数据代理+事件处理+计算属性与监视+绑定样式+条件渲染
- 1 数据代理
-
- 1.1 回顾Object.defineProperty方法
- 1.2 数据代理
- 2 事件处理
-
- 2.1 绑定监听
- 2.2 事件修饰符
- 2.3 键盘事件
- 3 计算属性与监视
-
- 3.1 计算属性
- 3.2 监视属性(侦视属性)
- 3.3 watch对比computed
- 4 绑定样式
-
- 4.1 绑定class样式
- 4.2 绑定style样式
- 4.3 总结
- 5 条件渲染
1 数据代理
1.1 回顾Object.defineProperty方法
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>回顾Object.defineProperty方法title>
head>
<body>
<script>
let number = 30
let person = {
name:'张三',
sex:'男'
}
Object.defineProperty(person,'age',{
get(){
console.log('有人读取了age属性');
return number
},
set(value){
console.log('有人修改了age属性,且值是',value);
number = value
}
})
script>
body>
html>
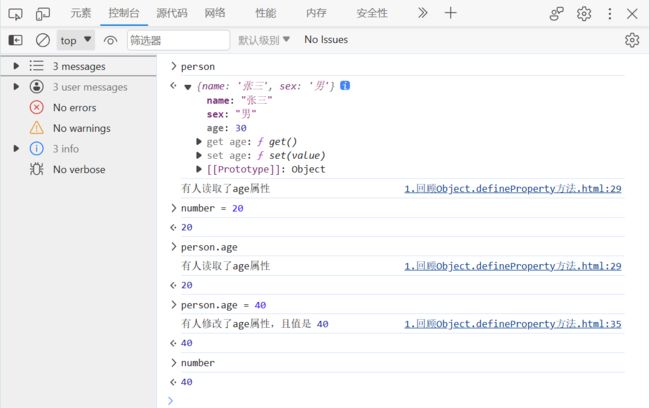
1.2 数据代理
- 数据代理:通过一个对象代理对另一个对象中的属性的操作(读/写)。
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>何为数据代理title>
head>
<body>
<script>
let obj = { x: 100 }
let obj2 = { y: 200 }
Object.defineProperty(obj2,'x',{
get(){
return obj.x
},
set(value){
obj.x = value
}
})
script>
body>
html>
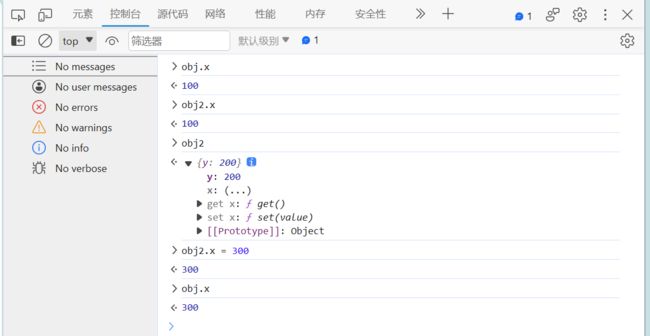
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue中的数据代理title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>学校名称:{{name}}h2>
<h2>学校地址:{{address}}h2>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el: '#root',
data: {
name:'西财',
address:'西安市长安区'
}
})
script>
html>
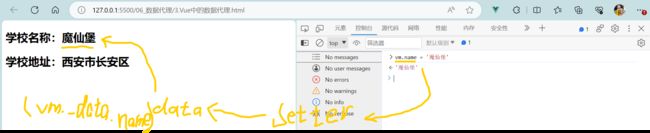
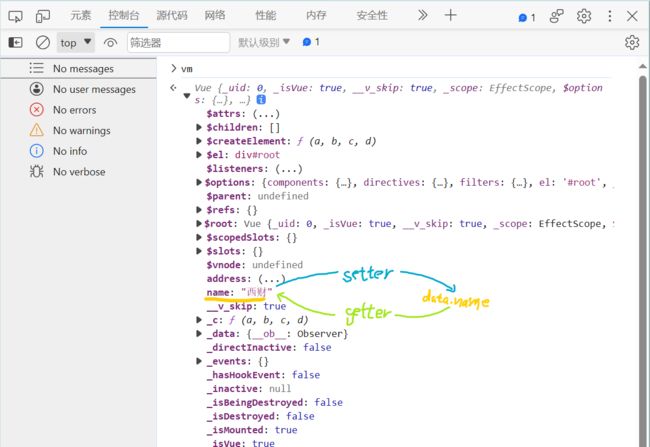
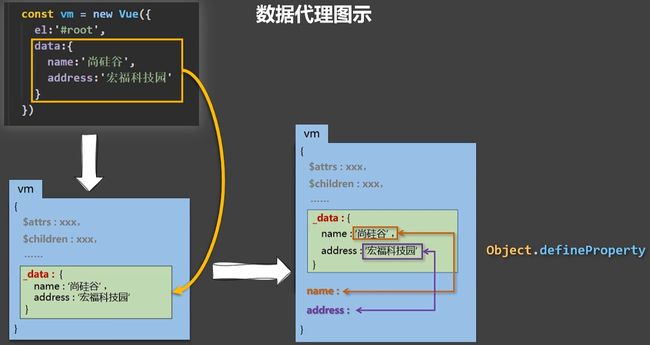
2 事件处理
2.1 绑定监听
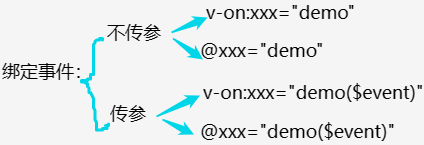
- 默认事件形参:event
- 隐含属性对象:$event
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>事件的基本使用title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>欢迎来到{{name}}的博客h2>
<button @click="showInfo1">点我提示信息1(不传参)button>
<button @click="showInfo2($event,66)">点我提示信息2(传参)button>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
name:'小王'
},
methods:{
showInfo1(event) {
alert('同学你好!')
},
showInfo2(event,number) {
console.log(event,number);
}
}
})
script>
html>
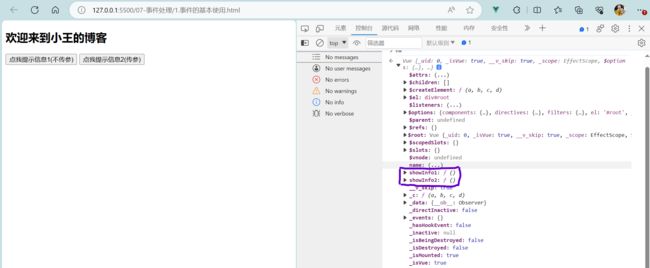
2.2 事件修饰符
- prevent:阻止默认事件(常用)
- stop:阻止事件冒泡(常用)
- once:事件只触发一次(常用)
- capture:使用事件的捕获模式
- self:只有event.target是当前操作的元素时才触发事件
- passive:事件的默认行为立即执行,无需等待事件回调执行完毕
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>事件修饰符title>
<script src="../JS/vue.js">script>
<style>
*{
margin-top: 13px;
}
.demo1{
height: 50px;
background-color: skyblue;
}
.box1{
padding: 5px;
background-color: pink;
}
.box2{
padding: 5px;
background-color: orange;
}
.list{
width: 200px;
height: 200px;
background-color: peru;
overflow: auto;
}
li{
height: 100px;
}
style>
head>
<body>
<div id="root">
<h2>欢迎来到{{name}}的博客h2>
<a href="https://blog.csdn.net/weixin_64875217?type=blog" @click.prevent="showInfo">点我提示信息a>
<div class="demo1" @click="showInfo">
<button @click.stop="showInfo">点我提示信息button>
div>
<button @click.once="showInfo">点我提示信息button>
<div class="box1" @click.capture="showMsg(1)">
div1
<div class="box2" @click="showMsg(2)">
div2
div>
div>
<div class="demo1" @click.self="showInfo">
<button @click="showInfo">点我提示信息button>
div>
<ul @wheel.passive="demo" class="list">
<li>1li>
<li>2li>
<li>3li>
<li>4li>
ul>
div>
body>
<script>
Vue.config.productionTip = false
new Vue({
el: '#root',
data: {
name:'小王',
},
methods: {
showInfo(e) {
alert('同学你好!')
},
showMsg(msg) {
console.log(msg);
},
demo() {
for (let i = 0; i < 100000; i++) {
console.log('#');
}
console.log('累坏了');
}
}
})
script>
html>
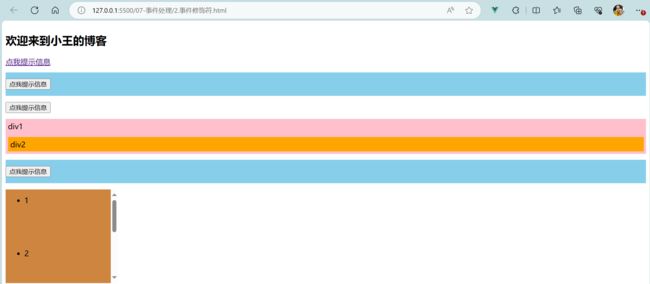
2.3 键盘事件
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>键盘事件title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>欢迎来到{{name}}的博客h2>
<input type="text" placeholder="按下回车提示输入" @keyup.ctrl.y = "showInfo"> // 只有按ctrl+y时才会输出
div>
body>
<script>
Vue.config.productionTip = false
Vue.config.keyCodes.huiche = 13
new Vue({
el:'#root',
data:{
name:'小王'
},
methods:{
showInfo(e){
console.log(e.key,e.keyCode);
}
}
})
script>
html>
3 计算属性与监视
3.1 计算属性
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_插值语法实现title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{firstName.slice(0,3)}}-{{lastName}}span>
div>
body>
<script>
Vue.config.productionTip = false
new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三'
}
})
script>
html>
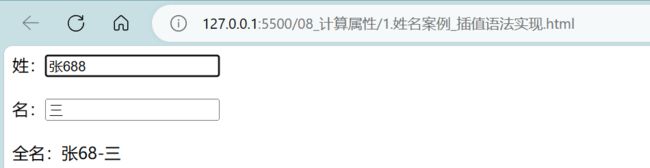
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_methods实现title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{fullName()}}span>
div>
body>
<script>
Vue.config.productionTip = false
new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三'
},
methods:{
fullName(){
return this.firstName + '-' + this.lastName
}
}
})
script>
html>
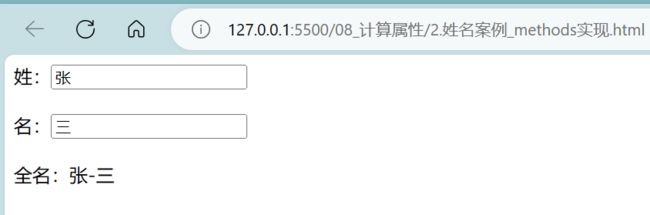
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_计算属性实现title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{fullName}}span>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三'
},
computed:{
fullName:{
get(){
console.log('get被调用了');
return this.firstName + '-' + this.lastName
},
set(value){
console.log('set',value);
const arr = value.split('-')
this.firstName = arr[0]
this.lastName = arr[1]
}
}
}
})
script>
html>
- 姓名案例_计算属性简写实现(确定只读不改,就用简写):
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_计算属性简写实现title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{fullName}}span>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三'
},
computed:{
fullName() {
console.log('get被调用了');
return this.firstName + '-' + this.lastName
}
}
})
script>
html>
3.2 监视属性(侦视属性)
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>今天天气很{{info}}h2>
<button @click = 'isHot = !isHot'>切换天气button>
div>
body>
<script>
Vue.config.productionTip = false
new Vue({
el:'#root',
data:{
isHot:true
},
computed:{
info(){
return this.isHot ? '炎热' : '凉爽'
}
},
methods:{
}
})
script>
html>


DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例_监视属性title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>今天天气很{{info}}h2>
<button @click = 'changeWeather'>切换天气button>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
isHot:true
},
computed:{
info(){
return this.isHot ? '炎热' : '凉爽'
}
},
methods:{
changeWeather(){
this.isHot = !this.isHot
}
},
})
vm.$watch('isHot',{
immediate:true,
handler(newValue,oldValue){
console.log('isHot被修改了',newValue,oldValue);
}
})
script>
html>

DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例_深度监视title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>今天天气很{{info}}h2>
<button @click = 'changeWeather'>切换天气button>
<hr/>
<h3>a的值是{{numbers.a}}h3>
<button @click="numbers.a++">点我让a+1button>
<h3>b的值是{{numbers.b}}h3>
<button @click="numbers.b++">点我让b+1button><br/><br/>
<button @click="numbers = {a:666,b:888}">彻底替换掉numbersbutton>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
isHot:true,
numbers:{
a:1,
b:1
}
},
computed:{
info(){
return this.isHot ? '炎热' : '凉爽'
}
},
methods:{
changeWeather(){
this.isHot = !this.isHot
}
},
watch:{
isHot:{
handler(newValue,oldValue){
console.log('isHot被修改了',newValue,oldValue);
}
},
numbers:{
deep:true,
handler(){
console.log('numbers改变了');
}
}
}
})
script>
html>
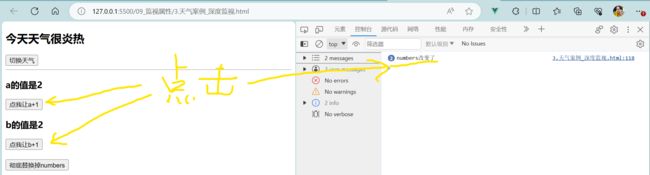
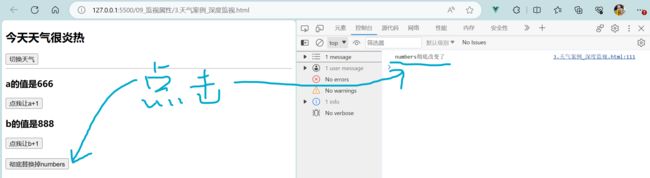
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例_深度监视简写title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>今天天气很{{info}}h2>
<button @click = 'changeWeather'>切换天气button>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
isHot:true,
numbers:{
a:1,
b:1
}
},
computed:{
info(){
return this.isHot ? '炎热' : '凉爽'
}
},
methods:{
changeWeather(){
this.isHot = !this.isHot
}
},
watch:{
}
})
vm.$watch('isHot',function(newValue,oldValue){
console.log('isHot被修改了',newValue,oldValue);
})
script>
html>
3.3 watch对比computed
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_watch实现title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{fullName}}span>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三',
fullName:'张-三'
},
computed:{
},
watch:{
firstName(newValue){
this.fullName = newValue + '-' + this.lastName
},
lastName(newValue){
this.fullName = this.firstName + '-' + newValue
}
}
})
script>
html>
4 绑定样式
4.1 绑定class样式
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>绑定样式title>
<script src="../JS/vue.js">script>
<style>
.basic{
width: 400px;
height: 100px;
border: 1px solid black;
}
.happy{
border: 4px solid red;;
background-color: rgba(255, 255, 0, 0.644);
background: linear-gradient(30deg,yellow,pink,orange,yellow);
}
.sad{
border: 4px dashed rgb(2, 197, 2);
background-color: gray;
}
.normal{
background-color: skyblue;
}
.atguigu1{
background-color: yellowgreen;
}
.atguigu2{
font-size: 30px;
text-shadow:2px 2px 10px red;
}
.atguigu3{
border-radius: 20px;
}
style>
head>
<body>
<div id="root">
<div class="basic" :class="mood" id="demo" @click="changeMood">{{name}}div><br/><br/>
<div class="basic" :class="classArr">{{name}}div><br/><br/>
<div class="basic" :class="classObj">{{name}}div>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
name:'小王',
mood:'normal',
classArr:['atguigu1','atguigu2','atguigu3'],
classObj:{
atguigu1:false,
atguigu2:false
}
},
methods:{
changeMood(){
const arr = ['happy','sad','normal']
const index = Math.floor(Math.random()*3)
this.mood = arr[index]
}
}
})
script>
html>
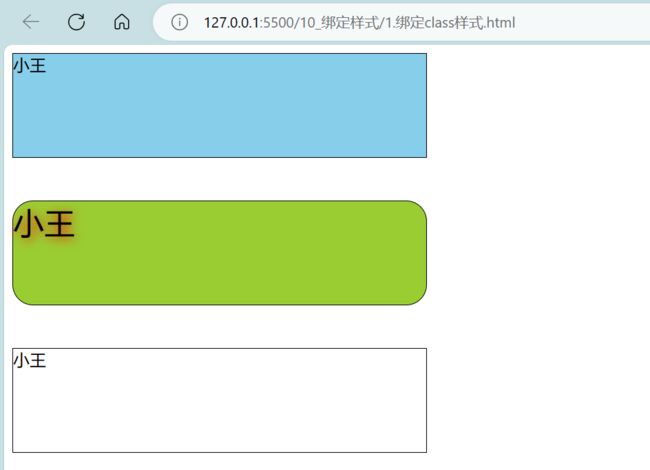
4.2 绑定style样式
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>绑定样式title>
<script src="../JS/vue.js">script>
<style>
.basic{
width: 400px;
height: 100px;
border: 1px solid black;
}
.happy{
border: 4px solid red;;
background-color: rgba(255, 255, 0, 0.644);
background: linear-gradient(30deg,yellow,pink,orange,yellow);
}
.sad{
border: 4px dashed rgb(2, 197, 2);
background-color: gray;
}
.normal{
background-color: skyblue;
}
.atguigu1{
background-color: yellowgreen;
}
.atguigu2{
font-size: 30px;
text-shadow:2px 2px 10px red;
}
.atguigu3{
border-radius: 20px;
}
style>
head>
<body>
<div id="root">
<div class="basic" :style="styleObj">{{name}}div><br/><br/>
<div class="basic" :style="styleArr">{{name}}div>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
name:'小王',
styleObj:{
fontSize: '40px',
color:'skyblue',
},
styleArr:[
{
fontSize: '40px',
color:'skyblue',
},
{
backgroundColor:'orange'
}
]
}
})
script>
html>
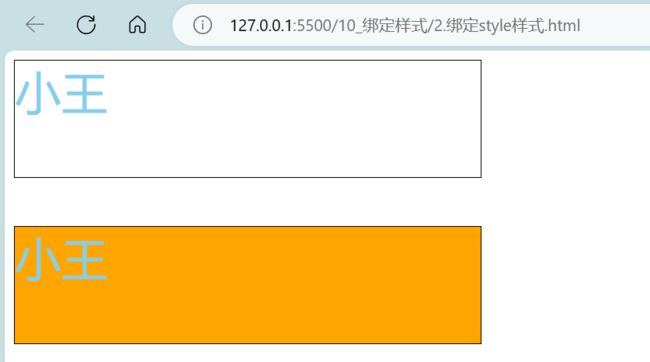
4.3 总结
5 条件渲染
DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>条件渲染title>
<script src="../JS/vue.js">script>
head>
<body>
<div id="root">
<h2>当前的n值是:{{n}}h2>
<button @click="n++">点我n+1button>
<template v-if="n === 1">
<h2>你好h2>
<h2>小王h2>
<h2>魔仙堡h2>
template>
div>
body>
<script>
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
name:'魔仙堡',
n:0
}
})
script>
html>
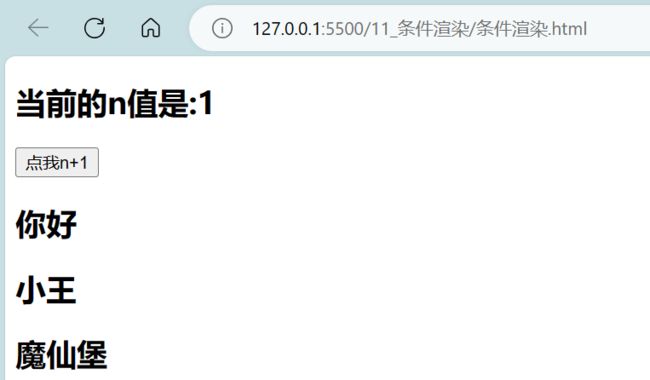