1. 捕获媒体数据的步骤:
(1) 查询CaptureDeviceManager,来定位你需要使用的媒体采集设备。
(2) 得到此设备的CaptureDeviceInfo实例。
(3) 从此CaptureDeviceInfo实例获得一个MediaLocator,并通过它来创建一个DataSource。
(4)用此DataSource创建一个Player或Processor。
(5) 启动此Player或Processor开始捕获媒体数据。
2.CaptureDeviceManager、CaptureDeviceInfo、MediaLocator
在JMF中,CaptureDeviceManager也是一个manager类,它提供给了一个列表,这个列表显示当前系统可以被使用的设备名称。同时CaptureDeviceManager可以通过查询的方法对设备进行定位并返回设备的配置信息对象CaptureDeviceInfo,它也可以通过注册的方法向列表加入一个新的设备信息,以便为JMF使用。
设备可通过CaptureDeviceManager的getDevice()方法直接获得设备控制权,设备的控制权一旦得到,就可以以此设备作为一个MediaLocator,可以通过CaptureDeviceInfo的getLocator()方法得到。
3.JMF识别的音频采集设备
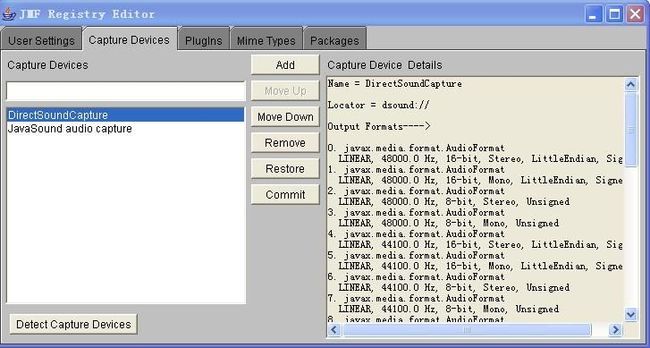
4.一个实例实现音频捕获
实例有两个文件组成。CaptureDemo.java实现
①查询、获得音频采集设备。
②捕获音频。
③将音频保存到本地文件foo.wav。
StateHelper实现处理器(processor)的状态控制管理。以下为流程图:
import java.util.Vector;
import javax.media.CaptureDeviceInfo;
import javax.media.CaptureDeviceManager;
import javax.media.DataSink;
import javax.media.Manager;
import javax.media.MediaLocator;
import javax.media.NoDataSinkException;
import javax.media.NoProcessorException;
import javax.media.Processor;
import javax.media.control.StreamWriterControl;
import javax.media.format.AudioFormat;
import javax.media.protocol.DataSource;
import javax.media.protocol.FileTypeDescriptor;
public
class
CaptureDemo
{
/*
*
* @param args
*/
public
static
void
main(String[] args)
{
//
TODO Auto-generated method stub
CaptureDeviceInfo di
=
null
;
Processor p
=
null
;
StateHelper sh
=
null
;
Vector devList
=
CaptureDeviceManager.getDeviceList(
new
AudioFormat(
"
linear
"
,
44100
,
16
,
2
));
if
(devList.size()
>
0
)
{
di
=
(CaptureDeviceInfo)devList.firstElement();
System.
out
.println(di.getName());
System.
out
.println(di.toString());
}
else
System.exit(
-
1
);
try
{
p
=
Manager.createProcessor(di.getLocator());
sh
=
new
StateHelper(p);
}
catch
(java.io.IOException ex)
{
System.exit(
-
1
);
}
catch
(NoProcessorException e)
{
//
TODO Auto-generated catch block
System.exit(
-
1
);
}
if
(
!
sh.configure(
10000
))
{
System.exit(
-
1
);
}
p.setContentDescriptor(
new
FileTypeDescriptor(FileTypeDescriptor.WAVE));
if
(
!
sh.realize(
10000
))
{
System.exit(
-
1
);
}
DataSource source
=
p.getDataOutput();
MediaLocator dest
=
new
MediaLocator(
"
file:///c:/foo.wav
"
);
DataSink fileWriter
=
null
;
try
{
fileWriter
=
Manager.createDataSink(source, dest);
fileWriter.open();
}
catch
(NoDataSinkException e)
{
//
TODO Auto-generated catch block
System.exit(
-
1
);
}
catch
(java.io.IOException ex)
{
System.exit(
-
1
);
}
catch
(SecurityException ex)
{
System.exit(
-
1
);
}
StreamWriterControl swc
=
(StreamWriterControl) p.getControl(
"
javax.media.control.StreamWriterControl
"
);
if
(swc
!=
null
)
{
swc.setStreamSizeLimit(
500000000
);
}
try
{
fileWriter.start();
}
catch
(java.io.IOException ex)
{
System.exit(
-
1
);
}
sh.playToEndOfMedia(
5000
*
1000
);
sh.close();
fileWriter.close();
}
}
import javax.media.ConfigureCompleteEvent;
import javax.media.ControllerClosedEvent;
import javax.media.ControllerErrorEvent;
import javax.media.ControllerEvent;
import javax.media.ControllerListener;
import javax.media.EndOfMediaEvent;
import javax.media.Player;
import javax.media.Processor;
import javax.media.RealizeCompleteEvent;
public
class
StateHelper implements ControllerListener
{
Player player
=
null
;
boolean configured
=
false
;
boolean realized
=
false
;
boolean prefetched
=
false
;
boolean endOfMedia
=
false
;
boolean failed
=
false
;
boolean closed
=
false
;
public
StateHelper(Player p)
{
this
.player
=
p;
p.addControllerListener(
this
);
}
public
boolean configure(
int
timeOutMillis)
{
long
startTime
=
System.currentTimeMillis();
synchronized(
this
)
{
if
(
this
.player instanceof Processor)
{
((Processor)player).configure();
}
else
return
false
;
while
(
!
this
.configured
&&!
this
.failed)
{
try
{
this
.wait(timeOutMillis);
}
catch
(InterruptedException ex)
{
}
if
(System.currentTimeMillis()
-
startTime
>
timeOutMillis)
break
;
}
}
return
this
.configured;
}
public
boolean realize(
int
timeOutMillis)
{
long
startTime
=
System.currentTimeMillis();
synchronized(
this
)
{
this
.player.realize();
while
(
!
this
.realized
&&!
this
.failed)
{
try
{
this
.wait(timeOutMillis);
}
catch
(InterruptedException ex)
{
}
if
(System.currentTimeMillis()
-
startTime
>
timeOutMillis)
break
;
}
}
return
this
.realized;
}
public
boolean prefetch(
int
timeOutMillis)
{
long
startTime
=
System.currentTimeMillis();
synchronized(
this
)
{
this
.player.prefetch();
while
(
!
this
.prefetched
&&!
this
.failed)
{
try
{
this
.wait(timeOutMillis);
}
catch
(InterruptedException ex)
{
}
if
(System.currentTimeMillis()
-
startTime
>
timeOutMillis)
break
;
}
}
return
this
.prefetched
&&!
this
.failed;
}
public
boolean playToEndOfMedia(
int
timeOutMillis)
{
long
startTime
=
System.currentTimeMillis();
this
.endOfMedia
=
false
;
synchronized(
this
)
{
this
.player.start();
while
(
!
this
.endOfMedia
&&!
this
.failed)
{
try
{
this
.wait(timeOutMillis);
}
catch
(InterruptedException ex)
{
}
if
(System.currentTimeMillis()
-
startTime
>
timeOutMillis)
break
;
}
}
return
this
.endOfMedia
&&!
this
.failed;
}
public
void
close()
{
synchronized(
this
)
{
this
.player.close();
while
(
!
this
.closed)
{
try
{
this
.wait(
100
);
}
catch
(InterruptedException ex)
{
}
}
}
this
.player.removeControllerListener(
this
);
}
public
synchronized
void
controllerUpdate(ControllerEvent ce)
{
//
TODO Auto-generated method stub
if
(ce instanceof RealizeCompleteEvent)
{
this
.realized
=
true
;
}
else
if
(ce instanceof ConfigureCompleteEvent)
{
this
.configured
=
true
;
}
else
if
(ce instanceof EndOfMediaEvent)
{
this
.endOfMedia
=
true
;
}
else
if
(ce instanceof ControllerErrorEvent)
{
this
.failed
=
true
;
}
else
if
(ce instanceof ControllerClosedEvent)
{
this
.closed
=
true
;
}
else
{
return
;
}
this
.notifyAll();
}
}