准备工作
- 理解java中的面向对象
- 理解GUI编程
- 理解多线程的使用
- java中集合的使用
实体类
public class BackGround {
private int x;
private int y;
private int width;
private int height;
private ImageIcon icon=new ImageIcon("src\\image\\bg.jpg");
public BackGround(int x, int y) {
this.x = x;
this.y = y;
this.width = icon.getIconWidth();
this.height = icon.getIconHeight();
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public ImageIcon getIcon() {
return icon;
}
public void move(){
this.y+=1;
}
}
public class Bomb {
private int x;
private int y;
public int time;
private ImageIcon icon=new ImageIcon("src\\image\\bomb.png");
public void setTime(int time) {
this.time = time;
}
public Bomb(int x, int y, int time) {
this.x = x;
this.y = y;
this.time=time;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public ImageIcon getIcon() {
return icon;
}
}
public class Bullet {
private int x;
private int y;
private int width;
private int height;
private ImageIcon icon=new ImageIcon("src\\image\\bullet.png");
public Bullet(int x, int y) {
this.x = x;
this.y = y;
this.width = icon.getIconWidth();
this.height = icon.getIconHeight();
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public ImageIcon getIcon() {
return icon;
}
public void move(){
this.y-=3;
}
}
public class Enemy {
private int x;
private int y;
private int width;
private int height;
private ImageIcon icon=new ImageIcon("src\\image\\enemy.png");
public Enemy(int x, int y) {
this.x = x;
this.y = y;
this.width = icon.getIconWidth();
this.height = icon.getIconHeight();
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public ImageIcon getIcon() {
return icon;
}
public void move(){
this.y+=4;
}
}
public class HeroDestroy {
private int x;
private int y;
private int width;
private int height;
private ImageIcon icon=new ImageIcon("src/image/hero_destory.png");
public HeroDestroy(int x, int y) {
this.x = x;
this.y = y;
this.width = icon.getIconWidth();
this.height = icon.getIconHeight();
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public ImageIcon getIcon() {
return icon;
}
}
public class HeroPlane {
private int x;
private int y;
private int width;
private int height;
private ImageIcon icon=new ImageIcon("src\\image\\hero.png");
public HeroPlane(int x, int y) {
this.x = x;
this.y = y;
this.width = icon.getIconWidth();
this.height = icon.getIconHeight();
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
public ImageIcon getIcon() {
return icon;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
}
public class LoginFrame extends JFrame {
private JLabel label=new JLabel();
public LoginFrame(){
label.setBounds(0,0,480,800);
label.setIcon(new ImageIcon("src/image/login.jpg"));
this.add(label);
label.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
super.mouseClicked(e);
if (e.getX()>=120 &&e.getX()<=355 &&e.getY()>=565 && e.getY()<=630){
LoginFrame.this.setVisible(false);
new Thread(new GameJFrame("飞机大战")).start();
}
}
});
label.addMouseMotionListener(new MouseAdapter() {
@Override
public void mouseMoved(MouseEvent e) {
super.mouseMoved(e);
if (e.getX()>=120 &&e.getX()<=355 &&e.getY()>=565 && e.getY()<=630) {
label.setCursor(Cursor.getPredefinedCursor(Cursor.HAND_CURSOR));
}else {
label.setCursor(Cursor.getDefaultCursor());
}
}
});
this.setBounds(750,100,480,800);
this.setVisible(true);
}
}
public class GameJFrame extends JFrame implements Runnable{
private GameJPanel panel=new GameJPanel();
public GameJFrame(String title){
super(title);
this.addElements();
this.addAction();
this.setJFrameSelf();
}
public void addElements(){
this.add(panel);
}
public void addAction(){
this.addMouseMotionListener(panel);
}
public void setJFrameSelf(){
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setBounds(750,100,480,700);
this.setResizable(false);
}
@Override
public void run() {
panel.init();
}
}
public class GameJPanel extends JPanel implements MouseMotionListener {
private HeroPlane hero=new HeroPlane(200,520);
private ArrayList<Bullet> bullets=new ArrayList<>();
private ArrayList<Enemy> enemyArrayList=new ArrayList<>();
private ArrayList<BackGround> backGroundArrayList=new ArrayList<>();
private ArrayList<Bomb> bombArrayList=new ArrayList<>();
private HeroDestroy heroDestroy;
private int score=0;
public void init() {
int count = 0;
backGroundArrayList.add(new BackGround(0,0));
while (true) {
count++;
if (backGroundArrayList.size()==1){
backGroundArrayList.add(new BackGround(0,-700));
}
for (int i = 0; i <backGroundArrayList.size() ; i++) {
BackGround backGround = backGroundArrayList.get(i);
if (backGround!=null){
backGround.move();
if (backGround.getY()>700){
backGroundArrayList.remove(backGround);
}
}
}
if (count%20==0) {
bullets.add(new Bullet(hero.getX() + 45, hero.getY() - 15));
}
for (int i = 0; i <bullets.size() ; i++) {
Bullet bullet = bullets.get(i);
if (bullets!=null){
bullet.move();
if (bullet.getY()<-15){
bullets.remove(bullet);
}
}
}
if (count%80==0){
enemyArrayList.add(new Enemy((int)(Math.random()*430),-30));
}
for (int i = 0; i <enemyArrayList.size() ; i++) {
Enemy enemy = enemyArrayList.get(i);
if (enemy!=null){
enemy.move();
if (enemy.getY()>700){
enemyArrayList.remove(enemy);
}
for (int j = 0; j <bullets.size() ; j++) {
Bullet bullet = bullets.get(j);
if (bullet!=null){
if (isHit(enemy,bullet)){
bombArrayList.add(new Bomb(enemy.getX(),enemy.getY(),0));
enemyArrayList.remove(enemy);
bullets.remove(bullet);
score+=10;
}
}
}
if (isHit(enemy,hero)){
bombArrayList.add(new Bomb(enemy.getX(),enemy.getY(),0));
enemyArrayList.remove(enemy);
heroDestroy =new HeroDestroy(hero.getX(),hero.getY());
hero=null;
JOptionPane.showMessageDialog(this,"游戏结束,您的得分为"+score);
return;
}
}
}
for (int i = 0; i <bombArrayList.size() ; i++) {
Bomb bomb = bombArrayList.get(i);
bomb.setTime(bomb.time+1);
if (bomb!=null && bomb.time==10){
bombArrayList.remove(bomb);
}
}
repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public boolean isHit(Enemy enemy,Bullet bullet){
Rectangle renemy=new Rectangle(enemy.getX(),enemy.getY(),enemy.getWidth(),enemy.getHeight());
Rectangle benemy=new Rectangle(bullet.getX(),bullet.getY(),bullet.getWidth(),bullet.getHeight());
return renemy.intersects(benemy);
}
public boolean isHit(Enemy enemy,HeroPlane hero){
Rectangle renemy=new Rectangle(enemy.getX(),enemy.getY(),enemy.getWidth(),enemy.getHeight());
Rectangle rhero=new Rectangle(hero.getX(),hero.getY(),hero.getWidth(),hero.getHeight());
return renemy.intersects(rhero);
}
@Override
public void paint(Graphics g) {
super.paint(g);
for (int i = 0; i <backGroundArrayList.size() ; i++) {
BackGround backGround = backGroundArrayList.get(i);
if (backGround!=null){
g.drawImage(backGround.getIcon().getImage(),backGround.getX(),backGround.getY(),null);
}
}
if (hero!=null) {
g.drawImage(hero.getIcon().getImage(), hero.getX(), hero.getY(), null);
}
for (int i = 0; i <bullets.size() ; i++) {
Bullet bullet = bullets.get(i);
if (bullet!=null){
g.drawImage(bullet.getIcon().getImage(),bullet.getX(),bullet.getY(),null);
}
}
for (int i = 0; i <enemyArrayList.size() ; i++) {
Enemy enemy = enemyArrayList.get(i);
if (enemy!=null){
g.drawImage(enemy.getIcon().getImage(),enemy.getX(),enemy.getY(),null);
}
}
for (int i = 0; i <bombArrayList.size() ; i++) {
Bomb bomb = bombArrayList.get(i);
if (bomb!=null){
g.drawImage(bomb.getIcon().getImage(),bomb.getX(),bomb.getY(),null);
}
}
if (heroDestroy !=null){
g.drawImage(heroDestroy.getIcon().getImage(), heroDestroy.getX(), heroDestroy.getY(),null);
}
if (hero!=null){
setFont(new Font("宋体",Font.BOLD,24));
g.drawString("当前积分"+score,10,30);
}
}
@Override
public void mouseDragged(MouseEvent e) {
}
@Override
public void mouseMoved(MouseEvent e) {
int x = e.getX();
int y = e.getY();
if (hero!=null){
if (x>0 && x<480) {
hero.setX(x - 50);
}
if (y>100 && y<650) {
hero.setY(y - 100);
}
}
repaint();
}
}
public class StartTest {
public static void main(String[] args) {
new LoginFrame();
}
}
图片素材

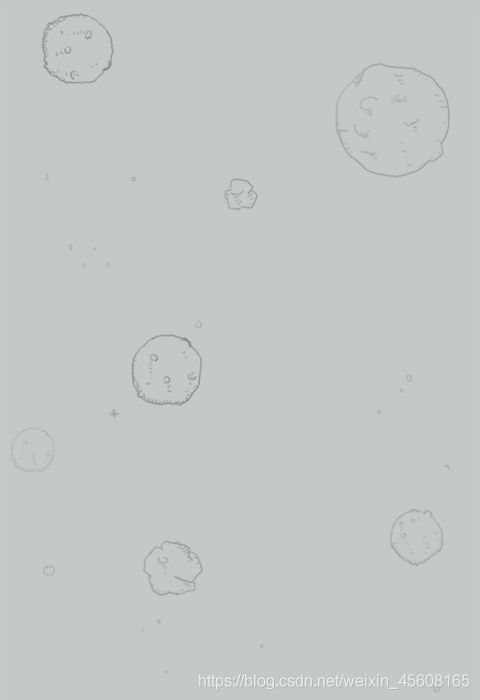
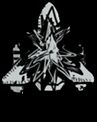
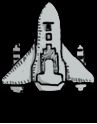
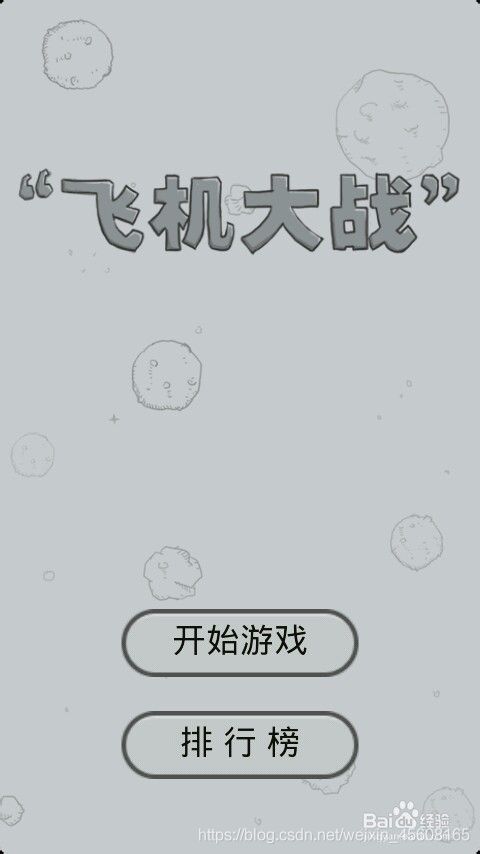

