springboot整合freemarker
- 1 依赖
- 2 application.yml
- 3 实体类
- 4 controller
- 5.取普通变量的值
- 6.遍历集合
- 7.遍历map
- 8.转义,加r
- 9.定义数值,且格式化数值
- 10.定义布尔运算符
- 11.三目运算符,用内置函数string实现
- 12.字符串的拼接
- 13.集合,map的拼接
- 14.算术运算符
- 15.比较运算符
- 16.逻辑运算符
- 17.分支语句
- 18.内置函数
- 19.如何判断某个变量为空?
- 20.如何引入其他模板
- 21.不解析内容
- 22.自定义宏
- 23 导入宏,并且使用宏
1 依赖
org.springframework.boot
spring-boot-starter-freemarker
2 application.yml
server:
port: 8080
spring:
datasource:
url: jdbc:mysql://localhost:3306/test?characterEncoding=utf-8
username: root
password: root
driver-class-name: com.mysql.jdbc.Driver
freemarker:
allow-request-override: false
cache: false
check-template-location: true
charset: UTF-8
content-type: text/html; charset=utf-8
expose-request-attributes: false
expose-session-attributes: false
expose-spring-macro-helpers: false
#prefix:
suffix: .ftlh
template-loader-path:
- classpath:/templates/
mvc:
static-path-pattern: /static/**
web:
resources:
static-locations: classpath:/static
3 实体类
public class User implements Serializable {
private int id;
private String name;
private int age;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
4 controller
@Controller
public class UserController {
@GetMapping("/hello")
public String hello(Model model) {
List users = new ArrayList<>();
for (int i = 0; i < 10; i++) {
User user = new User();
user.setId(i);
user.setName("xiaolan"+i);
user.setAge(18);
users.add(user);
}
Map map = new HashMap<>();
map.put("name","yangl");
map.put("age",20);
map.put("salary",7000);
model.addAttribute("info",map);
model.addAttribute("sno","2020001");
model.addAttribute("birth",new Date());
model.addAttribute("users",users);
return "hello";
}
}
5.取普通变量的值
${sno}
6.遍历集合
<#list ['hello','java','jq'] as x>
${x}
#list>
<#list 1..5 as x>
${x}
#list>
<#list 5..1 as x>
${x}
#list>
<#list users as user>
${user.id} |
${user.name} |
${user.age} |
${user_index} |
${user_has_next?string('yes','no')} |
#list>
${users[1].age}
<#list users[2..5] as user>
${user.id} |
${user.name} |
${user.age} |
#list>
7.遍历map
<#assign usermap={"name":"小兰","age":18}>
<#list usermap?keys as key>
${key}--${usermap[key]}
#list>
<#list usermap?values as value>
${value}
#list>
8.转义,加r
${r"C:\"}
${r"hello vue"}
9.定义数值,且格式化数值
<#assign price=99>
${price?string.currency}
${price?string.percent}
10.定义布尔运算符
<#assign flag=true>
11.三目运算符,用内置函数string实现
${flag?string('yes','no')}
12.字符串的拼接
${'hello ${sno}'}
${'hello ' + sno }
${sno[0]}${sno[2]}
${sno[1..3]}
13.集合,map的拼接
<#list [1,2,3]+[6,8,9] as x>
${x}
#list>
<#list (info+{"message":"soho"})?keys as key>
${key}
#list>
14.算术运算符
<#assign num=15>
${num+10/1*7}
15.比较运算符
<#assign i=10>
<#if i = 10>i = 10#if>
<#if i != 10>i != 10#if>
<#if i == 10>i == 10#if>
<#if i gt 10>i gt 10#if>
<#if i gte 10>i gte 10#if>
<#if i lt 10>i lt 10#if>
<#if i lte 10>i lte 10#if>
16.逻辑运算符
<#assign b=20>
<#if (b gt 10 && b lt 100)>aaa#if>
<#if !(b==10)>bbb#if>
<#if (b == 100 || 1==1)>ccc#if>
17.分支语句
<#assign score=50>
<#if score gt 90> A
<#elseif score gt 80>B
<#elseif score gt 60>C
<#else>D
#if>
<#assign b=90>
<#switch b>
<#case 10>10<#break>
<#case 20>20<#break>
<#case 30>30<#break>
<#default> 999
#switch>
18.内置函数
${"hello"?cap_first}
${"hello"?lower_case}
${"hello"?upper_case}
${" hello "?trim}
${3.14566?int}
${users?size}
${birth?string("yyyy-MM-dd")}
19.如何判断某个变量为空?
<#if qq??>
qq
#if>
${dd!"dd"}
20.如何引入其他模板
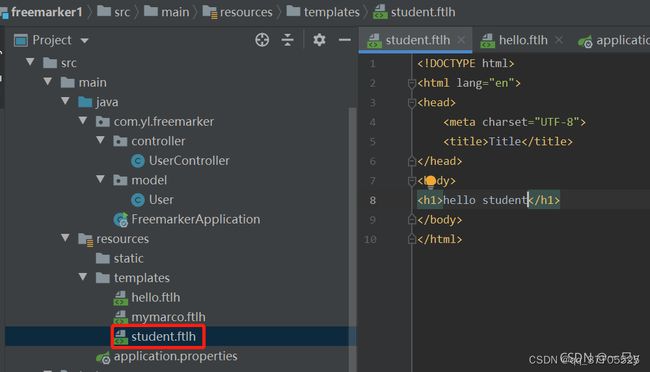
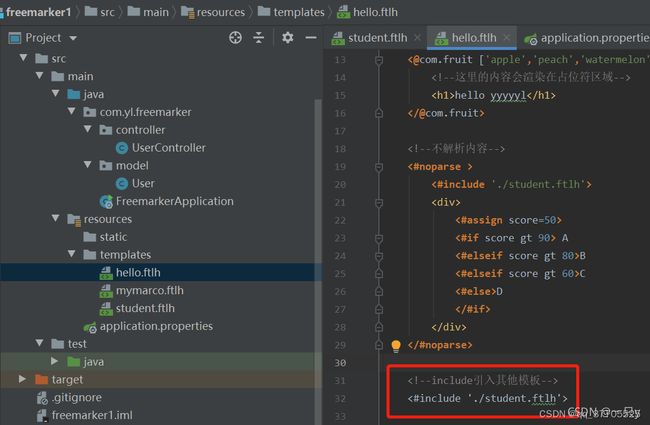
21.不解析内容
<#noparse >
<#include './student.ftlh'>
<#assign score=50>
<#if score gt 90> A
<#elseif score gt 80>B
<#elseif score gt 60>C
<#else>D
#if>
#noparse>
22.自定义宏
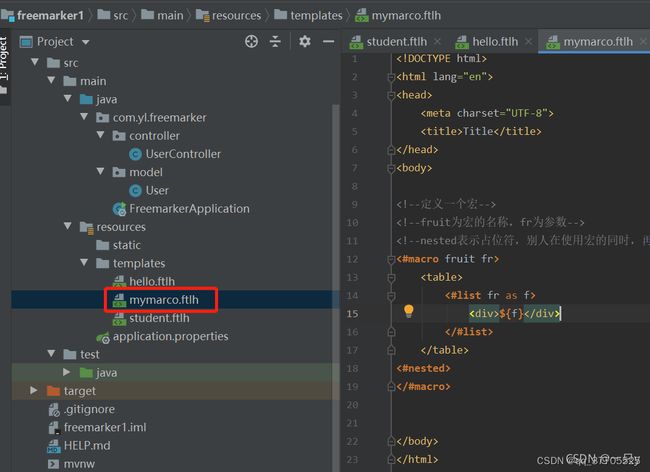
23 导入宏,并且使用宏
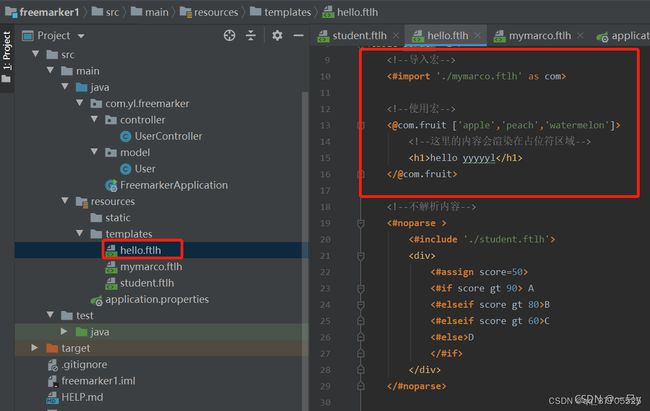