React Native封装Form表单组件
为什么我们在移动端开发中急需要一个完善的Form表单组件尼?做过移动端开发的小伙伴们应该都清楚,一个完整的App项目中避免不了要有登录注册功能,用户信息,密码等一系列需要新用户填写信息的页面,这些页面的UI如果都是按部就班的去绘制,其实是做了很多无用功,因为大部分UI都长的差不多,就是有些文案类的略微不同,既然这样,我们将这些长的差不多的UI抽离成组件,在需要用到的地方,直接调用封装好的组件,这样极大的降低了编写代码量,大大的提高开发者的效率
Form表单支持的可配置项
- TextInput
- TextArea
- Button
- ButtonGroup
- CheckBox
- Radio
- Switch
- DatePicker
- CustomView
- Selector
- CustomAction
后续待添加的配置项
- 地区选择
- CustomSelector
- 用户图像
- 图片上传
- 短信验证码
- 登录注册
- 待补充
效果图
image
主要功能
后期完成登录注册、身份认证等表单信息页面的开发,我们只需要一个简单的配置文件即可搞定,不必每次相同的表单项都重新绘制一遍UI。对于哪些不常见的表单项,我们可以完全使用自定义UI来搞定,这样一来,我们在平时开发中的80%的表单需求都能轻松的搞定了
核心代码
renderFields(fields) {
let fieldCount = fields.length ? fields.length : 0
return fields.map((field, index) => {
let isLastField = true
if (fieldCount === index + 1 || fields[index + 1].title !== undefined) {
isLastField = false
}
if (field.title !== undefined && field.show !== false) {
return this.renderGroupForm(field)
} else {
let {show, type, label, subLabel, subTitle, ...other} = field
let otherProps = {...other}
Object.keys(otherProps).map((key, index) => {
if (key.indexOf('on') === 0 || key === 'callback' || key === 'dataFunc' || key === 'view') {
otherProps[key] = this.props[otherProps[key]]
}
})
let isEditable = !this.props.readOnly && !this._isReadOnly(field.readOnly)
if (show !== false) {
if (this.fieldsType[type]) {
if (type === 'TextInput') {
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
{
this.state[field.key] = value
this.setState(this.state)
let _obj = {}
_obj[field.key] = value
this.props.onChange && this.props.onChange(_obj)
}}
/>
)
} else if (type === 'Button') {
return (
)
} else if (type === 'ButtonGroup') {
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
{
field.items.map((item, index) => {
let _cloneBtn = deepClone(item)
Object.keys(_cloneBtn).map((key, index) => {
if (key.indexOf('on') === 0) {
_cloneBtn[key] = this.props[_cloneBtn[key]]
}
})
if (typeof _cloneBtn.selected === 'boolean') {
return (
)
} else {
return (
)
}
})
}
)
} else if (type === 'Switch') {
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
{
this.state[field.key] = value
this.setState(this.state)
let _obj = {}
_obj[field.key] = value
this.props.onChange && this.props.onChange(_obj)
}}
/>
)
} else if (type === 'View') {
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
{otherProps.view}
)
} else if (type === 'TextArea') {
let Comp = this.fieldsType[type]
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
this._component[field.key] = ref}
name={field.key}
value={this.state}
editable={isEditable}
{...field}
{...otherProps}
onChange={this.props.onChange && this.props.onChange()}
/>
)
} else {
let Comp = this.fieldsType[type]
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
this._component[field.key] = ref}
name={field.key}
value={this.state}
editable={isEditable}
{...field}
{...otherProps}
descValue={field.name}
onChange={this.props.onChange && this.props.onChange()}
/>
)
}
} else {
return (
{
label ?
{label}
{subLabel}
{
subTitle ?
{subTitle}
: null
}
: null
}
this._component[field.key] = ref}
name={field.key} value={this.state}
{...field}
{...otherProps}
editable={isEditable}
onChange={this.props.onChange}
descValue={field.name}
/>
)
}
}
}
})
}
详细的使用方式请参照项目源码OneM
https://github.com/guangqiang-liu/OneM
更多文章
- 作者React Native开源项目OneM【500+ star】地址(按照企业开发标准搭建框架完成开发的):https://github.com/guangqiang-liu/OneM:欢迎小伙伴们 star
- 作者主页:包含60多篇RN开发相关的技术文章http://www.jianshu.com/u/023338566ca5 欢迎小伙伴们:多多关注,多多点赞
- 作者React Native QQ技术交流群:620792950 欢迎小伙伴进群交流学习
- 友情提示:在开发中有遇到RN相关的技术问题,欢迎小伙伴加入交流群(620792950),在群里提问、互相交流学习。交流群也定期更新最新的RN学习资料给大家,谢谢大家支持!
小伙伴们扫下方二维码加入RN技术交流QQ群
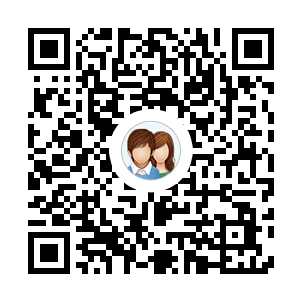
QQ群二维码,500+ RN工程师在等你加入哦