演示如何在WTL窗口中容纳ActiveX控件(Windows自带的IE(WebBrowser)控件):
#pragma once
#include "stdafx.h"
#include <ExDisp.h>
#include <ExDispid.h>
//ID of WebBrowser control
const UINT ID_WebBrowser = 1;
typedef CWinTraits<WS_OVERLAPPEDWINDOW|WS_CLIPCHILDREN> CMainWinTraits;
class CMainWindow :
public CWindowImpl<CMainWindow,CWindow,CMainWinTraits>,
public IDispEventImpl<ID_WebBrowser,CMainWindow>
{
public:
DECLARE_WND_CLASS(_T("WTL ActiveX host window"))
//Windows message handler
BEGIN_MSG_MAP(CMainWindow)
MSG_WM_CREATE(OnCreate)
MSG_WM_DESTROY(OnDestroy)
MSG_WM_SIZE(OnSize)
END_MSG_MAP()
//WebBrowser control event message handler
BEGIN_SINK_MAP(CMainWindow)
SINK_ENTRY(ID_WebBrowser,DISPID_DOWNLOADBEGIN,OnDownloadBegin)
SINK_ENTRY(ID_WebBrowser,DISPID_DOWNLOADCOMPLETE,OnDownloadComplete)
END_SINK_MAP()
int OnCreate(LPCREATESTRUCT lpCreateStruct)
{
CRect rc;
GetClientRect(&rc);
/*
//Create the WebBrowser control(IE) with ProgId
HWND hWndIE = m_ax.Create(
m_hWnd,
rc,
_T("Shell.Explorer.2"), //ProgId of WebBrowser control
WS_CHILD|WS_VISIBLE,
0,
ID_WebBrowser);
ATLASSERT(hWndIE);
*/
//Create the WebBrowser control(IE) with CLSID
CComPtr<IUnknown> spUnkWebBrowser;
HRESULT hr = spUnkWebBrowser.CoCreateInstance(CLSID_WebBrowser);
CHECKHR(hr);
//Create the ActiveX host window
HWND hWndWB = m_ax.Create(
m_hWnd,
rc,
NULL,
WS_CHILD|WS_VISIBLE,
0,
ID_WebBrowser);
ATLASSERT(hWndWB);
//Attach the WebBrowser control to host window
CComPtr<IUnknown> spUnkContainer;
m_ax.AttachControl(spUnkWebBrowser,&spUnkContainer);
//Advise sink map
AtlAdviseSinkMap(this,true);
//Query interface
hr = m_ax.QueryControl(&m_spIWebBrowser);
CHECKHR(hr);
CComBSTR site(_T("http://www.hust.edu.cn"));
CComVariant v;
hr = m_spIWebBrowser->Navigate(site,&v,&v,&v,&v);
CHECKHR(hr);
return 0;
}
void OnDestroy()
{
//Unadvise sink map
AtlAdviseSinkMap(this,false);
PostQuitMessage(0);
}
void OnSize(UINT nType, CSize size)
{
CRect rc;
GetClientRect(&rc);
m_ax.SetWindowPos(m_hWnd,&rc,SWP_NOZORDER|SWP_NOACTIVATE);
}
void __stdcall OnDownloadBegin()
{
SetWindowText(_T("Download begin:"));
}
void __stdcall OnDownloadComplete()
{
SetWindowText(_T("Download completed."));
}
private:
CAxWindow m_ax;
CComPtr<IWebBrowser2> m_spIWebBrowser;
};
效果:
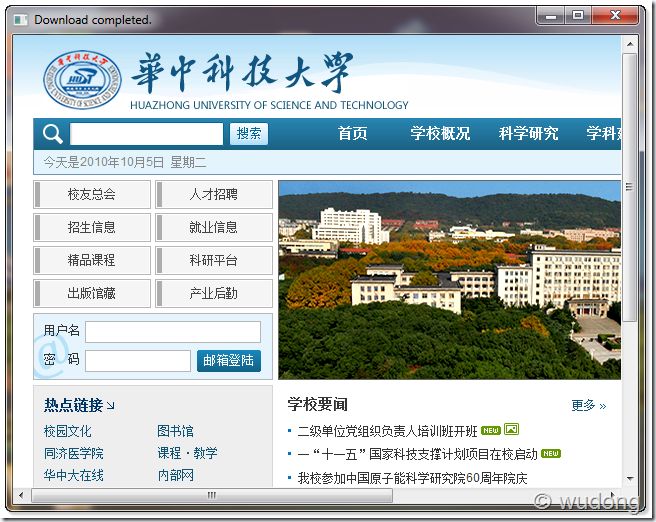