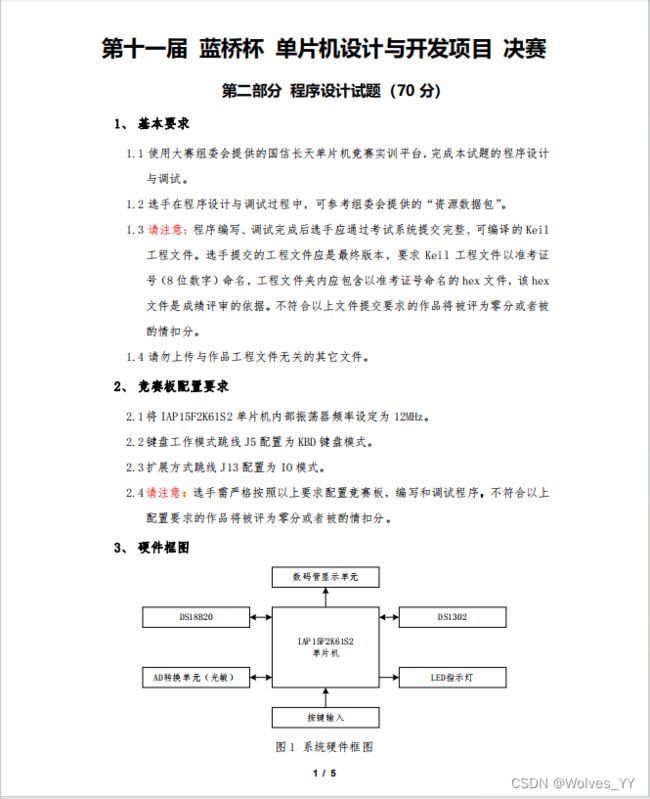
iic.c
/* # I2C代码片段说明
1. 本文件夹中提供的驱动代码供参赛选手完成程序设计参考。
2. 参赛选手可以自行编写相关代码或以该代码为基础,根据所选单片机类型、运行速度和试题
中对单片机时钟频率的要求,进行代码调试和修改。
*/
#include
#include "iic.h"
#include "intrins.h"
sbit scl = P2^0;
sbit sda = P2^1;
#define DELAY_TIME 5
//
static void I2C_Delay(unsigned char n)
{
do
{
_nop_();_nop_();_nop_();_nop_();_nop_();
_nop_();_nop_();_nop_();_nop_();_nop_();
_nop_();_nop_();_nop_();_nop_();_nop_();
}
while(n--);
}
//
void I2CStart(void)
{
sda = 1;
scl = 1;
I2C_Delay(DELAY_TIME);
sda = 0;
I2C_Delay(DELAY_TIME);
scl = 0;
}
//
void I2CStop(void)
{
sda = 0;
scl = 1;
I2C_Delay(DELAY_TIME);
sda = 1;
I2C_Delay(DELAY_TIME);
}
//
void I2CSendByte(unsigned char byt)
{
unsigned char i;
for(i=0; i<8; i++){
scl = 0;
I2C_Delay(DELAY_TIME);
if(byt & 0x80){
sda = 1;
}
else{
sda = 0;
}
I2C_Delay(DELAY_TIME);
scl = 1;
byt <<= 1;
I2C_Delay(DELAY_TIME);
}
scl = 0;
}
//
unsigned char I2CReceiveByte(void)
{
unsigned char da;
unsigned char i;
for(i=0;i<8;i++){
scl = 1;
I2C_Delay(DELAY_TIME);
da <<= 1;
if(sda)
da |= 0x01;
scl = 0;
I2C_Delay(DELAY_TIME);
}
return da;
}
//
unsigned char I2CWaitAck(void)
{
unsigned char ackbit;
scl = 1;
I2C_Delay(DELAY_TIME);
ackbit = sda;
scl = 0;
I2C_Delay(DELAY_TIME);
return ackbit;
}
//
void I2CSendAck(unsigned char ackbit)
{
scl = 0;
sda = ackbit;
I2C_Delay(DELAY_TIME);
scl = 1;
I2C_Delay(DELAY_TIME);
scl = 0;
sda = 1;
I2C_Delay(DELAY_TIME);
}
unsigned int Read_v()
{
unsigned int temp;
I2CStart();
I2CSendByte(0x90);
I2CWaitAck();
I2CSendByte(0x01);
I2CWaitAck();
I2CStart();
I2CSendByte(0x91);
I2CWaitAck();
temp = I2CReceiveByte();
I2CSendAck(1);
I2CStop();
return temp;
}
iic.h
#ifndef __iic_h
#define __iic_h
static void I2C_Delay(unsigned char n);
void I2CStart(void);
void I2CStop(void);
void I2CSendByte(unsigned char byt);
unsigned char I2CReceiveByte(void);
unsigned char I2CWaitAck(void);
void I2CSendAck(unsigned char ackbit);
unsigned int Read_v();
#endif
onewire.c
/* # 单总线代码片段说明
1. 本文件夹中提供的驱动代码供参赛选手完成程序设计参考。
2. 参赛选手可以自行编写相关代码或以该代码为基础,根据所选单片机类型、运行速度和试题
中对单片机时钟频率的要求,进行代码调试和修改。
*/
//
#include
#include "onewire.h"
sbit DQ = P1^4;
void Delay_OneWire(unsigned int t)
{
unsigned char i;
while(t--){
for(i=0;i<12;i++);
}
}
//
void Write_DS18B20(unsigned char dat)
{
unsigned char i;
for(i=0;i<8;i++)
{
DQ = 0;
DQ = dat&0x01;
Delay_OneWire(5);
DQ = 1;
dat >>= 1;
}
Delay_OneWire(5);
}
//
unsigned char Read_DS18B20(void)
{
unsigned char i;
unsigned char dat;
for(i=0;i<8;i++)
{
DQ = 0;
dat >>= 1;
DQ = 1;
if(DQ)
{
dat |= 0x80;
}
Delay_OneWire(5);
}
return dat;
}
//
bit init_ds18b20(void)
{
bit initflag = 0;
DQ = 1;
Delay_OneWire(12);
DQ = 0;
Delay_OneWire(80);
DQ = 1;
Delay_OneWire(10);
initflag = DQ;
Delay_OneWire(5);
return initflag;
}
unsigned int Read_temp()
{
unsigned char LSB ,MSB;
init_ds18b20();
Write_DS18B20(0xcc);
Write_DS18B20(0x44);
init_ds18b20();
Write_DS18B20(0xcc);
Write_DS18B20(0xbe);
LSB = Read_DS18B20();
MSB = Read_DS18B20();
return (MSB << 8 | LSB ) * 0.625;
}
onewire.h
#ifndef __onewire_h
#define __onewire_h
void Delay_OneWire(unsigned int t);
void Write_DS18B20(unsigned char dat);
unsigned char Read_DS18B20(void);
bit init_ds18b20(void);
unsigned int Read_temp();
#endif
ds1302.c
/* # DS1302代码片段说明
1. 本文件夹中提供的驱动代码供参赛选手完成程序设计参考。
2. 参赛选手可以自行编写相关代码或以该代码为基础,根据所选单片机类型、运行速度和试题
中对单片机时钟频率的要求,进行代码调试和修改。
*/
//
#include
#include "ds1302.h"
#include "intrins.h"
sbit SCK = P1^7;
sbit SDA = P2^3;
sbit RST = P1^3;
code unsigned char Write_addr[] = {0x80,0x82,0x84,0x86,0x88,0x8a,0x8c};
code unsigned char Read_addr[] = {0x81,0x83,0x85,0x87,0x89,0x8b,0x8d};
unsigned char time[] = {0x50,0x59,0x16};
void Write_Ds1302(unsigned char temp)
{
unsigned char i;
for (i=0;i<8;i++)
{
SCK = 0;
SDA = temp&0x01;
temp>>=1;
SCK=1;
}
}
//
void Write_Ds1302_Byte( unsigned char address,unsigned char dat )
{
RST=0; _nop_();
SCK=0; _nop_();
RST=1; _nop_();
Write_Ds1302(address);
Write_Ds1302(dat);
RST=0;
}
//
unsigned char Read_Ds1302_Byte ( unsigned char address )
{
unsigned char i,temp=0x00;
RST=0; _nop_();
SCK=0; _nop_();
RST=1; _nop_();
Write_Ds1302(address);
for (i=0;i<8;i++)
{
SCK=0;
temp>>=1;
if(SDA)
temp|=0x80;
SCK=1;
}
RST=0; _nop_();
SCK=0; _nop_();
SCK=1; _nop_();
SDA=0; _nop_();
SDA=1; _nop_();
return (temp);
}
void Write_time()
{
char i;
Write_Ds1302_Byte(0x8e,0x00);
for(i = 0;i < 3;i++)
Write_Ds1302_Byte(Write_addr[i],time[i]);
Write_Ds1302_Byte(0x8e,0x80);
}
void Read_time()
{
char i;
for(i = 0;i < 3;i++)
time[i] = Read_Ds1302_Byte(Read_addr[i]);
}
ds1302.h
#ifndef __ds1302_h
#define __ds1302_h
void Write_Ds1302(unsigned char temp) ;
void Write_Ds1302_Byte( unsigned char address,unsigned char dat ) ;
unsigned char Read_Ds1302_Byte ( unsigned char address );
void Write_time();
void Read_time();
#endif
sys.c
#include
#include "sys.h"
#include "intrins.h"
void Delay_ms(unsigned int t) //@12.000MHz
{
while(t--)
{
unsigned char i, j;
i = 12;
j = 169;
do
{
while (--j);
} while (--i);
}
}
void Select_Hc573(char n)
{
switch(n)
{
case 4:P2 = P2 & 0x1f | 0x80;break;
case 5:P2 = P2 & 0x1f | 0xa0;break;
case 6:P2 = P2 & 0x1f | 0xc0;break;
case 7:P2 = P2 & 0x1f | 0xe0;break;
}
P2 = P2 & 0x1f;
}
void Sys_Init()
{
P0 = 0x00;
Select_Hc573(5);
P0 = 0xff;
Select_Hc573(4);
}
void Select_Bit(unsigned char pos,dat)
{
P0 = 0x01 << pos;
Select_Hc573(6);
P0 = dat;
Select_Hc573(7);
Delay_ms(1);
P0 = 0xff;
Select_Hc573(7);
}
sys.h
#ifndef __sys_h
#define __sys_h
void Delay_ms(unsigned int t);
void Select_Hc573(char n);
void Sys_Init();
void Select_Bit(unsigned char pos,dat);
#endif
main.c
#include
#include "ds1302.h"
#include "iic.h"
#include "onewire.h"
#include "sys.h"
sbit R1 = P3^0;
sbit R2 = P3^1;
sbit R3 = P3^2;
sbit R4 = P3^3;
sbit C1 = P4^4;
sbit C2 = P4^2;
sbit C3 = P3^5;
sbit C4 = P3^4;
code unsigned char SMG[] = { ~0x3F,~0x06,~0x5B,~0x4F,~0x66,~0x6D,~0x7D,~0x07,~0x7F,~0x6F,~0x71,~0x76};
extern unsigned char time[];
unsigned int temp;//温度
unsigned int v;//电压
bit v_stata;// 1-亮 0-暗
bit flag_500ms;
bit flag_10ms;
unsigned char count1;
unsigned int count2,count3;
char param_time = 17;//时间参数
char param_temp = 25;//温度参数
char param_led = 4;//led参数
unsigned char key_val;//键值
bit mode;//0-数据界面 1-参数界面
unsigned char mode_dat;//0-时间 1-温度 2-亮暗状态
unsigned char mode_param;//0-时间 1-温度 2-LED
bit L3_flag;// 0-灭 1-亮
void Display_time()//时间界面
{
Select_Bit(0,SMG[time[2] / 16]);
Select_Bit(1,SMG[time[2] % 16]);
Select_Bit(2,SMG[0]);
Select_Bit(3,SMG[time[1] / 16]);
Select_Bit(4,SMG[time[1] % 16]);
Select_Bit(5,SMG[0]);
Select_Bit(6,SMG[time[0] / 16]);
Select_Bit(7,SMG[time[0] % 16]);
}
void Display_temp()//温度界面
{
Select_Bit(0,SMG[10]);
Select_Bit(5,SMG[temp / 100]);
Select_Bit(6,SMG[temp / 10 % 10] - 0x80);
Select_Bit(7,SMG[temp % 10]);
}
void Display_v()//亮暗状态显示
{
Select_Bit(0,SMG[11]);
Select_Bit(2,SMG[v / 100] - 0x80);
Select_Bit(3,SMG[v / 10 % 10]);
Select_Bit(4,SMG[v % 10]);
Select_Bit(7,SMG[v_stata]);
}
void Display_param_time()//时间参数界面
{
Select_Bit(0,SMG[5]);
Select_Bit(1,SMG[4]);
Select_Bit(6,SMG[param_time / 10]);
Select_Bit(7,SMG[param_time % 10]);
}
void Display_param_temp()//温度参数界面
{
Select_Bit(0,SMG[5]);
Select_Bit(1,SMG[5]);
Select_Bit(6,SMG[param_temp / 10]);
Select_Bit(7,SMG[param_temp % 10]);
}
void Display_param_led()//指示灯参数界面
{
Select_Bit(0,SMG[5]);
Select_Bit(1,SMG[6]);
Select_Bit(7,SMG[param_led]);
}
void Timer2Init(void) //10毫秒@12.000MHz
{
AUXR &= 0xFB; //定时器时钟12T模式
T2L = 0xF0; //设置定时初值
T2H = 0xD8; //设置定时初值
AUXR |= 0x10; //定时器2开始计时
IE2 = 1 << 2;
EA = 1;
}
void Timer2_isr() interrupt 12
{
if(++count1 > 50)
{
count1 = 0;
flag_500ms = 1;//光敏电阻半秒刷新
}
flag_10ms = 1;//按键扫描刷新
if(!v_stata)//L3判断
{
if(++count2 > 300)
{
count2 = 0;
L3_flag = 1;
}
count3 = 0;
}
else
{
count2 = 0;
if(++count3 > 300)
{
count3 = 0;
L3_flag = 0;
}
}
}
unsigned char Key_Scan()//按键扫描函数
{
unsigned char temp = 0;
static unsigned char cnt4 = 0;
static unsigned char cnt5 = 0;
static unsigned char cnt8 = 0;
static unsigned char cnt9 = 0;
if(flag_10ms)
{
R3 = 0;
R1 = R2 = R4 = C1 = C2 = C3 = C4 = 1;
if(C1 == 0) cnt5++;
if(C1 == 1)
{
if(cnt5 > 2) temp = 5;
cnt5 = 0;
}
if(C2 == 0) cnt9++;
if(C2 == 1)
{
if(cnt9 > 2) temp = 9;
cnt9 = 0;
}
R4 = 0;
R1 = R2 = R3 = C1 = C2 = C3 = C4 = 1;
if(C1 == 0) cnt4++;
if(C1 == 1)
{
if(cnt4 > 2) temp = 4;
cnt4 = 0;
}
if(C2 == 0) cnt8++;
if(C2 == 1)
{
if(cnt8 > 2) temp = 8;
cnt8 = 0;
}
flag_10ms = 0;
}
return temp;
}
void Key_Pro()
{
switch(key_val)
{
case 4:
mode ^= 1;
if(!mode) mode_param = 0;
else mode_dat = 0;
break;
case 5:
if(!mode)
{
if(++mode_dat > 2)
mode_dat = 0;
}
else
{
if(++mode_param > 2)
mode_param = 0;
}
break;
case 8:
if(mode)
{
switch(mode_param)
{
case 0:
if(--param_time < 0)
param_time = 23;
break;
case 1:
if(--param_temp < 0)
param_temp = 99;
break;
case 2:
if(--param_led < 4)
param_led = 8;
break;
}
}
break;
case 9:
if(mode)
{
switch(mode_param)
{
case 0:
if(++param_time > 23)
param_time = 0;
break;
case 1:
if(++param_temp > 99)
param_temp = 0;
break;
case 2:
if(++param_led > 8)
param_led = 4;
break;
}
}
break;
}
}
void Led(unsigned char addr,enable)//LED底层代码
{
static unsigned char temp = 0x00;
static unsigned char temp_old = 0xff;
if(enable) temp |= 0x01 << addr;
else temp &= ~(0x01 << addr);
if(temp != temp_old)
{
P0 = ~temp;
Select_Hc573(4);
temp_old = temp;
}
}
void Led_Pro()//LED控制代码
{
if(param_time <= time[2] / 16 * 10 + time[2] % 16) Led(0,1);
else Led(0,0);
if(((float)temp) / 10 < param_temp) Led(1,1);
else Led(1,0);
if(L3_flag) Led(2,1);
else Led(2,0);
if(!v_stata)
{
switch(param_led)
{
case 4:
Led(3,1);Led(4,0);Led(5,0);Led(6,0);Led(7,0);
break;
case 5:
Led(4,1);Led(3,0);Led(5,0);Led(6,0);Led(7,0);
break;
case 6:
Led(5,1);Led(4,0);Led(3,0);Led(6,0);Led(7,0);
break;
case 7:
Led(6,1);Led(4,0);Led(5,0);Led(3,0);Led(7,0);
break;
case 8:
Led(7,1);Led(4,0);Led(5,0);Led(6,0);Led(3,0);
break;
}
}
else
{
Led(3,0);Led(4,0);Led(5,0);Led(6,0);Led(7,0);
}
}
void main()
{
Sys_Init();//系统初始化关闭继电器和蜂鸣器
Read_temp();//读取温度延时750ms以消除上电显示85
Delay_ms(750);
Write_time();//初始化写入时间
Timer2Init();//定时器2初始化
while(1)
{
temp = Read_temp();//读取温度
Read_time();//读取时间
if(flag_500ms)//半秒读取一次光敏电阻电压
{
flag_500ms = 0;
v = Read_v() / 51.0 * 100;
}
if(v > 150) v_stata = 1;
else v_stata = 0;
key_val = Key_Scan();//读取键值
Key_Pro();//按键处理函数
Led_Pro();//LED处理函数
if(!mode)//0-数据界面 1-参数界面
{
switch(mode_dat)//0-时间界面 1-温度界面 2-亮暗状态界面
{
case 0:Display_time();break;
case 1:Display_temp();break;
case 2:Display_v();break;
}
}
else//参数界面下
{
switch(mode_param)//0-时间参数界面 1-温度参数界面 2-LED参数界面
{
case 0:Display_param_time();break;
case 1:Display_param_temp();break;
case 2:Display_param_led();break;
}
}
}
}