统计字符串中字母、数字、空格和其他字符的个数
题目举例:
编写一个函数void StatCharCount(char str[]),该函数接收一个字符串作为参数,请统计该字符串中字母、数字、空格和其他字符的个数,在main函数调用该函数,分别打印字母、数字、空格 和 其他字符的出现次数。
返回提示:一个函数只能返回一个值,此处的统计结果有4个,因此返回结果可以使用全局变量带出来。
写法一:
1.1函数的实现
#include
int letters = 0 , digits = 0, spaces = 0, others = 0;
void statCharCount(char str[])
{
int i;
int len = strlen(str);
for (i = 0; i < len; i++)
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'z'))
letters++;
else if (str[i] >= '0' && str[i] <= '9')
digits++;
else if (str[i] == ' ')
spaces++;
else
others++;
}
1.2实际问题代入:
#include
#include
int letters = 0 , digits = 0, spaces = 0, others = 0;
void statCharCount(char str[])
{
int i;
int len = strlen(str);
for (i = 0; i < len; i++)
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'z'))
letters++;
else if (str[i] >= '0' && str[i] <= '9')
digits++;
else if (str[i] == ' ')
spaces++;
else
others++;
}
int main()
{
char text[80]; printf("请输入一行字符串\n");
gets(text);
StatCharCount(text);
printf("统计结果如下:\n");
printf("letters: %d\n", letters);
printf("digits: %d\n", digits);
printf("spaces: %d\n", spaces);
printf("others: %d\n", others);
return 0;
}
1.3结果举例:
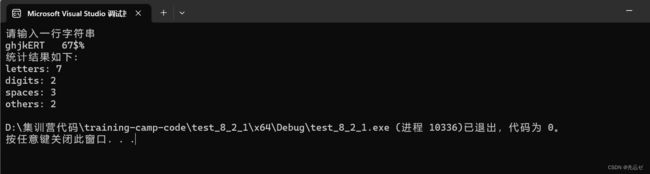
写法二:
2.1函数的实现
int letters = 0, digits = 0, spaces = 0, others = 0;
void StatCharCount(char str[])
{
for (int i = 0; str[i] != '\0'; i++)
{
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z'))
letters++;
else if (str[i] >= '0' && str[i] <= '9')
digits++;
else if (str[i] == ' ')
spaces++;
else
others++;
}
}
2.2实际问题代入
#include
int letters = 0, digits = 0, spaces = 0, others = 0;
void StatCharCount(char str[])
{
for (int i = 0; str[i] != '\0'; i++)
{
if ((str[i] >= 'a' && str[i] <= 'z') || (str[i] >= 'A' && str[i] <= 'Z'))
letters++;
else if (str[i] >= '0' && str[i] <= '9')
digits++;
else if (str[i] == ' ')
spaces++;
else
others++;
}
}
int main()
{
char str[1000] = "\0";
printf("请输入字符串:");
int j = 0;
gets(str);
StatCharCount(str);
printf("该字符串中:\n");
printf("字母有:%d \n数字有:%d \n空格有:%d \n其他符号有: %d ", letters, digits, spaces, others);
return 0;
}
2.3结果举例:
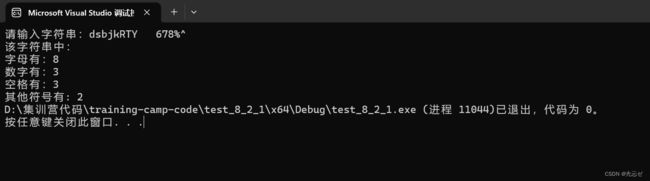
感谢观看,制作不易,可以一键三连吗,谢谢您!!!