不错的资料:
LLVM+Clang+编译器+链接器--保值【进阶之路二】 - 掘金
——————————————————————————————————————
下载 llvm-cookbook example:
$ git clone https://github.com/elongbug/llvm-cookbook.git
也可以参照llvm-projec中的官方代码,一样的差不多:
https://github.com/llvm/llvm-project/tree/main/llvm/examples/Kaleidoscope
开发环境:
从apt source 安装llvm、clang和lldb:
$ apt install llvm
$ apt install llvm-dev
$ apt install clang
$ apt install lldb
$ cd llvm-cookbook/Chapter_2
$ make
$ ./toy example1
运行效果:
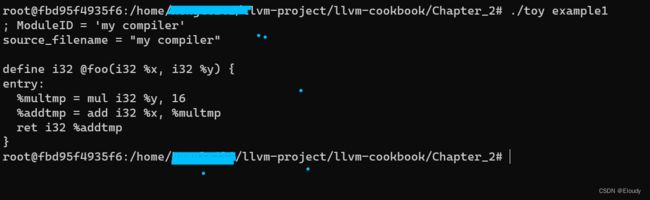
事隔十个版本,llvm的接口如故,还是让人惊讶与当时接口定义的周到;
具体代码如下:
Makefile:
CC = clang++
SOURCE = ch2_toy.cpp
TARGET = toy
$(TARGET) : $(SOURCE)
$(CC) $(SOURCE) -o $(TARGET) -g -O3 `llvm-config --cxxflags --ldflags --system-libs --libs core mcjit native`
clean :
rm $(TARGET)
ch2_toy.cpp:
#include "llvm/IR/DerivedTypes.h"
#include "llvm/IR/IRBuilder.h"
#include "llvm/IR/LLVMContext.h"
#include "llvm/IR/Module.h"
#include "llvm/IR/Verifier.h"
#include
#include
#include
————————————————————————————————————————
一,从源码编译 llvm
下载源码:
$ git clone https://github.com/llvm/llvm-project.git
创建 对应 commit id分支:
$ cd llvm-project
$ git checkout 5b78868661f42a70fa30 -b 17.x.greater
源码成功编译 llvm-project commit id:
~/ex/llvm-project$ git log -1
commit 5b78868661f42a70fa3006b1db41f78a6178d596 (HEAD -> main)
生成构建:
cmake -G "Unix Makefiles" ../llvm \
-DLLVM_ENABLE_PROJECTS=all \
-DLLVM_BUILD_EXAMPLES=ON \
-DLLVM_TARGETS_TO_BUILD="host" \
-DCMAKE_BUILD_TYPE=Release \
-DLLVM_ENABLE_ASSERTIONS=ON \
-DLLVM_ENABLE_RUNTIMES=all \
-DLLVM_BUILD_LLVM_DYLIB=ON \
-DCMAKE_INSTALL_PREFIX=../inst_clanglld_rtall_5b78868661
make -j8
(i9 9900k 8物理core 16logic core, 64GB mem, 64GB swap)
make install
二,编译Chapter2 example
可行的 Makefile:
CC = /home/kleenelan/ex/cookbook_llvm/inst_clanglld_rtall_5b78868661/bin/clang++
SOURCE = ch2_toy.cpp
TARGET = toy
$(TARGET) : $(SOURCE)
$(CC) $(SOURCE) -o $(TARGET) -g `/home/kleenelan/ex/cookbook_llvm/inst_clanglld_rtall_5b78868661/bin/llvm-config --cxxflags --ldflags --system-libs --libs core mcjit native` -I/home/kleenelan/ex/cookbook_llvm/inst_clanglld_rtall_5b78868661/include/c++/v1 -I/home/kleenelan/ex/cookbook_llvm/inst_clanglld_rtall_5b78868661/include/x86_64-unknown-linux-gnu/c++/v1 -L/usr/lib/gcc/x86_64-linux-gnu/11/ -L/home/kleenelan/ex/cookbook_llvm/inst_clanglld_rtall_5b78868661/lib/x86_64-unknown-linux-gnu -lc++
clean :
rm $(TARGET)
ch2_toy.cpp
#include "llvm/IR/DerivedTypes.h"
#include "llvm/IR/IRBuilder.h"
#include "llvm/IR/LLVMContext.h"
#include "llvm/IR/Module.h"
#include "llvm/IR/Verifier.h"
#include
#include
#include
$ make