- java 同步redis到mysql_Yii2 redis同步数据到mysql
兰艳知己
java同步redis到mysql
将redis数据写入mysql中:本次案例讲解将如何将商城中商品浏览次数通过缓存记录并写入mysql中具体的redis安装过程暂且就省略了.....一、安装redis插件|配置rediscomposerrequireyiisoft/yii2-redis找到common的config文件,在components下加入redis配置参数'redis'=>['class'=>'yii\redis\Con
- linux环境下tomcat安装
M.za
linuxtomcat运维服务器
Tomcat一、什么是Tomcat?1.1、Tomcat介绍Tomcat又叫ApacheTomcat最早是sun公司开发的,1999年捐献给apache基金会,隶属于雅加达项目,现在已经独立成一个顶级项目,因为tomcat技术先进,性能稳定,又是一个开源的web应用服务器,所以很多企业都在使用,很多Java开发者也在使用,开发调试jsp的首选,被更多企业用于Java容器。Tomcat官网:http
- redis做同步或异步队列
瞧着不像好人呐
redisredisjava数据库
redis实现队列主要是使用数据结构中的list,相当于Java中的ArrayList因为它是按照塞入顺序排序的结构,我们就可以按照左边塞入,右边取出的方式来实现先入先出的队列需求。publicvoidrpush(Stringkey,Stringvalue){Jedisjedis=null;try{jedis=jedisPool.getResource();jedis.rpush(key,valu
- 企业内网系统:从传统开发到智能赋能的进化之路
飞算JavaAI开发助手
科技人工智能大数据java
在当今数字化浪潮中,企业内网系统作为支撑日常运营的核心基础设施,其开发效率与质量直接关系到企业的竞争力。传统开发模式下,程序员需要手动完成需求分析、架构设计、代码编写、测试调试等全流程工作,不仅耗时费力,还容易因人为疏忽导致质量隐患。而随着人工智能技术的突破性进展,以飞算JavaAI为代表的智能开发工具正在重塑企业内网系统的开发范式,为程序员提供从设计到落地的全链路智能支持。一、传统企业内网系统开
- 钉钉企业应用开发系列:前端实现钉钉扫码登录功能
脑袋大大的
钉钉生态创业者专栏钉钉前端第三方登录
本文将围绕“钉钉扫码登录”这一功能点展开讲解,并结合前端技术栈(HTML+JavaScript+Vue3)进行实现。我们将通过调用钉钉开放平台提供的JSAPI来实现扫码登录的功能,并展示完整的代码示例。一、前置准备1.注册钉钉开发者账号并创建企业应用访问钉钉开放平台。创建一个企业内部应用或第三方企业应用。获取corpId和redirect_uri等信息,用于后续配置。2.获取扫码登录权限确保你的应
- Java基础学习笔记2
qichi333
学习笔记javaeclipse
今天是Java基础学习第二天,加油!!!下面是我今天记的一些笔记。(有点懒惰了,爬虫今天没学,因为赖床了(bushi),但我会勤奋起来的^_^,一定一定!明天不能偷懒了天!!)一、运算符例子:inta=10;intb=20;intc=a+b;其中,“+”是运算符,且是算术运算符;“a+b”是表达式,且是算术表达式。1.算术运算符例1:publicclassdemo3{publicstaticvoi
- Spring框架中的Component与Bean注解
SpringBoot中的@Bean与@Component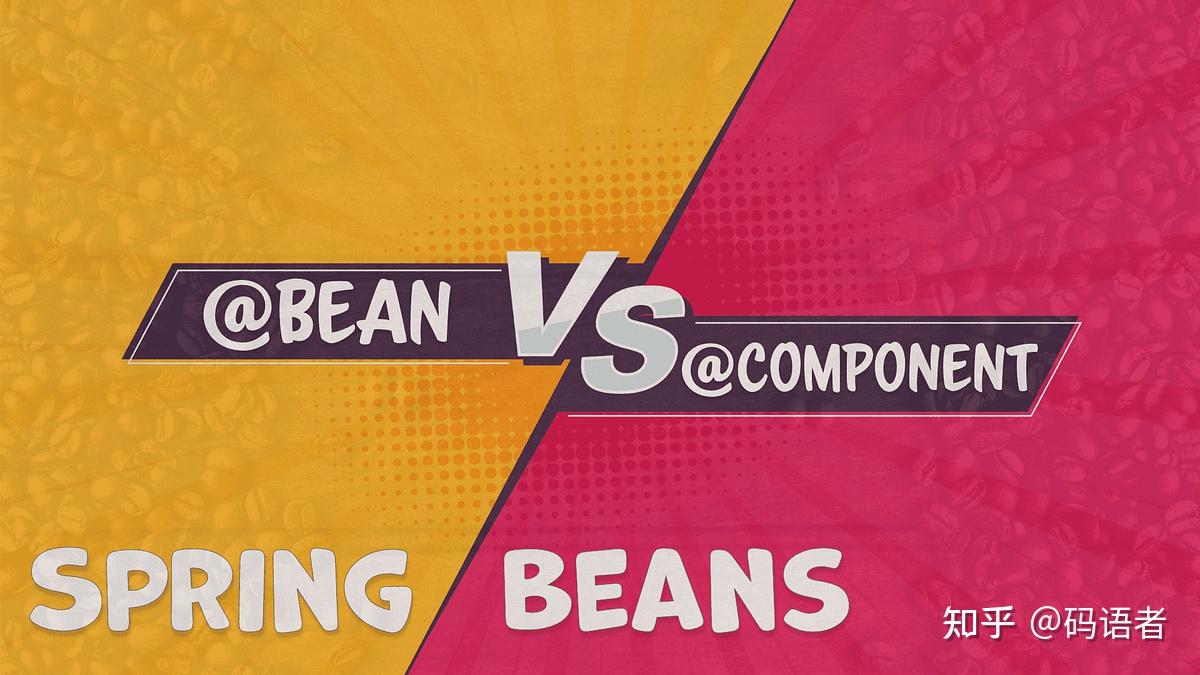Spring的@Component和@Bean注解的关键区别在于:@Bean注解可用于暴露您自己编写的JavaBeans,而@Component注解可用于暴露源代码由他人维护的JavaBeans。Sprin
- JavaScript中的系统对话框:alert、confirm、prompt
JavaScript中的系统对话框:alert、confirm、prompt在Web开发的世界里,JavaScript始终扮演着“桥梁”的角色——它连接用户与网页,让静态的页面焕发活力。而在这座桥梁上,系统对话框(SystemDialogs)是最基础却最实用的工具之一。它们像是一位贴心的助手,在用户需要确认、提示或输入时,悄然出现,又在任务完成后无声退场。今天,我们就来聊聊JavaScript中三
- Vue3 面试不再慌:这 8 个问题答得好,基本稳了!
掘金安东尼
vue.js前端javascript
面试Vue3岗位,真的只是复习CompositionAPI、生命周期和响应式吗?你以为自己准备得差不多了,但一上来,面试官问的是:“Vue3中的响应式是怎么实现的?和Vue2有什么本质不同?”——你还在“setup里写逻辑就完事了”的认知,可能就被这一问击穿了。作为Vue开发者,这些年我面过别人,也被别人面过。今天就来整理一份Vue3面试高频问题+高质量回答,不止是应试,更是一次知识体系的整理升级
- 品诺维新硬件实习生试题解析与答案
本文还有配套的精品资源,点击获取简介:本文档为苏州品诺维新公司硬件开发实习生面试准备材料。包含了三极管工作状态相关的面试题目及其解析,三极管的三种工作状态(截止、放大、饱和)被详细解释,并指出正确答案。考生需深入理解三极管的工作原理,这不仅是电子技术的基础理论,也是实际电路设计与故障排查的基础。通过理解三极管特性,可以更好地应用于开关电路、放大电路及模拟数字转换等场景。考生在准备面试时,应全面复习
- 【Flutter】面试记录
古希腊被code拿捏的神
flutter面试职场和发展
本文部分内容参考博文目录Flutter三棵树渲染原理渲染原理三者之间的关系参数位置参数mixin、extends和implementsmixin(混入)extends(继承)implements(实现)Flutter如何与Native通信的?如何从Flutter传递一个dart类到原生?常用的三种状态管理框架provider的渲染机制二叉树遍历递归与迭代什么是迭代?什么是递归?(怎么写一个递归函数
- SpringBoot+AOP+自定义注解,实现日志记录
一.定义自定义注解importjava.lang.annotation.*;/***@authorawen*定义注解目的想让他当作切点*/@Target({ElementType.METHOD})@Retention(RetentionPolicy.RUNTIME)//.java.class字节码@Documentedpublic@interfaceLog{/***处理类型**@return{@l
- 2021面试题——js面试题总结
yizhangzzzz
js面试题总结1.js的typeof返回哪些数据类型?alert(typeofnull)//objectalert(typeofundefined)//undefinedalert(typeofNaN)//numberalert(NaN==undefined)//falsealert(NaN==NaN)//falsevarstr="123abc"alert(typeofstr++)//number
- Android Java 版本与 Gradle 版本兼容问题:use incompatible Java 21.0.3 and Gradle 7.2
我命由我12345
Android-问题清单androidjava开发语言安卓androidruntimeandroidjetpackjava-ee
在AndroidStudio中,打开项目时,出现如下错误信息YourbuildiscurrentlyconfiguredtouseincompatibleJava21.0.3andGradle7.2.Cannotsynctheproject.WerecommendupgradingtoGradleversion8.9.TheminimumcompatibleGradleversionis8.5.T
- 有效避免 Cannot read property ‘xxx‘ of undefined 这类运行时错误。避免因数据字段缺失导致的报错
@Dream_Chaser
vue前端前端javascript开发语言
hasOwnProperty方法是JavaScript对象的内置方法用于检测对象自身(非原型链)是否包含指定属性返回布尔值(true/false)constfetchedData={"order":"21570921","orderType":"1",//其他属性...};constitem={value:"orderType"//我们想检查fetchedData是否有这个属性};if(fet
- 04_JavaWeb回顾笔记
skping-go
javajavaweb
JavaWeb回顾笔记[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-Wh1nKopi-1605668744709)(F:\资料\Java\笔记\笔记\assets\javaweb阶段知识体系.png)]Day01HTML1.1HTML简介HTML:HyperTextMarkupLanguage,超文本标记/标签语言。超文本:超出了普通文本的能力标记:标签W3C(Wo
- Django核心知识点详解:JSON、AJAX、Cookie、Session与用户认证
PythonicCC
djangojsonajax
1.JSON数据格式详解1.1什么是JSON?JSON(JavaScriptObjectNotation)是一种轻量级的数据交换格式,具有以下特点:独立于语言,几乎所有编程语言都支持易于人阅读和编写易于机器解析和生成基于文本,比XML更简洁1.2JSON基本格式对象格式{"name":"rose","age":20}使用大括号{}包裹键值对形式,键必须用双引号包裹多个键值对用逗号分隔数组格式["j
- 前端面试的话术集锦第 25 篇博文——CSS面试题上
互联网全栈开发实战
面试专栏-前端后端面试前端面试css跳槽职场和发展职场发展求职招聘
这是记录前端面试的话术集锦第二十五篇博文——CSS面试题上,我会不断更新该博文。❗❗❗1.介绍一下标准的CSS的盒子模型?与低版本IE的盒子模型有什么不同的?标准盒子模型:宽度=内容的宽度(content)+border+padding+margin低版本IE盒子模型:宽度=内容宽度(content+border+padding)+margin2.box-sizing属性用来控制元素的盒子模型的解
- 经典JS面试题——数组去重
MonsterQy
javascript前端开发语言
文章目录一、双指针二、filter方法三、includes四、indexOf五、reduce()六、set一、双指针基本思想:遍历数组,两层for循环比较元素是否相等,相等就删除重复元素。代码如下for(vari=0;i{returnarr.indexOf(value)==index})三、includes基本思想:利用includes方法去判断新声明的数组中是否已存在待操作的元素。vararr=
- Kotlin JVM 注解详解
前言Kotlin作为一门现代JVM语言,提供了出色的Java互操作性。为了更好地支持与Java代码的交互,Kotlin提供了一系列JVM相关注解。这些注解不仅能帮助我们控制Kotlin代码编译成Java字节码的行为,还能让我们的Kotlin代码更好地被Java代码调用。虽然在日常开发中我们最常用的是@JvmOverloads、@JvmStatic、@JvmName和@JvmField这几个注解,但
- 使用ENO将您的JSON对象生成HTML显示
土族程序员
jsonhtmljavascripteno前端
ENO是简单易用,性能卓越,自由灵活开源的WEB前端组件;实现JSON与HTML互操作的JavaScript函数库。没有任何其它依赖,足够轻量。WEBPackNPM工程安装。npminstall@joyzl/eno然后在JS中引用import"@joyzl/eno";将JS实体对象填充到表单假设有一个如下的HTML表单TYPE1TYPE2通过以下代码将实体对象,设置到表单中,实体对象可以从服务器请
- Java程序设计(二十七):基于SSM框架的OA办公自动化管理平台的设计与实现
人工智能_SYBH
2025年java程序设计java数据挖掘开发语言vue.js后端人工智能springboot
1.项目概述办公自动化(OA,OfficeAutomation)管理平台是企业实现内部管理信息化的重要工具。本文提出并实现了一个基于Java的OA办公自动化管理平台。该平台基于SSM架构(Spring+SpringMVC+MyBatis),数据库采用MySQL,并通过HTML、CSS、JavaScript等技术实现用户界面。1.1平台功能简介平台提供了管理员、普通用户和部门三类角色,分别具有不同的
- LeetCode 1:两数之和(Two Sum)解法汇总
文章目录暴力解法/我的解法两遍哈希表一遍哈希表更多LeetCode题解暴力解法/我的解法这种办法很容易理解,就不赘述了,直接上代码首先上javapublicint[]twoSum(int[]nums,inttarget){for(inti=0;itwoSum(vector&nums,inttarget){vectorresult;vector::iteratorib=nums.begin();ve
- 新手向:实现ATM模拟系统
nightunderblackcat
开发语言javatomcatmavenintellij-ideaspringcloudspringboot
本教程将通过一个完整的ATM模拟系统项目,带你深入了解Java的核心概念和实际应用。这个ATM系统将涵盖以下功能:7.2图形用户界面使用JavaSwing或JavaFX实现图形界面:importjavax.swing.*;publicclassATMGUI{publicstaticvoidmain(String[]args){JFrameframe=newJFrame("ATM系统");//添加各
- brew java 切换_如何在Mac下配置多个Java版本
weixin_39904522
brewjava切换
说明使用工具:brewcaskbrewcask是一个用命令行管理Mac下应用的工具,提供了自动安装和卸载功能,能够自动从官网上下载并安装最新的版本,它是基于homebrew的一个增强工具。一.安装最新版的Java#如何没有安装brewcask。请执行$brewtapcaskroom/versions$brewcaskinstalljava二.安装其他版本的Java如果你需要安装其他的jdk(JDK
- brew 下载java8,mac使用brew安装Java8
homebrew不多说,java8也不多说。brew安装不上java8的例子太多了。最后的做法无非这么几个,安装openjdk版本,或者安装其他的版本,或者直接去官网装。我今天就要硬装!就要用brew硬装官网版本的java8!一.安装报错brewcaskinstallhomebrew/cask-versions/java8复制代码执行这个,然后肯定报错Error:Cask'java8'isunav
- brew java 切换_Java jdk11 在Mac上的安装和配置以及JDK多个版本之间切换
weixin_39570838
brewjava切换
1、JDK11安装1)下载JDK11wgethttps://download.java.net/java/GA/jdk11/13/GPL/openjdk-11.0.1_osx-x64_bin.tar.gz2)解压安装包(系统中默认安装位置:/Library/Java/JavaVirtualMachines/)sudotar-zxfopenjdk-11.0.1_osx-x64_bin.tar.gz-
- JVM内存泄漏与内存溢出:原理详解与实战应对策略
一、核心概念深度解析内存问题一直是Java开发者面临的重要挑战,理解内存泄漏和内存溢出的本质区别是解决这类问题的第一步。1.1内存泄漏(MemoryLeak)定义:当应用程序不再需要某些对象时,由于仍然存在对这些对象的引用,导致垃圾收集器(GC)无法回收这些内存空间。关键特征:渐进式发展,如同慢性病通常由编码缺陷引起最终可能导致内存溢出1.2内存溢出(OutOfMemoryError)定义:是内存
- JSZip 使用详解
啃火龙果的兔子
开发DEMO前端javascript
JSZip使用详解JSZip是一个用于创建、读取和编辑ZIP文件的JavaScript库,完全在浏览器中运行,也支持Node.js环境。安装浏览器环境Node.js环境npminstalljszip#或yarnaddjszip基本使用1.创建一个ZIP文件constJSZip=require("jszip");//Node.js中需要constzip=newJSZip();//添加文本文件zip.
- Mammoth.js 使用详解
啃火龙果的兔子
开发DEMO前端javascript
Mammoth.js使用详解Mammoth.js是一个用于将Word文档(.docx)转换为HTML或Markdown的JavaScript库,支持浏览器和Node.js环境。安装浏览器环境Node.js环境npminstallmammoth#或yarnaddmammoth基本使用1.将DOCX转换为HTML//浏览器中使用input[type=file]获取文件document.getEleme
- 辗转相处求最大公约数
沐刃青蛟
C++漏洞
无言面对”江东父老“了,接触编程一年了,今天发现还不会辗转相除法求最大公约数。惭愧惭愧!
为此,总结一下以方便日后忘了好查找。
1.输入要比较的两个数a,b
忽略:2.比较大小(因为后面要的是大的数对小的数做%操作)
3.辗转相除(用循环不停的取余,如a%b,直至b=0)
4.最后的a为两数的最大公约数
&
- F5负载均衡会话保持技术及原理技术白皮书
bijian1013
F5负载均衡
一.什么是会话保持? 在大多数电子商务的应用系统或者需要进行用户身份认证的在线系统中,一个客户与服务器经常经过好几次的交互过程才能完成一笔交易或者是一个请求的完成。由于这几次交互过程是密切相关的,服务器在进行这些交互过程的某一个交互步骤时,往往需要了解上一次交互过程的处理结果,或者上几步的交互过程结果,服务器进行下
- Object.equals方法:重载还是覆盖
Cwind
javagenericsoverrideoverload
本文译自StackOverflow上对此问题的讨论。
原问题链接
在阅读Joshua Bloch的《Effective Java(第二版)》第8条“覆盖equals时请遵守通用约定”时对如下论述有疑问:
“不要将equals声明中的Object对象替换为其他的类型。程序员编写出下面这样的equals方法并不鲜见,这会使程序员花上数个小时都搞不清它为什么不能正常工作:”
pu
- 初始线程
15700786134
暑假学习的第一课是讲线程,任务是是界面上的一条线运动起来。
既然是在界面上,那必定得先有一个界面,所以第一步就是,自己的类继承JAVA中的JFrame,在新建的类中写一个界面,代码如下:
public class ShapeFr
- Linux的tcpdump
被触发
tcpdump
用简单的话来定义tcpdump,就是:dump the traffic on a network,根据使用者的定义对网络上的数据包进行截获的包分析工具。 tcpdump可以将网络中传送的数据包的“头”完全截获下来提供分析。它支 持针对网络层、协议、主机、网络或端口的过滤,并提供and、or、not等逻辑语句来帮助你去掉无用的信息。
实用命令实例
默认启动
tcpdump
普通情况下,直
- 安卓程序listview优化后还是卡顿
肆无忌惮_
ListView
最近用eclipse开发一个安卓app,listview使用baseadapter,里面有一个ImageView和两个TextView。使用了Holder内部类进行优化了还是很卡顿。后来发现是图片资源的问题。把一张分辨率高的图片放在了drawable-mdpi文件夹下,当我在每个item中显示,他都要进行缩放,导致很卡顿。解决办法是把这个高分辨率图片放到drawable-xxhdpi下。
&nb
- 扩展easyUI tab控件,添加加载遮罩效果
知了ing
jquery
(function () {
$.extend($.fn.tabs.methods, {
//显示遮罩
loading: function (jq, msg) {
return jq.each(function () {
var panel = $(this).tabs(&
- gradle上传jar到nexus
矮蛋蛋
gradle
原文地址:
https://docs.gradle.org/current/userguide/maven_plugin.html
configurations {
deployerJars
}
dependencies {
deployerJars "org.apache.maven.wagon
- 千万条数据外网导入数据库的解决方案。
alleni123
sqlmysql
从某网上爬了数千万的数据,存在文本中。
然后要导入mysql数据库。
悲剧的是数据库和我存数据的服务器不在一个内网里面。。
ping了一下, 19ms的延迟。
于是下面的代码是没用的。
ps = con.prepareStatement(sql);
ps.setString(1, info.getYear())............;
ps.exec
- JAVA IO InputStreamReader和OutputStreamReader
百合不是茶
JAVA.io操作 字符流
这是第三篇关于java.io的文章了,从开始对io的不了解-->熟悉--->模糊,是这几天来对文件操作中最大的感受,本来自己认为的熟悉了的,刚刚在回想起前面学的好像又不是很清晰了,模糊对我现在或许是最好的鼓励 我会更加的去学 加油!:
JAVA的API提供了另外一种数据保存途径,使用字符流来保存的,字符流只能保存字符形式的流
字节流和字符的难点:a,怎么将读到的数据
- MO、MT解读
bijian1013
GSM
MO= Mobile originate,上行,即用户上发给SP的信息。MT= Mobile Terminate,下行,即SP端下发给用户的信息;
上行:mo提交短信到短信中心下行:mt短信中心向特定的用户转发短信,你的短信是这样的,你所提交的短信,投递的地址是短信中心。短信中心收到你的短信后,存储转发,转发的时候就会根据你填写的接收方号码寻找路由,下发。在彩信领域是一样的道理。下行业务:由SP
- 五个JavaScript基础问题
bijian1013
JavaScriptcallapplythisHoisting
下面是五个关于前端相关的基础问题,但却很能体现JavaScript的基本功底。
问题1:Scope作用范围
考虑下面的代码:
(function() {
var a = b = 5;
})();
console.log(b);
什么会被打印在控制台上?
回答:
上面的代码会打印 5。
&nbs
- 【Thrift二】Thrift Hello World
bit1129
Hello world
本篇,不考虑细节问题和为什么,先照葫芦画瓢写一个Thrift版本的Hello World,了解Thrift RPC服务开发的基本流程
1. 在Intellij中创建一个Maven模块,加入对Thrift的依赖,同时还要加上slf4j依赖,如果不加slf4j依赖,在后面启动Thrift Server时会报错
<dependency>
- 【Avro一】Avro入门
bit1129
入门
本文的目的主要是总结下基于Avro Schema代码生成,然后进行序列化和反序列化开发的基本流程。需要指出的是,Avro并不要求一定得根据Schema文件生成代码,这对于动态类型语言很有用。
1. 添加Maven依赖
<?xml version="1.0" encoding="UTF-8"?>
<proj
- 安装nginx+ngx_lua支持WAF防护功能
ronin47
需要的软件:LuaJIT-2.0.0.tar.gz nginx-1.4.4.tar.gz &nb
- java-5.查找最小的K个元素-使用最大堆
bylijinnan
java
import java.util.Arrays;
import java.util.Random;
public class MinKElement {
/**
* 5.最小的K个元素
* I would like to use MaxHeap.
* using QuickSort is also OK
*/
public static void
- TCP的TIME-WAIT
bylijinnan
socket
原文连接:
http://vincent.bernat.im/en/blog/2014-tcp-time-wait-state-linux.html
以下为对原文的阅读笔记
说明:
主动关闭的一方称为local end,被动关闭的一方称为remote end
本地IP、本地端口、远端IP、远端端口这一“四元组”称为quadruplet,也称为socket
1、TIME_WA
- jquery ajax 序列化表单
coder_xpf
Jquery ajax 序列化
checkbox 如果不设定值,默认选中值为on;设定值之后,选中则为设定的值
<input type="checkbox" name="favor" id="favor" checked="checked"/>
$("#favor&quo
- Apache集群乱码和最高并发控制
cuisuqiang
apachetomcat并发集群乱码
都知道如果使用Http访问,那么在Connector中增加URIEncoding即可,其实使用AJP时也一样,增加useBodyEncodingForURI和URIEncoding即可。
最大连接数也是一样的,增加maxThreads属性即可,如下,配置如下:
<Connector maxThreads="300" port="8019" prot
- websocket
dalan_123
websocket
一、低延迟的客户端-服务器 和 服务器-客户端的连接
很多时候所谓的http的请求、响应的模式,都是客户端加载一个网页,直到用户在进行下一次点击的时候,什么都不会发生。并且所有的http的通信都是客户端控制的,这时候就需要用户的互动或定期轮训的,以便从服务器端加载新的数据。
通常采用的技术比如推送和comet(使用http长连接、无需安装浏览器安装插件的两种方式:基于ajax的长
- 菜鸟分析网络执法官
dcj3sjt126com
网络
最近在论坛上看到很多贴子在讨论网络执法官的问题。菜鸟我正好知道这回事情.人道"人之患好为人师" 手里忍不住,就写点东西吧. 我也很忙.又没有MM,又没有MONEY....晕倒有点跑题.
OK,闲话少说,切如正题. 要了解网络执法官的原理. 就要先了解局域网的通信的原理.
前面我们看到了.在以太网上传输的都是具有以太网头的数据包. 
- Android相对布局属性全集
dcj3sjt126com
android
RelativeLayout布局android:layout_marginTop="25dip" //顶部距离android:gravity="left" //空间布局位置android:layout_marginLeft="15dip //距离左边距
// 相对于给定ID控件android:layout_above 将该控件的底部置于给定ID的
- Tomcat内存设置详解
eksliang
jvmtomcattomcat内存设置
Java内存溢出详解
一、常见的Java内存溢出有以下三种:
1. java.lang.OutOfMemoryError: Java heap space ----JVM Heap(堆)溢出JVM在启动的时候会自动设置JVM Heap的值,其初始空间(即-Xms)是物理内存的1/64,最大空间(-Xmx)不可超过物理内存。
可以利用JVM提
- Java6 JVM参数选项
greatwqs
javaHotSpotjvmjvm参数JVM Options
Java 6 JVM参数选项大全(中文版)
作者:Ken Wu
Email:
[email protected]
转载本文档请注明原文链接 http://kenwublog.com/docs/java6-jvm-options-chinese-edition.htm!
本文是基于最新的SUN官方文档Java SE 6 Hotspot VM Opt
- weblogic创建JMC
i5land
weblogicjms
进入 weblogic控制太
1.创建持久化存储
--Services--Persistant Stores--new--Create FileStores--name随便起--target默认--Directory写入在本机建立的文件夹的路径--ok
2.创建JMS服务器
--Services--Messaging--JMS Servers--new--name随便起--Pers
- 基于 DHT 网络的磁力链接和BT种子的搜索引擎架构
justjavac
DHT
上周开发了一个磁力链接和 BT 种子的搜索引擎 {Magnet & Torrent},本文简单介绍一下主要的系统功能和用到的技术。
系统包括几个独立的部分:
使用 Python 的 Scrapy 框架开发的网络爬虫,用来爬取磁力链接和种子;
使用 PHP CI 框架开发的简易网站;
搜索引擎目前直接使用的 MySQL,将来可以考虑使
- sql添加、删除表中的列
macroli
sql
添加没有默认值:alter table Test add BazaarType char(1)
有默认值的添加列:alter table Test add BazaarType char(1) default(0)
删除没有默认值的列:alter table Test drop COLUMN BazaarType
删除有默认值的列:先删除约束(默认值)alter table Test DRO
- PHP中二维数组的排序方法
abc123456789cba
排序二维数组PHP
<?php/*** @package BugFree* @version $Id: FunctionsMain.inc.php,v 1.32 2005/09/24 11:38:37 wwccss Exp $*** Sort an two-dimension array by some level
- hive优化之------控制hive任务中的map数和reduce数
superlxw1234
hivehive优化
一、 控制hive任务中的map数: 1. 通常情况下,作业会通过input的目录产生一个或者多个map任务。 主要的决定因素有: input的文件总个数,input的文件大小,集群设置的文件块大小(目前为128M, 可在hive中通过set dfs.block.size;命令查看到,该参数不能自定义修改);2. 
- Spring Boot 1.2.4 发布
wiselyman
spring boot
Spring Boot 1.2.4已于6.4日发布,repo.spring.io and Maven Central可以下载(推荐使用maven或者gradle构建下载)。
这是一个维护版本,包含了一些修复small number of fixes,建议所有的用户升级。
Spring Boot 1.3的第一个里程碑版本将在几天后发布,包含许多