轮廓着色
#include
#include
#include "opencv2/core/core.hpp"
#include "opencv2/imgproc/imgproc.hpp"
#include "opencv2/calib3d/calib3d.hpp"
#include "opencv2/highgui/highgui.hpp"
#include "opencv2/opencv.hpp"
#include
#include
#include
#define SHOW_TEMP 1;
using namespace std;
using namespace cv;
Point2f operator-(Point2f a, Point2f b)
{
Point2f temp(0, 0);
temp.x = a.x - b.x;
temp.y = a.y - b.y;
return temp;
}
Point2f operator+(Point2f a, Point2f b)
{
Point2f temp(0, 0);
temp.x = a.x + b.x;
temp.y = a.y + b.y;
return temp;
}
float operator*(Point2f a, Point2f b)
{
float multiplicate = a.x * b.x + a.y * b.y;
return multiplicate;
}
struct edgePoint {
int ind;
cv::Point2f pos;
float angle;
public:
edgePoint(const int& _ind, const cv::Point2f& _pos, const double& _angle) : ind(_ind),
pos(_pos), angle(_angle)
{
}
edgePoint() {}
};
bool cmp(edgePoint a, edgePoint b)
{
return a.angle > b.angle;
};
double dis2(double x1, double y1, double x2, double y2) {
return (x2 - x1) * (x2 - x1) + (y2 - y1) * (y2 - y1);
}
double arccos(double x0, double y0, double x1, double y1)
{
double angle = 0;
double l = dis2(x0, y0, x1, y1);
l = sqrt(l);
if (y1 - y0 > 0) { angle = acos((x1 - x0) / l); }
else { angle = 3.141592653 + 3.141592653 - acos((x1 - x0) / l); }
return angle;
}
double arccos(cv::Point cneter, cv::Point p) {
return arccos(double(cneter.x), double(cneter.y), double(p.x), double(p.y));
}
bool isArcCurve(std::vector<cv::Point>& curve)
{
bool isArc = false;
cv::Point ps, pe, vec;
ps = cv::Point(curve[0]);
pe = cv::Point(curve[curve.size() - 1]);
double angle0 = arccos(ps, pe);
std::vector<double > rls;
for (int i = 1; i < curve.size() - 1; ++i) {
double angle1 = arccos(ps, curve[i]);
rls.push_back(angle1);
}
std::vector<bool > rlbs;
for (int i = 0; i < rls.size(); ++i) {
bool isRight = false;
if (rls[i] > angle0) {
isRight = true;
}
else {
isRight = false;
}
rlbs.push_back(isRight);
}
bool isSinVec = false;
bool isRight = false;
if (rlbs.size() > 0) {
isRight = rlbs[0];
for (int i = 1; i < rlbs.size(); ++i) {
if (isRight != rlbs[i]) {
isSinVec = true;
}
}
}
else {
}
isArc = isSinVec;
return isArc;
}
int main() {
Mat imgSrc = imread("./8MS4.bmp");
if (imgSrc.empty())
{
std::cout << "打开图片失败,请检查路径!!" << endl;
return -1;
}
Mat imgGray;
Mat imgGauss;
Mat imgBin;
cvtColor(imgSrc, imgGray, COLOR_BGR2GRAY);
adaptiveThreshold(imgGray, imgBin, 255, ADAPTIVE_THRESH_GAUSSIAN_C, THRESH_BINARY_INV, 5, 5);
imshow("adaptiveThreshold", imgBin);
waitKey(3000);
Mat imgDil;
Mat imgEro;
Mat kerneld;
Mat kernele;
kerneld = getStructuringElement(MORPH_RECT, Size(3, 3));
dilate(imgBin, imgDil, kerneld);
kernele = getStructuringElement(MORPH_RECT, Size(5, 5));
erode(imgDil, imgEro, kernele);
kernele = getStructuringElement(MORPH_RECT, Size(3, 1));
erode(imgEro, imgEro, kernele);
kerneld = getStructuringElement(MORPH_RECT, Size(3, 1));
dilate(imgEro, imgEro, kerneld);
Mat dstImage = Mat::zeros(imgSrc.rows, imgSrc.cols, CV_8UC3);
vector<vector<Point> > contours;
vector<Vec4i> hierarchy;
findContours(imgEro, contours, hierarchy,
CV_RETR_CCOMP, CV_CHAIN_APPROX_SIMPLE);
int index = 0;
for (; index >= 0; index = hierarchy[index][0])
{
Scalar color(rand() & 255, rand() & 255, rand() & 255);
drawContours(dstImage, contours, index, color, 1);
if (isArcCurve(contours[index])) {
Scalar color(rand() & 255, rand() & 255, rand() & 255);
drawContours(dstImage, contours, index, color, CV_FILLED, 8, hierarchy);
}
}
imshow("轮廓图", dstImage);
waitKey(3000);
imshow("image", imgEro);
waitKey(0);
return 0;
}
运行图:
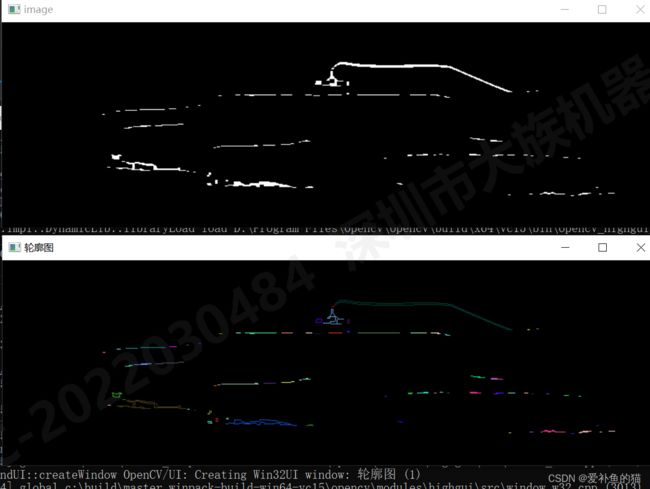