Linux内核(三)对文件的操作(读取、光标)
读取完整代码送上(附注释)
#include
#include
#include
#include
#include
#include
#include
int main(){
char *buf = "pjy!yyds!by鸭鸭";
int fd;
fd = open("./file1",O_RDWR);
if(fd == -1){
printf("file open error!\n");
fd = open("./file1",O_RDWR|O_CREAT,0600);
if(fd > 0)
{
printf("create file1 sucessfully!\n");
}
}
printf ("open file1 successfully!&fd = %d\n",fd);
int n_write = write(fd,buf,strlen(buf));
if (n_write != -1){
printf("write%d byte to file\n",n_write);
}
close(fd);
fd = open("./file1",O_RDWR);
char *readBuf;
readBuf = (char*)malloc(sizeof(char)*n_write+1);
int n_read = read(fd,readBuf,n_write);
printf("read %d,context%s\n",n_read,readBuf);
close(fd);
return 0;
}
送上效果图
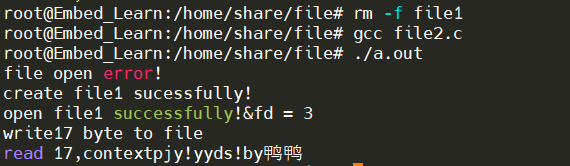
时隔几个月后复习,加了一些内容及思路
思路:
//1.打开(什么方式)
//2.打印文件描述符
//3.如果打开失败(fd=-1)以可读写的方式再来一次
//4.记得加上或操作方便创建新文件,新文件的权限可以写0600
//5.内循环判断文件描述符大于0,打印创建成功
//6.定义n_write
//7.如果fd!=-1,打印(已经写入了多少byte)
//8.暂时关闭文件
//9.读写方式打开
//10.char指针readbuf
//11.赋值readbuf
//12.赋值nread read()api
//13.打印nread和readbuf,nread统计字数,readbuf记录内容
//14.关闭文件
#include
#include
#include
#include
#include
#include
#include
int main(){
int fd;
char *buf = "pjy yyds!";
open("./file1",O_RDWR);
printf("fd = %d\n",fd);
if (fd = -1)
{
fd = open("./file1",O_RDWR|O_CREAT,0600);
printf("file not exit!\n");
if(fd > 0){
printf("create file successfully!\n");
}
}
int n_write = write(fd,buf,strlen(buf));
if(fd!=-1){
printf("write %d byte already\n",n_write);
}
close(fd);
fd = open("./file1",O_RDWR);
char *readBuf;
readBuf = (char*)malloc(sizeof(char)*n_write+1);
int n_read = read(fd,readBuf,n_write);
printf("write %d byte,and the text is :%s\n",n_read,readBuf);
close(fd);
return 0;
}
以下代码也可实现这个效果
(2)光标移到最开始,偏移值为0
#include
#include
#include
#include
#include
#include
#include
int main(){
char *buf = "pjy!yyds!by鸭鸭";
int fd;
fd = open("./file1",O_RDWR);
if(fd == -1){
printf("file open error!\n");
fd = open("./file1",O_RDWR|O_CREAT,0600);
if(fd > 0)
{
printf("create file1 sucessfully!\n");
}
}
printf ("open file1 successfully!&fd = %d\n",fd);
int n_write = write(fd,buf,strlen(buf));
if (n_write != -1){
printf("write%d byte to file\n",n_write);
}
char *readBuf;
readBuf = (char*)malloc(sizeof(char)*n_write+1);
lseek(fd,0,SEEK_SET);
int n_read = read(fd,readBuf,n_write);
printf("read %d,context%s\n",n_read,readBuf);
close(fd);
return 0;
}
(3)光标停留在当下(最后),偏移值为【-所占字节】
#include
#include
#include
#include
#include
#include
#include
int main(){
char *buf = "pjy!yyds!by鸭鸭";
int fd;
fd = open("./file1",O_RDWR);
if(fd == -1){
printf("file open error!\n");
fd = open("./file1",O_RDWR|O_CREAT,0600);
if(fd > 0)
{
printf("create file1 sucessfully!\n");
}
}
printf ("open file1 successfully!&fd = %d\n",fd);
int n_write = write(fd,buf,strlen(buf));
if (n_write != -1){
printf("write%d byte to file\n",n_write);
}
char *readBuf;
readBuf = (char*)malloc(sizeof(char)*n_write+1);
lseek(fd,-n_write,SEEK_CUR);
int n_read = read(fd,readBuf,n_write);
printf("read %d,context%s\n",n_read,readBuf);
close(fd);
return 0;
}
使用lseek()函数计算已存在的文件大小
#include
#include
#include
#include
#include
#include
#include
int main(){
char *buf = "pjy!yyds!by鸭鸭";
int fd;
fd = open("./file1",O_RDWR);
int fileSize = lseek(fd,0,SEEK_END);
printf("this file's size is %d",fileSize);
close(fd);
return 0;
}
使用lseek()函数计算已存在的文件大小的效果图

今天的内容到这里就结束了,点开博主的主页,可以看同系列的文章,可以给博主一个免费的三连鼓励一下吗?