1. 普通的文本组件
Text("默认样式文本组件")
2. 给文本组件添加一些属性
eg:
Text(
"测试文本"*10,
textAlign: TextAlign.start,
maxLines: 1,
overflow: TextOverflow.ellipsis,
textScaleFactor: 2,
style: TextStyle(
color: Colors.yellow,
fontSize: 7.0,
background: Paint()..color = Colors.blue,
decoration: TextDecoration.underline
),
),
-
textAligin:属性定义文本对齐方式,start、end、center(起始位置对齐、结尾位置对齐和居中对齐)。起始位置对其等价于左对齐,结尾位置对齐等价于右对齐。如果文本本身已经相对于父组件居中对齐,那么问自己是设置了非居中对齐,最终也还是相对于父组件居中对齐。
-
maxLines:改属性定义文本组件最大显示行数,默认情况下自动换行。如果值为1,当文本长度超过1行,超出范围会被省略。
-
overflow:改属性知名文本超出范围的省略方式。TextOverflow.ellipsis值为(…)
-
textScaleFactor:该文字属性定义字体缩放比例,默认1.0。
-
Flutter支持定义属性,style: TextStyle,例如:color(字体颜色)、fontSize(字体大小,注意,这是精确的尺寸值,因此不会像textScaleFactor那样随系统变化而变化)、background(背景颜色)、decoration(背景装饰,上文时指下划线)
3. Text.rich,同时存在不同样式的文本
eg:
Text.rich(
TextSpan(children: [
TextSpan(
text: "Red text ",
style: TextStyle(color: Colors.red, fontSize: 25.0),
),
TextSpan(
text:"Blue text ",
style: TextStyle(color: Colors.blue, fontSize: 25.0),
recognizer: TapGestureRecognizer()..onTap = (){
debugPrint("Blue text clicked");
}
)
])
),
-
上述代码中,TextSpan包含了两个子组件,每个TextSpan代表一个文本片段,其中第二文本片段中添加了触摸事件,然后在后台输出debug中的文字。
4. DefaltTextStyle,文本默认样式的继承
eg:
DefaultTextStyle(
style: TextStyle(
color: Colors.green,
fontSize: 20.0
),
textAlign: TextAlign.start,
child: Column(
children: [
Text("Green Text"),
Text(
"Another text",
style: TextStyle(inherit:false, color:Colors.black),
),
Text(
"My color is not green",
style: TextStyle(color:Colors.grey),
)
],
),
)
-
上述代码中,在DefaultTextStyle中包裹的,未单独定义提到样式,全部会使用DefaultTextStyle 的样式,Another text中,“inherif:false”,意思是完全不继承, “My color is not green”, 单独添加了color,因此除了color以外的样式都会被继承。
代码样式
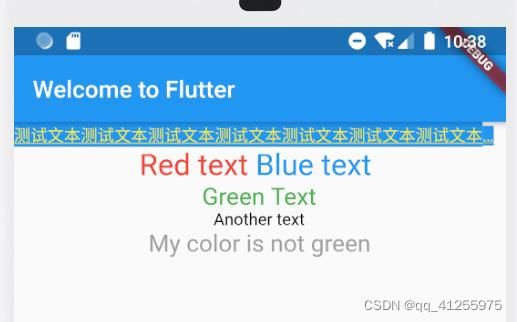
源码
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text('Welcome to Flutter'),
),
body: Center(
child: Column(
children: [
Text(
"测试文本"*10,
textAlign: TextAlign.start,
maxLines: 1,
overflow: TextOverflow.ellipsis,
textScaleFactor: 2,
style: TextStyle(
color: Colors.yellow,
fontSize: 7.0,
background: Paint()..color = Colors.blue,
decoration: TextDecoration.underline
),
),
Text.rich(
TextSpan(children: [
TextSpan(
text: "Red text ",
style: TextStyle(color: Colors.red, fontSize: 25.0),
),
TextSpan(
text:"Blue text ",
style: TextStyle(color: Colors.blue, fontSize: 25.0),
recognizer: TapGestureRecognizer()..onTap = (){
debugPrint("Blue text clicked");
}
)
])
),
DefaultTextStyle(
style: TextStyle(
color: Colors.green,
fontSize: 20.0
),
textAlign: TextAlign.start,
child: Column(
children: [
Text("Green Text"),
Text(
"Another text",
style: TextStyle(inherit:false, color:Colors.black),
),
Text(
"My color is not green",
style: TextStyle(color:Colors.grey),
)
],
),
)
],
)
),
),
);
}
}