对这个数据:
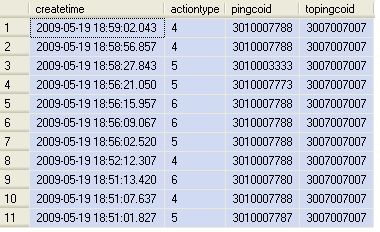
进行如下如下查询:
select top 8 max(createtime) as createtime,actiontype,pingcoid from AppleGrange.ActionLog
where topingcoid = '3007007007' and pingcoid<>'3007007007'
group by actiontype,pingcoid
order by createtime desc
的到
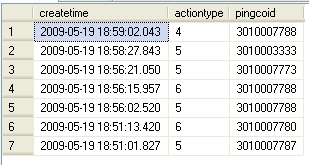
相应的Linq查询:
Code
from l in ActionLogs
where l.ToPingcoID=="3007007007" && l.ToPingcoID!=l.Pingcoid
orderby l.CreateTime descending
group l by new { l.ActionType,l.Pingcoid } into xx select xx.Key
或者:
Code
ActionLogs
.Where (l => ((l.ToPingcoID == "3007007007") && (l.ToPingcoID != l.Pingcoid)))
.OrderByDescending (l => l.CreateTime)
.GroupBy (
l =>
new
{
ActionType = l.ActionType,
Pingcoid = l.Pingcoid
}
)
.Select (xx => xx.Key)
结果:
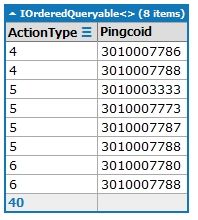
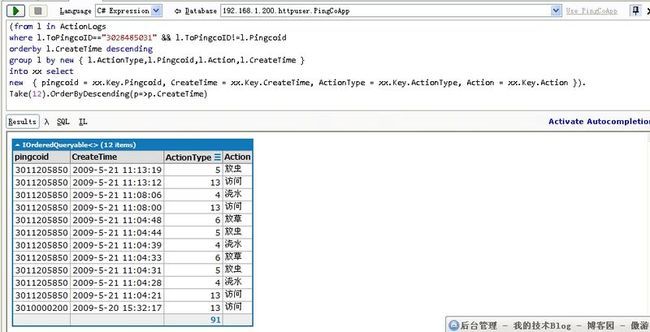
///
<summary>
///
拨打记录
///
</summary>
///
<param name="pingcoId"></param>
///
<returns></returns>
public
List
<
CallLog
>
GetUserCallLog(
string
pingcoId)
{
var list
=
new
List
<
CallLog
>
();
var query
=
_context.CallLog.Where(u
=>
u.PingCoId
==
pingcoId).GroupBy(u
=>
u.Called);
foreach
(IGrouping
<
string
, CallLog
>
log
in
query)
{
var v
=
log.OrderByDescending(l
=>
l.CreateTime).FirstOrDefault();
//
注意此时log还是list
list.Add(v);
}
var vv
=
from l
in
list orderby l.CreateTime descending select l;
return
vv.ToList();
}
--
Group
Group是进行分组操作,同SQL中的Group By类似。
原型如下:
public
static
IEnumerable
<
IGrouping
<
TKey, TSource
>>
GroupBy
<
TSource, TKey
>
(
this
IEnumerable
<
TSource
>
source,
Func
<
TSource, TKey
>
keySelector)
它有几个重载,返回类型有两种:IEnumerable<IGrouping<TKey, TSource>> 和 IEnumerable<TResult>。
返回类型为:IEnumerable<IGrouping<TKey, TSource>>
示例:
返回按PIngcoId分组 的人数:
var r = from pingcoid in _context.AskPwdLog group pingcoid by pingcoid.PingcoId into p select p;
或:等效的扩展方法调用实现为:
v = _context.AskPwdLog.GroupBy(u => u.PingcoId);
注:
scoreGroup为IGrouping<TKey, TSource>类型,返回结果为IEnumerable<IGrouping<TKey, TSource>>,既集合的集合,因此输出时需用双重循环。
IGrouping<TKey, TElement>接口定义为:
public
interface
IGrouping
<
TKey, TElement
>
: IEnumerable
<
TElement
>
, IEnumerable
{
TKey Key {
get
; }
}
其中Key为分组依据的字段。
foreach
(
var
group
in
result)
{
//
输出分组依据的字段
Console.WriteLine(
"
\n Id:
"
+
group
.Key);
//
输出组内成员
foreach
(
var
s
in
group
)
{
Console.WriteLine(s.time);
}
}
//
result:
//
Student ID:1
//
Student ID:1,Course ID:1,time:2010-09-11
//
Student ID:1,Course ID:2,time:2010-09-12
//
...
//
Student ID:2
//
Student ID:2,Course ID:1,time:2010-09-11
//
...
---2010-04-28---实例:---
// <summary>
/// 每个人索要的总次数
/// </summary>
/// <param name="type"></param>
/// <returns></returns>
public List<AskingPassword> GetAskPasswordGroupNum()
{
var askingPasswords = new List<AskingPassword>();
var v = _context.AskPwdLog.GroupBy(u => u.PingcoId);
foreach (IGrouping<string, AskPwdLog> askPwdLogs in v)
{
var ask = new AskingPassword();
ask.PingCoId = askPwdLogs.Key;
ask.AskNum = askPwdLogs.Count(); //分组后的条数
askingPasswords.Add(ask);
}
return askingPasswords;
}