Unity ToLua 之 Lua调用C#(二)
一.ToLua调用C#中的List和Dictionary
- 注意List和dictionary对应的泛型需要先填写到CustomSetting中,然后生成
- XLua 中遍历Dictionary可以使用Pairs,但是ToLua中只能使用迭代器
- ToLua中当使用string为Key时不能通过[]来直接访问对应的Value
public class TestListAndDic
{
public List<string> lst = new List<string>(){"zzs","wy","lzq"};
public Dictionary<int,string> dic = new Dictionary<int, string>()
{
{1,"zzs"},
{2,"wy"},
{3,"lzq"}
};
}
local a = TestListAndDic()
for i = 0, a.lst.Count - 1 do
print(a.lst[i])
end
local list = System.Collections.Generic.List_string()
list:Add("zzs")
print(list[0])
local list2 = System.Collections.Generic.List_int()
list2:Add(999)
print(list2[0])
local iter = a.dic:GetEnumerator()
while iter:MoveNext() do
local v = iter.Current
print(v.Key.."_"..v.Value)
end
local dic1 = System.Collections.Generic.Dictionary_int_string()
dic1:Add(1,"zzs")
print(dic1[1])
local dic2 = System.Collections.Generic.Dictionary_string_int()
dic2:Add("zzs",1)
local b,val = dic2:TryGetValue("zzs",nil)
print(val)
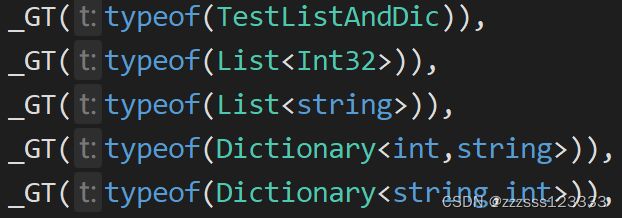
二.ToLua调用C#中的拓展方法
- 拓展方法需要在CustomSettings进行添加绑定
public static class PersonExt
{
public static void Play(this Person person)
{
Debug.Log(person.name+"玩");
}
}
public class Person
{
public string name = "zzs";
public void Eat()
{
Debug.Log(name+"吃饭");
}
}
local p = Person()
p:Eat()
p:Play()

三.ToLua调用C# ref,out参数的函数
- 和XLua非常相似
- Out参数的时候不能直接省略不写
public class Lesson06
{
public int RefFunc(int a, ref int b, ref int c, int d)
{
b = a + d;
c = a - d;
return 100;
}
public int OutFunc(int a, out int b, out int c, int d)
{
b = a + d;
c = a - d;
return 200;
}
public int RefAndOutFunc(int a, ref int b, out int c, int d)
{
b = a + d;
c = a - d;
return 300;
}
}
local a = Lesson06()
local a1,a2,a3 = a:RefFunc(10,0,0,1);
print(a1,a2,a3)
local b1,b2,b3 = a:OutFunc(10,nil,nil,1);
print(b1,b2,b3)
local c1,c2,c3 = a:RefAndOutFunc(10,0,nil,1);
print(c1,c2,c3)