01.百味窗图像动画效果
Bitmap对象的GetPixel()方法的应用
Bitmap对象的SetPixel()方法的应用
代码
Bitmap MyBitmap;
//
事件公用变量
private
void
button1_Click(
object
sender, EventArgs e)
{
openFileDialog1.Filter
=
//
设置打开图像的类型
"
*.jpg,*.jpeg,*.bmp,*.gif,*.ico,*png,*.tif,*.wmf|*.jpg;*.jpeg;*.bmp;*.gif;*.ico;*.png;*.tif;*.wmf
"
;
this
.openFileDialog1.ShowDialog();
//
打开对话框
if
(
this
.openFileDialog1.FileName.Trim()
==
""
)
return
;
try
{
Bitmap SrcBitmap
=
new
Bitmap(
this
.openFileDialog1.FileName);
//
把打开的图像赋给Bitmap变量
MyBitmap
=
new
Bitmap(SrcBitmap,
this
.pictureBox1.Width,
this
.pictureBox1.Height);
this
.pictureBox1.Image
=
MyBitmap;
//
在控件上显示图像
}
catch
(Exception Err)
{
MessageBox.Show(
this
,
"
打开图像文件错误!
"
,
"
信息提示
"
,
//
提示对话框
MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
private
void
button2_Click(
object
sender, EventArgs e)
{
MyBitmap
=
(Bitmap)
this
.pictureBox1.Image.Clone();
//
为公用变量MyBitmap赋值
int
dh
=
MyBitmap.Height
/
20
;
//
定义变量并赋值
int
dw
=
MyBitmap.Width;
Graphics g
=
this
.pictureBox1.CreateGraphics();
//
定义Graphics对象案例
g.Clear(Color.Gray);
Point[] MyPoint
=
new
Point[
20
];
//
定义点数组
for
(
int
y
=
0
; y
<
20
; y
++
)
//
利用For循环为点数组赋值
{
MyPoint[y].X
=
0
;
MyPoint[y].Y
=
y
*
dh;
}
Bitmap bitmap
=
new
Bitmap(MyBitmap.Width, MyBitmap.Height);
for
(
int
i
=
0
; i
<
dh; i
++
)
{
for
(
int
j
=
0
; j
<
20
; j
++
)
{
for
(
int
k
=
0
; k
<
dw; k
++
)
{
bitmap.SetPixel(MyPoint[j].X
+
k, MyPoint[j].Y
+
i, MyBitmap.GetPixel(MyPoint[j].X
+
k, MyPoint[j].Y
+
i));
}
}
this
.pictureBox1.Refresh();
//
刷新图像
this
.pictureBox1.Image
=
bitmap;
System.Threading.Thread.Sleep(
10
);
}
}
private
void
button3_Click(
object
sender, EventArgs e)
{
MyBitmap
=
(Bitmap)
this
.pictureBox1.Image.Clone();
int
dw
=
MyBitmap.Width
/
30
;
int
dh
=
MyBitmap.Height;
Graphics g
=
this
.pictureBox1.CreateGraphics();
g.Clear(Color.Gray);
Point[] MyPoint
=
new
Point[
30
];
for
(
int
x
=
0
; x
<
30
; x
++
)
{
MyPoint[x].Y
=
0
;
MyPoint[x].X
=
x
*
dw;
}
Bitmap bitmap
=
new
Bitmap(MyBitmap.Width, MyBitmap.Height);
for
(
int
i
=
0
;i
<
dw;i
++
)
{
for
(
int
j
=
0
;j
<
30
;j
++
)
{
for
(
int
k
=
0
;k
<
dh;k
++
)
{
bitmap.SetPixel(MyPoint[j].X
+
i,MyPoint[j].Y
+
k,MyBitmap.GetPixel(MyPoint[j].X
+
i,MyPoint[j].Y
+
k));
}
}
this
.pictureBox1.Refresh();
this
.pictureBox1.Image
=
bitmap;
System.Threading.Thread.Sleep(
10
);
}
}
private
void
button4_Click(
object
sender, EventArgs e)
{
this
.Close();
Application.Exit();
}
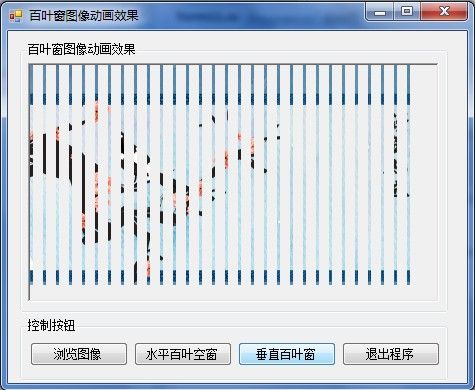
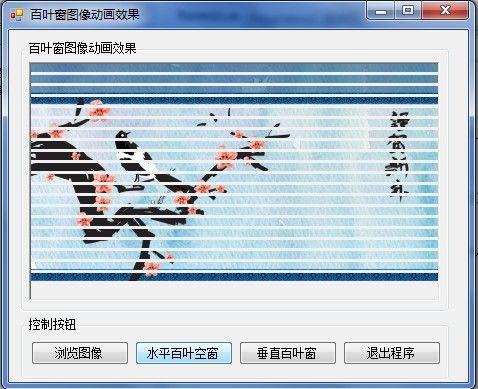
02.翻转和扩展图像动画效果
MessageBox.Show()方法的应用
Graphics对象的DrawImage()方法的应用
代码
Bitmap MyBitmap;
//
事件公用变量
private
void
button1_Click(
object
sender, EventArgs e)
{
openFileDialog1.Filter
=
//
设置打开图像的类型
"
*.jpg,*.jpeg,*.bmp,*.gif,*.ico,*.png,*.tif,*.wmf|*.jpg;*.jpeg;*.bmp;*.gif;*.ico;*.png;*.tif;*.wmf
"
;
this
.openFileDialog1.ShowDialog();
//
打开对话框
if
(
this
.openFileDialog1.FileName.Trim()
==
""
)
return
;
try
{
Bitmap SrcBitmap
=
new
Bitmap(
this
.openFileDialog1.FileName);
//
把打开的图像赋给Bitmap变量
MyBitmap
=
new
Bitmap(SrcBitmap,
this
.pictureBox1.Width,
this
.pictureBox1.Height);
this
.pictureBox1.Image
=
MyBitmap;
//
在控件上显示图像
}
catch
(Exception Err)
{
MessageBox.Show(
this
,
"
打开图像文件错误!
"
,
"
信息提示
"
,
//
提示对话框
MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
private
void
button2_Click(
object
sender, EventArgs e)
{
int
iWidth
=
this
.pictureBox1.Width;
//
图像宽度
int
iHeight
=
this
.pictureBox1.Height;
//
图像高度
Graphics g
=
this
.pictureBox1.CreateGraphics();
//
创建Graphics对象实例
g.Clear(Color.Gray);
//
初始为全灰色
for
(
int
x
=
-
iWidth
/
2
; x
<=
iWidth
/
2
; x
++
)
//
翻转图像
{
Rectangle DestRect
=
new
Rectangle(
0
, iHeight
/
2
-
x, iWidth,
2
*
x);
Rectangle SrcRect
=
new
Rectangle(
0
,
0
, MyBitmap.Width, MyBitmap.Height);
g.DrawImage(MyBitmap, DestRect, SrcRect, GraphicsUnit.Pixel);
System.Threading.Thread.Sleep(
10
);
}
}
private
void
button3_Click(
object
sender, EventArgs e)
{
int
iWidth
=
this
.pictureBox1.Width;
//
图像宽度
int
iHeight
=
this
.pictureBox1.Height;
//
图像高度
Graphics g
=
this
.pictureBox1.CreateGraphics();
//
创建Graphics对象实例
g.Clear(Color.Gray);
//
初始为全灰色
for
(
int
x
=
0
; x
<=
iWidth
/
2
; x
++
)
//
扩展图像
{
Rectangle DestRect
=
new
Rectangle(iWidth
/
2
-
x, iHeight
/
2
-
x,
2
*
x,
2
*
x);
Rectangle SrcRect
=
new
Rectangle(
0
,
0
, MyBitmap.Width, MyBitmap.Height);
g.DrawImage(MyBitmap, DestRect, SrcRect, GraphicsUnit.Pixel);
System.Threading.Thread.Sleep(
10
);
}
}
private
void
button4_Click(
object
sender, EventArgs e)
{
this
.Close();
}
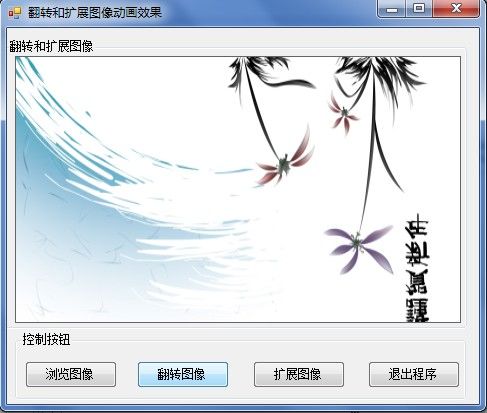
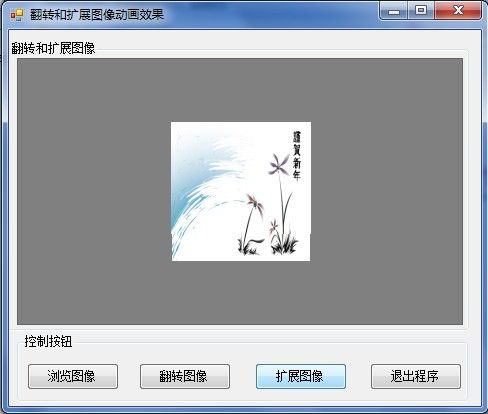
03.图像的纹理和浮雕效果
Bitmap对象的GetPixel()方法的应用
Bitmap对象的SetPixel()方法的应用
BitmapData对象的Scan0属性
Bitmap对象的LockBits()方法,语法结构:
public System.Drawing.Imaging.BitmapData LockBits(
System.Drawing.Rectangle rect,
System.Drawing.Imaging.ImageLockMode flags,
System.Drawing.Imaging.PixelFormat format,
System.Drawing.Imaging.BitmapData bitmapData)
参数意义:
rect:矩形结构,它指定要锁定的System.Drawing.Bitmap的部分。
flags:指定System.Drawing.Bitmap的访问级别(读/写)。
format:一个PixelFormat值,它指示System.Drawing.Bitmap的数据格式。
bitmapData:包含有关锁定操作的信息。
System.Runtime.InteropServices.Marshal类的Copy()方法。
public static void Copy(byte[] source,int startIndex,System.IntPtr destination,int length)
参数意义:
source从中进行复制的一维数据。
startIndex:数据中复制(Copy)开始位置的从0开始的索引。
destination:要复制的内存指针。
length:要复制的数组元素的数目。
代码
Bitmap Mybitmap;
//
事件公用变量
private
void
button1_Click(
object
sender, EventArgs e)
{
openFileDialog1.Filter
=
//
设置打开图像的类型
"
*.jpg,*.jpeg,*.bmp,*.gif,*.ico,*.png,*.tif,*.wmf|*.jpg;*.jpeg;*.bmp;*.gif;*.ico;*.png;*.tif;*.wmf
"
;
this
.openFileDialog1.ShowDialog();
//
打开对话框
if
(
this
.openFileDialog1.FileName.Trim()
==
""
)
return
;
try
{
Bitmap SrcBitmap
=
new
Bitmap(
this
.openFileDialog1.FileName);
//
把打开的图像赋给Bitmap变量
Mybitmap
=
new
Bitmap(SrcBitmap,
this
.pictureBox1.Width,
this
.pictureBox1.Height);
this
.pictureBox1.Image
=
Mybitmap;
//
在控件上显示图像
}
catch
{
MessageBox.Show(
this
,
"
打开图像文件错误!
"
,
"
信息提示
"
,
//
提示对话框
MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
private
void
button2_Click(
object
sender, EventArgs e)
{
Image myImage
=
System.Drawing.Image.FromFile(openFileDialog1.FileName);
Mybitmap
=
new
Bitmap(myImage);
Rectangle rect
=
new
Rectangle(
0
,
0
, Mybitmap.Width, Mybitmap.Height);
//
将指定图像锁定到内存中
System.Drawing.Imaging.BitmapData bmpData
=
Mybitmap.LockBits(rect,
System.Drawing.Imaging.ImageLockMode.ReadWrite,Mybitmap.PixelFormat);
IntPtr ptr
=
bmpData.Scan0;
int
bytes
=
Mybitmap.Width
*
Mybitmap.Height
*
3
;
byte
[] rgbValues
=
new
byte
[bytes];
//
使用RGB值为声明的rgbValues数组赋值
System.Runtime.InteropServices.Marshal.Copy(ptr, rgbValues,
0
, bytes);
for
(
int
counter
=
0
; counter
<
rgbValues.Length; counter
+=
3
)
rgbValues[counter]
=
125
;
//
使用RGB值为图像的像素点着色
System.Runtime.InteropServices.Marshal.Copy(rgbValues,
0
, ptr, bytes);
//
从内存中解锁图像
Mybitmap.UnlockBits(bmpData);
this
.pictureBox1.Image
=
Mybitmap;
}
private
void
button3_Click(
object
sender, EventArgs e)
{
Image myImage
=
System.Drawing.Image.FromFile(openFileDialog1.FileName);
Bitmap myBitmap
=
new
Bitmap(myImage);
//
创建Bitmap对象实例
for
(
int
i
=
0
; i
<
myBitmap.Width
-
1
; i
++
)
{
for
(
int
j
=
0
; j
<
myBitmap.Height
-
1
; j
++
)
{
Color Color1
=
myBitmap.GetPixel(i, j);
//
调用GetPixel方法获取像素点的颜色
Color Color2
=
myBitmap.GetPixel(i
+
1
, j
+
1
);
int
red
=
Math.Abs(Color1.R
-
Color2.R
+
128
);
//
调用绝对值Abs函数
int
green
=
Math.Abs(Color1.G
-
Color2.G
+
128
);
int
blue
=
Math.Abs(Color1.B
-
Color2.B
+
128
);
//
颜色处理
if
(red
>
255
) red
=
255
;
if
(red
<
0
) red
=
0
;
if
(green
>
255
) green
=
255
;
if
(green
<
0
) green
=
0
;
if
(blue
>
255
) blue
=
255
;
if
(blue
<
0
) blue
=
0
;
myBitmap.SetPixel(i, j, Color.FromArgb(red, green, blue));
//
用SetPixel()方法设置像素点的颜色
}
}
this
.pictureBox1.Image
=
myBitmap;
}
private
void
button4_Click(
object
sender, EventArgs e)
{
this
.Close();
Application.Exit();
}
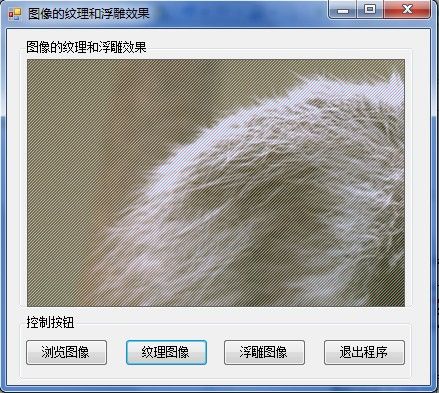
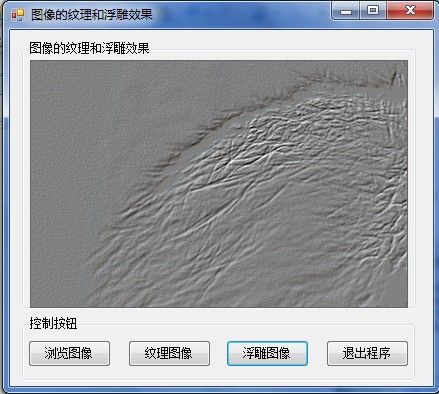
04.图像的马赛克效果
Bitmap对象的SetPixel()方法的应用
随机函数Random的应用
代码
Bitmap Mybitmap;
//
事件公用变量
private
void
button1_Click(
object
sender, EventArgs e)
{
openFileDialog1.Filter
=
//
设置打开图像的类型
"
*.jpg,*.jpeg,*.bmp,*.gif,*.ico,*.png,*.tif,*.wmf|*.jpg;*.jpeg;*.bmp;*.gif;*.ico;*.png;*.tif;*.wmf
"
;
this
.openFileDialog1.ShowDialog();
//
打开对话框
if
(
this
.openFileDialog1.FileName.Trim()
==
""
)
return
;
try
{
Bitmap SrcBitmap
=
new
Bitmap(
this
.openFileDialog1.FileName);
//
把打开的图像赋给Bitmap变量
Mybitmap
=
new
Bitmap(SrcBitmap,
this
.pictureBox1.Width,
this
.pictureBox1.Height);
this
.pictureBox1.Image
=
Mybitmap;
//
在控件上显示图像
}
catch
{
MessageBox.Show(
this
,
"
打开图像文件错误!
"
,
"
信息提示
"
,
//
提示对话框
MessageBoxButtons.OK, MessageBoxIcon.Information);
}
}
private
void
button2_Click(
object
sender, EventArgs e)
{
Image myImage
=
System.Drawing.Image.FromFile(openFileDialog1.FileName);
Mybitmap
=
new
Bitmap(myImage);
//
创建Bitmap对象实例
int
intWidth
=
Mybitmap.Width
/
10
;
//
定义变量并赋值
int
intHeight
=
Mybitmap.Height
/
10
;
Graphics myGraphics
=
this
.CreateGraphics();
myGraphics.Clear(Color.WhiteSmoke);
Point[] myPoint
=
new
Point[
100
];
//
定义点数组
for
(
int
i
=
0
; i
<
10
; i
++
)
//
利用双For循环语句为点数组赋值
{
for
(
int
j
=
0
; j
<
10
; j
++
)
{
myPoint[i
*
10
+
j].X
=
i
*
intWidth;
myPoint[i
*
10
+
j].Y
=
j
*
intHeight;
}
}
Bitmap bitmap
=
new
Bitmap(Mybitmap.Width, Mybitmap.Height);
for
(
int
i
=
0
; i
<
100
; i
++
)
{
Random rand
=
new
Random();
//
随机函数
int
intPos
=
rand.Next(
100
);
for
(
int
m
=
0
; m
<
intWidth; m
++
)
{
for
(
int
n
=
0
; n
<
intHeight; n
++
)
{
bitmap.SetPixel(myPoint[intPos].X
+
m, myPoint[intPos].Y
+
n,
Mybitmap.GetPixel(myPoint[intPos].X
+
m, myPoint[intPos].Y
+
n));
}
}
this
.Refresh();
//
刷新程序
this
.pictureBox1.Image
=
bitmap;
}
for
(
int
k
=
0
; k
<
100
; k
++
)
{
for
(
int
m
=
0
; m
<
intWidth; m
++
)
{
for
(
int
n
=
0
; n
<
intHeight; n
++
)
{
bitmap.SetPixel(myPoint[k].X
+
m, myPoint[k].Y
+
n,
//
调用SetPixel方法
Mybitmap.GetPixel(myPoint[k].X
+
m, myPoint[k].Y
+
n));
}
}
this
.Refresh();
this
.pictureBox1.Image
=
bitmap;
//
在PictureBox控件上显示图像
}
}
private
void
button3_Click(
object
sender, EventArgs e)
{
this
.Close();
}
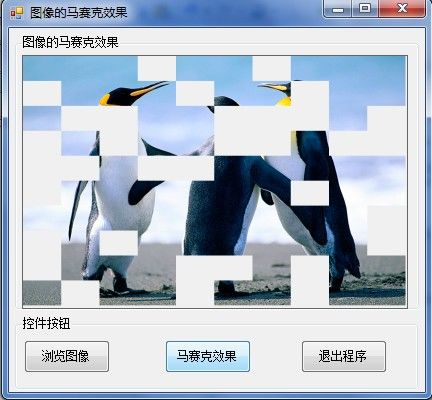
窗体界面设计(完)
心得:c#界面功能强大,窗体界面易变形,展示效果突出。基本语法得定期练习,窗体的把握准确度有待提高。