效果图
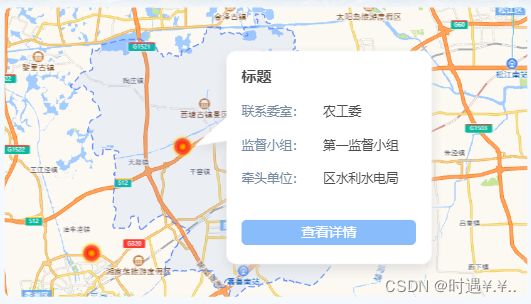
代码如下
main.js 中引入
import VueAMap from 'vue-amap'
Vue.use(VueAMap)
VueAMap.initAMapApiLoader({
key: '5c0afa7353977f66b3880f3c91ca01e4',
plugin: ['AMap.Autocomplete', 'AMap.PlaceSearch', 'AMap.Scale', 'AMap.OverView', 'AMap.ToolBar', 'AMap.MapType', 'AMap.PolyEditor', 'AMap.CircleEditor', 'AMap.Geolocation', 'AMap.DistrictSearch', 'AMap.setPointToCenter'],
uiVersion: '1.0',
v: '1.4.4'
})
组件代码 .vue 文件
<template>
<div class="mapContainerWrap">
<!-- -->
<el-amap ref="elAmap" vid="amapDemo" v-bind="mapConfig" class="map-container" view-mode="3D">
<!-- 地图城市边界配置 -->
<el-amap-polygon v-bind="polygonConfig" :path="polygons.De.path"></el-amap-polygon>
<!-- 标记点 -->
<el-amap-marker v-for="marker in markers" :key="marker.id" :events="marker.events" :position="marker.position" :icon="marker.icon" />
<!-- 消息窗体 -->
<el-amap-info-window v-if="showWindow" ref="windowRef" :position="window.position" :visible="true" :offset="[225, 210]">
<div class="window-ctn">
<div class="window">
<div class="li title">标题</div>
<div class="li">
<div class="label">联系委室:</div>
<div class="value">农工委 </div>
</div>
<div class="li">
<div class="label">监督小组:</div>
<div class="value">第一监督小组 </div>
</div>
<div class="li">
<div class="label">牵头单位:</div>
<div class="value">区水利水电局 </div>
</div>
<div class="btn">查看详情</div>
</div>
</div>
</el-amap-info-window>
</el-amap>
</div>
</template>
<script>
import * as api from '@/api/station'
export default {
name: 'VueAmap',
data() {
return {
mapConfig: {
center: [120.96, 30.90],
zoom: 11,
city: '嘉善县',
zoomEnable: true,
dragEnable: true,
features: ['bg', 'road', 'point'],
mapStyle: 'amap://styles/normal',
events: this.eventsFun(),
},
polygonConfig: {
strokeColor: '#5D87FB',
strokeWeight: 2,
strokeStyle: 'dashed',
fillColor: '#5D87FB',
fillOpacity: 0.1
},
polygons: {
De: {}
},
district: null,
markers: [],
lnglats: [
{
title: '标题',
bigUnit: '农工委',
group: ' 第一监督小组',
unit: '区水利水电局',
visible:true,
id:123,
lng: 120.86,
lat: 30.90
},
{
title: '标题',
bigUnit: '农工委',
group: ' 第一监督小组',
unit: '区水利水电局',
id:456,
visible:false,
lng: 120.76,
lat: 30.80
}
],
markers: [],
window: { visible: false },
showWindow: false,
iconPoint: require('@/assets/images/home/map-point.png')
}
},
mounted() {
this.mapInit()
},
methods: {
mapInit() {
},
createBorder() {
if (!this.district) {
var opts = {
subdistrict: 0,
extensions: "all",
};
this.district = new AMap.DistrictSearch(opts);
}
this.district.search(this.mapConfig.city, (status, result) => {
this.polygons = [];
var bounds = result.districtList[0].boundaries;
if (bounds) {
this.polygons = new AMap.Polygon({
path: bounds[0],
});
}
AMap.Polygon.bind(this.polygons);
});
},
createMark() {
let icon = new AMap.Icon({
size: new window.AMap.Size(43, 60),
image: this.iconPoint,
imageSize: new window.AMap.Size(43, 60)
})
const that = this
this.lnglats.map((item, index) => {
const marker = {
position: [item.lng, item.lat],
icon,
events: {
click() {
that.window = JSON.parse(JSON.stringify(that.markers[index]))
that.window.visible = false
that.showWindow = false
setTimeout(() => {
that.window.visible = true
that.showWindow = true
}, 50)
} }
}
this.markers.push(marker)
console.log(this.markers)
})
this.$nextTick(() => {
setTimeout(() => {
this.$refs.elAmap.$$getInstance().setFitView()
}, 1000)
})
},
eventsFun() {
const that = this
return {
complete() {
that.createBorder()
that.createMark()
}
}
}
}
}
</script>
<style lang='scss' scoped>
.map-container,
.mapContainerWrap {
height: 100%;
width: 100%;
border-radius: 13px;
overflow: hidden;
}
.map-container {
transform: scale(1.28);
}
.mapContainerWrap {
::v-deep .amap-info-content {
background: transparent;
box-shadow: none;
padding: 0;
&:hover {
box-shadow: none;
}
}
::v-deep .amap-info-sharp {
display: none;
}
.window-ctn {
width: 400px;
height: 380px;
background: url('~@/assets/images/home/bg-wind.png') 0 0 /100% 100% no-repeat;
padding: 40px 40px 54px 82px;
.window {
display: flex;
flex-flow: column;
height: 100%;
.li-wrap{
flex: 1;
overflow: auto;
}
.li {
margin-bottom: 15px;
display: flex;
font-size: 24px;
&.title {
font-size: 24px;
font-weight: bold;
}
.label {
color: #778ca2;
flex: none;
}
}
.btn {
margin-top: auto;
background: #88bcfb;
color: #fff;
height: 46px;
display: flex;
align-items: center;
justify-content: center;
border-radius: 8px;
font-size: 22px;
}
}
}
}
</style>
<style lang="scss">
.amap-logo {
display: none;
opacity: 0 !important;
}
.amap-copyright {
opacity: 0;
}
</style>