剩余油领域模型持久化设计
OOA
通过健壮性分析图和交互图(时序图或协作图)分析用例
以C.1产量递减为例:
[一、读取用例]
主成功事件流
1、 系统要求用户提供基础数据源:产量递减基础数据
2、 用户提供产量递减基础数据
3、 系统读取产量递减基础数据,要求用户选择递减类型
4、 用户选择递减类型(指数递减、双曲递减、衰减递减)
5、 系统执行产量递减算法产生结果:产量递减结果
6、 用户要求系统保存产量递减结果
7、系统保存产量递减结果
[二、绘制健壮性分析图]
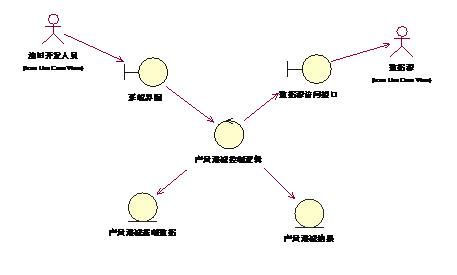
绘制健壮性分析图的目的是为了寻找分析类,在用例文本和图形之间寻找一种对应关系,在寻找对应关系的同时,会对需求的整理进行一次正确性和完整性的检查,如果发现问题,重新回到需求进行整理。必要时发起讨论。
[三、绘制交互图]
时序图:
协作图:
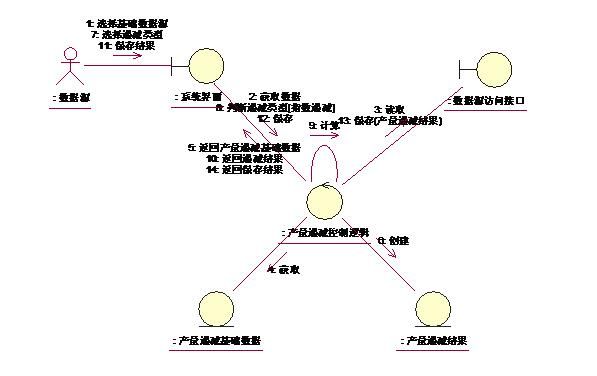
根据用例文本绘制时序图,在此注意事件流的顺序。将这种时序性和对象之间寻找关系,转换为协作图。绘制交互图的过程尽可能的不要考虑类的设计,重点关注对象在需求中的交互。将对象中的关系简单的抽象为分析类的职责。如下图所示:
考虑项目组目前的实施。注意与项目组其它成员的合作情况。在需要与其它人进行合作时,安排好自己的迭代计划,在每日开始前的五分钟会议与项目组人员计划当日结对情况。同时对分析类进行简单的设计。比如:
数据源访问接口可以采用Nhibernate完成,而系统的界面部分可以暂时用原型代替。这样我们只需要关心产量递减控制逻辑、产量递减基础数据和产量递减结果。
快速实现初探代码
[一、建立单元测试类]
由以上用例分析可知,我们需要对产量递减基础数据进行计算,然后产生产量递减结果。因此,我们需要对产量递减控制逻辑的计算进行测试。
添加单元测试类
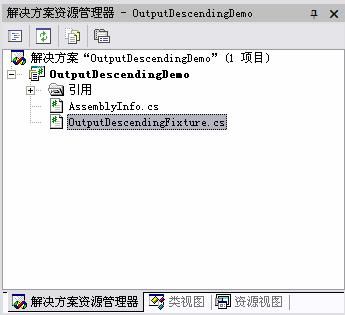
添加单元测试库引用
添加测试代码:OutputDescendingFixture
OutputDescendingFixture

/**//// <summary>
/// 产量递减测试类
/// </summary>
[TestFixture]
public class OutputDescendingFixture

{
[Test]
//对产量递减类OutputDescendingControl的计算方法Calculate进行测试

public void CalculateTest()
{
//创建产量递减控制类
OutputDescendingControl control=new OutputDescendingControl();
//创建代理产量递减对象集合
IList dataBaseList=new ArrayList();
dataBaseList.Add(new OutputDescendingDataBase("#1",1997,23.4));
dataBaseList.Add(new OutputDescendingDataBase("#2",1998,23.5));
dataBaseList.Add(new OutputDescendingDataBase("#3",1999,23.6));
dataBaseList.Add(new OutputDescendingDataBase("#4",2000,23.7));
//获取结果,与预期值相比较,在第一次测试时应故意报错
Assert.AreEqual(23.6,(control.Calculate(dataBaseList) as ResultofOutputDescending).MaxOutput);
}
}
[二、建立设计类]
产量递减控制逻辑类:OutputDescendingControl
OutputDescendingControl

/**//// <summary>
/// 产量递减控制逻辑类
/// </summary>
public class OutputDescendingControl

{
public OutputDescendingControl()

{
}

/**//// <summary>
/// 计算方法
/// </summary>
/// <param name="outputDescendingDataBaseList">产量递减基础数据集合</param>
/// <returns>产量递减结果</returns>

public ResultofOutputDescending Calculate(IList outputDescendingDataBaseList)
{
//此处应实现你的计算代码
ResultofOutputDescending resultofOutputDescending=new ResultofOutputDescending(23.7);
return resultofOutputDescending;
}
}
产量递减基础数据:OutputDescendingDataBase

/**//// <summary>
/// 产量递减基础数据
/// </summary>
public class OutputDescendingDataBase

{
public OutputDescendingDataBase()

{
}

/**//// <summary>
/// 构造函数
/// </summary>
/// <param name="wellID">油田</param>
/// <param name="year">时间</param>
/// <param name="resultofOutput">产量</param>
public OutputDescendingDataBase(string wellID,float year,double resultofOutput)

{
this.wellID=wellID;
this.year=year;
this.resultofOutput=resultofOutput;
}
private string wellID; //油田
private float year; //时间
private double resultofOutput; //产量

/**//// <summary>
/// 油田
/// </summary>

public string WellID
{

get
{return wellID;}
}

/**//// <summary>
/// 时间
/// </summary>

public float Year
{

get
{return year;}
}

/**//// <summary>
/// 产量
/// </summary>

public double ResultofOutput
{

get
{return resultofOutput;}
}
}
产量递减结果:ResultofOutputDescending

/**//// <summary>
/// 产量递减结果
/// </summary>
public class ResultofOutputDescending

{
public ResultofOutputDescending(double maxOutput)

{
this.maxOutput=maxOutput;
}
private double maxOutput;//最大累积产量

/**//// <summary>
/// 最大累积产量
/// </summary>

public double MaxOutput
{

get
{return maxOutput;}
}
}
[三、运行测试]
编译OutputDescendingDemo项目,产生程序集OutputDescending.dll。用Nunit打开该程序集,运行。
错了!很正常。
修改测试类的预测结果改为23.7。
Assert.AreEqual(
23.7
,(control.Calculate(dataBaseList)
as
ResultofOutputDescending).MaxOutput);
运行,通过。
[四、重构,添加新代码]
对代码不合理的部分进行重构。
我们目前完成的是对计算进行测试的代码,计算的基础数据皆采用代理数据。现在我们将其替换成实际使用的数据。在项目中添加Nhibernate引用。
创建与领域模型类相对应的
XML
配置文档和测试类。(可以采用
MyGeneration
自动生成代码,然后进行更改)
起动
MyGeneration
,打开项目模板
Iverson_NHibernate.csgen
。
F5运行,配置生成项
OK,产生领域模型、XML配置文档和单元测试类
对生成的代码进行修改。XML配置文档Outputdescendingdatabase.hbm.xml
<?
xml version
=
"
1.0
"
encoding
=
"
utf-8
"
?>
<
hibernate
-
mapping xmlns
=
"
urn:nhibernate-mapping-2.0
"
>
<
class
name
=
"
OutputDescendingDemo.OutputDescendingDataBase,OutputDescendingDemo
"
table
=
"
OUTPUTDESCENDINGDATABASE
"
>
翻译一下,类
OutputDescendingDemo.OutputDescendingDataBase
,位于程序集
OutputDescendingDemo
,它对应于
OUTPUTDESCENDINGDATABASE
数据表
<
id name
=
"
ID
"
column
=
"
id
"
type
=
"
String
"
>
<
generator
class
=
"
uuid.hex
"
/>
</
id
>
唯一值,Nhibernate的要求。<generator class="uuid.hex"/>表示生成唯一值的方式是uuid.hex
<
property column
=
"
WELLID
"
type
=
"
String
"
name
=
"
WellID
"
not
-
null
=
"
true
"
length
=
"
100
"
/>
<
property column
=
"
YEAR
"
type
=
"
Single
"
name
=
"
Year
"
not
-
null
=
"
true
"
/>
<
property column
=
"
RESULTOFOUTPUT
"
type
=
"
Single
"
name
=
"
ResultofOutput
"
not
-
null
=
"
true
"
/>
属性对应的列名,以及类型
</
class
>
</
hibernate
-
mapping
>
更改原OutputDescendingDataBase.cs 文件,添加ID字段。对应于Nhibernate的唯一值。或应用自动生成的Outputdescendingdatabase.cs文件替换原文件。替换掉产量递减测试类OutputDescendingFixture中CalculateTest的原有代码。
using System;
using System.Collections;
using NUnit.Framework;
using NHibernate;
using System.Reflection;

namespace OutputDescendingDemo


{

/**//// <summary>
/// 产量递减测试类
/// </summary>
[TestFixture]
public class OutputDescendingFixture

{
NHibernate.Cfg.Configuration configuration;
ISessionFactory iSessionFactory;
ISession session;
[TestFixtureSetUp]
public void Init()

{
configuration=new NHibernate.Cfg.Configuration();
configuration.AddAssembly(Assembly.GetExecutingAssembly());
iSessionFactory=configuration.BuildSessionFactory();
session = iSessionFactory.OpenSession();
}
[TestFixtureTearDown]
public void Dispose()

{
session=null;
iSessionFactory=null;
configuration=null;
}
[Test]
//对产量递减类OutputDescendingControl的计算方法Calculate进行测试

public void CalculateTest()
{
//创建产量递减控制类
OutputDescendingControl control=new OutputDescendingControl();
//采用真实的产量递减对象集合
IQuery query = session.CreateQuery(" from OutputDescendingDataBase");
IList dataBaseList = query.List();
//获取结果,与预期值相比较,在第一次测试时应故意报错
Assert.AreEqual(23.7,(control.Calculate(dataBaseList) as ResultofOutputDescending).MaxOutput);
}
}
}
将XML配置文档Outputdescendingdatabase.hbm.xml添加到当前项目中, 设定XML配置文档为嵌入资源
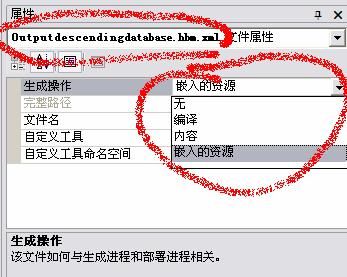
在项目的生成目录下创建以程序集为名称的Config文档OutputDescending.dll.config。
<?
xml version
=
"
1.0
"
encoding
=
"
utf-8
"
?>
<
configuration
>
<
configSections
>
<
section name
=
"
nhibernate
"
type
=
"
System.Configuration.NameValueSectionHandler, System, Version=1.0.5000.0,Culture=neutral, PublicKeyToken=b77a5c561934e089
"
/>
<
section name
=
"
log4net
"
type
=
"
log4net.Config.Log4NetConfigurationSectionHandler,log4net
"
/>
</
configSections
>

<
nhibernate
>
<
add
key
=
"
hibernate.connection.driver_class
"
value
=
"
NHibernate.Driver.OleDbDriver
"
/>
<
add
key
=
"
hibernate.dialect
"
value
=
"
NHibernate.Dialect.Oracle9Dialect
"
/>
<
add
key
=
"
hibernate.connection.provider
"
value
=
"
NHibernate.Connection.DriverConnectionProvider
"
/>
<
add
key
=
"
hibernate.connection.connection_string
"
value
=
"
Provider=MSDAORA.1;user id=ROS;data source=ROSA;password=ROSP
"
/>
</
nhibernate
>
<
log4net
>
<
appender name
=
"
rollingFile
"
type
=
"
log4net.Appender.RollingFileAppender,log4net
"
>

<
param name
=
"
File
"
value
=
"
log.txt
"
/>
<
param name
=
"
AppendToFile
"
value
=
"
true
"
/>
<
param name
=
"
RollingStyle
"
value
=
"
Date
"
/>
<
param name
=
"
DatePattern
"
value
=
"
yyyy.MM.dd
"
/>
<
param name
=
"
StaticLogFileName
"
value
=
"
true
"
/>

<
layout type
=
"
log4net.Layout.PatternLayout,log4net
"
>
<
param name
=
"
ConversionPattern
"
value
=
"
%d [%t] %-5p %c [%x] <%X{auth}> - %m%n
"
/>
</
layout
>
</
appender
>
<!--
Setup the root category, add the appenders and
set
the
default
priority
-->
<
root
>
<
priority value
=
"
ALL
"
/>
<
appender
-
ref
ref
=
"
rollingFile
"
/>
</
root
>
</
log4net
>
</
configuration
>
更改帐号和数据源,使其适应你的应用程序