1:接口自动化测试,分很多种,今天这个比较简单,也比较通用,有点java基础的人方可。
2:创建一个maven项目【省略,可以查看百度怎么建立maven项目】
3:在pom文件里面添加以下依赖
4.0.0
org.example
one
1.0-SNAPSHOT
one
http://www.example.com
ch.qos.logback
logback-classic
1.1.11
com.alibaba
easyexcel
2.1.7
org.apache.poi
poi-ooxml
org.slf4j
slf4j-simple
1.7.5
net.sourceforge.jexcelapi
jxl
2.6.10
org.apache.xmlbeans
xmlbeans
3.1.0
junit
junit
4.12
org.apache.poi
poi-ooxml
com.alibaba
easyexcel
2.1.1
org.apache.poi
poi
5.0.0
org.projectlombok
lombok
1.18.0
org.apache.poi
poi-ooxml
5.0.0
junit
junit
4.11
test
org.testng
testng
7.0.0
test
top.jfunc.common
converter
1.8.0
com.squareup.okhttp3
okhttp
4.9.0
org.apache.httpcomponents
httpclient
4.5.12
io.airlift
log
201
org.json
json
20200518
net.sf.json-lib
json-lib-ext-spring
1.0.2
org.testng
testng
7.0.0
compile
javax.mail
mail
1.4.7
org.apache.logging.log4j
log4j-core
2.13.3
com.aventstack
extentreports
3.1.5
maven-clean-plugin 3.1.0 maven-resources-plugin 3.0.2 maven-compiler-plugin 3.8.0 maven-surefire-plugin 2.22.1 maven-jar-plugin 3.0.2 maven-install-plugin 2.5.2 maven-deploy-plugin 2.8.2 maven-site-plugin 3.7.1 maven-project-info-reports-plugin 3.0.0
org.apache.maven.plugins
maven-surefire-plugin
3.0.0-M4
testng.xml
org.apache.maven.plugins
maven-compiler-plugin
8
8
代码如下:
package Utils;
import com.alibaba.fastjson.JSONObject;
import okhttp3.*;
import org.testng.Assert;
import javax.print.attribute.standard.Media;
import java.io.File;
import java.io.IOException;
import java.util.Map;
import static java.lang.String.valueOf;
public class Quest {
public static final MediaType MEDIA_TYPE_PNG = MediaType.parse(“image/png”);
public static OkHttpClient clients = new OkHttpClient() ;
OkHttpClient client = new OkHttpClient();
/**
* post使用的json头
/
public static final MediaType JSON = MediaType.parse(“application/json; charset=utf-8”);
/*
* get接口操作方法 *
*
* @param url 接口地址
* @return
* @throws IOException
*/
public String get(String url) throws IOException {
Request request = new Request.Builder().url(url).build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("错误码: " + response);
}
}
/**
* post接口操作方法 *
*
* @param url 接口地址
* @param map
* @param file
* @return
* @throws IOException
*/
public String post(String url, Map map, File file) throws IOException {
Request request = new Request.Builder().url(url).build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("错误码: " + response);
}
}
/**
* 使用键值对调用post方法
* @param url 接口链接
* @param map 键值对
* @return
* @throws IOException
*/
String response=null;
public String post(String url, Map map) throws IOException {
FormBody.Builder build = new FormBody.Builder();
for (Map.Entry item : map.entrySet()) {
build.add(item.getKey(), item.getValue());
}
RequestBody formBody = build.build();
Request request = new Request.Builder()
.url(url)
.post(formBody)
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
public String postone(String url, Map map) throws IOException {
FormBody.Builder build = new FormBody.Builder();
for (Map.Entry item : map.entrySet()) {
build.add(item.getKey(), item.getValue());
}
RequestBody formBody = build.build();
Request request = new Request.Builder()
.url(url)
.post(formBody)
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
public String postobjec(String url, Map map) throws IOException {
FormBody.Builder build = new FormBody.Builder();
for (Map.Entry item : map.entrySet()) {
build.add(item.getKey(), String.valueOf(item.getValue()));
}
RequestBody formBody = build.build();
Request request = new Request.Builder()
.url(url)
.post(formBody)
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
/**
* post方法,使用json
*
* @param url 接口链接
* @param json 请求体
* @return
* @throws IOException
*/
public String post(String url, String json) throws IOException {
RequestBody body = RequestBody.create(JSON, json);
Request request = new Request.Builder().url(url).post(body).build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("错误码:" + response);
}
}
/**
* put方法,使用map传递键值对
* @param url 接口链接
* @param map 键值对
* @return
* @throws IOException
*/
public String put(String url, Map map) throws IOException {
FormBody.Builder build = new FormBody.Builder();
for (Map.Entry item : map.entrySet()) {
build.add(String.valueOf(item.getKey()), String.valueOf(item.getValue()));
}
RequestBody formBody = build.build();
Request request = new Request.Builder()
.url(url)
.put(formBody)
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
/**
* put方法,使用json
* @param url 接口链接
* @param json 键值对
* @return
* @throws IOException
*/
public String put(String url, String json) throws IOException {
RequestBody body = RequestBody.create(JSON, json);
Request request = new Request.Builder().url(url).put(body).build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("错误码:" + response);
}
}
/**
* put方法,使用json
* @param url 接口链接
* @param
* @return
* @throws IOException
*/
public String tupian(String url, Map map ,File file) throws IOException {
RequestBody body = RequestBody.create(MEDIA_TYPE_PNG, file);
FormBody.Builder build = new FormBody.Builder();
for (Map.Entry item : map.entrySet()) {
build.add(item.getKey(), item.getValue());
}
RequestBody formBody = build.build();
Request request = new Request.Builder()
.url(url)
.put(body)
.put(formBody)
.build();
Response response = client.newCall(request).execute();
if (response.isSuccessful()) {
System.out.println("");
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
/**
* put方法,使用json
* @param
* @param
* @param
* @return
* @throws
*/
public String postFile( String url, Map map, File file) throws IOException {
final MediaType MEDIA_TYPE_PNG = MediaType.parse("image/jpg");
OkHttpClient client2 = new OkHttpClient();
FormBody.Builder build = new FormBody.Builder();
for (Map.Entry item : map.entrySet()) {
build.add(item.getKey(), item.getValue());
}
MultipartBody requestBody = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("file",file.getName(),RequestBody.create(MEDIA_TYPE_PNG, file))
.addFormDataPart("build", String.valueOf(build))
.build();
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.build();
Response response = client2.newCall(request).execute();
if (response.isSuccessful()) {
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
/**
*根据session_id,file 上传图片;
*
*/
public String post_file(String url, String session_id, File file) throws IOException {
final MediaType MEDIA_TYPE_PNG = MediaType.parse("image/jpg");
OkHttpClient client2 = new OkHttpClient();
MultipartBody requestBody = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("session_id",session_id)
.addFormDataPart("file",file.getName(),RequestBody.create(MEDIA_TYPE_PNG, file)).build();
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.build();
Response response = client2.newCall(request).execute();
if (response.isSuccessful()) {
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
/**
*根据session_id,file 上传图片 数组;
*
*/
public String post_files( String url, String sessionid,String leader_id, File file) throws IOException {
final MediaType MEDIA_TYPE_PNG = MediaType.parse("image/jpg");
OkHttpClient client2 = new OkHttpClient();
MultipartBody requestBody = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("session_id",sessionid)
.addFormDataPart("leader_id",leader_id)
.addFormDataPart("images",file.getName(),RequestBody.create(MEDIA_TYPE_PNG, file)).build();
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.build();
Response response = client2.newCall(request).execute();
if (response.isSuccessful()) {
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
public String TescherPost_File(String url, String session_id, File file) throws IOException {
final MediaType MEDIA_TYPE_PNG = MediaType.parse("image/jpg");
OkHttpClient client2 = new OkHttpClient();
MultipartBody requestBody = new MultipartBody.Builder().setType(MultipartBody.FORM)
.addFormDataPart("session_id",session_id)
.addFormDataPart("file",file.getName(),RequestBody.create(MEDIA_TYPE_PNG, file)).build();
Request request = new Request.Builder()
.url(url)
.post(requestBody)
.build();
Response response = client2.newCall(request).execute();
if (response.isSuccessful()) {
return response.body().string();
} else {
throw new IOException("Unexpected code " + response);
}
}
}
5;创建请求头,可以抓包,也可以根据Api 进行传参。demo如下:
6:进行启动:服务器响应结果:
7:查看测试报告,禁忌在启动测试类之前,要把java类放到test-output下面:
8:先看没有美化前的测试报告:【比较丑】
9:美化后的:
10:如何进行美化呢?
编写工具类:ExtentTestNGReporterListener,代码如下:
package Utils;
import com.aventstack.extentreports.ExtentReports;
import com.aventstack.extentreports.ExtentTest;
import com.aventstack.extentreports.ResourceCDN;
import com.aventstack.extentreports.Status;
import com.aventstack.extentreports.model.TestAttribute;
import com.aventstack.extentreports.reporter.ExtentHtmlReporter;
import com.aventstack.extentreports.reporter.configuration.ChartLocation;
import com.aventstack.extentreports.reporter.configuration.Theme;
import org.testng.*;
import org.testng.xml.XmlSuite;
import java.io.File;
import java.util.*;
import java.util.Date;
public class ExtentTestNGReporterListener implements IReporter {
//生成的路径以及文件名
private static final String OUTPUT_FOLDER = “test-output/”;
private static final String FILE_NAME = “index.html”;
private ExtentReports extent;
public void generateReport(List xmlSuites, List suites, String outputDirectory) {
init();
boolean createSuiteNode = false;
if(suites.size()>1){
createSuiteNode=true;
}
for (ISuite suite : suites) {
Map result = suite.getResults();
//如果suite里面没有任何用例,直接跳过,不在报告里生成
if(result.size()==0){
continue;
}
//统计suite下的成功、失败、跳过的总用例数
int suiteFailSize=0;
int suitePassSize=0;
int suiteSkipSize=0;
ExtentTest suiteTest=null;
//存在多个suite的情况下,在报告中将同一个suite的测试结果归为一类,创建一级节点。
if(createSuiteNode){
suiteTest = extent.createTest(suite.getName()).assignCategory(suite.getName());
}
boolean createSuiteResultNode = false;
if(result.size()>1){
createSuiteResultNode=true;
}
for (ISuiteResult r : result.values()) {
ExtentTest resultNode;
ITestContext context = r.getTestContext();
if(createSuiteResultNode){
//没有创建suite的情况下,将在SuiteResult的创建为一级节点,否则创建为suite的一个子节点。
if( null == suiteTest){
resultNode = extent.createTest(r.getTestContext().getName());
}else{
resultNode = suiteTest.createNode(r.getTestContext().getName());
}
}else{
resultNode = suiteTest;
}
if(resultNode != null){
resultNode.getModel().setName(suite.getName()+" : "+r.getTestContext().getName());
if(resultNode.getModel().hasCategory()){
resultNode.assignCategory(r.getTestContext().getName());
}else{
resultNode.assignCategory(suite.getName(),r.getTestContext().getName());
}
resultNode.getModel().setStartTime(r.getTestContext().getStartDate());
resultNode.getModel().setEndTime(r.getTestContext().getEndDate());
//统计SuiteResult下的数据
int passSize = r.getTestContext().getPassedTests().size();
int failSize = r.getTestContext().getFailedTests().size();
int skipSize = r.getTestContext().getSkippedTests().size();
suitePassSize += passSize;
suiteFailSize += failSize;
suiteSkipSize += skipSize;
if(failSize>0){
resultNode.getModel().setStatus(Status.FAIL);
}
resultNode.getModel().setDescription(String.format("Pass: %s ; Fail: %s ; Skip: %s ;",passSize,failSize,skipSize));
}
buildTestNodes(resultNode,context.getFailedTests(), Status.FAIL);
buildTestNodes(resultNode,context.getSkippedTests(), Status.SKIP);
buildTestNodes(resultNode,context.getPassedTests(), Status.PASS);
}
if(suiteTest!= null){
suiteTest.getModel().setDescription(String.format("Pass: %s ; Fail: %s ; Skip: %s ;",suitePassSize,suiteFailSize,suiteSkipSize));
if(suiteFailSize>0){
suiteTest.getModel().setStatus(Status.FAIL);
}
}
}
extent.flush();
}
private void init() {
//文件夹不存在的话进行创建
File reportDir= new File(OUTPUT_FOLDER);
if(!reportDir.exists()&& !reportDir .isDirectory()){
reportDir.mkdir();
}
ExtentHtmlReporter htmlReporter = new ExtentHtmlReporter(OUTPUT_FOLDER + FILE_NAME);
//设置静态文件的DNS
//解决cdn.rawgit.com访问不了的情况.
htmlReporter.config().setResourceCDN(ResourceCDN.EXTENTREPORTS);
htmlReporter.config().setDocumentTitle("自动化接口测试报告");
htmlReporter.config().setReportName("自动化接口测试报告");
htmlReporter.config().setChartVisibilityOnOpen(true);
htmlReporter.config().setTestViewChartLocation(ChartLocation.TOP);
htmlReporter.config().setTheme(Theme.STANDARD);
htmlReporter.config().setEncoding("UTF-8");
htmlReporter.config().setCSS(".node.level-1 ul{ display:none;} .node.level-1.active ul{display:block;}");
extent = new ExtentReports();
extent.attachReporter(htmlReporter);
extent.setReportUsesManualConfiguration(true);
}
private void buildTestNodes(ExtentTest extenttest, IResultMap tests, Status status) {
//存在父节点时,获取父节点的标签
String[] categories=new String[0];
if(extenttest != null ){
List categoryList = extenttest.getModel().getCategoryContext().getAll();
categories = new String[categoryList.size()];
for(int index=0;index 0) {
//调整用例排序,按时间排序
Set treeSet = new TreeSet(new Comparator() {
public int compare(ITestResult o1, ITestResult o2) {
return o1.getStartMillis()0){
if(name.length()>50){
name= name.substring(0,49)+"...";
}
}else{
name = result.getMethod().getMethodName();
}
if(extenttest==null){
test = extent.createTest(name);
test = extenttest.createNode("");
}else{
//作为子节点进行创建时,设置同父节点的标签一致,便于报告检索。
test = extenttest.createNode(name).assignCategory(categories);
}
for (String group : result.getMethod().getGroups())
test.assignCategory(group);
List outputList = Reporter.getOutput(result);
for(String output:outputList){
//将用例的log输出报告中
test.debug(output);
}
if (result.getThrowable() != null) {
test.log(status, result.getThrowable());
}
else {
test.log(status, "Test " + status.toString().toLowerCase() + "ed");
}
test.getModel().setStartTime(getTime(result.getStartMillis()));
test.getModel().setEndTime(getTime(result.getEndMillis()));
}
}
}
private Date getTime(long millis) {
Calendar calendar = Calendar.getInstance();
calendar.setTimeInMillis(millis);
return calendar.getTime();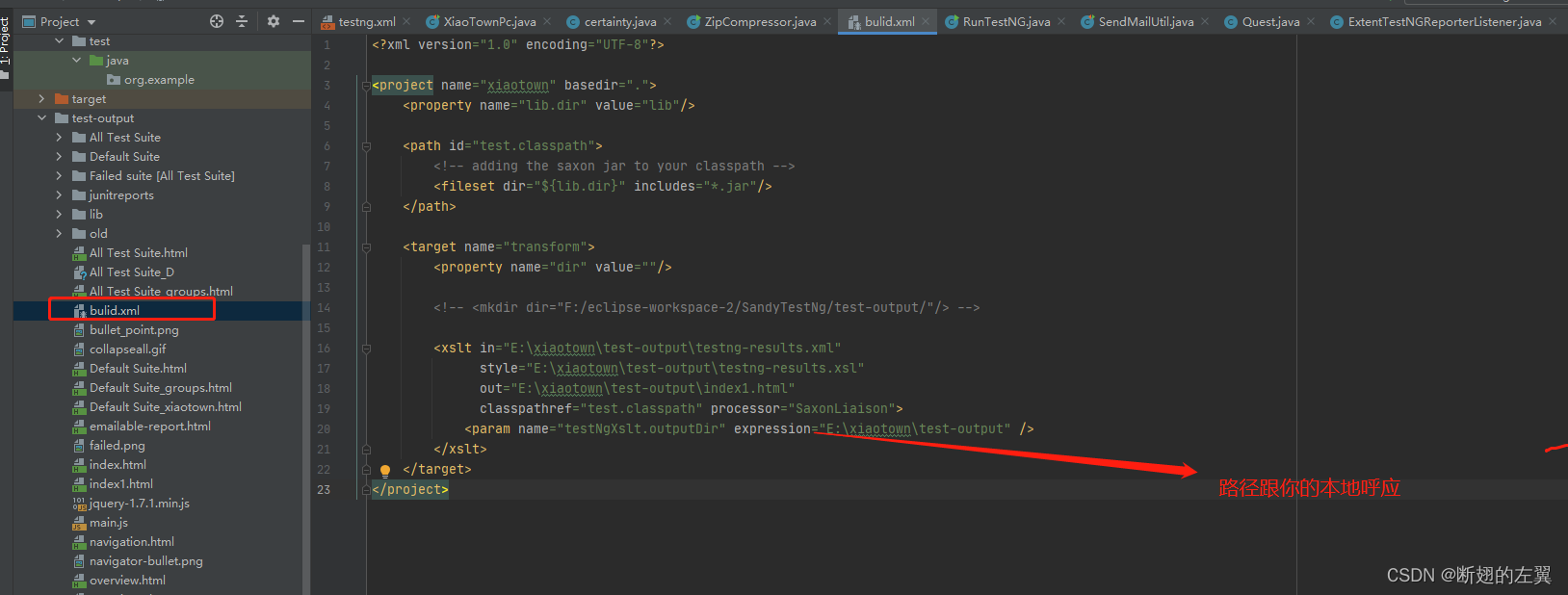
}
}
11:找到test-output,下面的bulid.xml 进行配置:
12:运行bulid.xml,后,在test-output下面找到index1.html,使用浏览器打开: