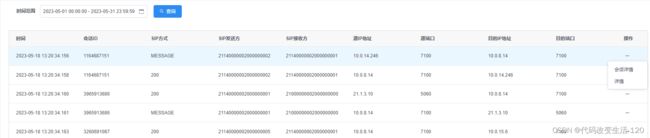
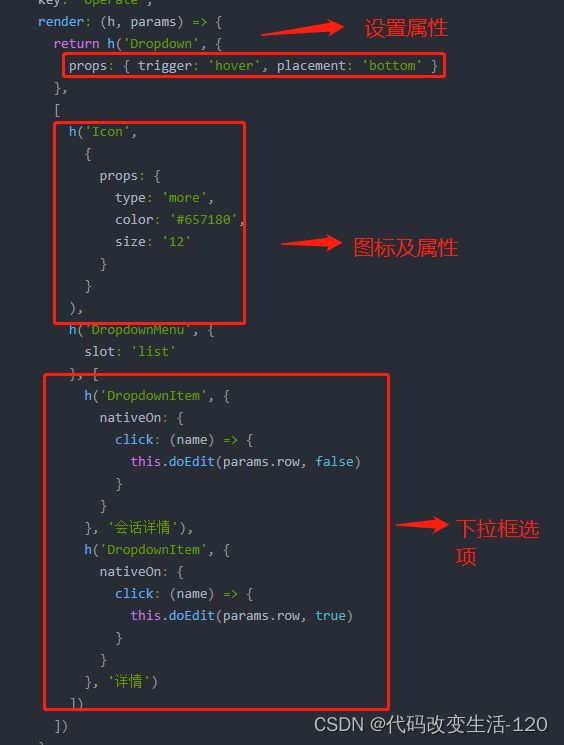
代码段
<template lang="pug">
.wrap
load-mask(:loadMaskShowFlag='loading')
.table-info
sip-condition(
ref='condition',
@query='queryCondition()',
@exportData='exportData()'
)
.table-button
Table.sip-table(:columns='columns', :data='tableData' @on-selection-change="selectChange")
.page
Page(
:total='page.total',
:page-size='page.pageSize',
show-total,
show-sizer,
:current='page.pageNum',
@on-change='changePage',
@on-page-size-change='changeSize'
)
Modal.sip-modal(v-model='showModal', :title='modalTitle' width="70%")
sip(
v-if="!detailFlag"
ref='sip',
@query='queryCondition()'
)
message-details(v-else ref="messageDetails")
div(slot='footer')
</template>
<script>
import util from '@/libs/util'
import indexApi from '@/api/sipApi.js'
import Sip from './components/Sip.vue'
import SipCondition from './components/SipCondition.vue'
import MessageDetails from './components/MessageDetails.vue'
export default {
name: 'SipTable',
components: {
Sip,
SipCondition,
MessageDetails
},
props: {
align: {
type: String,
default: 'left'
}
},
data () {
return {
detailFlag: false,
selectedIds: [],
showModal: false,
modalTitle: '',
loading: false,
page: {
total: 0,
pageNum: 1,
pageSize: 20
},
tableData: [],
columns: [
{
title: '时间',
align: this.align,
key: 'create_date',
render: (h, p) => {
let res = ''
res = this.moment(p.row.create_date).format('YYYY-MM-DD HH:mm:ss.SSS')
return h('div', res)
}
},
{
title: '会话ID',
align: this.align,
key: 'correlation_id',
render: (h, params) => {
return h('div', params.row.correlation_id)
}
},
{
title: 'SIP方式',
align: this.align,
key: 'method',
render: (h, params) => {
return h('div', params.row.method)
}
},
{
title: 'SIP发送方',
align: this.align,
key: 'from_user'
},
{
title: 'SIP接收方',
align: this.align,
key: 'to_user'
},
{
title: '源IP地址',
align: this.align,
key: 'srcIp'
},
{
title: '源端口',
align: this.align,
key: 'srcPort'
},
{
title: '目的IP地址',
align: this.align,
key: 'dstIp'
},
{
title: '目的端口',
align: this.align,
key: 'dstPort'
},
{
title: '操作',
align: this.align,
width: 80,
key: 'operate',
render: (h, params) => {
return h('Dropdown', {
props: { trigger: 'hover', placement: 'bottom' }
},
[
h('Icon',
{
props: {
type: 'more',
color: '#657180',
size: '12'
}
}
),
h('DropdownMenu', {
slot: 'list'
}, [
h('DropdownItem', {
nativeOn: {
click: (name) => {
this.doEdit(params.row, false)
}
}
}, '会话详情'),
h('DropdownItem', {
nativeOn: {
click: (name) => {
this.doEdit(params.row, true)
}
}
}, '详情')
])
])
}
}
]
}
},
mounted () {
this.init()
},
methods: {
init () {
this.query()
},
queryCondition () {
this.showModal = false
this.page.pageNum = 1
this.page.total = 0
this.query()
},
async query () {
try {
this.loading = true
const params = {
pageNum: this.page.pageNum,
pageSize: this.page.pageSize
}
const condition = this.$refs.condition
if (condition) {
Object.assign(params, condition.formData)
params.startTime = this.moment(condition.formData.time[0]).format('YYYYMMDDHHmmss')
params.endTime = this.moment(condition.formData.time[1]).format('YYYYMMDDHHmmss')
delete params.stime
delete params.etime
delete params.time
}
const resData = await indexApi.getSipPage(params)
if (resData.code === 0) {
this.tableData = resData.data.list
this.page.total = resData.data.total
this.page.pageNum = resData.data.pageNum
} else {
this.tableData = []
this.page.total = 0
this.page.pageNum = 1
}
} catch (error) {
this.$Message.error(error)
} finally {
this.loading = false
}
},
changePage (no) {
this.page.pageNum = no
this.query()
},
changeSize (size) {
this.page.pageSize = size
this.query()
},
async exportData () {
if (this.selectedIds.length <= 0) return this.$Message.warning('请至少选择一条要导出的数据!')
try {
this.loading = true
const params = {
pageNum: this.page.pageNum,
pageSize: this.page.pageSize
}
const condition = this.$refs.condition
if (condition) {
Object.assign(params, condition.formData)
}
const resData = await indexApi.downloadSipData(params)
if (resData) {
const fileName = 'sip'
util.exportExcel(resData, fileName, '.xlsx')
} else {
this.$Message.error('导出失败!')
}
} catch (error) {
this.$Message.error(error)
} finally {
this.loading = false
}
},
doEdit (data, flag) {
this.detailFlag = flag
let id = null
if (!this.detailFlag) {
this.modalTitle = '会话'
id = data.sid
} else {
this.modalTitle = '详情'
id = data.id
}
this.showModal = true
this.$nextTick(() => {
if (!this.detailFlag) {
this.$refs.sip.queryDataById(id)
} else {
this.$refs.messageDetails.queryDataById(id)
}
})
},
selectChange (e) {
this.selectedIds = []
if (e.length > 0) {
e.map(item => {
this.selectedIds.push(item.id)
})
}
}
}
}
</script>
<style lang="less">
.sip-table {
.ivu-dropdown-rel {
position: relative;
left: 5px;
}
}
</style>
<style lang="less" scoped>
@import url(../../../../assets/css/var.less);
.wrap {
display: flex;
height: calc(100vh - 150px);
flex-direction: column;
.icon {
margin-right: 5px;
}
.table-info {
padding: 10px 20px;
background-color: @BGE;
border-radius: 5px;
margin-bottom: 10px;
.table-button {
display: flex;
margin: 0 0 10px 0;
align-items: center;
justify-content: flex-end;
}
.page {
display: flex;
justify-content: flex-end;
margin-top: 10px;
}
}
}
</style>