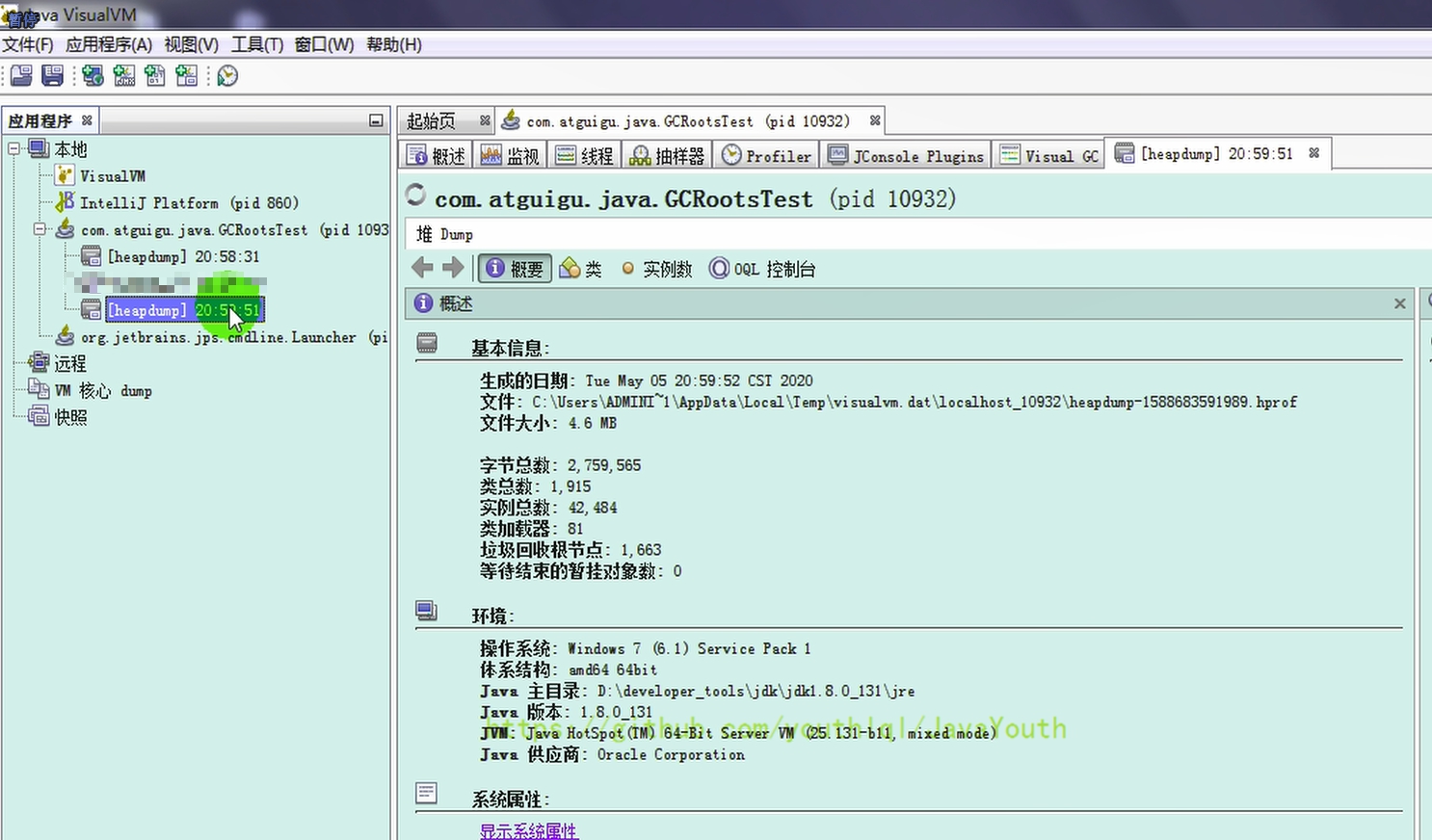
1、打开 MAT ,选择File --> Open File,打开刚刚的两个dump文件,我们先打开第一个dump文件
点击Open Heap Dump也行
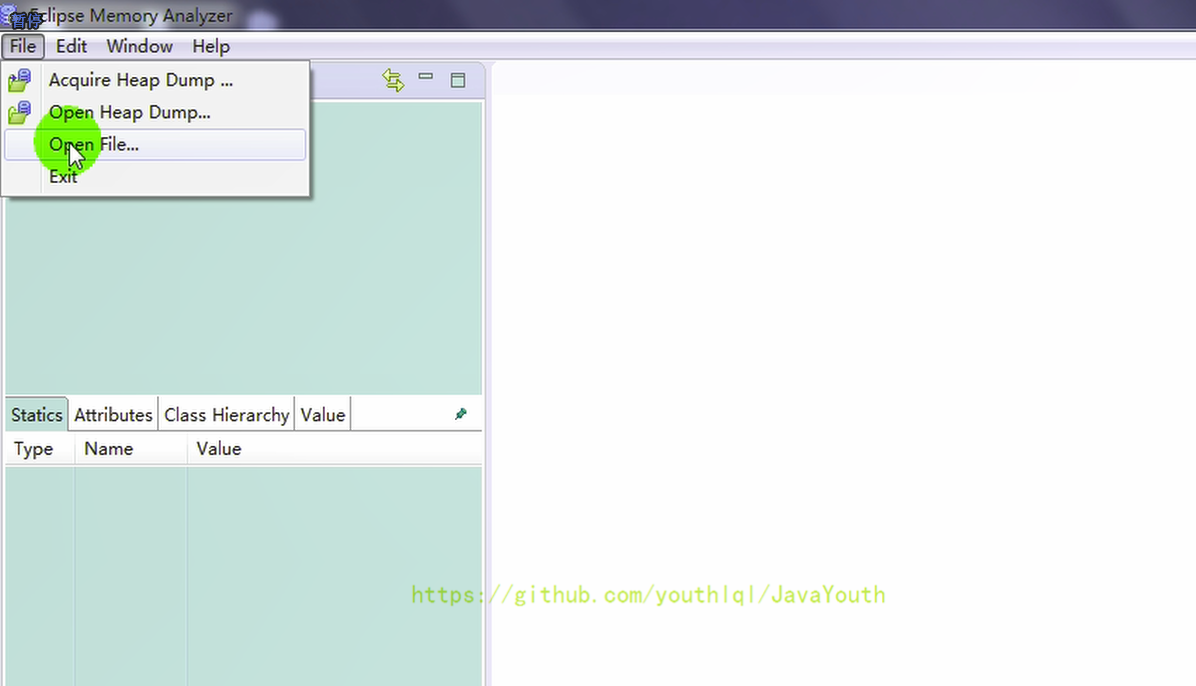
2、选择Java Basics --> GC Roots
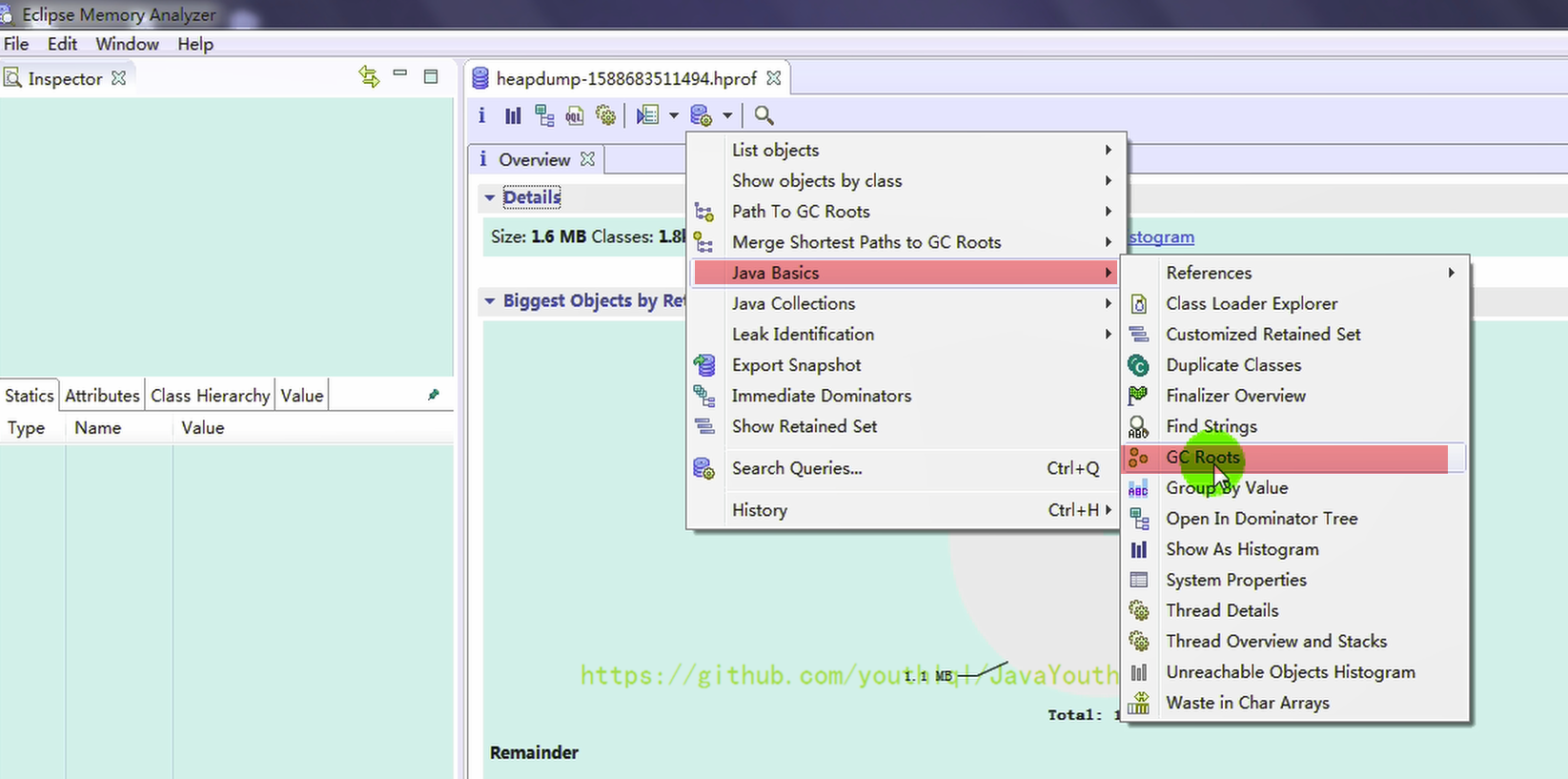
3、第一次捕捉堆内存快照时,GC Roots 中包含我们定义的两个局部变量,类型分别为 ArrayList 和 Date,Total:21
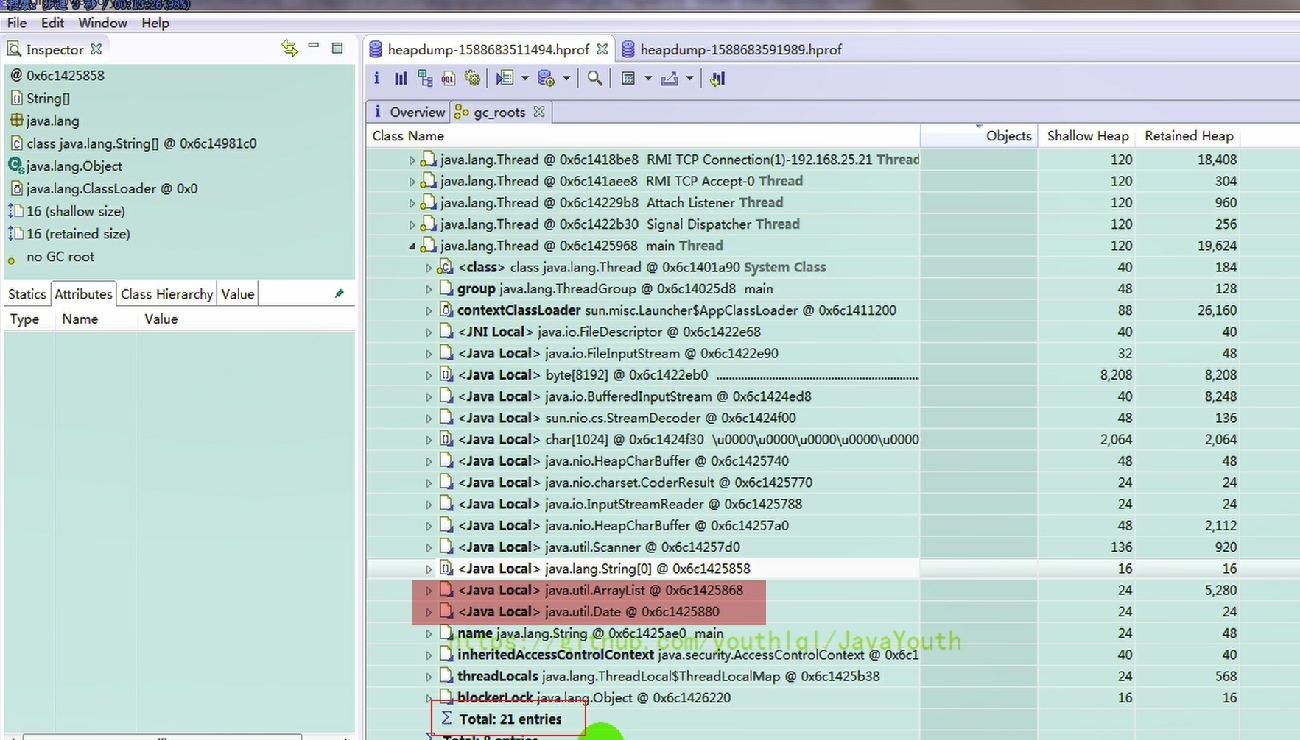
4、打开第二个dump文件,第二次捕获内存快照时,由于两个局部变量引用的对象被释放,所以这两个局部变量不再作为 GC Roots ,从 Total Entries = 19 也可以看出(少了两个 GC Roots)
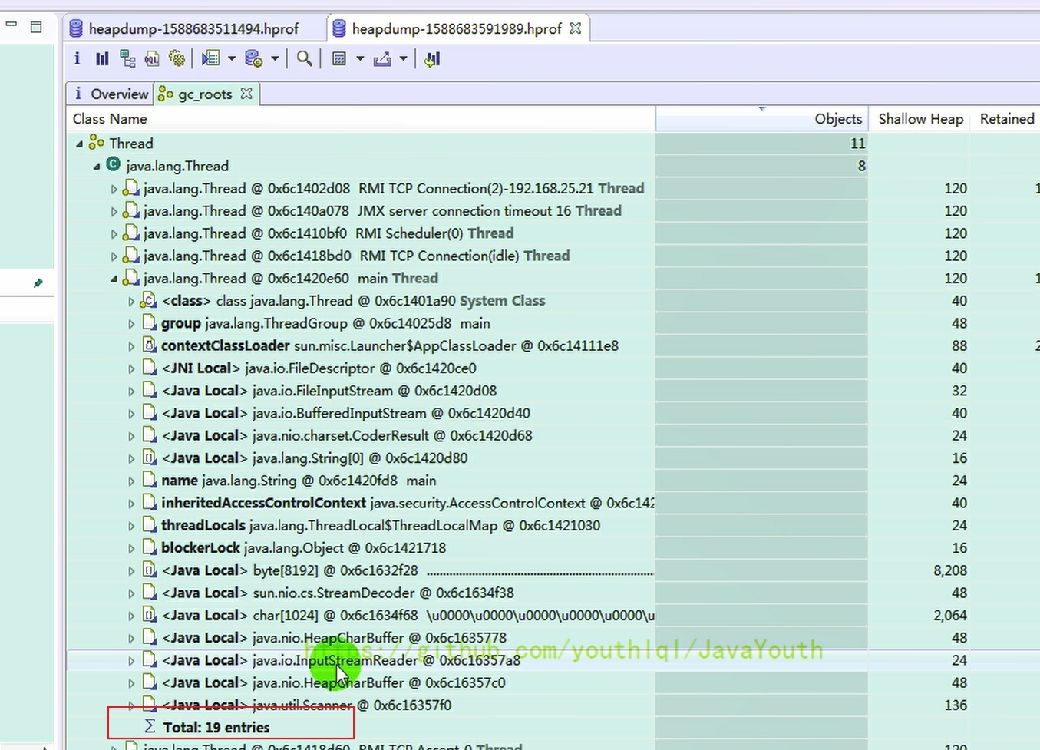
1、在实际开发中,我们很少会查看所有的GC Roots。一般都是查看某一个或几个对象的GC Root是哪个,这个过程叫GC Roots 溯源
2、下面我们使用使用 JProfiler 进行 GC Roots 溯源演示
依然用下面这个代码
public class GCRootsTest {
public static void main(String[] args) {
List<Object> numList = new ArrayList<>();
Date birth = new Date();
for (int i = 0; i < 100; i++) {
numList.add(String.valueOf(i));
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("数据添加完毕,请操作:");
new Scanner(System.in).next();
numList = null;
birth = null;
System.out.println("numList、birth已置空,请操作:");
new Scanner(System.in).next();
System.out.println("结束");
}
}
1、
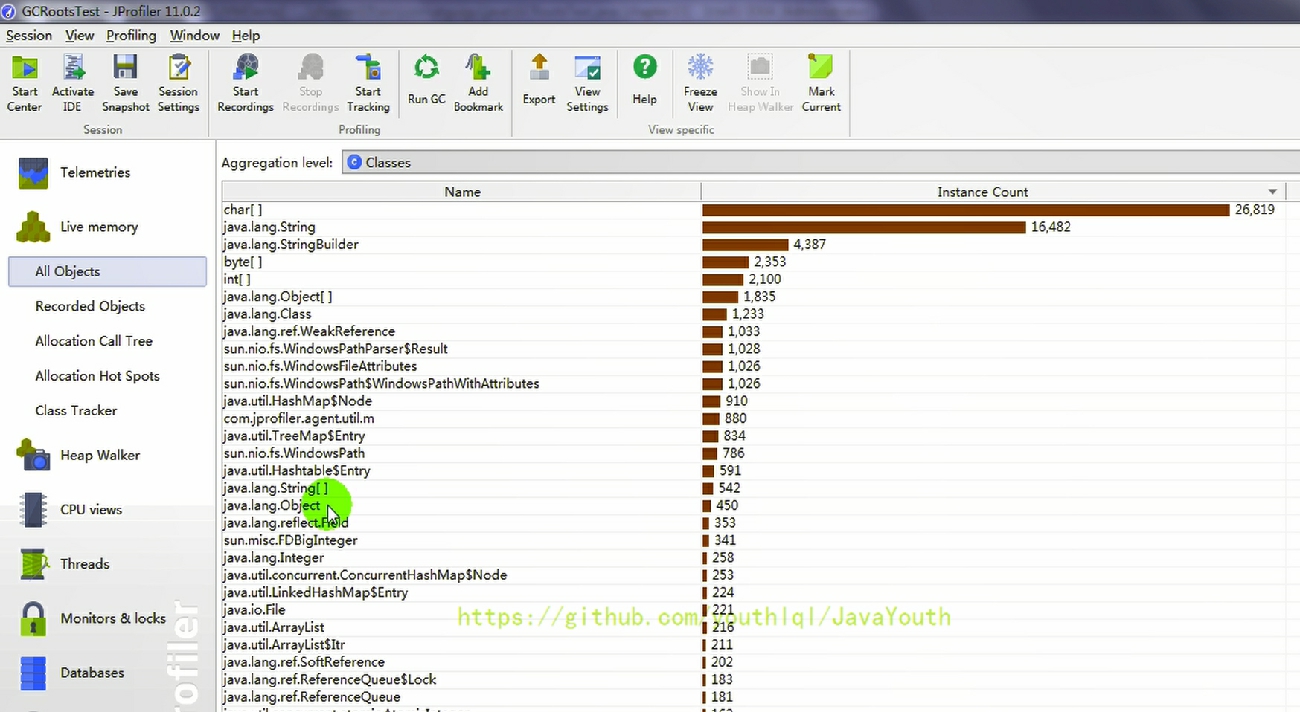
2、
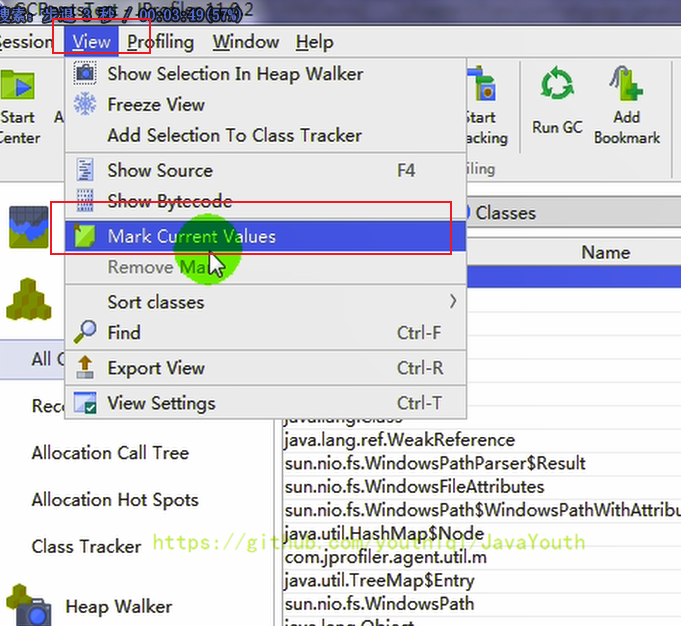
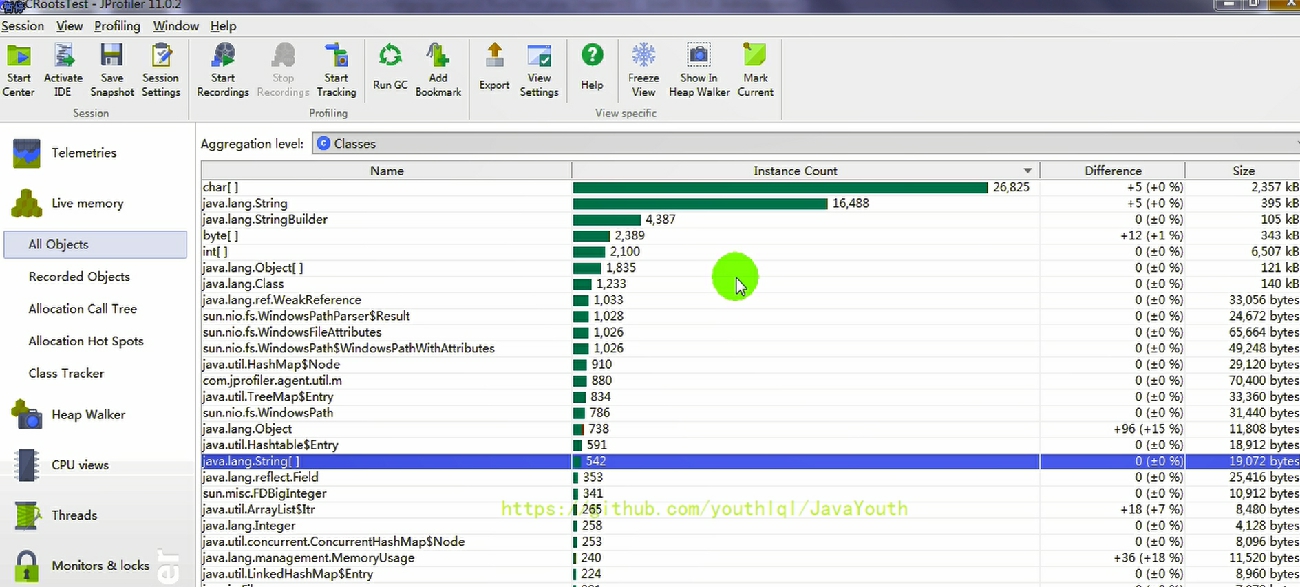
可以发现颜色变绿了,可以动态的看变化
3、右击对象,选择 Show Selection In Heap Walker,单独的查看某个对象
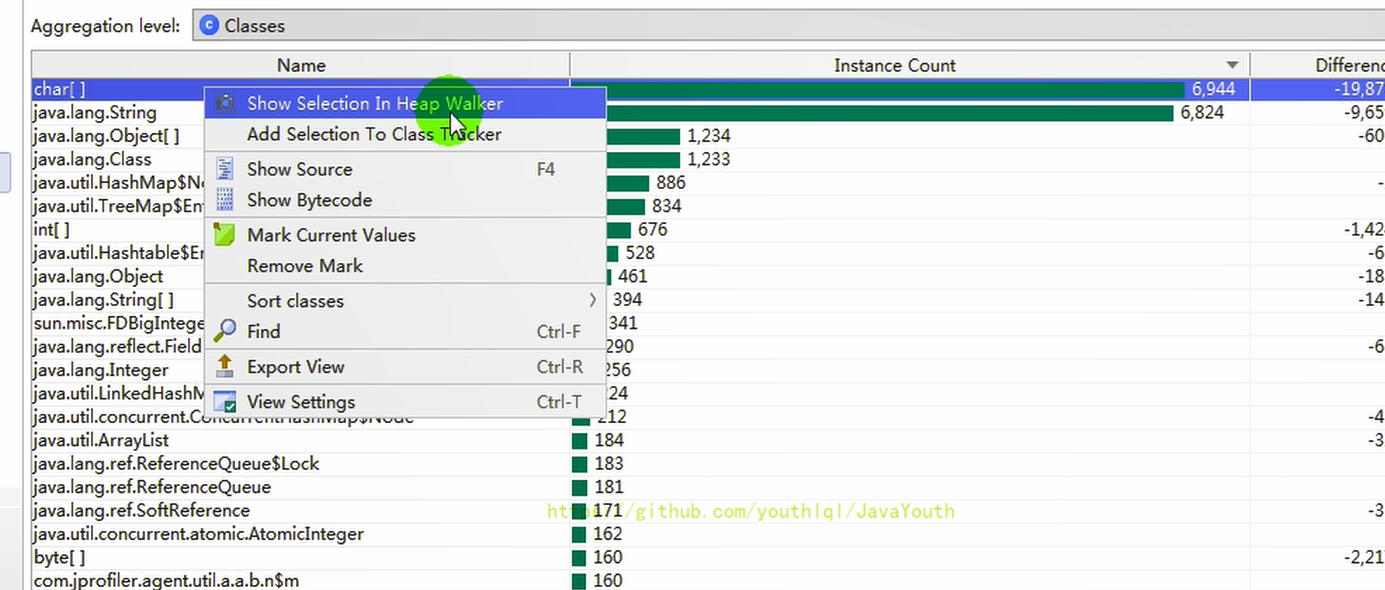
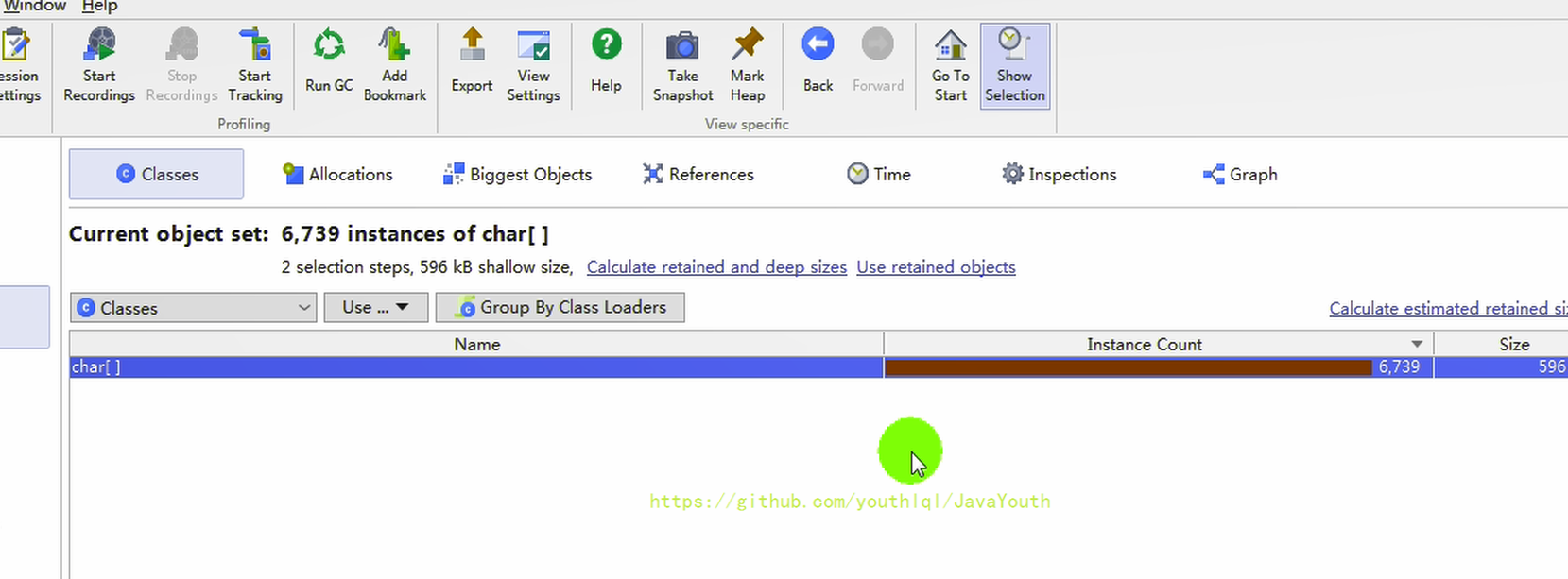
4、选择Incoming References,表示追寻 GC Roots 的源头
点击Show Paths To GC Roots,在弹出界面中选择默认设置即可
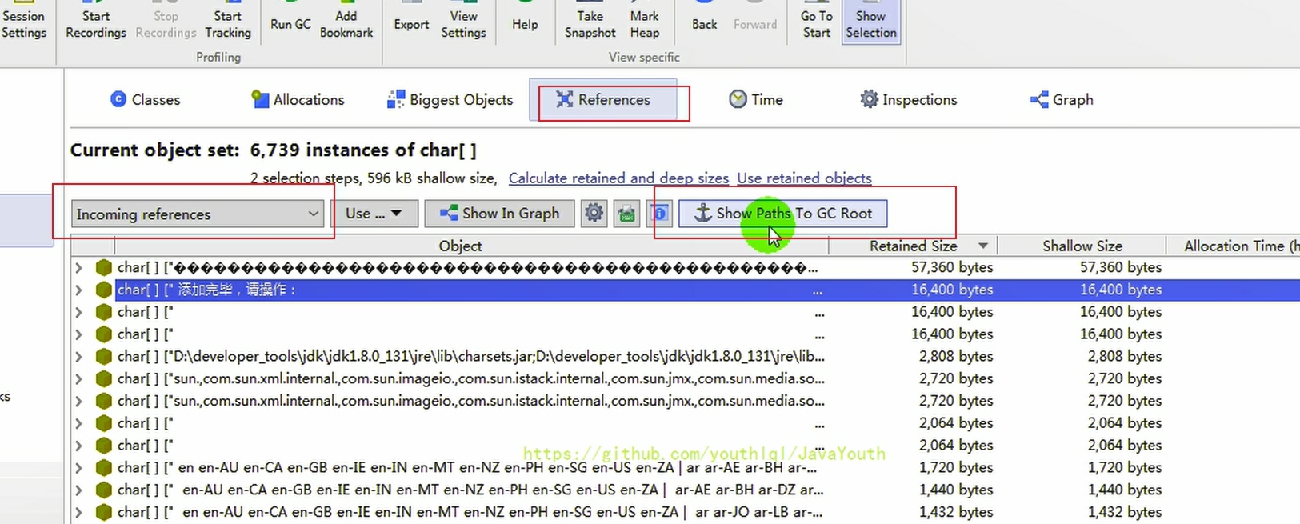
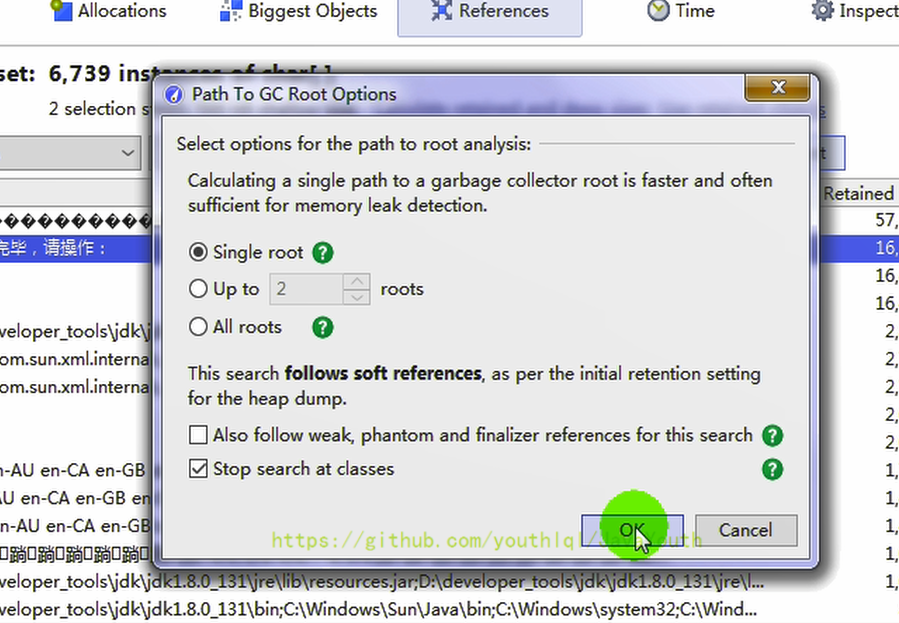
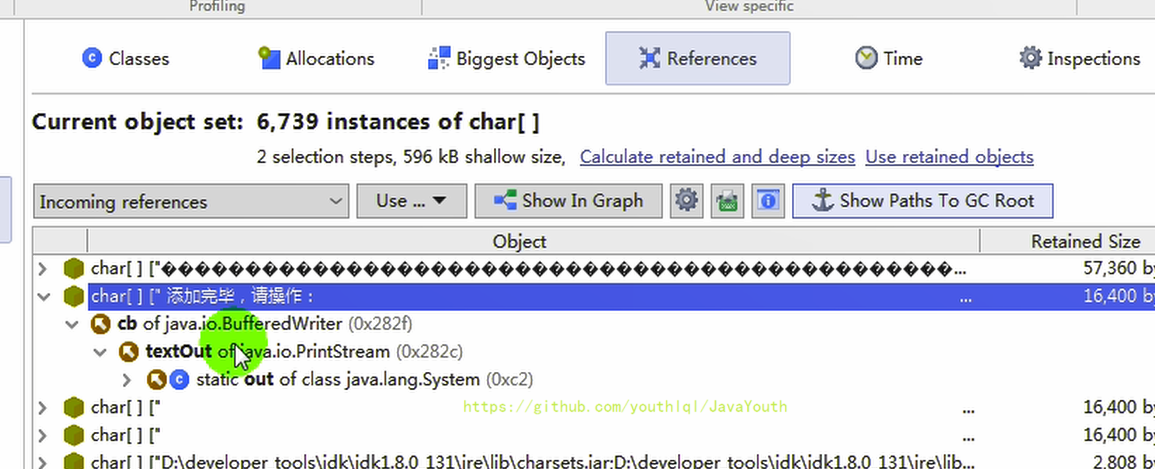
这里是简单的讲一下,后面篇章会详解
/**
* -Xms8m -Xmx8m
* -XX:+HeapDumpOnOutOfMemoryError 这个参数的意思是当程序出现OOM的时候就会在当前工程目录生成一个dump文件
*/
public class HeapOOM {
byte[] buffer = new byte[1 * 1024 * 1024];//1MB
public static void main(String[] args) {
ArrayList<HeapOOM> list = new ArrayList<>();
int count = 0;
try{
while(true){
list.add(new HeapOOM());
count++;
}
}catch (Throwable e){
System.out.println("count = " + count);
e.printStackTrace();
}
}
}
程序输出日志
com.atguigu.java.HeapOOM
java.lang.OutOfMemoryError: Java heap space
Dumping heap to java_pid14608.hprof ...
java.lang.OutOfMemoryError: Java heap space
at com.atguigu.java.HeapOOM.<init>(HeapOOM.java:12)
at com.atguigu.java.HeapOOM.main(HeapOOM.java:20)
Heap dump file created [7797849 bytes in 0.010 secs]
count = 6
打开这个dump文件
1、看这个超大对象
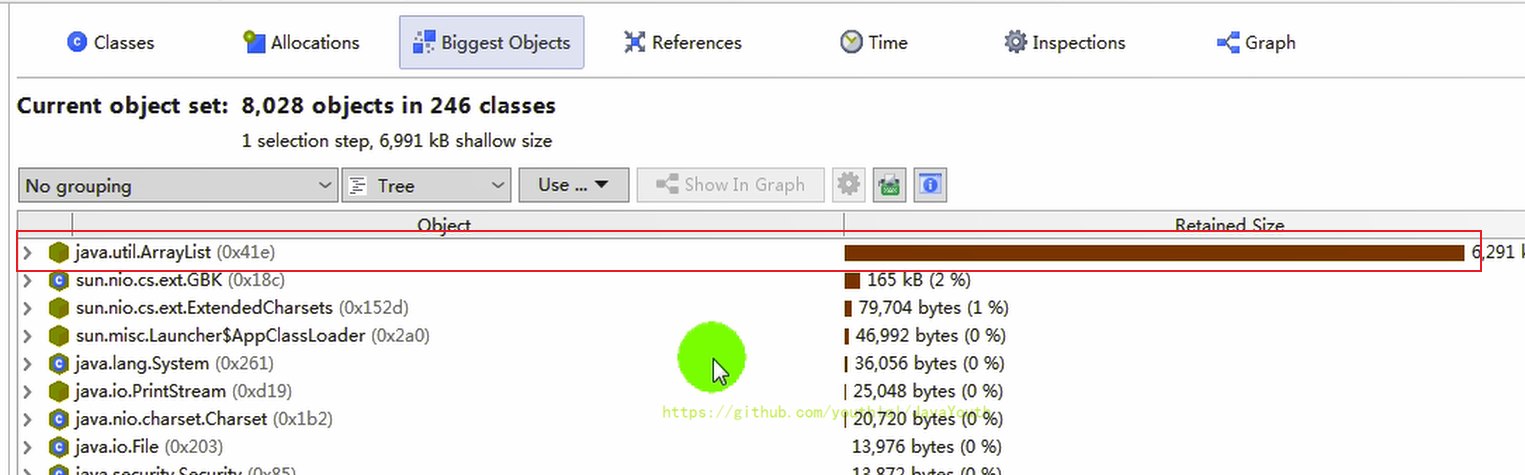
2、揪出 main() 线程中出问题的代码
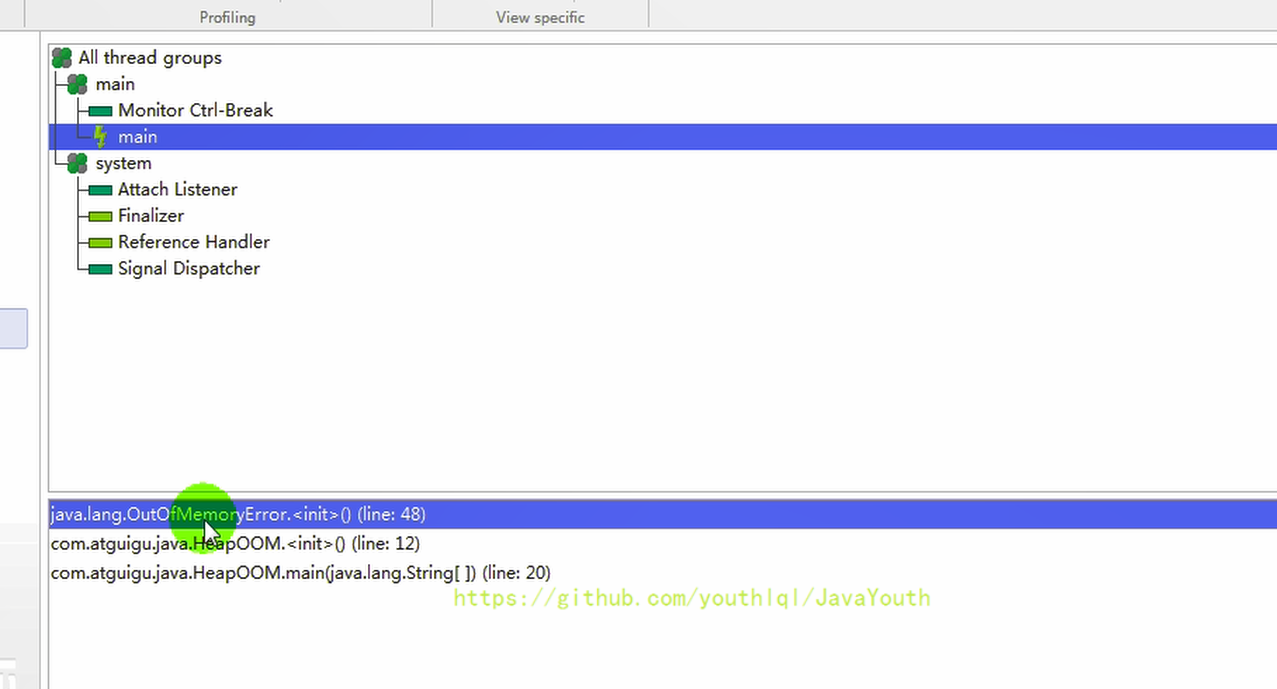
垃圾清除阶段
背景
标记-清除算法(Mark-Sweep)是一种非常基础和常见的垃圾收集算法,该算法被J.McCarthy等人在1960年提出并并应用于Lisp语言。
执行过程
当堆中的有效内存空间(available memory)被耗尽的时候,就会停止整个程序(也被称为stop the world),然后进行两项工作,第一项则是标记,第二项则是清除
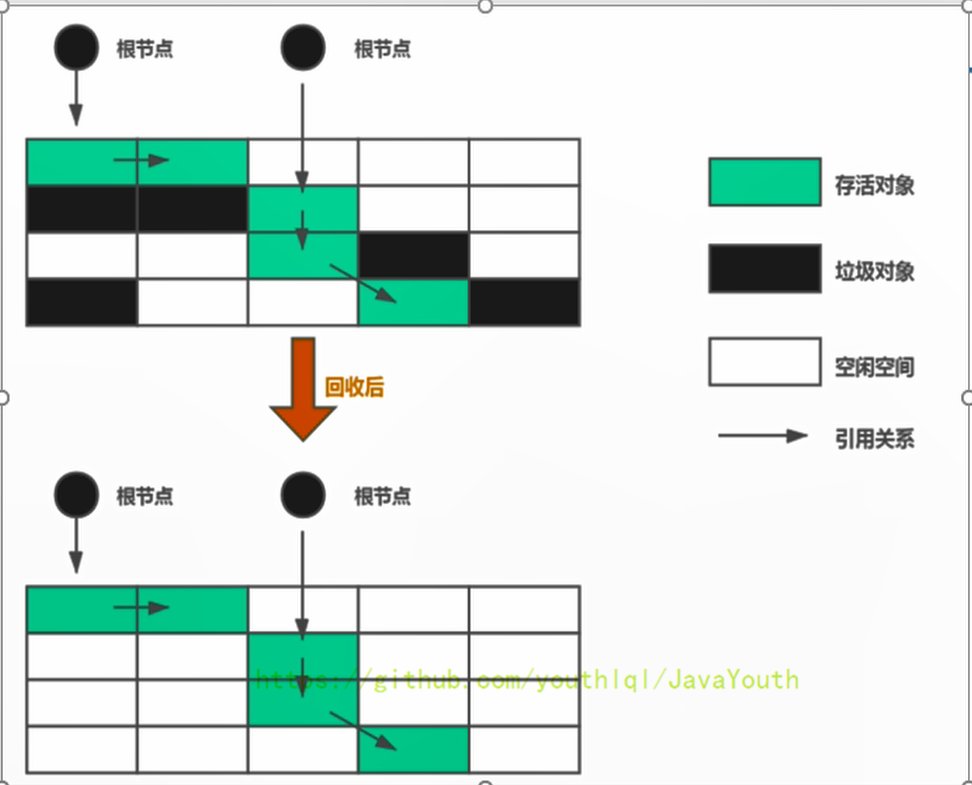
标记-清除算法的缺点
注意:何为清除?
这里所谓的清除并不是真的置空,而是把需要清除的对象地址保存在空闲的地址列表里。下次有新对象需要加载时,判断垃圾的位置空间是否够,如果够,就存放(也就是覆盖原有的地址)。
关于空闲列表是在为对象分配内存的时候提过:
背景
核心思想
将活着的内存空间分为两块,每次只使用其中一块,在垃圾回收时将正在使用的内存中的存活对象复制到未被使用的内存块中,之后清除正在使用的内存块中的所有对象,交换两个内存的角色,最后完成垃圾回收
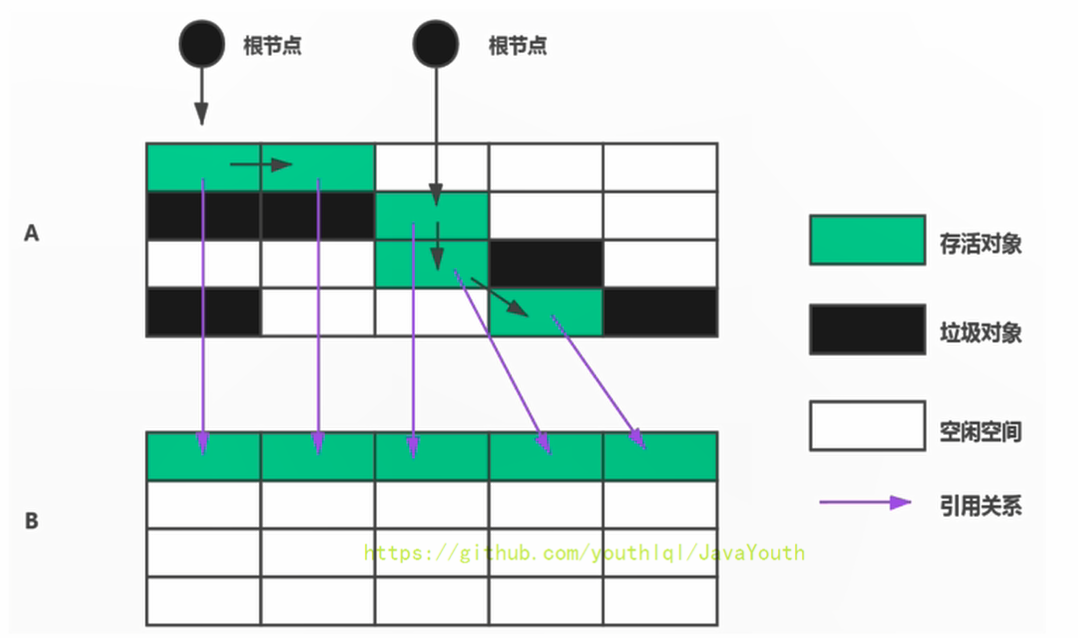
新生代里面就用到了复制算法,Eden区和S0区存活对象整体复制到S1区
复制算法的优缺点
优点
缺点
复制算法的应用场景
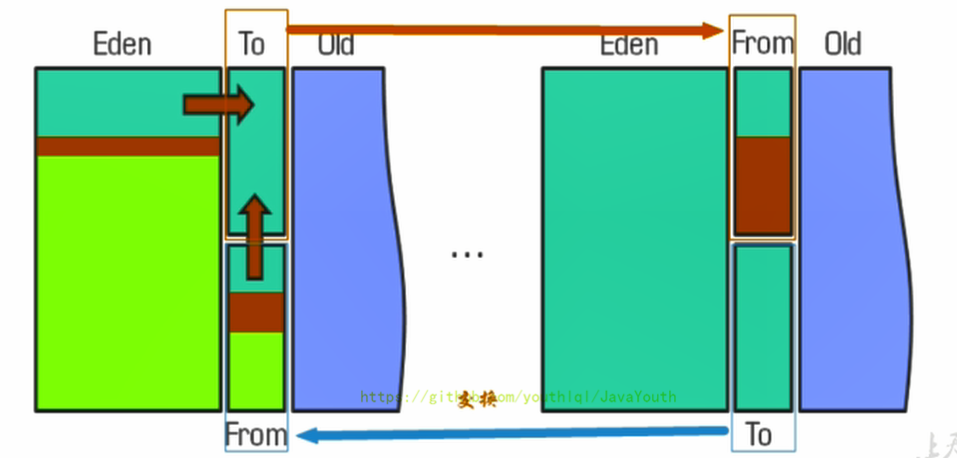
标记-压缩(或标记-整理、Mark - Compact)算法
背景
执行过程
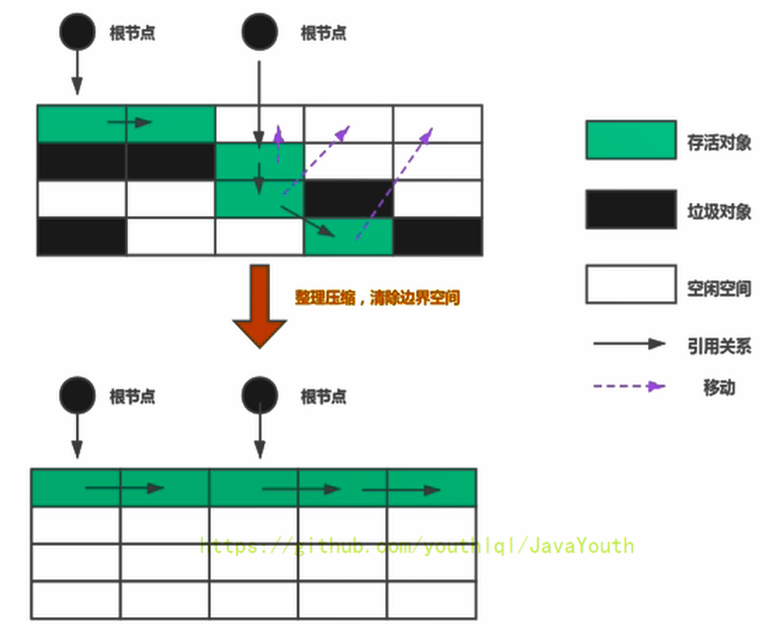
标记-压缩算法与标记-清除算法的比较
标记-压缩算法的优缺点
优点
缺点
对比三种清除阶段的算法
Q:难道就没有一种最优的算法吗?
A:无,没有最好的算法,只有最合适的算法
为什么要使用分代收集算法
目前几乎所有的GC都采用分代手机算法执行垃圾回收的
在HotSpot中,基于分代的概念,GC所使用的内存回收算法必须结合年轻代和老年代各自的特点。
上述现有的算法,在垃圾回收过程中,应用软件将处于一种Stop the World的状态。在Stop the World状态下,应用程序所有的线程都会挂起,暂停一切正常的工作,等待垃圾回收的完成。如果垃圾回收时间过长,应用程序会被挂起很久,将严重影响用户体验或者系统的稳定性。为了解决这个问题,即对实时垃圾收集算法的研究直接导致了增量收集(Incremental Collecting)算法的诞生。
增量收集算法基本思想
增量收集算法的缺点
使用这种方式,由于在垃圾回收过程中,间断性地还执行了应用程序代码,所以能减少系统的停顿时间。但是,因为线程切换和上下文转换的消耗,会使得垃圾回收的总体成本上升,造成系统吞吐量的下降。
主要针对G1收集器来说的
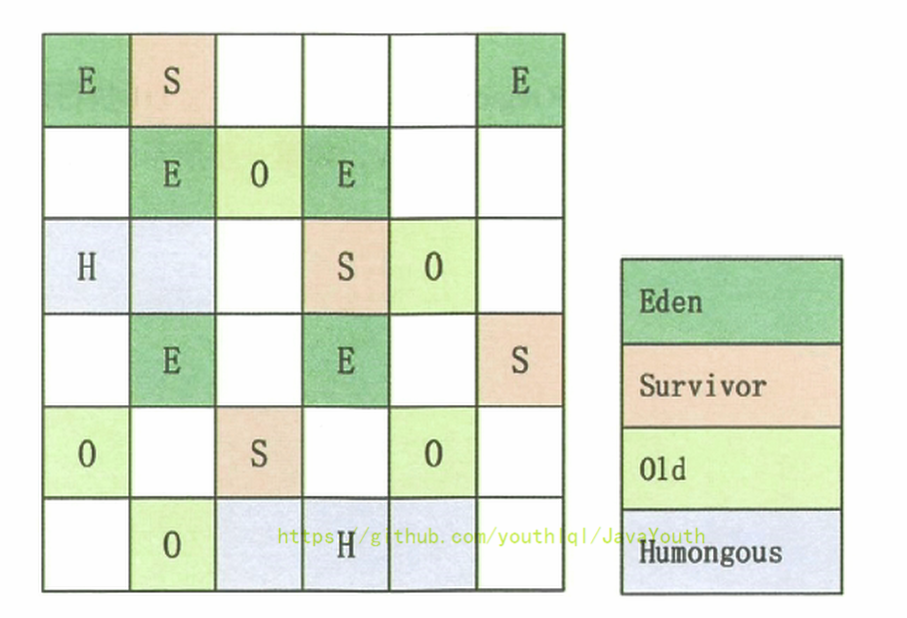
注意,这些只是基本的算法思路,实际GC实现过程要复杂的多,目前还在发展中的前沿GC都是复合算法,并且并行和并发兼备。