百度人脸识别
1 .创建应用,拿到APPID、AK、SK
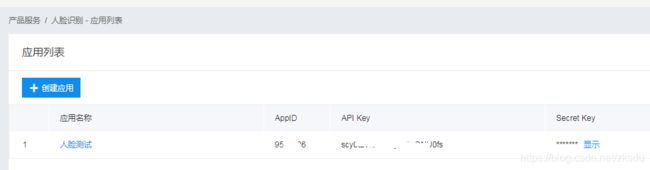
2 .下载并导入jar包
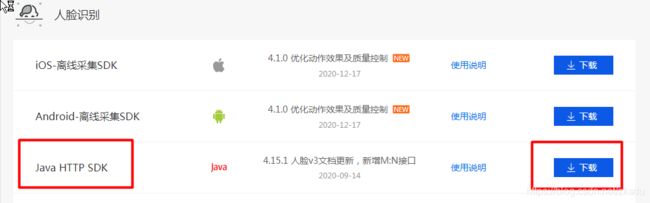
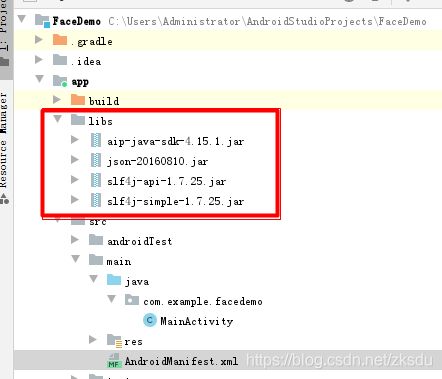
在界面添加一个Button 实现点击事件
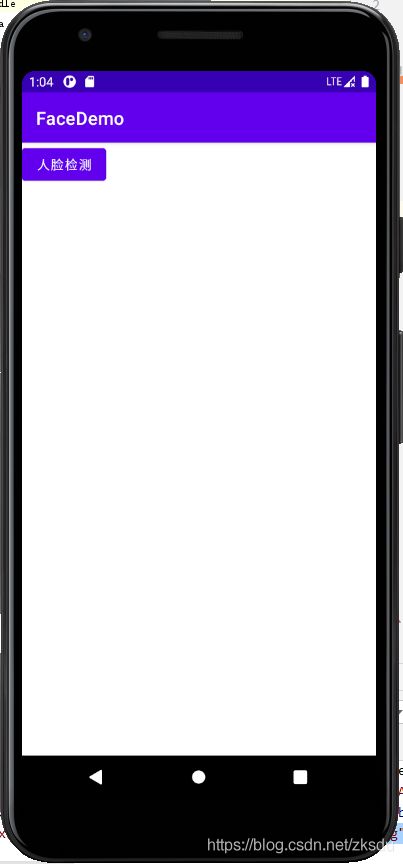
Java代码如下
package com.example.facedemo;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import android.Manifest;
import android.app.Activity;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.util.Base64;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import com.baidu.aip.face.AipFace;
import org.json.JSONObject;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.HashMap;
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_EXTERNAL_STORAGE = 1;
private static final int MSG_SHOW = 1001;
private static String[] PERMISSIONS_STORAGE = {
Manifest.permission.READ_EXTERNAL_STORAGE,
Manifest.permission.WRITE_EXTERNAL_STORAGE};
public static final String APP_ID = " ";
public static final String API_KEY = " ";
public static final String SECRET_KEY = " ";
private Button btn_face_detect;
private FaveView faveView;
private Handler handler;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
verifyStoragePermissions(this);
handler = new Handler(new Handler.Callback() {
@Override
public boolean handleMessage(@NonNull Message msg) {
if(msg.what == MSG_SHOW){
JSONObject detect = (JSONObject) msg.obj;
faveView.showFace(detect);
return true;
}
return false;
}
});
faveView = findViewById(R.id.face_view);
String filePath= "/sdcard/DCIM/liuhaocun.jpeg";
Bitmap bitmap = BitmapFactory.decodeFile(filePath);
faveView.setImageBitmap(bitmap);
btn_face_detect = findViewById(R.id.face_detect);
btn_face_detect.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String imageType = "BASE64";
String filePath= "/sdcard/DCIM/liuhaocun.jpeg";
String image = ImageToBase64(filePath);
Log.e("Base64", image);
HashMap<String, String> options = new HashMap<String, String>();
options.put("face_field", "age");
options.put("max_face_num", "10");
options.put("face_type", "LIVE");
options.put("liveness_control", "LOW");
AipFace aipFace = new AipFace(APP_ID, API_KEY, SECRET_KEY);
new Thread(new Runnable() {
@Override
public void run() {
JSONObject detect = aipFace.detect(image, imageType, options);
Log.e("FACE", detect.toString());
Message msg = new Message();
msg.what = MSG_SHOW;
msg.obj = detect;
handler.sendMessage(msg);
}
}).start();
}
});
}
private static String ImageToBase64(String imgPath) {
byte[] data = null;
try {
InputStream in = new FileInputStream(imgPath);
data = new byte[in.available()];
in.read(data);
in.close();
} catch (IOException e) {
e.printStackTrace();
}
String str = Base64.encodeToString(data, Base64.NO_WRAP);
return str;
}
public static void verifyStoragePermissions(Activity activity) {
int permission = ActivityCompat.checkSelfPermission(activity,
Manifest.permission.WRITE_EXTERNAL_STORAGE);
if (permission != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(activity, PERMISSIONS_STORAGE,
REQUEST_EXTERNAL_STORAGE);
}
}
}
绘制脸
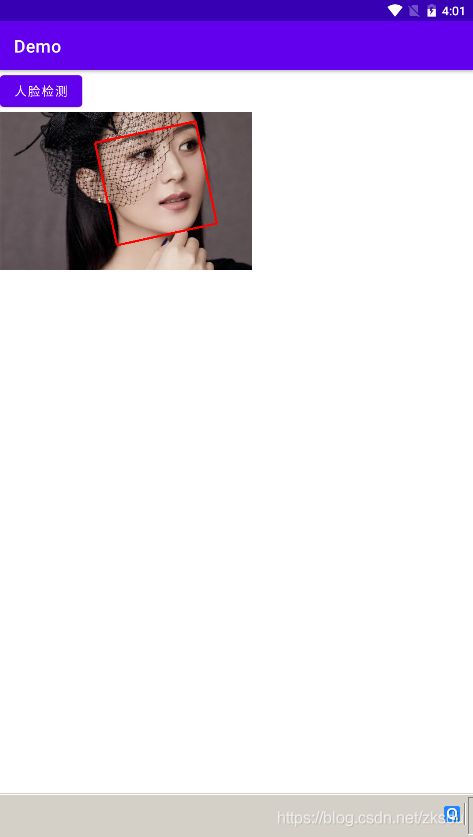
package com.example.demo;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Point;
import android.util.AttributeSet;
import android.util.Log;
import android.widget.ImageView;
import androidx.annotation.Nullable;
import com.google.gson.Gson;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.List;
public class FaceView extends androidx.appcompat.widget.AppCompatImageView {
List<Face_list> face_list;
Paint paint;
public FaceView(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
paint = new Paint();
paint.setColor(Color.RED);
paint.setStrokeWidth(5);
paint.setStyle(Paint.Style.STROKE);
}
public void showFace(JSONObject detect) throws JSONException {
Gson gson = new Gson();
FaceResult faceResult = gson.fromJson(detect.toString(), FaceResult.class);
face_list = faceResult.getResult().getFace_list();
int face_num = faceResult.getResult().getFace_num();
if(face_num > 0){
invalidate();
}
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
if(face_list == null){
return;
}
for (int i = 0; i < face_list.size(); i++) {
Location location = face_list.get(i).getLocation();
if (location != null) {
Point p1 = new Point((int) location.getLeft(), (int) location.getTop());
int x2 = (int) (p1.x + location.getWidth() * Math.cos(Math.PI * location.getRotation() / 180));
int y2 = (int) (p1.y + location.getWidth() * Math.sin(Math.PI * location.getRotation() / 180));
Point p2 = new Point(x2, y2);
canvas.drawLine(p1.x, p1.y, p2.x, p2.y, paint);
int x3 = (int) (x2 - location.getHeight() * Math.sin(Math.PI * location.getRotation() / 180));
int y3 = (int) (y2 + location.getHeight() * Math.cos(Math.PI * location.getRotation() / 180));
Point p3 = new Point(x3, y3);
canvas.drawLine(p2.x, p2.y, p3.x, p3.y, paint);
int x4 = (int) (location.getLeft() - location.getHeight() * Math.sin(Math.PI * location.getRotation() / 180));
int y4 = (int) (location.getTop() + location.getHeight() * Math.cos(Math.PI * location.getRotation() / 180));
Point p4 = new Point(x4, y4);
canvas.drawLine(p3.x, p3.y, p4.x, p4.y, paint);
canvas.drawLine(p1.x, p1.y, p4.x, p4.y, paint);
}
}
}
}
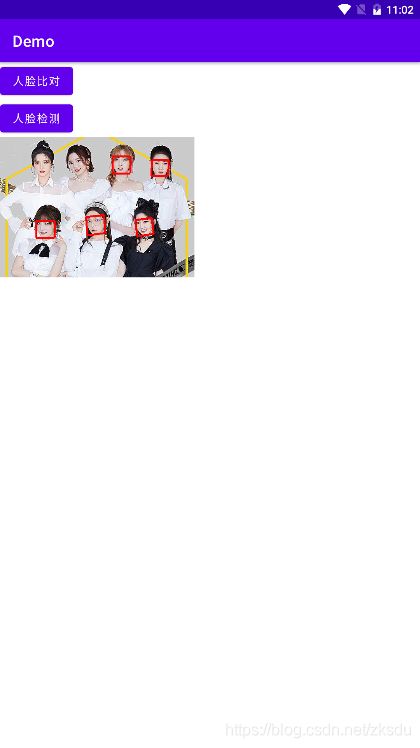
获取图片的Base64编码 抽取为一个方法
private String getImage64Str(String filePath) {
FileInputStream fileInputStream = null;
try {
fileInputStream = new FileInputStream(filePath);
byte[] bytes = new byte[fileInputStream.available()];
fileInputStream.read(bytes);
fileInputStream.close();
return Base64.encodeToString(bytes, Base64.NO_WRAP);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
人脸对比的代码
private void faceMatch(String filePath, String filePath2) {
AipFace client = new AipFace(APP_ID, API_KEY, SECRET_KEY);
String imgStr1 = getImage64Str(filePath);
String imgStr2 = getImage64Str(filePath2);
Log.e("FaceMatch", "imgStr1 = " + imgStr1);
Log.e("FaceMatch", "imgStr2 = " + imgStr2);
MatchRequest req1 = new MatchRequest(imgStr1, "BASE64");
MatchRequest req2 = new MatchRequest(imgStr2, "BASE64");
ArrayList<MatchRequest> matchRequestArrayList = new ArrayList<>();
matchRequestArrayList.add(req1);
matchRequestArrayList.add(req2);
JSONObject match = client.match(matchRequestArrayList);
Log.e("Match", match.toString());
}