import cv2
import os
import hashlib
file_dict = {"2017-07-04-07-39-28_fovs5.h264": (list(range(2030,3150)) + list(range(3960,4050))),
"2017-07-04-09-41-55_fovs5.h264": (list(range(1100,1700)) + list(range(2430,4450))),
"2017-07-04-09-50-22_fovs5.h264": (list(range(0,1240)) + list(range(2200,9000)) + list(range(11320,14000)))}
def getFileMD5(filepath):
f = open(filepath, 'rb')
md5obj = hashlib.md5()
md5obj.update(f.read())
hash = md5obj.hexdigest()
f.close()
return str(hash).upper()
def selct_sample(video_path,target_path,video_index,filemd5,frameFrequency,extract_num):
cap = cv2.VideoCapture(video_path)
frame_index = 0
while True:
res, image = cap.read()
if not res:
print('not res, not image')
break
if frame_index % frameFrequency == 0 and frame_index in extract_num:
image_name = ("{}_{}_{}.png".format(
(str(video_index)).zfill(4),
filemd5,
(str(frame_index)).zfill(5))
)
cv2.imwrite(os.path.join(target_path,image_name),image)
print("正在截取:{} ---> {}帧".format(os.path.basename(target_path),frame_index))
frame_index += 1
print("图片提取结束")
cap.release()
def gen_sample(video_path,target_path,video_index,filemd5,frameFrequency):
cap = cv2.VideoCapture(video_path)
frame_index = 0
while True:
res, image = cap.read()
if not res:
print('not res, not image')
break
if frame_index % frameFrequency == 0:
image_name = ("{}_{}_{}.png".format(
(str(video_index)).zfill(4),
filemd5,
(str(frame_index)).zfill(5))
)
cv2.imwrite(os.path.join(target_path,image_name),image)
print("正在截取:{} ---> {}帧".format(os.path.basename(target_path),frame_index))
frame_index += 1
print("图片提取结束")
cap.release()
def main():
src_path = r'D:/Desktop/fovs5_rc/02/'
target_path = r'D:/Desktop/fovs5_rc/02/'
frameFrequency = 10
filelist = os.listdir(src_path)
video_num = len(filelist)
print("视频个数为:%d" % video_num)
for item in filelist:
video_index = 0
if item.endswith(('.h264','.mp4','.mkv','avi')):
video_path = os.path.join(src_path,item)
filemd5 = getFileMD5(video_path)
filename = item.split(".")[0]
outPutDir = os.path.join(target_path,filename)
if not os.path.exists(outPutDir):
os.mkdir(outPutDir)
gen_sample(video_path,outPutDir,video_index,filemd5,frameFrequency)
video_index += 1
if __name__ == '__main__':
main()
- 通过读取视频的md5值能够保证在批量处理视频文件时,生成的图片文件名不会有重复的情况,图片的命名可以根据实际情况添加相应的标签
- select_sample支持提取视频中特定帧数段的图片,使用较少
- 代码运行过程中会打印相应的信息
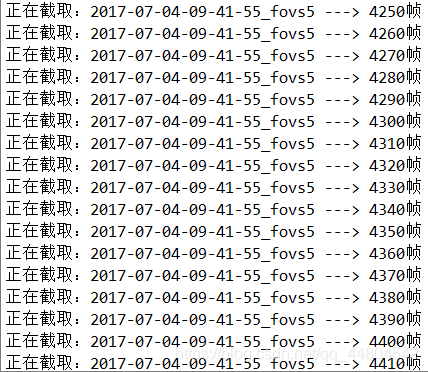
- 代码运行结束后,会在对应的目标文件夹下,按照设置的隔间帧数生成对应的文件,如设置每隔10帧取一帧,将得到如下结果:
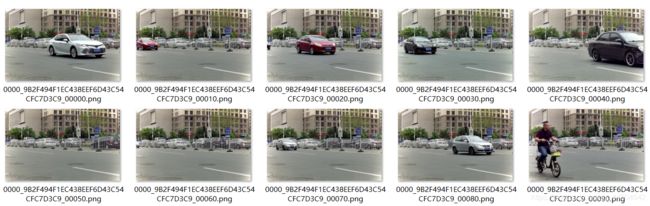