文章目录
- 1.迷宫中离入口最近的出口
- 2.最小基因变化
- 3.单词接龙
- 4.为高尔夫比赛砍树
1.迷宫中离入口最近的出口
迷宫中离入口最近的出口
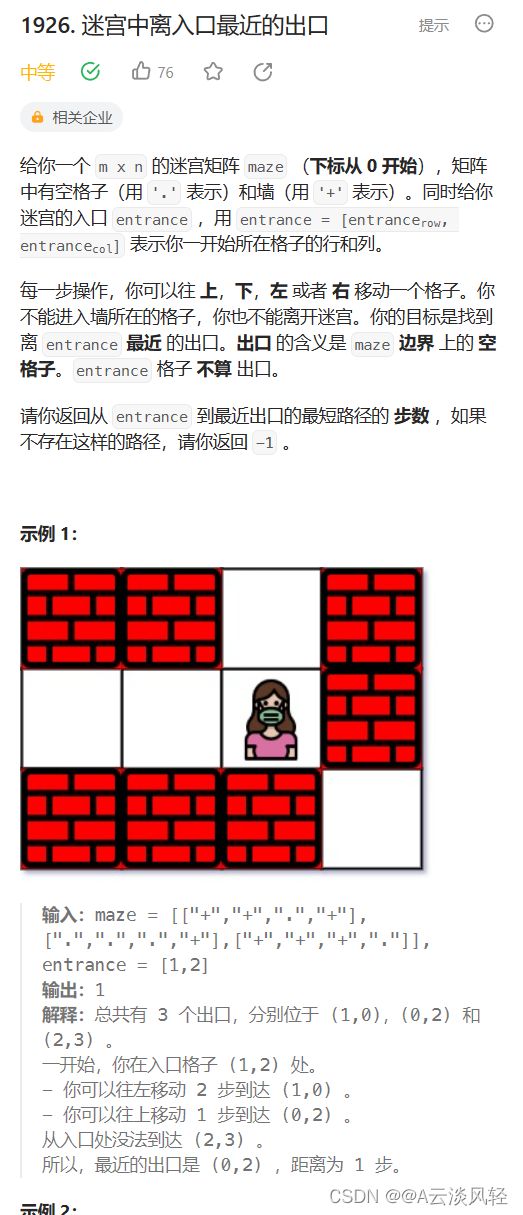
class Solution {
int dx[4] = {0,0,1,-1};
int dy[4] = {1,-1,0,0};
bool vis[101][101];
public:
int nearestExit(vector<vector<char>>& maze, vector<int>& e) {
int m = maze.size(), n = maze[0].size();
queue<pair<int,int>> q;
q.push({e[0],e[1]});
vis[e[0]][e[1]] = true;
int step = 0;
while(q.size())
{
step++;
int sz = q.size();
while(sz--)
{
auto [a,b] = q.front();
q.pop();
for(int k=0;k<4;k++)
{
int x = a+dx[k],y = b+dy[k];
if(x>=0 && x<m && y>=0 && y<n && maze[x][y]=='.'&& !vis[x][y])
{
if(x==0 || x==m-1 || y==0 || y==n-1) return step;
q.push({x,y});
vis[x][y] = true;
}
}
}
}
return -1;
}
};
2.最小基因变化
最小基因变化

class Solution {
public:
int minMutation(string startGene, string endGene, vector<string>& bank) {
unordered_set<string> vis;
unordered_set<string> hash(bank.begin(),bank.end());
string change = "ACGT";
if(startGene == endGene) return 0;
if(!hash.count(endGene)) return -1;
int ret = 0;
queue<string> q;
q.push(startGene);
vis.insert(startGene);
while(q.size())
{
ret++;
int sz = q.size();
while(sz--)
{
auto t = q.front();
q.pop();
for(int i=0;i<8;i++)
{
string tmp = t;
for(int j=0;j<4;j++)
{
tmp[i] = change[j];
if(hash.count(tmp) && !vis.count(tmp))
{
if(tmp == endGene) return ret;
q.push(tmp);
vis.insert(tmp);
}
}
}
}
}
return -1;
}
};
3.单词接龙
单词接龙
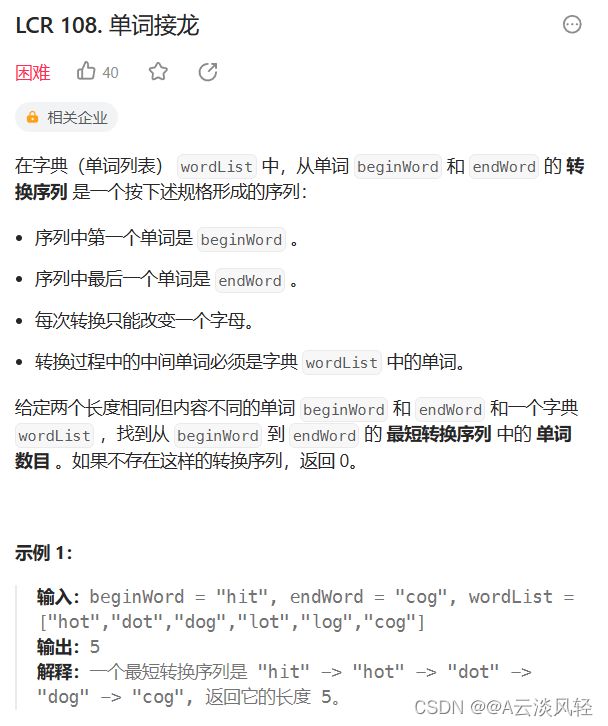
class Solution {
public:
int ladderLength(string beginWord, string endWord, vector<string>& wordList) {
unordered_set<string> vis;
unordered_set<string> hash(wordList.begin(),wordList.end());
if(!hash.count(endWord)) return 0;
queue<string> q;
q.push(beginWord);
vis.insert(beginWord);
int ret = 1;
while(q.size())
{
ret++;
int sz = q.size();
while(sz--)
{
string t = q.front();q.pop();
for(int i=0;i<t.size();i++)
{
string tmp = t;
for(char ch ='a';ch<='z';ch++)
{
tmp[i]=ch;
if(hash.count(tmp)&&!vis.count(tmp))
{
if(tmp == endWord) return ret;
q.push(tmp);
vis.insert(tmp);
}
}
}
}
}
return 0;
}
};
4.为高尔夫比赛砍树
为高尔夫比赛砍树
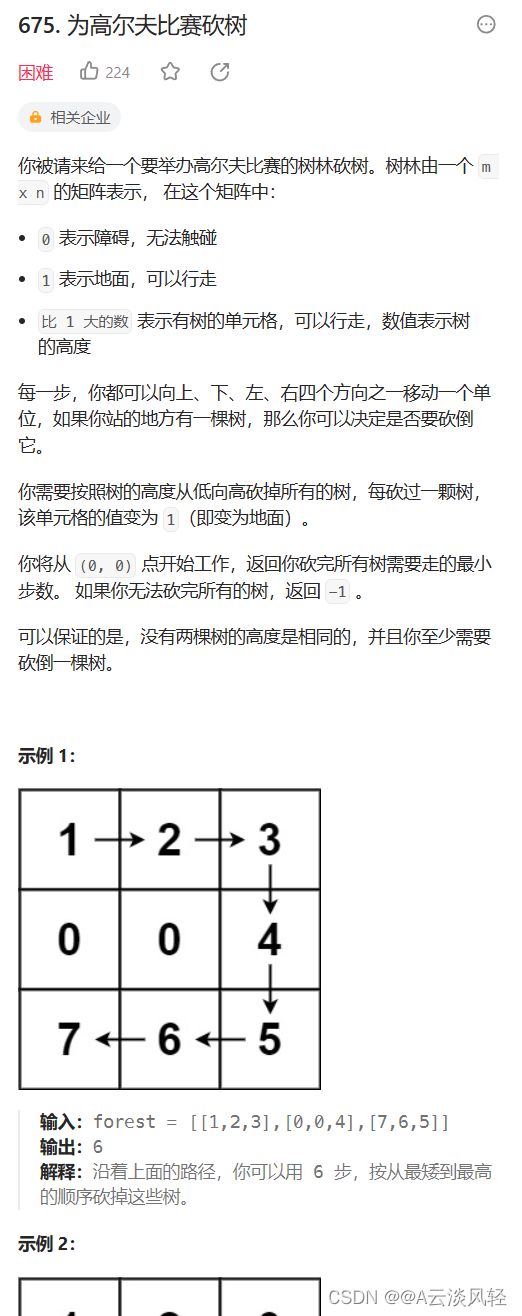
class Solution {
int dx[4] = {0,0,1,-1};
int dy[4] = {1,-1,0,0};
bool vis[51][51];
int m,n;
public:
int cutOffTree(vector<vector<int>>& f) {
m = f.size(), n = f[0].size();
vector<pair<int,int>> trees;
for(int i=0;i<m;i++)
{
for(int j=0;j<n;j++)
{
if(f[i][j]>1)
{
trees.push_back({i,j});
}
}
}
sort(trees.begin(),trees.end(),[&](const pair<int,int>& p1,const pair<int,int>& p2)
{
return f[p1.first][p1.second] < f[p2.first][p2.second];
});
int ret = 0;
int bx = 0,by = 0;
for(auto&[a,b]: trees)
{
int step = bfs(f,bx,by,a,b);
if(step == -1) return -1;
ret += step;
bx = a,by = b;
}
return ret;
}
int bfs(vector<vector<int>>& f,int bx,int by,int x1,int y1)
{
if(bx == x1 && by == y1) return 0;
queue<pair<int,int>> q;
memset(vis,0,sizeof vis);
q.push({bx,by});
vis[bx][by] = true;
int step = 0;
while(q.size())
{
step++;
int sz = q.size();
while(sz--)
{
auto [a,b] = q.front();
q.pop();
for(int k =0;k<4;k++)
{
int x = a+dx[k] , y = b+dy[k];
if(x>=0 && x<m && y>=0 && y<n && f[x][y] && !vis[x][y])
{
if(x == x1&& y == y1 ) return step;
q.push({x,y});
vis[x][y] = true;
}
}
}
}
return -1;
}
};